introduction
In front-end development, images are one of the most loaded static resource types in web pages, but loading failures may also occur during image loading, which greatly affects user experience. So how to correctly judge whether the picture is successfully loaded, and how to deal with it when the picture fails to load, is the main content of this article.
set the alt attribute
Let's take a look at w3c's explanation of this attribute.
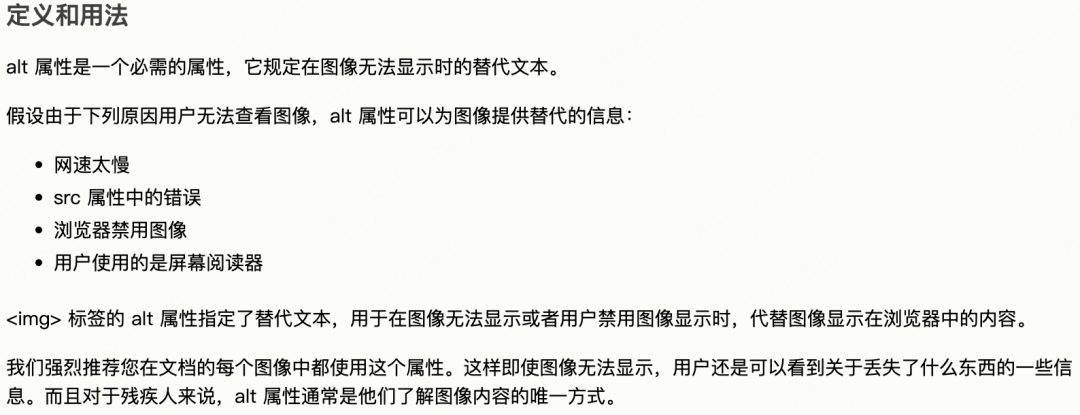
Its usage is also very simple, just add this attribute directly to the img tag.
<img src="https://p4.ssl.qhimg.com/t019f326a5524ce5fcc.png" />
<img src="https://p5.ssl.qhimg.com/t0139228d9f6f26225c.png" />
<img
src="https://p5.ssl.qhimg.com/t01950dfde27027b6191.jpg"
alt="图片加载失败啦"
/>
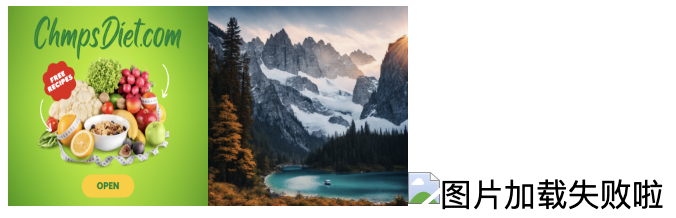
But this effect is actually not ideal. This is a broken picture by default, and the alt content is used as a text prompt. Users can know through the copy that the picture failed to load. Although the above method achieves the goal, it is indeed relatively rough. In the future, we will have better means to optimize.
Set up a bottom map
This is the most commonly used method in image error handling, and the subsequent codes are all based on react for demonstration.
single element processing
This processing method is similar to the lazy loading of images. When the image is not fully loaded, we give the img tag a default image. When the image fails to load, we will show our bottom image.
The first step is how to judge whether our image is loaded incorrectly. The img tag has an onerror event. The following is the description of the event by mdn.
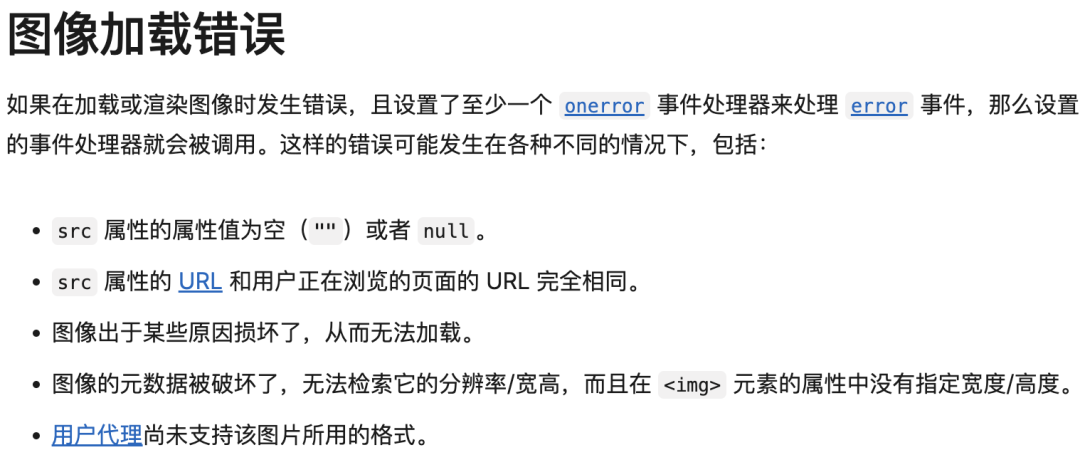
When an error occurs in our image loading process, this event will almost always be triggered, and we can use this event for targeted processing. like below
<img src="https://p4.ssl.qhimg.com/t019f326a5524ce5fcc.png" />
<img src="https://p5.ssl.qhimg.com/t0139228d9f6f26225c.png" />
<img
src="https://p5.ssl.qhimg.com/t01950dfde27027b6191.jpg"
onError={handleImgError}
/>
const handleImgError = (e: any) => {
console.log("e", e.target);
e.target.src = "https://p3.ssl.qhimg.com/t011d7a9a61e6d6be72.png";
};
The effect of the above code is as follows. The third picture failed to load, but our default bottom picture took effect, and the overall look is much more harmonious than before.
