1. Exercise 2-1 Programming in C is fun!
2. Exercise 2-3 output inverted triangle pattern
3. Exercise 2-4 temperature conversion
4. Exercises 2-6 Calculate the distance of an object in free fall
5. Exercise 2-8 Calculation of Celsius temperature
6. Exercise 2-9 Four arithmetic operations on integers
7. Exercise 2-10 Calculating piecewise functions [1]
8. Exercise 2-11 Calculate piecewise function [2]
9. Exercise 2-12 Output Fahrenheit-Celsius temperature conversion table
10. Exercise 2-13 Find the sum of the first N terms of one-Nth of the sequence
11. Exercise 2-14 Find the sum of the first N items of the sequence of odd numbers
12. Exercise 2-15 Find the sum of the first N items of a simple interleaved sequence
13. Exercise 2-17 Generate the power table of 3
14. Exercise 2-18 Find the number of combinations
15. Exercise 2-1 Find the mean value of integers
16. Exercise 2-2 Tiered electricity price
17. Exercises 2-3 Find the partial sum of squares and reciprocal sequences
18. Exercise 2-4 Find the sum of the first N items of the interleaved sequence
19. Exercise 2-5 Find the sum of the first N terms of the square root sequence
20. Exercise 2-6 Find the sum of the first N terms of the factorial sequence
1. Exercise 2-1 Programming in C is fun!
This question requires writing a program and output a short sentence "Programming in C is fun!".
Input format:
There is no input for this topic.
Output format:
Output the phrase "Programming in C is fun!" on one line.
code:
#include<stdio.h>
int main()
{
printf("Programming in C is fun!\n");
}
2. Exercise 2-3 output inverted triangle pattern
This question requires writing a program to output the specified inverted triangle pattern composed of "*".
Input format:
There is no input for this topic.
Output format:
Output an inverted triangle pattern composed of "*" in the following format.
* * * *
* * *
* *
*
code:
#include<stdio.h>
int main()
{
printf("* * * *\n");
printf(" * * *\n");
printf(" * *\n");
printf(" *\n");
return 0;
}
3. Exercise 2-4 temperature conversion
This question asks to write a program to calculate the temperature in Celsius corresponding to 150°F in Fahrenheit. Calculation formula: C=5×(F−32)/9, where: C means Celsius temperature, F means Fahrenheit temperature, and the output data is required to be an integer.
Input format:
There is no input for this topic.
Output format:
output in the following format
fahr = 150, celsius = integer value of calculated Celsius temperature
code:
#include<stdio.h>
int main()
{
int F=150;
printf("fahr = 150, celsius = %d\n",5*(F-32)/9);
}
4. Exercises 2-6 Calculate the distance of an object in free fall
An object falls freely from a height of 100 meters. Write a program to find the vertical distance it falls in the first 3 seconds. Let the acceleration due to gravity be 10 m/s.
Input format:
There is no input for this topic.
Output format:
output in the following format
height = vertical distance value
Results are rounded to 2 decimal places.
code:
#include<stdio.h>
#include<math.h>
int main()
{
int g=10;
int t=3;
printf("height = %.2lf\n",(double)(g/2*(int)pow(t,2)));
}
5. Exercise 2-8 Calculation of Celsius temperature
Given a Fahrenheit temperature F, this question requires writing a program to calculate the corresponding Celsius temperature C. Calculation formula: C=5×(F−32)/9. The title ensures that both input and output are within the integer range.
Input format:
The input gives a temperature in Fahrenheit on one line.
Output format:
Outputs the integer value of the corresponding Celsius temperature C in one line in the format "Celsius = C".
Input sample:
150
Sample output:
Celsius = 65
code:
#include<stdio.h>
int main()
{
int c;
scanf("%d\n",&c);
printf("Celsius = %d\n",5*(c-32)/9);
}
6. Exercise 2-9 Four arithmetic operations on integers
This question requires writing a program to calculate the sum, difference, product, and quotient of two positive integers and output them. The title ensures that the input and output are all within the integer range.
Input format:
The input is given 2 positive integers A and B in one line.
Output format:
Output the sum, difference, product, and quotient sequentially in the format "A operator B = result" in 4 lines.
