1. What is spring
The Spring framework is an open source J2EE application framework initiated by Rod Johnson and is a lightweight container for bean lifecycle management. Spring solves many common problems encountered by developers in J2EE development, and provides powerful functions such as IOC, AOP and Web MVC. Spring can be used alone to build applications, and can also be used in combination with many Web frameworks such as Struts, Webwork, and Tapestry, and can be combined with desktop application APs such as Swing. Therefore, Spring can be applied not only to J2EE applications, but also to desktop applications and applets. The Spring framework is mainly composed of seven parts, namely Spring Core, Spring AOP, Spring ORM, Spring DAO, Spring Context, Spring Web and Spring Web MVC.
2. What is IOC
The full name is Inversion of Controller. The Chinese explanation is inversion of control, not a technology, but a design idea. In java development, IOC means that the object you designed is handed over to the container for control, rather than the traditional direct control inside your object. How to understand IOC? The key to understanding IOC is to clarify: "who controls whom", "what to control", why it is a reversal (if there is a reversal, there should be a positive reversal), and which aspects are reversed, let's analyze:
1) Who controls who and what controls: In traditional java SE programming, we create objects directly inside the object with new, and the program actively creates objects, while IOC has a special container to create these objects, which is controlled by the IOC container Object creation; who controls whom? Of course, the IOC container controls the object. What does it control? Mainly to control the acquisition of external resources (not just objects including files, etc.).
2) Why is it reversed? That aspect is reversed: if there is a reverse, there is a positive rotation. In traditional applications, we directly obtain the dependent objects in the object, that is, the forward rotation; while the reverse is created by the container and injecting dependent objects; why inversion? Because the container helps us find and inject dependent objects, the object only passively accepts the dependent object, so it is reversed; which aspects are reversed? The fetching of dependent objects is reversed.
3. What is DI
Introduction
DI: Dependency Injection, that is, dependency injection: the dependency relationship between components is determined by the container at runtime. Visually speaking, the container dynamically injects a certain dependency relationship into the component.
What is DI (Dependency Injection) useful for?
The purpose of dependency injection is not to bring more functions to the software system, but to increase the frequency of component reuse and build a flexible and scalable platform for the system. Through the dependency injection mechanism, we only need to specify the resources required by the target without any code through simple configuration, and complete our own business logic, without caring where the specific resources come from and who implements them
4. Code example
Overall picture
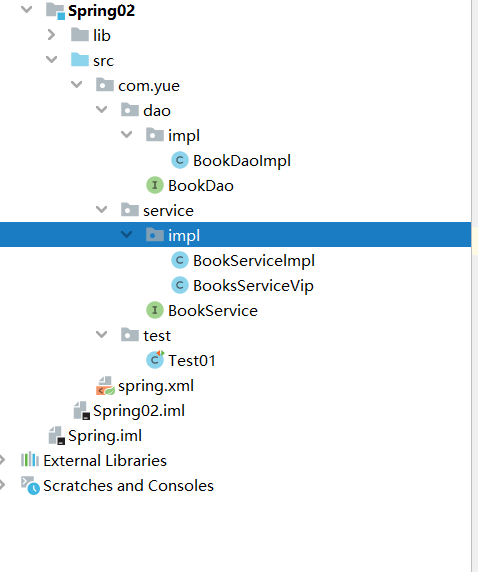
Write two interfaces and three implementation classes in dao package and service package
(1) BookDao interface and BookDaoImpl implementation class
package com.yue.dao;
public interface BookDao {
public void add();
}
package com.yue.dao.impl;
import com.yue.dao.BookDao;
import com.yue.service.impl.BooksServiceVip;
public class BookDaoImpl implements BookDao {
private BooksServiceVip booksServiceVip;
public void setBooksServiceVip(BooksServiceVip booksServiceVip) {
this.booksServiceVip = booksServiceVip;
}
@Override
public void add() {
System.out.println("BookDaoImpl...add");
booksServiceVip.seach();
}
}
(2) BookService interface and BookServiceImpl, BookServiceVip implementation class
package com.yue.service;
public interface BookService {
void seach();
}
package com.yue.service.impl;
import com.yue.dao.BookDao;
import com.yue.service.BookService;
public class BookServicelmpl implements BookService {
private BookDao bookDao;
public void setBookDao(BookDao bookDao) {
this.bookDao = bookDao;
}
@Override
public void seach() {
System.out.println("BookServicelmpl...seach");
bookDao.add();
}
}
package com.yue.service.impl;
import com.yue.service.BookService;
public class BooksServiceVip implements BookService {
@Override
public void seach() {
System.out.println("BooksServiceVip...seach");
}
}
Introduce the Spring framework
(1) Write in spring.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="BookService" class="com.yue.service.impl.BookServicelmpl">
<property name="bookDao" ref="BookDao"/>
</bean>
<bean id="BookDao" class="com.yue.dao.impl.BookDaoImpl">
<property name="booksServiceVip" ref="BooksService"/>
</bean>
<bean id="BooksService" class="com.yue.service.impl.BooksServiceVip"/>
</beans>
Implement the test class
(1) Write in Test01
package com.yue.test;
import com.yue.service.BookService;
import org.junit.Test;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test01 {
BookService bookService;
@Test
public void test01(){
ClassPathXmlApplicationContext context=new ClassPathXmlApplicationContext("spring.xml");
bookService = (BookService) context.getBean("BookService");
bookService.seach();
}
}
operation result:
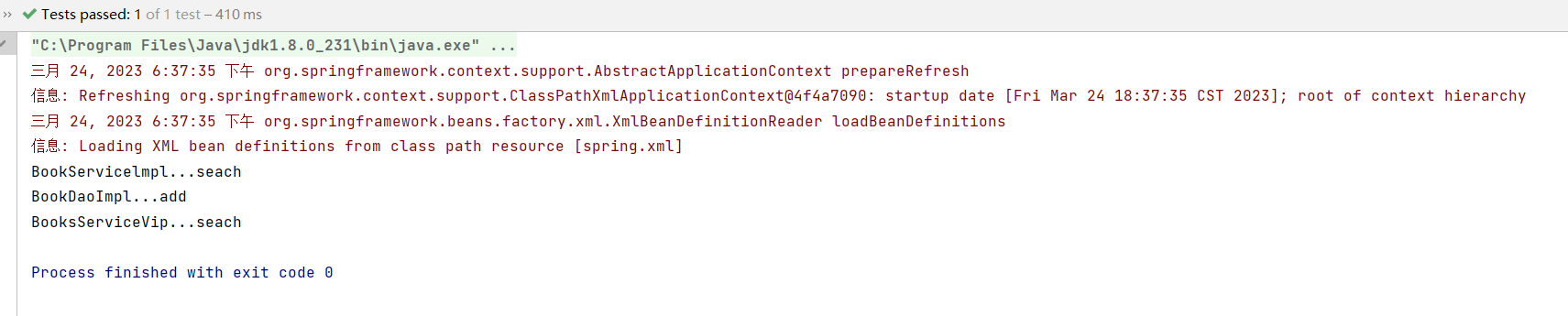