Tip: After the article is written, the table of contents can be automatically generated. How to generate it can refer to the help document on the right
Table of contents
2. The first C language program
4.1 Method of defining variables
4.2 Classification of variables
The following are the naming rules for variablesEdit
4.4 The scope and life cycle of variables
5. String + escape character + comment
\n and \t are the two most commonly used escape characters:
foreword
Basic understanding of the basics of C language, and a general understanding of C language.
1. What is C language
C language is a general-purpose computer programming language, which is widely used in low-level development. The design goal of the C language is to provide a simple
A programming language that compiles in the same way, handles low-level memory, generates a small amount of machine code, and can run without any runtime environment support.
Word.
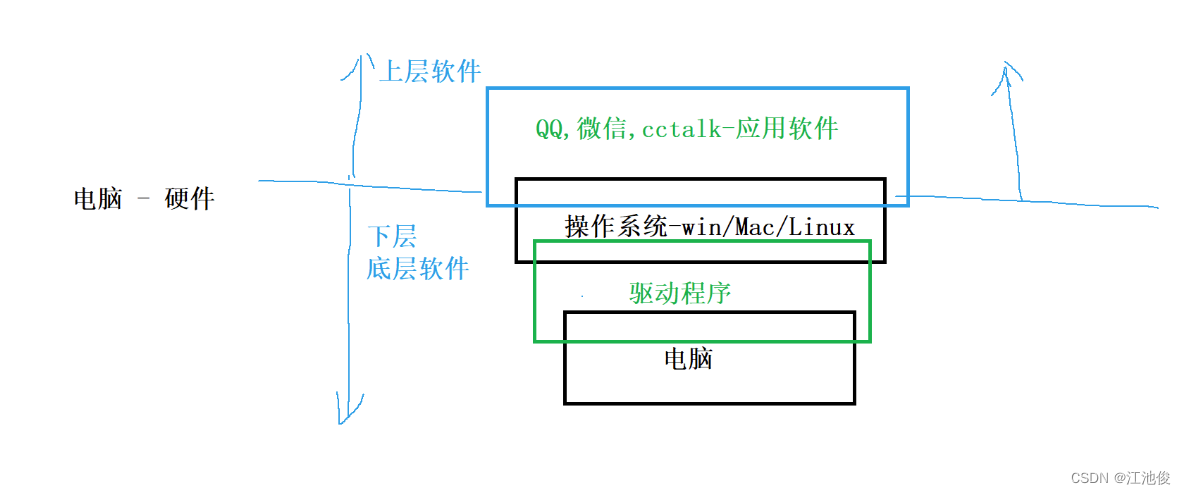
Although the C language provides many low-level processing functions, it still maintains a good cross-platform feature, written in a standard specification
C language programs can be compiled on many computer platforms, even some embedded processors (single-chip microcomputers or MCUs) and supercomputers
computer and other operating platforms.
In the 1980s, in order to avoid differences in the syntax of the C language used by various developers, the National Bureau of Standards established a standard for the C language.
A complete set of American National Standard syntax, called ANSI C, was established as the original standard of the C language. [1] Current December 8, 2011
The C11 standard released by the International Organization for Standardization (ISO) and the International Electrotechnical Commission (IEC) is the third official standard of the C language.
It is also the latest standard of C language. This standard better supports Chinese character function names and Chinese character identifiers, and realizes Chinese characters to a certain extent.
word programming.
C language is a process-oriented computer programming language, which is different from object-oriented programming languages such as C++ and Java.
Its compilers mainly include Clang, GCC , WIN-TC, SUBLIME, MSVC , Turbo C, etc.
2. The first C language program
#include <stdio.h>
int main()
{
printf("hello world\n");
printf("ha ha\n");
return 0;
}
//解释:
//main函数是程序的入口
//一个工程中main函数有且仅有一个
3. Data type
char //字符数据类型
short //短整型
int //整形
long //长整型
long long //更长的整形
float //单精度浮点数
double //双精度浮点数
//C语言有没有字符串类型?
Why such a type?
What is the size of each type?
