Table of contents:
2. Obtain the verification code
2. service implementation class
foreword
Email verification code is a common function, which is often used in operations such as mailbox binding and password modification. This blog only talks about how to use springboot to realize the sending function of verification code. I use the redis database to save temporary email verification code information.
1. POM dependency
<dependencies>
<!--mail邮件发送-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
<!--redis场景启动器-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!-- redis 连接池 -->
<!--新版本连接池lettuce-->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
</dependency>
</dependencies>
2. Obtain the verification code
Steps : 1. Log in to QQ mailbox --> 2. Settings --> 3. Account security --> 4. Enable services (POP3/IMAP/SMTP/Exchange/CardDAV/CalDAV services)
Send a text message as required to get the authorization code (remember to save the obtained authorization code, it will be used later)
3. Configuration file
- username: is your email address, not your username
- password: is an authorization code, not a password
- host: is the SMTP server, not the IP address
spring:
# mail邮箱
mail:
# SMTP服务器(我用的是QQ邮箱的SMTP服务器地址,如果用的其它邮箱请另行百度搜索)
host: smtp.qq.com
# 发送验证码的邮箱(发件人的邮箱)
username: ***@qq.com
# 授权码
password: ***
# 编码
default-encoding: utf-8
# 其它参数
properties:
mail:
smtp:
# 如果是用SSL方式,需要配置如下属性,使用qq邮箱的话需要开启
ssl:
enable: true
required: true
# 邮件接收时间的限制,单位毫秒
timeout: 10000
# 连接时间的限制,单位毫秒
connection-timeout: 10000
# 邮件发送时间的限制,单位毫秒
write-timeout: 10000
PS : I am using the QQ mailbox for the demonstration here. If you use other mailboxes, please search on Baidu and replace the SMTP address.
4. Business code
1. service interface
// 发送邮件
String sendEmail(String email);
2. service implementation class
PS : It is necessary to determine whether the mailbox already has a key in redis to prevent repeated sending.
import com.test.java.util.VerifyCodeUtil;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeUtility;
import java.util.concurrent.TimeUnit;
/**
* 用户业务实现类
*/
@Service
@Slf4j
public class UserServiceImpl implements UserService {
@Resource
private StringRedisTemplate stringRedisTemplate;
@Resource
private JavaMailSender javaMailSender;
@Resource
private VerifyCodeUtil verifyCodeUtil;
@Override
public String sendEmail(String email) {
if (StringUtils.isBlank(email)) {
throw new RuntimeException("未填写收件人邮箱");
}