Input sample:
3 2
Sample output:
3 + 2 = 5
3 - 2 = 1
3 * 2 = 6
3 / 2 = 1
code:
#include<stdio.h>
int main()
{
int m,n;
scanf("%d %d\n",&m,&n);
if(n!=0){
printf("%d + %d = %d\n",m,n,m+n);
printf("%d - %d = %d\n",m,n,m-n);
printf("%d * %d = %d\n",m,n,m*n);
printf("%d / %d = %d\n",m,n,m/n);
}
return 0;
}
7. Exercise 2-10 Calculating piecewise functions [1]
This question asks to calculate the value of the following piecewise function f(x):
Input format:
The input gives the real number x in one line.
Output format:
Output in the format of "f(x) = result" in one line, where both x and result retain one decimal place.
Input example 1:
10
Output sample 1:
f(10.0) = 0.1
Input example 2:
0
Sample output 2:
f(0.0) = 0.0
code:
#include<stdio.h>
int main()
{
double x;
scanf("%lf\n",&x);
if(x==0) printf("f(%.1lf) = %.1lf\n",x,x);
else printf("f(%.1lf) = %.1lf\n",x,1/x);
return 0;
}
8. Exercise 2-11 Calculate piecewise function [2]
This question asks to calculate the value of the following piecewise function f(x):
Note: You can include math.h in the header file, and call the sqrt function to find the square root, and call the pow function to find the exponentiation.
Input format:
The input gives the real number x in one line.
Output format:
Output in the format of "f(x) = result" in one line, where both x and result have two decimal places.
Input example 1:
10
Output sample 1:
f(10.00) = 3.16
Input example 2:
-0.5
Sample output 2:
f(-0.50) = -2.75
code:
#include<stdio.h>
#include<math.h>
int main()
{
double x;
scanf("%lf\n",&x);
if(x>=0) printf("f(%.2lf) = %.2lf\n",x,pow(x,0.5));
else printf("f(%.2lf) = %.2lf\n",x,pow(x+1,2)+2.0*x+1/x);
return 0;
}
9. Exercise 2-12 Output Fahrenheit-Celsius temperature conversion table
Input 2 positive integers lower and upper (lower≤upper≤100), please output a Fahrenheit-Celsius temperature conversion table with a value range of [lower, upper] and each increment of 2 degrees Fahrenheit.
The calculation formula of temperature conversion: C=5×(F−32)/9, where: C means Celsius temperature, F means Fahrenheit temperature.
Input format:
Enter 2 integers in one line, representing the values of lower and upper respectively, separated by spaces.
Output format:
The first line of output: "fahr celsius"
Then each line outputs a Fahrenheit temperature fahr (integer) and a Celsius temperature celsius (occupies a width of 6 characters, aligns to the right, and retains 1 decimal place).
If the input range is invalid, "Invalid." will be output.
Input example 1:
32 35
Output sample 1:
driving celsius
32 0.0
34 1.1
Input example 2:
40 30
Sample output 2:
Invalid.
code:
#include<stdio.h>
int main()
{
int lower,upper;
int i;
scanf("%d %d",&lower,&upper);
if(lower<=upper)
{
printf("fahr celsius\n");
for(i=lower;i<=upper;i+=2)
{
printf("%d%6.1lf\n",i,(double)(5.0*(i-32)/9.0));
}
}
else printf("Invalid.\n");
return 0;
}
10. Exercise 2-13 Find the sum of the first N terms of one-Nth of the sequence
This question requires writing a program to calculate the sum of the first N items of the sequence 1 + 1/2 + 1/3 + ....
Input format:
The input is given a positive integer N in one line.
Output format:
Output the value S of the partial sum in the format "sum = S" in one line, accurate to 6 decimal places. The title guarantees that the calculation result does not exceed the double precision range.
Input sample:
6
Sample output:
sum = 2.450000
code:
#include<stdio.h>
int main()
{
int n;
scanf("%d",&n);
int i;
double sum=0.0;
for(i=1;i<=n;i++)
{
sum+=1.0/i;
}
printf("sum = %.6lf\n",sum);
return 0;
}
11. Exercise 2-14 Find the sum of the first N items of the sequence of odd numbers
This question requires writing a program to calculate the sum of the first N items of the sequence 1 + 1/3 + 1/5 + ....