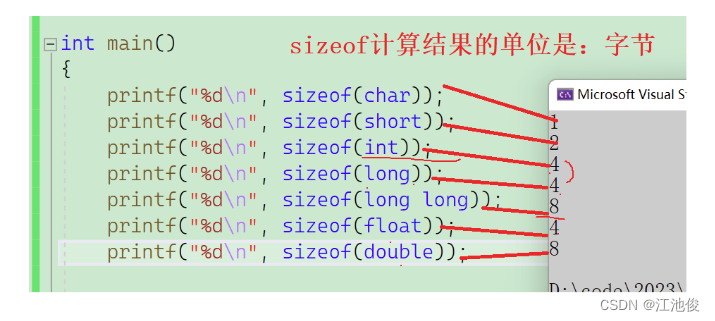
//计算机中常见的计算机单位有:
//bit-字节
//byte-比特位
//KB
//MB
//GB
//TB
//PB
//....
//它们间的换算关系如下:
//1 byte = 8 bit
//1 KB = 1024 byte
//1 MB = 1024 KB
//1 GB = 1024 MB
//1 TB = 1024 GB
//1 PB = 1024 TB
//....
Note: There are so many types, in fact, to express various values in life more abundantly.
Type of use:
char ch = 'w';
int weight = 120;
int salary = 20000;
Fourth, variables, constants
Some values in life are constant (for example: pi, gender, ID number, blood type, etc.)
Some values are variable (eg: age, weight, salary).
4.1 Method of defining variables
int age = 150;
float weight = 45.5f;
char ch = 'w';
4.2 Classification of variables
#include <stdio.h>
int global = 2019;//全局变量
int main()
{
int local = 2018;//局部变量
//下面定义的global会不会有问题?
int global = 2020;//局部变量
printf("global = %d\n", global);
return 0;
}
Summarize:
There is actually nothing wrong with the definition of the local variable global variable above !
When a local variable has the same name as a global variable, the local variable takes precedence.
4.3 Use of variables
#include <stdio.h>
int main()
{
int num1 = 0;
int num2 = 0;
int sum = 0;
printf("输入两个操作数:>");
scanf("%d %d", &num1, &num2);
sum = num1 + num2;
printf("sum = %d\n", sum);
return 0;
}
//scanf是输入语句
//printf是输出语句
The following are the naming rules for variables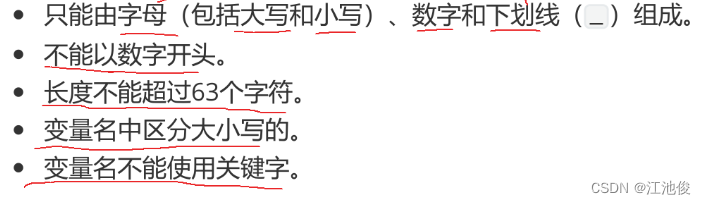
4.4 The scope and life cycle of variables
scope
Scope (scope) is a programming concept, generally speaking, the names used in a piece of program code are not always valid/available
of
The scope of code that limits the availability of the name is the scope of the name.
1. The scope of a local variable is the local scope in which the variable resides.
2. The scope of global variables is the entire project.
life cycle
The life cycle of a variable refers to a period of time between the creation of the variable and the destruction of the variable
1. The life cycle of a local variable is: the life cycle of entering the scope begins, and the life cycle of the out of scope ends.
2. The life cycle of global variables is: the life cycle of the entire program.
4.5 Constants
The definition forms of constants and variables in C language are different.
The constants in C language are divided into the following categories:
literal constant
const modified constant variable
Identifier constants defined by #define
enumeration constant
#include <stdio.h>
//举例
enum Sex
{
MALE,
FEMALE,
SECRET
};
//括号中的MALE,FEMALE,SECRET是枚举常量
int main()
{
//字面常量演示
3.14;//字面常量
1000;//字面常量
//const 修饰的常变量
const float pai = 3.14f; //这里的pai是const修饰的常变量
pai = 5.14;//是不能直接修改的!
//#define的标识符常量 演示
#define MAX 100
printf("max = %d\n", MAX);
//枚举常量演示
printf("%d\n", MALE);
printf("%d\n", FEMALE);
printf("%d\n", SECRET);
//注:枚举常量的默认是从0开始,依次向下递增1的
return 0;
}
Note:
The pai
in the above example
is called
a constant variable modified by
const , and
the constant variable modified by const is only restricted at the grammatical level in C language.
The variable
pai
cannot be changed directly, but
pai
is essentially a variable, so it is called a constant variable.
5. String + escape character + comment
5.1 Strings
"hello world!\n"
This string of characters enclosed by double quotes (
Double Quote
) is called a string literal (
String Literal
), or simply a string.