// 定义Redis的key
String key = "msg_" + email;
ValueOperations<String, String> valueOperations = stringRedisTemplate.opsForValue();
String verifyCode = valueOperations.get(key);
if (verifyCode == null) {
// 随机生成一个6位数字型的字符串
String code = verifyCodeUtil.generateVerifyCode(6);
// 邮件对象(邮件模板,根据自身业务修改)
SimpleMailMessage message = new SimpleMailMessage();
message.setSubject("**游戏注册邮箱验证码");
message.setText("尊敬的用户您好!\n\n感谢您注册**游戏。\n\n尊敬的: " + email + "您的校验验证码为: " + code + ",有效期5分钟,请不要把验证码信息泄露给其他人,如非本人请勿操作");
message.setTo(email);
try {
// 对方看到的发送人(发件人的邮箱,根据实际业务进行修改,一般填写的是企业邮箱)
message.setFrom(new InternetAddress(MimeUtility.encodeText("**游戏官方") + "<1*********@qq.com>").toString());
// 发送邮件
javaMailSender.send(message);
// 将生成的验证码存入Redis数据库中,并设置过期时间
valueOperations.set(key, code, 5L, TimeUnit.MINUTES);
log.info("邮件发送成功");
return "邮件发送成功";
} catch (Exception e) {
log.error("邮件发送出现异常");
log.error("异常信息为" + e.getMessage());
log.error("异常堆栈信息为-->");
return "邮件发送失败";
//e.printStackTrace();
//throw new RuntimeException(e);
}
} else {
return "验证码已发送至您的邮箱,请注意查收";
}
}
3. Related tools Util
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
import java.util.Random;
/**
* 验证码相关工具类
*/
@Component
public class VerifyCodeUtil {
@Resource
private StringRedisTemplate stringRedisTemplate;
/**
* 随机生成指定长度字符串验证码
*
* @param length 验证码长度
*/
public String generateVerifyCode(int length) {
String strRange = "1234567890";
StringBuilder strBuilder = new StringBuilder();
for (int i = 0; i < length; ++i) {
char ch = strRange.charAt((new Random()).nextInt(strRange.length()));
strBuilder.append(ch);
}
return strBuilder.toString();
}
/**
* 校验验证码(可用作帐号登录、注册、修改信息等业务)
* 思路:先检查redis中是否有key位对应email的键值对,没有代表验证码过期;如果有就判断用户输入的验证码与value是否相同,进而判断验证码是否正确。
*/
public Integer checkVerifyCode(String email, String code) {
int result = 1;
ValueOperations<String, String> valueOperations = stringRedisTemplate.opsForValue();
String msgKey = "msg_" + email;
String verifyCode = valueOperations.get(msgKey);
/*校验验证码:0验证码错误、1验证码正确、2验证码过期*/
if (verifyCode == null) {
result = 2;
} else if (!code.equals(verifyCode)) {
result = 0;
}
// 如果验证码正确,则从redis删除
if (result == 1) {
stringRedisTemplate.delete(msgKey);
}
return result;
}
}
4. Query the Redis database
5. Receive mail 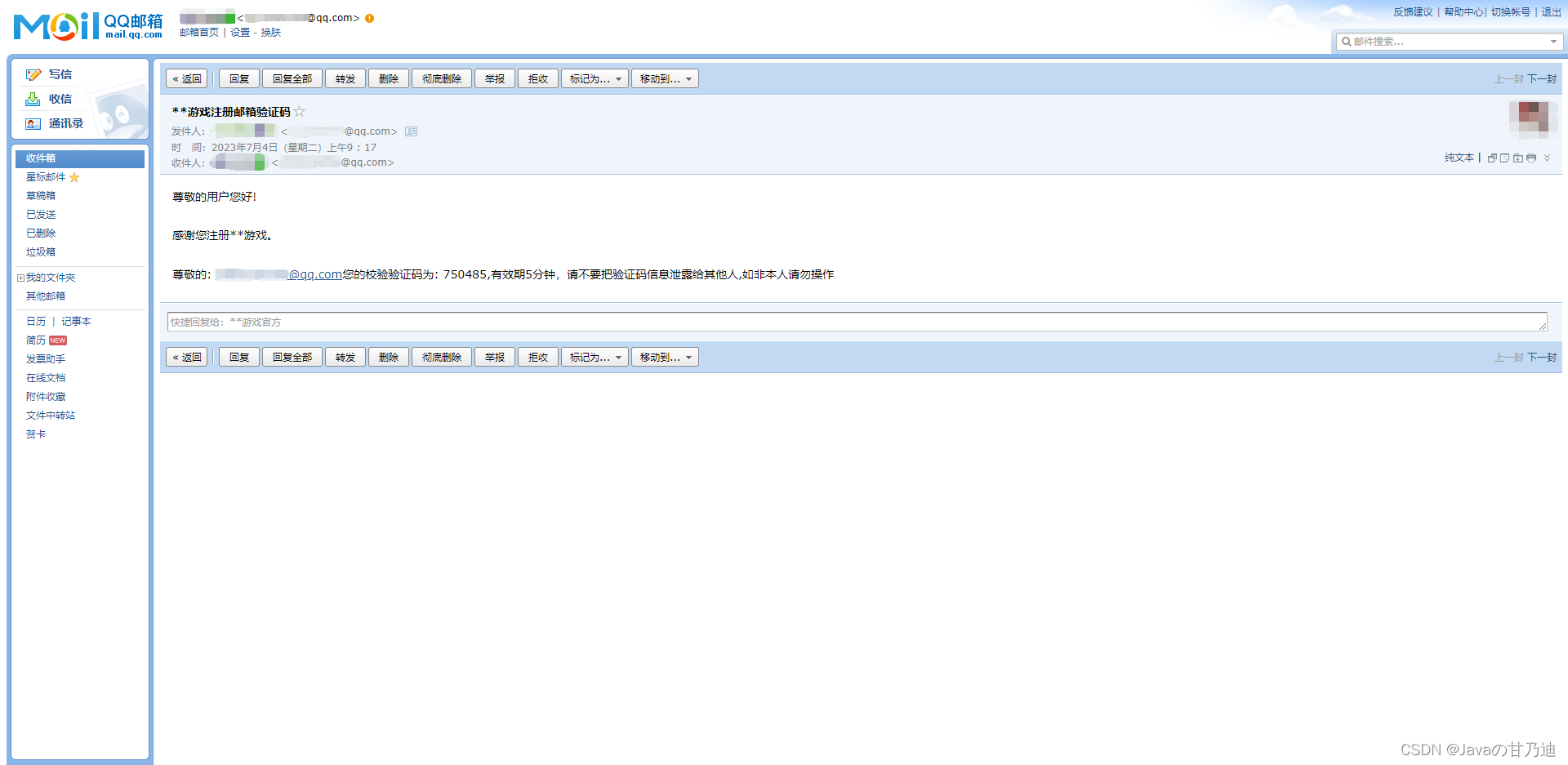
If this article is helpful or inspiring to you, please click three times: like, comment, bookmark ➕ follow, your support is my biggest motivation to keep writing.