Table of contents
1. Introduction to OLED
OLED stands for Organic Light Emitting Diode (Organic Light Emitting Diode). OLED is considered to be the most advanced technology due to its self-illumination, no need for backlight, high contrast, thin thickness, wide viewing angle, fast response speed, flexible panel, wide operating temperature range, and simple structure and manufacturing process. Next-generation flat-panel display emerging application technology.
LCDs all need a backlight, OLED doesn't because it's self-illuminating. In this way, the same display OLED effect is better. With the current technology, it is still difficult to enlarge the size of OLED, but the resolution can indeed be very high.
2. Introduction to IIC
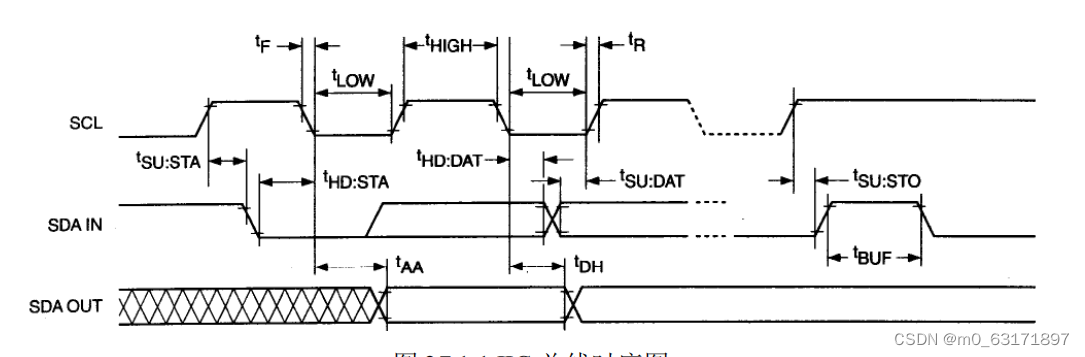
2.1 IIC interface module
as the picture shows:
Module interface definition:
1.GND - power ground
2.VCC——power supply positive (3.3v-5v)
3. SCL - IIC clock line
4. SDA——IIC data line
Resolution: 128*64
Display area: 21.744*10.864 (mm)
Interface type: IIC interface
Number of pins: 4
Working voltage: 3.5v
View direction: all directions
Control chip: SSD1306
Working temperature: -40~70
3. Code introduction
oled.c
//开始信号
void I2C_Start(void)
{
OLED_SDIN_Set();
OLED_SCLK_Set();
OLED_SDIN_Clr();
OLED_SCLK_Clr();
}
//结束信号
void I2C_Stop(void)
{
OLED_SCLK_Set();
OLED_SDIN_Clr();
OLED_SDIN_Set();
}
//应答信号
void I2C_WaitAck(void) //²âÊý¾ÝÐÅºÅµÄµçÆ½
{
OLED_SCLK_Set();
OLED_SCLK_Clr();
}
IIC writes a byte
//写入一个字节
void Send_Byte(u8 dat)
{
u8 i;
for(i=0;i<8;i++)
{
OLED_SCLK_Clr();//将时钟信号设置为低电平
if(dat&0x80)//将数据从高8位一次写入
{
OLED_SDIN_Set();
}
else
{
OLED_SDIN_Clr();
}
OLED_SCLK_Set();//将时钟信号设置为高电平
OLED_SCLK_Clr();/将时钟信号设置为低电平
dat<<=1;
}
}
Send a byte to SSD1306
//mode:数据/命令 0:数据;1:命令;
void OLED_WR_Byte(u8 dat,u8 mode)
{
I2C_Start();
Send_Byte(0x78);
I2C_WaitAck();
if(mode){Send_Byte(0x40);}
else{Send_Byte(0x00);}
I2C_WaitAck();
Send_Byte(dat);
I2C_WaitAck();
I2C_Stop();
}
OLED clear screen function
void OLED_Clear(void)
{
u8 i,n;
for(i=0;i<8;i++)
{
for(n=0;n<128;n++)
{
OLED_GRAM[n][i]=0;//清楚所有数据
}
}
OLED_Refresh();//更新显示
}
display a string
//显示字符串
//x,y:起点坐标
//size1:字体大小
//*chr:字符串起始地址
void OLED_ShowString(u8 x,u8 y,u8 *chr,u8 size1)
{
while((*chr>=' ')&&(*chr<='~'))//判断是不是非法字符
{
OLED_ShowChar(x,y,*chr,size1);
x+=size1/2;
if(x>128-size1) //换行
{
x=0;
y+=2;
}
chr++;
}
}
Display Chinese characters, the font size can be adjusted to 12, 16, 32, 64
//显示汉字
//x,y:起点坐标
//num:汉字对应的序号
//取模方式,列行式
void OLED_ShowChinese(u8 x,u8 y,u8 num,u8 size1)
{
u8 i,m,n=0,temp,chr1;
u8 x0=x,y0=y;
u8 size3=size1/8;
while(size3--)
{
chr1=num*size1/8+n;
n++;
for(i=0;i<size1;i++)
{
if(size1==16)
{temp=Hzk1[chr1][i];}//调用16*16字体
else if(size1==24)
{temp=Hzk2[chr1][i];}//调用24*24字体
else if(size1==32)
{temp=Hzk3[chr1][i];}//调用32*32字体
else if(size1==64)
{temp=Hzk4[chr1][i];}//调用64*64字体
else return;
for(m=0;m<8;m++)
{
if(temp&0x01)OLED_DrawPoint(x,y);
else OLED_ClearPoint(x,y);
temp>>=1;
y++;
}
x++;
if((x-x0)==size1)
{x=x0;y0=y0+8;}
y=y0;
}
}
}
show a next picture
//x0,y0起点坐标
//x1,y1终点坐标
//BMP[]要写入的图片数组
void OLED_ShowPicture(u8 x0,u8 y0,u8 x1,u8 y1,u8 BMP[])
{
u32 j=0;
u8 x=0,y=0;
if(y%8==0)y=0;
else y+=1;
for(y=y0;y<y1;y++)
{
OLED_WR_BP(x0,y);
for(x=x0;x<x1;x++)
{
OLED_WR_Byte(BMP[j],OLED_DATA);
j++;
}
}
}
Show results
Main function, display Chinese characters and characters at the same time
#include "delay.h"
#include "sys.h"
#include "oled.h"
#include "bmp.h"
#include "led.h"
int main(void)
{
u8 t;
delay_init();
OLED_Init();
OLED_ColorTurn(0);//0正常显示,1反色显示
OLED_DisplayTurn(0);//0正常显示 1 屏幕翻转显示
OLED_Refresh(); //更新显示到oled
t=' ';
while(1)
{
OLED_ShowChinese(64,0,0,64); //64*64 中
OLED_Refresh();
delay_ms(500);
OLED_Clear();
OLED_ShowString(0,0,"ABC",12);//6*12
OLED_ShowString(0,12,"ABC",16);//8*16
OLED_ShowString(0,28,"ABC",24);//12*24
OLED_Refresh();
delay_ms(500);
}
}
Mode of taking modulus:
4. Complete code
It is not easy to make, and complete engineering documents are required, like and collect, and leave an email in the comment area
STM32 IIC-based OLED display program resource - CSDN library