array
Article directory
- array
-
- Why do you need an array?
- Introduction to Arrays
- A case to quickly understand arrays
- Array definition and memory layout
- Array Usage Notes and Details
- Array application case
- Character counts and strings
- representation of a string
- String related functions
- String (Character Array) Usage Notes and Details
Why do you need an array?
There are 6 chickens in a chicken farm, and their weights are 3kg, 5kg, 1kg, 3.4kg, 2kg, 50kg respectively. What is the total weight of these six chickens? What is the average weight? Please write a program.
traditional solution
1. Define 6 double variables
2. Count them and find the average
3. The traditional solution is inflexible and unable to fulfill a large number of needs
4. Lead us to explain the array
Introduction to Arrays
An array can store multiple data of the same type . An array is also a data type , which is a structural type . The transfer is by reference (that is, the address is passed )
A case to quickly understand arrays
#include <stdio.h>
int main(){
/*一个养鸡场有6只鸡,它们的体重分别是3kg,5kg,1kg,
3.4kg,2kg,50kg 。请问这六只鸡的总体重是多少?
平 均体重是多少? 请你编一个程序。*/
//1.定义数组
double hens[6];
double totalWeight = 0.0;
double avgWeight = 0.0;
int i;
int arrLen;//数组的大小
//2.初始化数组的每个元素
hens[0] = 3;//第一个元素
hens[1] = 5;
hens[2] = 1;
hens[3] = 3.4;
hens[4] = 2;
hens[5] = 50;
//3.遍历数组
//如何得到数组大小
//sizeof(hens) 数组的总的大小
//一个double 8个字节 6*8=48
//sizeof(double) 返回一个double占用的字节数
//printf("sizeof(hens)=%d",sizeof(hens));
arrLen = sizeof(hens) / sizeof(double);
for(i=0;i<arrLen;i++){
totalWeight += hens[i];//累计每只鸡体重
}
avgWeight = totalWeight / 6;
printf("总体重totalWeight=%.2f 平均体重avgWeight=%.2f",totalWeight,avgWeight);
getchar();
}
Array definition and memory layout
(1) Definition of array
数据类型 数组名 [数组大小]
int a[5]; //a 数组名,类型int , [5]大小,即a数组最多存放5个int数据赋初值
(2) Array memory map (important)
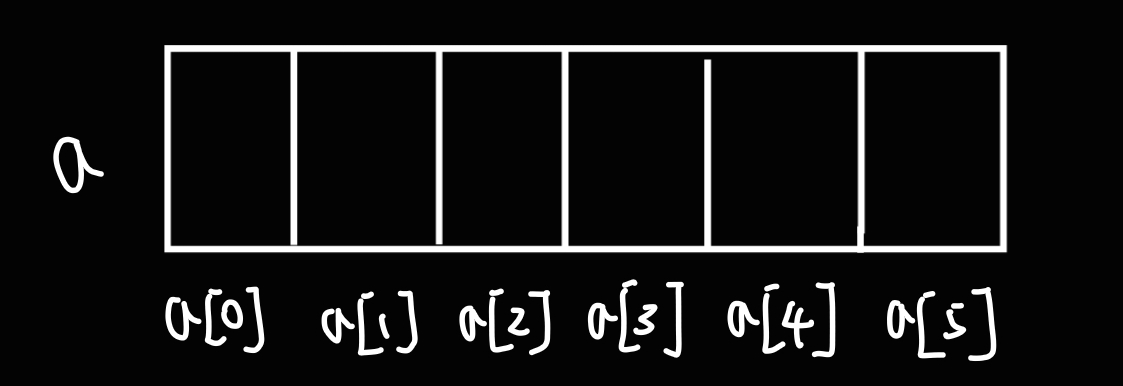
illustrate:
数组名 就代表 该数组的首地址 ,即a[0]地址
数组的各个元素是 连续发布的 ,假如a[0] 地址 0x1122
a[1]地址 = a[0]的地址 + int字节数(4) = 0x1122+4 = 0x1126
a[2]地址 = a[1]地址 + int字节数(4) = 0x1126+4 =0x112A
依次类推
(3) Accessing array elements
Array name [subscript] For example: you want to use the third element a[2] of the a array, and the subscript starts from 0
(4) Small case
Input 5 grades in a loop from the terminal, save them to a double array, and output
(5) Three ways to initialize arrays
Array Usage Notes and Details
Array application case
1. Create an array of 26 elements of char type, place 'A'-'Z' respectively. Use a for loop to iterate over all elements and print them. Tip : character data operation 'A'+1 -> 'B'