Input format:
The input is given a positive integer N in one line.
Output format:
Output the value S of the partial sum in the format "sum = S" in one line, accurate to 6 decimal places. The title guarantees that the calculation result does not exceed the double precision range.
Input sample:
23
Sample output:
sum = 2.549541
code:
#include<stdio.h>
int main()
{
int n;
scanf("%d",&n);
int i;
double sum=0.0;
int d=1;
for(i=1;i<=n;i++)
{
sum+=1.0/d;
d+=2;
}
printf("sum = %.6lf\n",sum);
return 0;
}
12. Exercise 2-15 Find the sum of the first N items of a simple interleaved sequence
This question requires writing a program to calculate the sum of the first N items of the sequence 1 - 1/4 + 1/7 - 1/10 + ....
Input format:
The input is given a positive integer N in one line.
Output format:
Output the value S of the partial sum in the format "sum = S" in one line, accurate to three decimal places. The title guarantees that the calculation result does not exceed the double precision range.
Input sample:
10
Sample output:
sum = 0.819
code:
#include<stdio.h>
int main()
{
int n;
int l=1;
scanf("%d",&n);
int i;
double sum=0.0;
int d=1;
for(i=1;i<=n;i++)
{
sum+=1.0/d*l;
d+=3;
l=-l;
}
printf("sum = %.3lf\n",sum);
return 0;
}
13. Exercise 2-17 Generate the power table of 3
Input a non-negative integer n, generate a power table of 3, and output the value of ~. The power function can be called to calculate the power of 3.
Input format:
The input is given a non-negative integer n on one line.
Output format:
Output n+1 lines in the order of increasing power, and the format of each line is "pow(3,i) = 3 to the i power value". The title ensures that the output data does not exceed the range of long integers.
Input sample:
3
Sample output:
pow(3,0) = 1
pow(3,1) = 3
pow(3,2) = 9
pow(3,3) = 27
code:
#include<stdio.h>
#include<math.h>
int main()
{
int n;
scanf("%d",&n);
int i;
for(i=0;i<=n;i++)
{
printf("pow(3,%d) = %d\n",i,(int)pow(3,i));
}
return 0;
}
14. Exercise 2-18 Find the number of combinations
This question requires writing a program, according to the formula
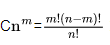
Calculate the number of combinations that take m elements (m≤n) out of n different elements.
It is recommended to define and call the function fact(n) to calculate n!, where the type of n is int and the function type is double.
Input format:
The input gives two positive integers m and n (m ≤ n) on one line, separated by spaces.
Output format:
Output according to the format "result = calculation result of the number of combinations". The title guarantees that the result is within the range of the double type.
Input sample:
2 7
Sample output:
result = 21
code:
#include<stdio.h>
double fun(int n)
{
int i;
double t=1.0;
for(i=1;i<=n;i++)
{
t*=i;
}
return t;
}
int main()
{
int m,n;
scanf("%d %d",&m,&n);
double result=fun(n)/(fun(m)*fun(n-m));
printf("result = %.0lf\n",result);
}
15. Exercise 2-1 Find the mean value of integers
This question requires writing a program to calculate the sum and average of 4 integers. The title ensures that both input and output are within the integer range.
Input format:
The input gives 4 integers in one line separated by spaces.
Output format:
Outputs the sum and the average value sequentially on one line in the format "Sum = sum; Average = average value", where the average value is accurate to one decimal place.
Input sample:
1 2 3 4
Sample output:
Sum = 10; Average = 2.5
code:
#include<stdio.h>
int main()
{
int a1,a2,a3,a4;
scanf("%d %d %d %d",&a1,&a2,&a3,&a4);
printf("Sum = %d; Average = %.1lf\n",a1+a2+a3+a4,(double)(a1+a2+a3+a4)/4.0);
return 0;
}
16. Exercise 2-2 Tiered electricity price
In order to encourage residents to save electricity, a provincial electric power company implements a "tiered electricity price". The electricity price of residential users who install one household and one meter is divided into two "tiers": monthly electricity consumption of less than 50 kWh (including 50 kWh), The electricity price is 0.53 yuan/kwh; if it exceeds 50 kwh, the electricity price will increase by 0.05 yuan/kwh. Please write a program to calculate the electricity bill.
