Table of contents
1. The result of the following logical expressions is false ()
2. What is the output result of the following JS code?
3. Which of the following objects are Javascript built-in iterable objects?
1. Multiple choice questions
1. The result of the following logical expressions is false ()
A、NaN == NaN
B、null == undefined
C、'' == 0
D、true == 1
Correct answer: A
Parse:
(1) Option explanation
Option A: NaN is false no matter who it is compared with, including itself . To judge that two NaNs are equal, use Object.is(NaN,NaN)
Option B: compare null with undefined, the result is true , otherwise undefined and null are compared with whom, the result is false
C option: compare String with Number, first convert String to Number type, and then compare , the result of converting to Number type string is 0==0, the result is true
D option: Boolean is compared with other types, Boolean is first converted to Number type, and the value of true to Number type is 1, so the result of true==1 is true
(2) Examples
Object.is('foo', 'foo'); // true
Object.is(window, window); // true
Object.is('foo', 'bar'); // false
Object.is([], []); // false
var foo = { a: 1 };
var bar = { a: 1 };
Object.is(foo, foo); // true
Object.is(foo, bar); // false
Object.is(null, null); // true
// 特例
Object.is(0, -0); // false
Object.is(0, +0); // true
Object.is(-0, -0); // true
Object.is(NaN, 0/0); // true
2. What is the output result of the following JS code?
let obj = {
num1: 117
}
let res = obj;
obj.child = obj = { num2: 935 };
var x = y = res.child.num2;
console.log(obj.child);
console.log(res.num1);
console.log(y);
A、117、117、undefined
B、117、117、935
C、undefined、117、935
D、undefined、117、undefined
Correct answer: C Your answer: B
Parse:
(1)let obj = { num1: 117 }
Put obj on the stack, put {num1:117} on the heap, and let obj point to {num1:117} in the heap
(2)let res = obj;
Put res on the stack and point res to { num1:117 } in the heap
(3) obj.child = obj = { num2: 935 };
① Important: The assignment operation first defines the variable ( from left to right ), and then assigns the value ( from right to left )
② Define variable: obj.child, add a child attribute to {num1:117} in the heap, get {num1:117, child:undefined}
③ Define variables: obj, the obj in the stack before
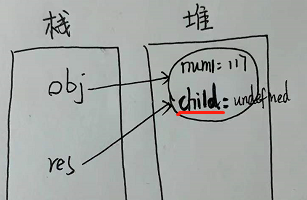
④ Assignment: obj = { num2: 935 }, put { num2: 935 } in the heap, point the obj in the stack to { num2: 935 } in the heap
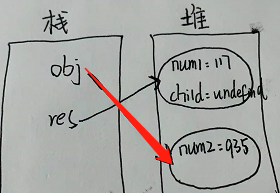
⑤ Assignment: obj.child = obj, point the child of {num1:117, child:undefined} in the heap to {num2: 935}
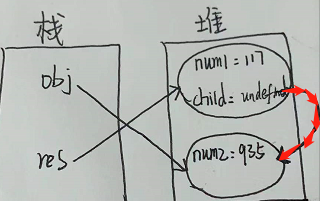
⑥Summary
obj = { num2: 935 }
res = { num1: 117,child:{ num2: 935 } }
(4)var x = y = res.child.num2;
Same as the principle of ① in step (3), the last assignment is from right to left, y = res.child.num2 ie y = 935, x = y ie x = 935
3. Which of the following objects are Javascript built-in iterable objects?
A、Array
B、Map
C、String
D、Object
Correct answer: ABC Your answer: ABCD
Parse:
(1) String, Array, TypedArray, Map and Set are all built-in iterable objects, because their prototype objects all have a Symbol.iterator method
(2) MDN reference documents
Iterators and Generators - JavaScript | MDN
2. Programming questions
1. Find the maximum value in the array parameter and return it. Note: Only numbers are included in the array
Parse:
(1) The Math.max() method finds the maximum. Note that the method cannot accept an array as a parameter, and needs to be deconstructed and assigned to deconstruct the array
<script>
let array = [23,65,12,5,34]
function _max(array){
return Math.max(...array)
}
console.log(_max(array));
</script>
(2) for() loops through the array values in turn to find the maximum value
<script>
let array = [23,65,12,5,34]
function _max(array){
let max = array[0]
for(let i =0;i<array.length;i++){
if(max<array[i]){
max = array[i]
}
}
return max
}
console.log(_max(array));
</script>
(3) The sort() method sorts the new array from large to small, and shift() returns the first value of the new array sorted
<script>
let array = [23,65,12,5,34]
function _max(array){
const newArr = array
newArr.sort((a,b)=>{
return b-a
})
return newArr.shift()
}
console.log(_max(array));
</script>
(4) The sort() method sorts the new array from small to large, and pop() returns the last value of the new array sorted
<script>
let array = [23,65,12,5,34]
function _max(array){
const newArr = array
newArr.sort((a,b)=>{
return a-b
})
return newArr.pop()
}
console.log(_max(array));
</script>
(5) The reduce() method traverses the array elements and returns the max value
<script>
let array = [23,65,12,5,34]
function _max(array){
let max = array.reduce((max,cur)=>{
return max>cur?max:cur
})
return max
}
console.log(_max(array));
</script>