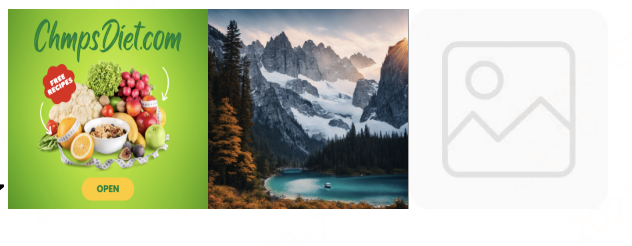
Unity catches errors
In the past, we bound the onerror event to the img tag to achieve the goal of failing to load the target image. However, there may be many images on the actual page. If we bind each image to a separate processing function, it will not only be troublesome , and there may be omissions, and it is not easy to maintain later.
So we can improve the processing method, by listening to the global error event, and then judge whether the element that triggers the error event is an Img tag, which is generated by the Img tag, and we will make corresponding changes to the image src.
window.addEventListener(
"error",
function (event) {
const target = event.target;
if (target instanceof HTMLImageElement) {
target.src = "https://p3.ssl.qhimg.com/t011d7a9a61e6d6be72.png";
console.log("图片加载异常", target);
}
},
true
);
<img src="https://p4.ssl.qhimg.com/t019f326a5524ce5fcc1.png" />
<img src="https://p5.ssl.qhimg.com/t0139228d9f6f26225c.png" />
<img src="https://p5.ssl.qhimg.com/t01950dfde27027b6191.jpg" />
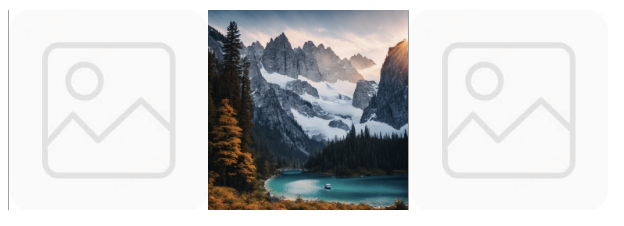
We can see that we did not add additional attributes to the Img tag, reducing additional overhead. Errors in multiple pictures can also be handled better.
base64 encoding
There are many reasons for the failure of image loading. In the above example, I invalidated the original src address of the image and responded with 404 to simulate an image loading error. However, if the loading failure is caused by network quality problems, then The resource that loads the bottom map may also fail, and the error event will be triggered infinitely. So we need further optimization, so that the picture does not need to obtain static resources from the remote server. At this point, we can think that the img tag can directly load images in base64 format, and we can use base64 as a string and hardcode it in our code. When the code is loaded, the image is equivalent to being loaded.
const errorImg ='base64字符串'; //因为base64字符串较长,所以这里就不贴上具体base64字符串
window.addEventListener(
"error",
function (event) {
const target = event.target;
if (target instanceof HTMLImageElement) {
target.src = errorImg;
console.log("图片加载异常", target);
}
},
true
);
Through the above code, we can avoid the embarrassment of infinitely triggering error events when the loading of the bottom map fails, but the characteristics of base64 itself determine that the image will be about 1/3 larger than the original, so when we choose the bottom map, The size of the image should be controlled as much as possible to avoid the packaged js file from becoming too large.
css processing
The above implementations are all implemented by js codes. We can also use css to achieve the above similar effects. If we use css to complete it, we can have greater customization of error styles. This is achieved using pseudo-elements in css. For error capture, we still use the previous method, but for subsequent processing, we don't need to add a bottom picture.
window.addEventListener(
"error",
function (event) {
const target = event.target;
if (target instanceof HTMLImageElement) {
target.classList.add("error"); //添加对应的类名
}
},
true
);
<img src="https://p4.ssl.qhimg.com/t019f326a5524ce5fcc.png" />
<img src="https://p5.ssl.qhimg.com/t0139228d9f6f26225c.png" />
<img src="https://p5.ssl.qhimg.com/t01950dfde27027b6191.jpg" />
.error {
position: relative;
width: 100px;
height: 100px;
}
img.error::before {
content: "";
position: absolute;
left: 0;
top: 0;
width: 100%;
height: 100%;
background-color: #f5f5f5;
color: transparent;
}
img.error::after {
content: "图片加载出错啦!";
position: absolute;
left: 0;
bottom: 0;
width: 100%;
height: 100%;
line-height: 100px;
background-color: rgba(0, 0, 0, 0.5);
color: white;
font-size: 12px;
text-align: center;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
When the picture is loaded incorrectly, we add a custom class name to the picture, and then implement the custom error style through pseudo-elements, set the background style before, and set the prompt copy after. Results as shown below.
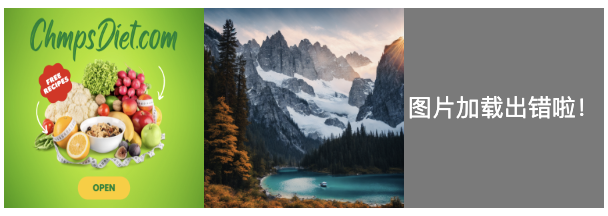
We only made the most basic settings for the style here, which can be beautified according to the scene. We did not introduce any picture resources, and achieved similar effects to the previous ones. Of course, if you want to add pictures, it is also very simple. We only need to insert the corresponding base64 format pictures in before.
- END -
About Qi Wu Troupe
Qi Wu Troupe is the largest front-end team of 360 Group, and participates in the work of W3C and ECMA members (TC39) on behalf of the group. Qi Wu Troupe attaches great importance to talent training, and has various development directions such as engineers, lecturers, translators, business interface people, and team leaders for employees to choose from, and provides corresponding technical, professional, general, and leadership training course. Qi Dance Troupe welcomes all kinds of outstanding talents to pay attention to and join Qi Dance Troupe with an open and talent-seeking attitude.