1. A brief overview of six point cloud feature descriptors
NARF (Normal Aligned Radial Feature) feature point descriptor : The NARF descriptor is a radial feature descriptor based on normal alignment. It extracts features by dividing the point cloud surface into small grid cells and computing the normal histogram in each cell. NARF descriptors are sensitive to curvature and shape changes of point clouds and can be used for tasks such as object detection and scene recognition .
PFH (Point Feature Histogram) and FPFH (Fast Point Feature Histogram) point feature histogram descriptors : PFH and FPFH are a type of commonly used point cloud feature descriptors. They construct histogram descriptors by computing the relative position and normal relationship between a point and other points in its neighborhood. The calculation of PFH descriptor is relatively complex, while FPFH optimizes PFH and improves the calculation efficiency. These descriptors are commonly used for tasks such as point cloud registration, recognition, and matching .
RoPs (Rotational Projection Statistics) feature : RoPs feature is a descriptor based on rotation projection statistics. It projects the point cloud onto multiple different viewpoints, and counts the projection information on each viewpoint, such as height, density, and curvature. The RoPs feature has good invariance to the rotation and scale changes of the point cloud , and can be used for tasks such as object classification and recognition .
VFH (Viewpoint Feature Histogram) viewpoint feature histogram descriptor : The VFH descriptor is a viewpoint-based feature descriptor. It computes the relationship between the relative position and normal of each point to other points in the point cloud, and builds histogram descriptors. The VFH descriptor is mainly used for point cloud recognition and classification tasks, and has good invariance to viewpoint changes .
GASD (Global Alignment of Spatial Distribution) globally aligned spatial distribution descriptor : The GASD descriptor is a global feature descriptor based on spatial distribution. It builds descriptors by projecting the point cloud into a 3D histogram and counting the distribution of the point cloud in different regions. The GASD descriptor models the global shape and distribution features of point clouds , and is often used for point cloud recognition and classification .
Descriptor based on moment of inertia and eccentricity : This descriptor is a feature descriptor based on the geometric properties of the point cloud . It characterizes the shape features of the point cloud by calculating geometric properties such as the moment of inertia and eccentricity of the point cloud. Such descriptors are commonly used for shape analysis and recognition tasks in point clouds
2. Extract PFH (Point Feature Histogram) feature descriptor
the code
#include <pcl/point_types.h>
#include <pcl/features/pfh.h>
#include <pcl/io/io.h>
#include <pcl/io/pcd_io.h>
#include <pcl/visualization/cloud_viewer.h>
#include <pcl/features/normal_3d.h>
int main(){
// 读取点云数据
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
pcl::io::loadPCDFile("/home/jason/file/pcl-learning/data/bunny.pcd", *cloud);
// --------------------
// K近邻搜索法线估计
// --------------------
pcl::PointCloud<pcl::Normal>::Ptr normals(new pcl::PointCloud<pcl::Normal>);
pcl::NormalEstimation<pcl::PointXYZ, pcl::Normal> normalEstimation;
normalEstimation.setInputCloud(cloud);
normalEstimation.setRadiusSearch(0.03);//领域半径,每个点的领域范围
pcl::search::KdTree<pcl::PointXYZ>::Ptr kdtree(new pcl::search::KdTree<pcl::PointXYZ>);
normalEstimation.setSearchMethod(kdtree);// kd树搜索每个点的领域点
normalEstimation.compute(*normals); // 用每个点的领域点估计其法线向量
// -----------------------------------------------------------------------------
// 计算PFH特征描述子
// 使用点云数据和对应的法线数据作为输入。
// 然后通过设置最近邻搜索方法和搜索半径,对每个点在其邻域内进行特征计算,生成PFH特征描述子。
// 计算结果存储在pfhs中,可以用于后续的点云处理任务
// -----------------------------------------------------------------------------
pcl::PFHEstimation<pcl::PointXYZ, pcl::Normal, pcl::PFHSignature125> pfh; // 创建PFHEstimation对象
pfh.setInputCloud(cloud); // 输入点云数据
pfh.setInputNormals(normals); // 输入法线数据
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>()); // 创建KdTree对象tree
pfh.setSearchMethod(tree); // 将tree设置为PFH估计过程中用于搜索最近邻点的方法
pcl::PointCloud<pcl::PFHSignature125>::Ptr pfhs(new pcl::PointCloud<pcl::PFHSignature125>()); // 用于存储计算得到的PFH特征描述子
pfh.setRadiusSearch(0.08); // 设置搜索半径,指定在计算每个点的PFH描述子时使用的邻域范围; 必须大与估算法向量的领域半径
pfh.compute(*pfhs); // 计算点云的PFH特征描述子
//--------------
// 打印PFH描述子
// --------------
unsigned long size = pfhs->points.size();
for (int j = 0; j < size ; ++j){
pcl::PFHSignature125 &signature125 = pfhs->points[j];
float *h = signature125.histogram;
printf("%d: %f, %f,%f \n", j, h[1], h[2], h[3]);
}
// -----------------
// 可视化法线
// ----------------
pcl::visualization::PCLVisualizer viewer("PCL Viewer");
viewer.setBackgroundColor(0.0, 0.0, 0.5);
viewer.addPointCloudNormals<pcl::PointXYZ, pcl::Normal>(cloud, normals, 1, 0.01, "normals");
while (!viewer.wasStopped()){
viewer.spinOnce();
}
return 0;
}
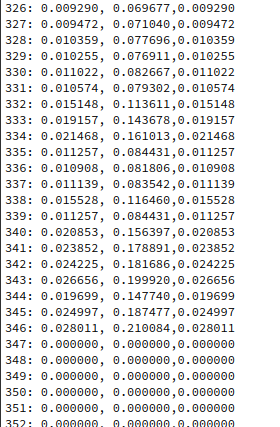
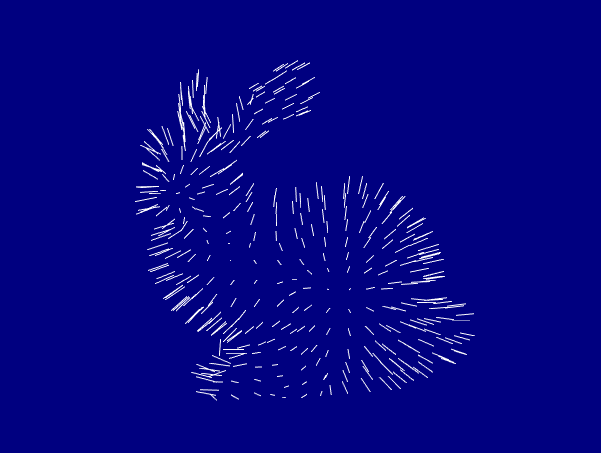