Note: The end of the string is a
\0
escape character.
\0 is the end mark
when calculating the length of the string
, and it is not counted as the content of the string.
//下面代码,打印结果是什么?为什么?(突出'\0'的重要性)
int main()
{
char arr1[] = "hello";
char arr2[] = {'h', 'e', 'l','l','o'};
char arr3[] = {'h', 'e', 'l','l','o','\0'};
printf("%s\n", arr1);
printf("%s\n", arr2);
printf("%s\n", arr3);
return 0;
}
#include <stdio.h>
int main()
{
printf("c:\code\test.c\n");
return 0;
}
5.2 Escape characters
If we want to print a directory to the screen: c:\code\test.c
So how do we write code like this?
#include <stdio.h>int main (){printf ( "c:\code\test.c\n" );return 0 ;}
In fact, the result of running the program is this:

Here I have to mention the escape character. The escape character, as the name suggests, is to change the meaning.
Let's look at some escape characters.
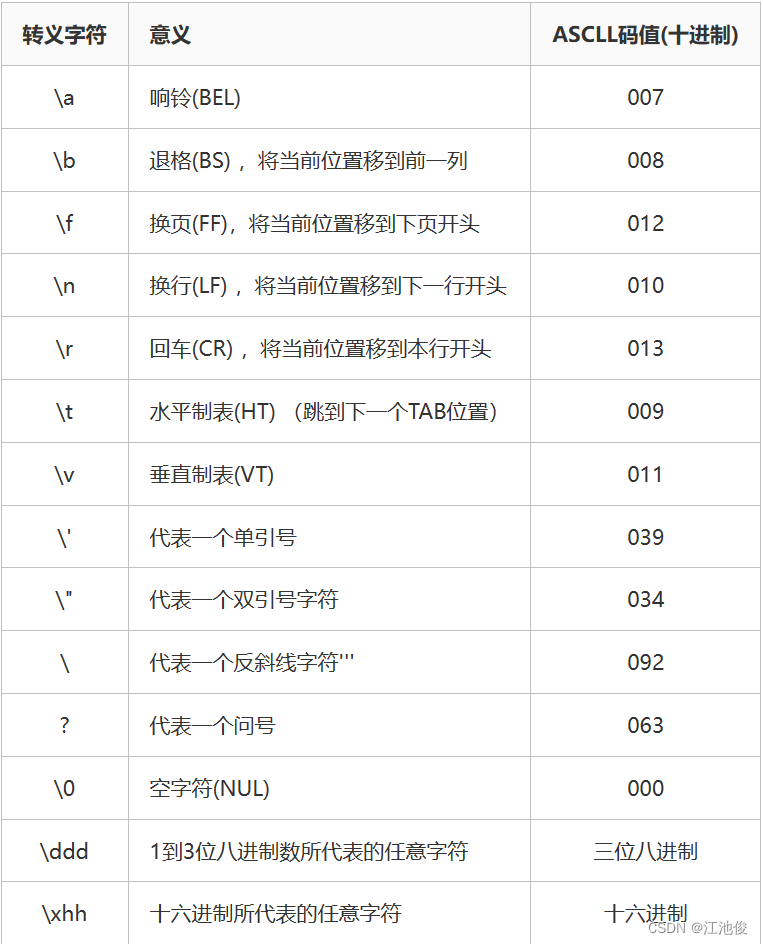
\n
and \t
are the two most commonly used escape characters:
\n
It is used to break the line and let the text output from the beginning of the next line, which has been used many times in the previous chapters;\t
It is used to occupy a place, generally equivalent to four spaces, or the function of the tab key.\xhh
Hexadecimal escape does not limit the number of characters'\x000000000000F' == '\xF'
6. Notes
1.
Unneeded code in the code can be deleted directly or commented out
2.
Some codes in the code are difficult to understand, you can add a comment text
for example:
#include <stdio.h>
int Add(int x, int y)
{
return x+y;
}
/*C语言风格注释
int Sub(int x, int y)
{
return x-y;
}
*/
int main()
{
//C++注释风格
//int a = 10;
//调用Add函数,完成加法
printf("%d\n", Add(1, 2));
return 0;
}
Comments come in two flavors:
C
-style comments
/*xxxxxx*/
Disadvantage: cannot nest comments
C++
style comments
//xxxxxxxx
You can comment one line or multiple lines