Article directory
An overview of object-oriented design principles
Single Responsibility Principle
An overview of object-oriented design principles
Software maintainability (Maintainability) and reusability (Reusability) are two very important attributes used to measure software quality. Software maintainability refers to the ease with which software can be understood, corrected, adapted, and expanded. The degree of reusability of software refers to the ease with which software can be reused .
One of the object-oriented design goals is to support maintainability and reuse. On the one hand, it is necessary to realize the reuse of design schemes or source codes, and on the other hand, to ensure that the system can be easily expanded and modified, and has good maintainability.
Object-oriented design principles are also the basis for subsequent learning of design patterns. A certain design pattern conforms to one or more object-oriented design principles.
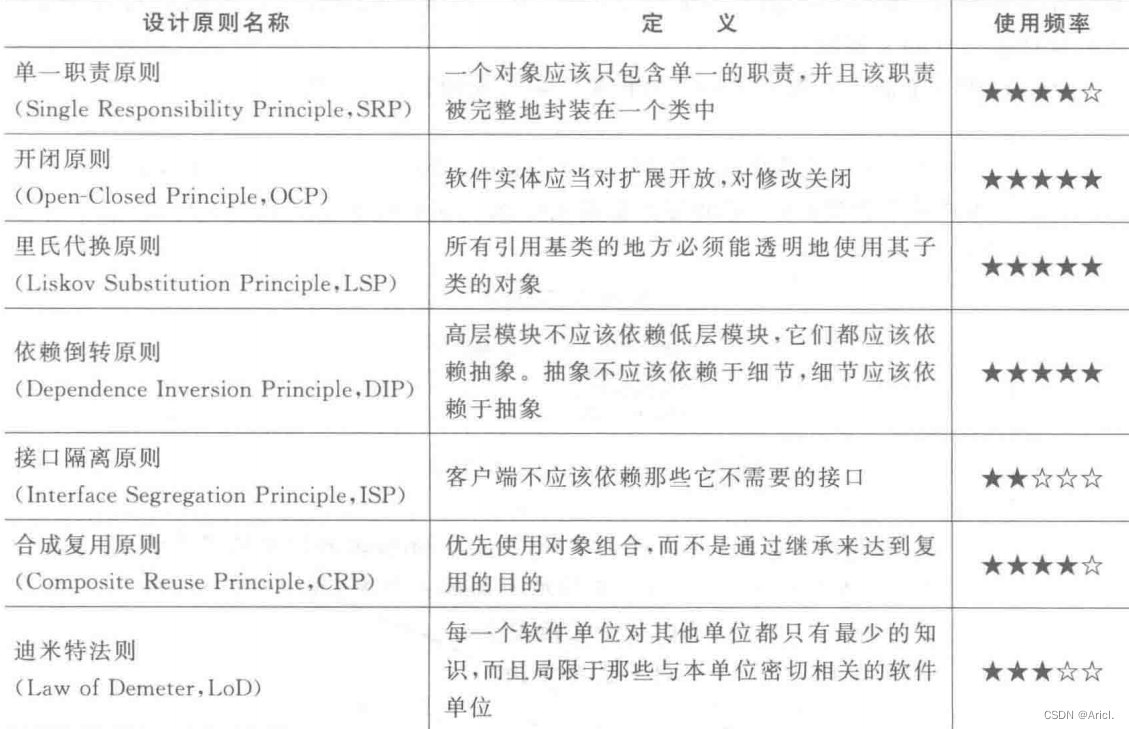
Single Responsibility Principle
overview
The Single Responsibility Principle is the simplest object-oriented design principle. It is used to control the granularity of classes . Its definition is as follows: An object should only contain a single responsibility, and this responsibility is encapsulated in a single class . Another definition is: As far as a class is concerned, there should be only one reason for it to change .
In a software system, the more responsibilities a class (from modules to methods) undertakes , the less likely it is to be reused , and a class takes too many responsibilities, which is equivalent to coupling these responsibilities together , when one of the responsibilities changes, it may affect the operation of other responsibilities, so these responsibilities should be separated , and different responsibilities should be encapsulated in different classes, that is, different reasons for changes should be encapsulated in different classes. If multiple responsibilities Always change at the same time to encapsulate them in the same class .
