Java --- stage project --- backgammon
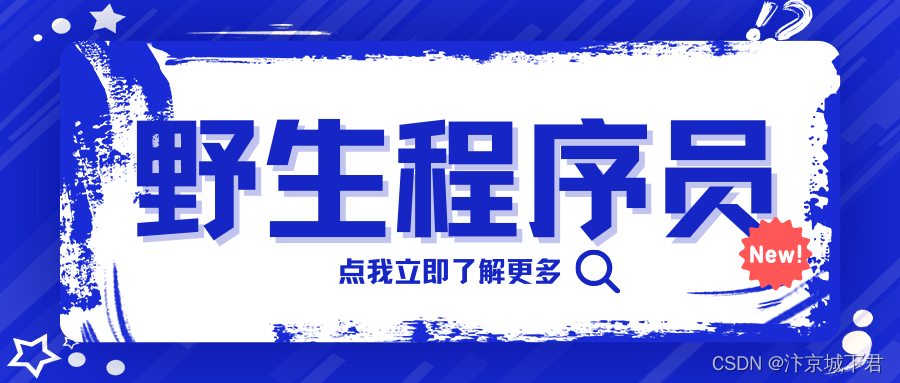
Statement of needs
The backgammon board is a 10×10 square, and there are two backgammon players (A, B). After A places a piece on the board, B places another piece, and so on, until one side wins or the space on the board is exhausted. The condition is that there are 5 consecutive pieces of A or B on a line or slash at the same time,
as shown in the figure:
Technical realization
static variable:
- grammar:
public static 数据类型 变量名 = 变量值;
Without static is a non-static variable
- Explain that
static variables can only be defined in classes, not in methods.
Static variables can be used in static modified methods, and can also be accessed in non-static methods. The
main solution is that non-static variables cannot be accessed in static methods.
public class study {
//类
//静态变量只能定义在类中,不能定义在方法中
public static String name = "张三";
public static void main(String[] args) {
//方法
System.out.println(name); //可以调用
}
}
static method:
- grammar
public static 返回值类型 方法名(){
}
- Explanation
The static method is equivalent to a box, but the code is placed in the box. When you need to use these codes, just put the box in the specified position
public class study {
//类
public static void main(String[] args) {
//方法
show();
}
public static void show(){
System.out.println("张三");
System.out.println("男");
System.out.println("20");
}
}
output:
Zhang San
Male
20
board making
1. Make a chess board
- Use tab characters in the input method to print out the chessboard directly on the console, and then look for the characteristics of the position
- Recreate the chessboard using a two-dimensional array
public class study {
public static char[][] chess_map={
{
'┌','┬','┬','┬','┬','┬','┬','┬','┬','┐'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'└','┴','┴','┴','┴','┴','┴','┴','┴','┘'}
};
public static String row = "────";
public static void main(String[] args) {
System.out.println(" 0 1 2 3 4 5 6 7 8 9");
for(int i=0;i<chess_map.length;i++) {
//外层循环控制行
System.out.print(i);
for(int j=0;j<chess_map[i].length;j++){
//内层循环控制列
if(j==chess_map[i].length-1){
//最后一行不打印——
System.out.print(chess_map[i][j]);
}else{
System.out.print(chess_map[i][j]+row);
}
}
System.out.println();
if(i<chess_map.length-1){
//排除最后一行
System.out.println(" │ │ │ │ │ │ │ │ │ │");
}
}
}
}
- The chessboard will be displayed repeatedly during the player's use, and methods need to be used to optimize it.