2. Request the maximum value of an array and get the corresponding subscript.
Character counts and strings
1. Basic introduction to character arrays
用来存放字符的数组称为字符数组, 看几个案例
char a[10]; //一维字符数组, 长度为10
char b[5][10]; //二维字符数组
char c[20]={'c', ' ', 'p', 'r', 'o', 'g', 'r', 'a','m'}; // 给部分数组元素赋值
字符数组实际上是一系列字符的集合,也就是字符串(String)。在C语言中,没 有专门的字符串变量,没有string类型,通常就用一个字符数组来存放一个字符串
2. String Considerations
(1) In the C language, a string is actually a one-dimensional array of characters terminated by a null character ('\0'). Thus, a null-terminated string contains the characters that make up the string.
(2) '\0' is the 0th character in the ASCII code table, represented by NUL, called a null character. This character cannot be displayed, nor is it a control character. Outputting this character will not have any effect. It is only used as the end symbol of the string in C language.
(3) Layout analysis of character array (string) in memory [case]
(4) Think about char str[3] = {'a', 'b', 'c'} What is the output? Why?
Conclusion: If (1) the number of assigned elements is less than the length of the array when assigning a value to a character array, it will automatically add '\0' at the end to indicate the end of the string, (2) assign The number of elements is equal to the length of the array, then '\0' will not be added automatically
(5) Case
3. String access and traversal
Because the essence of a string is an array of characters, you can traverse and access an element in the way of an array
The case is as follows:
representation of a string
1. Use a character array to store a string
char str[]="hello tom";
char str2[] = {'h', 'e'};
2. Use a character pointer to point to a string
char* pStr=" hello tom";
(1) The C language handles the string constant "hello tom" as a character array , and a character array is created in the memory to store the string constant. When the program defines the string pointer variable str (that is, the first address of the character array storing the string) is assigned to pStr
(2) printf("%s\n", str); can output the string pointed to by str
(3) Corresponding memory layout diagram
3. Discussion on using character pointer variables and character arrays to represent strings
(1) The character array is composed of several elements, and each element contains a character; while the character pointer variable stores the address (the first address of the string/character array), it is by no means putting the string into the character pointer variable ( is the first address of the string)
(2) You can only assign values to each element of a character array, and you cannot use the following methods to assign values to a character array
char str[14];//str实际是一个常量(存的地址),常量不能改变
str=" hello tom"; //错误
str[0] = 'i'; //ok
(3) It is possible to assign values to character pointer variables using the following method
Because the pointer is a variable, you can change its pointing
char* a="yes";
a=" hello tom";
Understanding: a is a pointer variable (it has an address), originally it pointed to the space of yes, now it points to hello tom, and then the space of yes is recycled, the essence is that the pointed address has changed
Code demo:
(4) If a character array is defined, it has a definite memory address (that is, the name of the character array is a constant); and when a character pointer variable is defined, it does not point to a definite character data, and it can be used multiple times assignment
String related functions
1. Commonly used string functions
2. Case presentation
String (Character Array) Usage Notes and Details
(1) The program often relies on detecting the position of '\0' to determine whether the string ends, rather than determining the length of the string based on the length of the array. Therefore, the length of the string will not count '\0', and the length of the character array will be counted
(2) When defining the character array, the actual string length should be estimated to ensure that the array length is always greater than the actual length of the string, otherwise, when outputting the character array Unknown characters may appear.
(3) The system also automatically adds a '\0' as the terminator to the string constant. For example, "C Program" has 9 characters in total, but occupies 10 bytes in the memory, and the last byte '\0' is automatically added by the system. (It can be verified by the sizeof() function)
(4) When defining a character array, if the number of characters given is smaller than the length of the array, the system will set the remaining element space to '\0' by default, such as char str [6] = "ab", str memory layout is [a][b][\0][\0][\0][\0]
(5) There are many ways to define and initialize character arrays, such as
char str1[ ]={
"I am happy"}; // 默认后面加 '\0'
char str2[ ]="I am happy"; // 省略{}号 ,默认后面加 '\0'
char str3[ ]={
'I',' ','a','m',' ','h','a','p','p','y'}; // 字符数组后面不会加 '\0', 可能有乱码
char str4[5]={
'C','h','i','n','a'}; //字符数组后面不会加 '\0', 可能有乱码
char * pStr = "hello";