The single responsibility principle is a guideline to achieve high cohesion and low coupling . It is the simplest but most difficult principle to apply.
example
This design principle is the simplest and easy to understand, so the sample code is omitted.
Open and close principle
overview
The principle of opening and closing is the first cornerstone of object-oriented reusable design, and it is the most important object-oriented design principle.
The definition of the open-closed principle is: software entities should be open for extension and closed for modification . In layman's terms, the principle of opening and closing refers to the expansion of software entities without modifying the original code as much as possible.
In order to meet the principle of opening and closing, it is necessary to design the system abstractly, and abstraction is the key to the principle of opening and closing. In many object-oriented languages, mechanisms such as interfaces and abstract classes are provided , through which the abstract layer of the system can be defined , and then extended through specific classes . If there are new business requirements, it is only necessary to add new concrete classes to realize new business functions without changing the abstraction layer, so that the functions of the system can be expanded without modifying the original code, and the requirements of the open-close principle can be met .
example
the code
AbstractSkin.java
package com.Demo.Principles.开闭原则;
/**
* 抽象皮肤类
*/
public abstract class AbstractSkin {
//用于显示
public abstract void display();
}
DefaultSkin.java
package com.Demo.Principles.开闭原则;
public class DefaultSkin extends AbstractSkin{
@Override
public void display() {
System.out.println("这是默认皮肤...");
}
}
HeimaSkin.java
package com.Demo.Principles.开闭原则;
public class HeimaSkin extends AbstractSkin{
@Override
public void display() {
System.out.println("这是黑马皮肤...");
}
}
SogoInput.java
This class realizes the aggregation relationship, and aggregates the abstract skin class and the concrete skin class, and the latter and the former belong to the part-to-whole relationship.
package com.Demo.Principles.开闭原则;
/**
* 聚合关系:搜狗输入法类
*/
public class SogoInput {
private AbstractSkin skin;
//传入皮肤类
public void setSkin(AbstractSkin skin){
this.skin=skin;
}
//调用当前皮肤的显示输出的方法
public void display(){
skin.display();
}
}
Test existing skin objects
At this time, if there is a new business requirement that requires adding a king skin, it is only necessary to add a new concrete skin class to inherit the abstract skin class , and implement all its methods to achieve the " opening and closing principle ".
KingSkin.java
Consistent with the previous specific skin class writing method, it is not necessary to modify any original code for business expansion
package com.Demo.Principles.开闭原则;
public class KingSkin extends AbstractSkin{
@Override
public void display() {
System.out.println("这是王者皮肤...");
}
}
Test newly added skins
This is the simple application of the principle of opening and closing. The expansion of business functions is realized without modifying the original code. It is open to expansion and closed to modification .
Liskov Substitution Principle
overview
The Liskov substitution principle means: all references to the base class (parent class) must be able to transparently use objects of its subclasses
The popular understanding is that subclasses can extend the functions of the parent class, but cannot change the original functions of the parent class.
In other words, when a subclass inherits the parent class, in addition to adding new methods to complete new business functions, try not to rewrite the methods of the parent class , otherwise the reusability of the entire inheritance system will deteriorate .
The Liskov substitution principle states that:
- Replace a base class object with its subclass object in the software, the program will not produce any errors and exceptions
- The opposite is not true, if a software entity uses a subclass object, it may not necessarily use the base class object
Therefore, when we design software:
- Try to use the base class type to define the object in the program , because the subclass object can be used where the base class object is used; and then determine the subclass type at runtime , and replace the parent class object with the subclass object
- When using this principle, the base class, that is, the parent class, should be designed as an abstract class or interface , so that the subclass inherits the parent class or implements the interface of the parent class, and implements the methods declared in the parent class. Replacing the parent class instance can easily expand the functions of the system without modifying the code of the original subclass. Adding new functions can be achieved by adding a new subclass
END.