Put the chessboard in a method and call it directly
2. Lay
- Players A and B will play alternately
- The position of the chess piece must be an integer between 0 and 100, and existing chess pieces cannot be used
public static char pieceA = '○';//玩家A的棋子
public static char pieceB = '■';//玩家B的棋子
public static void main(String[] args) {
draw_map();
int sum = chess_map.length*chess_map[0].length;
Scanner sc = new Scanner(System.in);
for(int i=0;i<sum;i++){
System.out.println(i%2==0 ? "玩家A落子:":"玩家B落子:");
int position;
while(true){
//保证落子成功
if(sc.hasNextInt()){
//判断Scanner中是否有输入的数据
position = sc.nextInt();
if(position >= 0 && position < sum){
char currentpiece = (i%2==0) ? pieceA : pieceB;
int row = position / chess_map.length;//位置除以棋盘数组长度得到行号
int col = position % chess_map[0].length;//位置取模棋盘数组的总列数得到列号
if(chess_map[row][col]==pieceA || chess_map[row][col]==pieceB) {
System.out.println("该位置已经有棋子,请重新输入:");
continue;
}else {
chess_map[row][col] = currentpiece;
break;
}
}else{
System.out.println("非法输入");
}
}else{
System.out.println("非法输入");
sc.next();//将Scanner中的数据取出来,防止死循环
}
}
//落子成功后棋盘需要重新打印
draw_map();
}
}
- After the drop is completed, you need to check whether you have won
- The chessboard has not been used yet and the winner needs to be prompted
for (int m = 0; m < chess_map.length; m++) {
for (int j = 0; j < chess_map[0].length; j++) {
//第一种,水平方向
boolean case1 = (j + 4 < chess_map[0].length)
&& chess_map[m][j] == currentpiece
&& chess_map[m][j + 1] == currentpiece
&& chess_map[m][j + 2] == currentpiece
&& chess_map[m][j + 3] == currentpiece
&& chess_map[m][j + 4] == currentpiece;
//第二种,垂直方向
boolean case2 = (m + 4 < chess_map.length)
&& chess_map[m][j] == currentpiece
&& chess_map[m + 1][j] == currentpiece
&& chess_map[m + 2][j] == currentpiece
&& chess_map[m + 3][j] == currentpiece
&& chess_map[m + 4][j] == currentpiece;
//第三种,135°角
boolean case3 = (i + 4 < chess_map.length)
&& (j + 4 < chess_map[0].length)
&& chess_map[m][j] == currentpiece
&& chess_map[m + 1][j + 1] == currentpiece
&& chess_map[m + 2][j + 2] == currentpiece
&& chess_map[m + 3][j + 3] == currentpiece
&& chess_map[m + 4][j + 4] == currentpiece;
//第四种,45°角
boolean case4 = (m > 4) && (j + 4 < chess_map[0].length)
&& chess_map[m][j] == currentpiece
&& chess_map[m - 1][j + 1] == currentpiece
&& chess_map[m - 2][j + 2] == currentpiece
&& chess_map[m - 3][j + 3] == currentpiece
&& chess_map[m - 4][j + 4] == currentpiece;
if (case1 || case2 || case3 || case4) {
System.out.println(m % 2 == 0 ? "玩家A胜利" : "玩家B胜利");
break outer;
}
}
}
i++;
}
if(i==100){
System.out.println("平局");
}
3. Sound effects
- Added sound effects for moves, illegal moves and wins
Need to prepare documents
public static void playAudio(String fileName){
URl url = Gobang.class.getResource(fileName);
AudioClip clip = Applet.newAudioClip(url);
clip.play();
try{
Thread.sleep(50L);
}catch (InterruptedException e){
}
}
full code
import java.util.Scanner;
public class study {
public static char[][] chess_map={
{
'┌','┬','┬','┬','┬','┬','┬','┬','┬','┐'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'├','┼','┼','┼','┼','┼','┼','┼','┼','┤'},
{