Input format:
Enter the monthly electricity consumption (unit: kWh) of a user given in one line.
Output format:
Output the electricity fee (yuan) that the user should pay in one line, and the result has two decimal places. The format is: "cost = payable electricity fee value"; if the electricity consumption is less than 0, then output "Invalid Value!".
Input example 1:
10
Output sample 1:
cost = 5.30
Input example 2:
100
Sample output 2:
cost = 55.50
code:
#include<stdio.h>
int main()
{
int n;
scanf("%d",&n);
if(n<=50)
{
if(n<0) printf("Invalid Value!\n");
else printf("cost = %.2lf\n",n*0.53);
}
else printf("cost = %.2lf\n",(50*0.53)+(n-50)*(0.53+0.05));
return 0;
}
17. Exercises 2-3 Find the partial sum of squares and reciprocal sequences
This question requires to write a program for two positive integers m and n (m≤n), and calculate the sequence sum

Input format:
The input gives two positive integers m and n (m ≤ n) on one line separated by spaces.
Output format:
Output the value S of the partial sum in the format "sum = S" in one line, accurate to six decimal places. The title guarantees that the calculation result does not exceed the double precision range.
Input sample:
5 10
Sample output:
sum = 355.845635
code:
#include<stdio.h>
int main()
{
int m,n;
scanf("%d %d",&m,&n);
int i;
if(m<=n&&m!=0&&n!=0){
double sum=0.0;
for(i=m;i<=n;i++)
{
sum+=(double)pow(i,2)+1.0/i;
}
printf("sum = %.6lf\n",sum);}
return 0;
}
18. Exercise 2-4 Find the sum of the first N items of the interleaved sequence
This question requires writing a program to calculate the sum of the first N items of the interleaved sequence 1 - 2/3 + 3/5 - 4/7 + 5/9 - 6/11 +....
Input format:
The input is given a positive integer N in one line.
Output format:
Print the values of the partial sums on one line, rounding the result to three decimal places.
Input sample:
5
Sample output:
0.917
code:
#include<stdio.h>
int main()
{
int n;
scanf("%d",&n);
int i;
if(n!=0){
double sum=0.0;
int t1=1;
int t2=1;
int temp=1;
for(i=1;i<=n;i++)
{
sum+=((double)t1/t2)*temp;
t1++;
t2+=2;
temp=-temp;
}
printf("%.3lf\n",sum);}
return 0;
}
19. Exercise 2-5 Find the sum of the first N terms of the square root sequence
This question requires writing a program to calculate the sum of the first N terms of the square root sequence +++…. Can include the header file math.h, and call the sqrt function to find the square root.
Input format:
The input is given a positive integer N in one line.
Output format:
Outputs the value S of the partial sum in the format "sum = S" in one line, accurate to two decimal places. The title guarantees that the calculation result does not exceed the double precision range.
Input sample:
10
Sample output:
sum = 22.47
code:
#include<stdio.h>
#include<math.h>
int main()
{
int n;
scanf("%d",&n);
int i;
//if(n!=0){
double sum=0.0;
for(i=1;i<=n;i++)
{
sum+=sqrt((double)i);
}
printf("sum = %.2lf\n",sum);
//}
return 0;
}
20. Exercise 2-6 Find the sum of the first N terms of the factorial sequence
This question requires writing a program to calculate the sum of the first N items of the sequence 1!+2!+3!+⋯.
Input format:
The input gives a positive integer N not exceeding 12 on one line.
Output format:
Output integer results on one line.
Input sample:
5
Sample output:
153
code:
#include<stdio.h>
#include<math.h>
int main()
{
int n;
scanf("%d",&n);
int i;
//if(n!=0){
long long sum=0;
for(i=1;i<=n;i++)
{
sum+=fun(i);
}
printf("%lld\n",sum);
//}
return 0;
}
int fun(int n)
{
int i;
long long t=1;
for(i=1;i<=n;i++)
{
t=t*i;
}
// printf("t=%lld\n",t);
return t;
}