'└','┴','┴','┴','┴','┴','┴','┴','┴','┘'}
};
public static String ro = "────";
public static char pieceA = '○';//玩家A的棋子
public static char pieceB = '■';//玩家B的棋子
public static int i = 0;//总次数
public static void main(String[] args) {
draw_map();
int sum = chess_map.length * chess_map[0].length;
Scanner sc = new Scanner(System.in);
outer:
while(i<sum){
System.out.println(i % 2 == 0 ? "玩家A落子:" : "玩家B落子:");
char currentpiece = (i % 2 == 0) ? pieceA : pieceB;
// int position_row,position_col;
int position;
while (true) {
//保证落子成功
if (sc.hasNextInt()) {
//判断Scanner中是否有输入的数据
// System.out.println("请输入行:");
// position_row = sc.nextInt();
// System.out.println("请输入列:");
// position_col = sc.nextInt();
position = sc.nextInt();
// if(position_row >= 0 && position_row < chess_map.length && position_col>=0 && position_col < chess_map[0].length){
if (position >= 0 && position < sum) {
int row = position / chess_map.length;//位置除以棋盘数组长度得到行号
int col = position % chess_map[0].length;//位置取模棋盘数组的总列数得到列号
// int row = position_row;
// int col = position_col;
if (chess_map[row][col] == pieceA || chess_map[row][col] == pieceB) {
System.out.println("该位置已经有棋子,请重新输入:");
continue;
} else {
chess_map[row][col] = currentpiece;
break;
}
} else {
System.out.println("非法输入");
}
} else {
System.out.println("非法输入");
sc.next();//将Scanner中的数据取出来,防止死循环
}
}
//落子成功后棋盘需要重新打印
draw_map();
for (int m = 0; m < chess_map.length; m++) {
for (int j = 0; j < chess_map[0].length; j++) {
//第一种,水平方向
boolean case1 = (j + 4 < chess_map[0].length)
&& chess_map[m][j] == currentpiece
&& chess_map[m][j + 1] == currentpiece
&& chess_map[m][j + 2] == currentpiece
&& chess_map[m][j + 3] == currentpiece
&& chess_map[m][j + 4] == currentpiece;
//第二种,垂直方向
boolean case2 = (m + 4 < chess_map.length)
&& chess_map[m][j] == currentpiece
&& chess_map[m + 1][j] == currentpiece
&& chess_map[m + 2][j] == currentpiece
&& chess_map[m + 3][j] == currentpiece
&& chess_map[m + 4][j] == currentpiece;
//第三种,135°角
boolean case3 = (i + 4 < chess_map.length)
&& (j + 4 < chess_map[0].length)
&& chess_map[m][j] == currentpiece
&& chess_map[m + 1][j + 1] == currentpiece
&& chess_map[m + 2][j + 2] == currentpiece
&& chess_map[m + 3][j + 3] == currentpiece
&& chess_map[m + 4][j + 4] == currentpiece;
//第四种,45°角
boolean case4 = (m > 4) && (j + 4 < chess_map[0].length)
&& chess_map[m][j] == currentpiece
&& chess_map[m - 1][j + 1] == currentpiece
&& chess_map[m - 2][j + 2] == currentpiece
&& chess_map[m - 3][j + 3] == currentpiece
&& chess_map[m - 4][j + 4] == currentpiece;
if (case1 || case2 || case3 || case4) {
System.out.println(m % 2 == 0 ? "玩家A胜利" : "玩家B胜利");
break outer;
}
}
}
i++;
}
if(i==100){
System.out.println("平局");
}
}
public static void draw_map () {
System.out.println(" 0 1 2 3 4 5 6 7 8 9");
for (int i = 0; i < chess_map.length; i++) {
//外层循环控制行
System.out.print(i);
for (int j = 0; j < chess_map[i].length; j++) {
//内层循环控制列
if (j == chess_map[i].length - 1) {
//最后一行不打印——
System.out.print(chess_map[i][j]);
} else {
System.out.print(chess_map[i][j] + ro);
}
}
System.out.println();
if (i < chess_map.length - 1) {
//排除最后一行
System.out.println(" │ │ │ │ │ │ │ │ │ │");
}
}
}
}