topic
Now I give you baskets of two colors. When needed, stack baskets with a size difference on top of each other so that when viewed from the top, the colors of the side baskets are interlaced. Now let the computer do this work, it depends on you.
enter
The input is a triplet, namely, the outer basket size n (n is an odd integer satisfying 0<n<80), the central suit character, and the outer basket suit character, and the latter two are ASCII visible characters;
output
Output the stacked basket patterns, the central suit and outer basket suits are stacked alternately from the inner layer, when multiple baskets are stacked, the corners of the outermost basket are always polished off. There should be a row of gaps between stacked baskets.
sample input
11 B A 5 @ W
sample output
AAAAAAAAA ABBBBBBBBBA ABAAAAAAABA ABABBBBBBABA ABAAAAAAAAA
@@@
@WWW@
@W@W@
@WWW@
@@@
The following is a C language program that meets the requirements:
#include <stdio.h>
#include <string.h>
int main() {
int n; char c1, c2;
while (scanf(“%d %c %c”, &n, &c1, &c2) == 3) {
char basket[n][n];
memset(basket, c2, sizeof(basket)); // fill the entire basket with outer color
for (int i = 0; i < (n+1)/2; i++) { // build each layer of the basket
char layer_c = (i % 2 == 0) ? c1 : c2; // determine center or outer color for this layer
int layer_size = n - 2 * i;
int start = i;
int end = n - 1 - i;
// fill top and bottom rows of the layer with outer color
for (int j = start; j <= end; j++) {
basket[start][j] = c2;
basket[end][j] = c2;
}
// fill left and right columns of the layer with outer color
for (int j = start+1; j < end; j++) {
basket[j][start] = c2;
basket[j][end] = c2;
}
// fill the center of the layer with center color
for (int j = start+1; j < end; j += 2) {
for (int k = start+1; k < end; k += 2) {
basket[j][k] = layer_c;
}
}
}
// print the basket
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
printf("%c", basket[i][j]);
}
printf("\n");
}
printf("\n");
}
return 0;
}
The idea of the program is to build a two-dimensional character array representing the pattern of the basket. First fill the entire basket with the outer color, then fill the grid with the center and outer colors layer by layer, and finally output the pattern of the entire basket.
topic
Now I give you baskets of two colors. When needed, stack baskets with a size difference on top of each other so that when viewed from the top, the colors of the side baskets are interlaced. Now let the computer do this work, it depends on you.
enter
The input is a triplet, namely, the outer basket size n (n is an odd integer satisfying 0<n<80), the central suit character, and the outer basket suit character, and the latter two are ASCII visible characters;
output
Output the stacked basket patterns, the central suit and outer basket suits are stacked alternately from the inner layer, when multiple baskets are stacked, the corners of the outermost basket are always polished off. There should be a row of gaps between stacked baskets.
sample input
11 B A 5 @ W
sample output
AAAAAAAAA ABBBBBBBBBA ABAAAAAAABA ABABBBBBBABA ABAAAAAAAAA
@@@
@WWW@
@W@W@
@WWW@
@@@
The following is a C language program that meets the requirements:
#include <stdio.h>
#include <string.h>
int main() { int n; char c1, c2; while (scanf(“%d %c %c”, &n, &c1, &c2) == 3) { char basket[n][n]; memset(basket, c2, sizeof(basket)); // fill the entire basket with outer color
for (int i = 0; i < (n+1)/2; i++) { // build each layer of the basket
char layer_c = (i % 2 == 0) ? c1 : c2; // determine center or outer color for this layer
int layer_size = n - 2 * i;
int start = i;
int end = n - 1 - i;
// fill top and bottom rows of the layer with outer color
for (int j = start; j <= end; j++) {
basket[start][j] = c2;
basket[end][j] = c2;
}
// fill left and right columns of the layer with outer color
for (int j = start+1; j < end; j++) {
basket[j][start] = c2;
basket[j][end] = c2;
}
// fill the center of the layer with center color
for (int j = start+1; j < end; j += 2) {
for (int k = start+1; k < end; k += 2) {
basket[j][k] = layer_c;
}
}
}
// print the basket
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
printf("%c", basket[i][j]);
}
printf("\n");
}
printf("\n");
}
return 0;
}
The idea of the program is to build a two-dimensional character array representing the pattern of the basket. First fill the entire basket with the outer color, then fill the grid with the center and outer colors layer by layer, and finally output the pattern of the entire basket.
topic description
Find the number of integers between 11 and n (including n) that are both prime numbers and palindromic numbers.
enter
An integer n greater than 11 and less than 1000.
output
The number of prime palindromic numbers between 11 and n.
sample
Enter 23
output 1
Prompt palindromic number refers to left-right symmetrical number, such as: 292,333. Source/Category Loop Structure
answer
#include <stdio.h>
int is_prime(int num) {
int i;
if (num == 2 || num == 3) {
return 1;
}
if (num == 1 || num % 2 == 0) {
return 0;
}
for (i = 3; i * i <= num; i += 2) {
if (num % i == 0) {
return 0;
}
}
return 1; }int is_palindrome(int num) {
int rev = 0, tmp = num;
while (tmp != 0) {
rev = rev * 10 + tmp % 10;
tmp /= 10;
}
return num == rev; }int main() {
int n, count = 0, i;
scanf(“%d”, &n);
for (i = 11; i <= n; i++) {
if (is_prime(i) && is_palindrome(i)) {
count++;
}
}
printf(“%d\n”, count);
return 0; }
This topic requires finding the number of integers between 11 and n (including n) that are both prime numbers and palindromic numbers. We can first write two functions is_prime
and is_palindrome
to determine whether a number is a prime number and whether it is a palindrome.
- The function
is_prime
judges whether a number is a prime number, the return value is 1, which means it is a prime number, and the return value is 0, which means it is not a prime number. - The function
is_palindrome
judges whether a number is a palindrome, the return value is 1, which means it is a palindrome, and the return value is 0, which means it is not a palindrome.
In main
the function , we first read in the input integer n
. Then use a loop to iterate over each number between 11 and n, and for each number, count
increment . The final count
output value is sufficient.
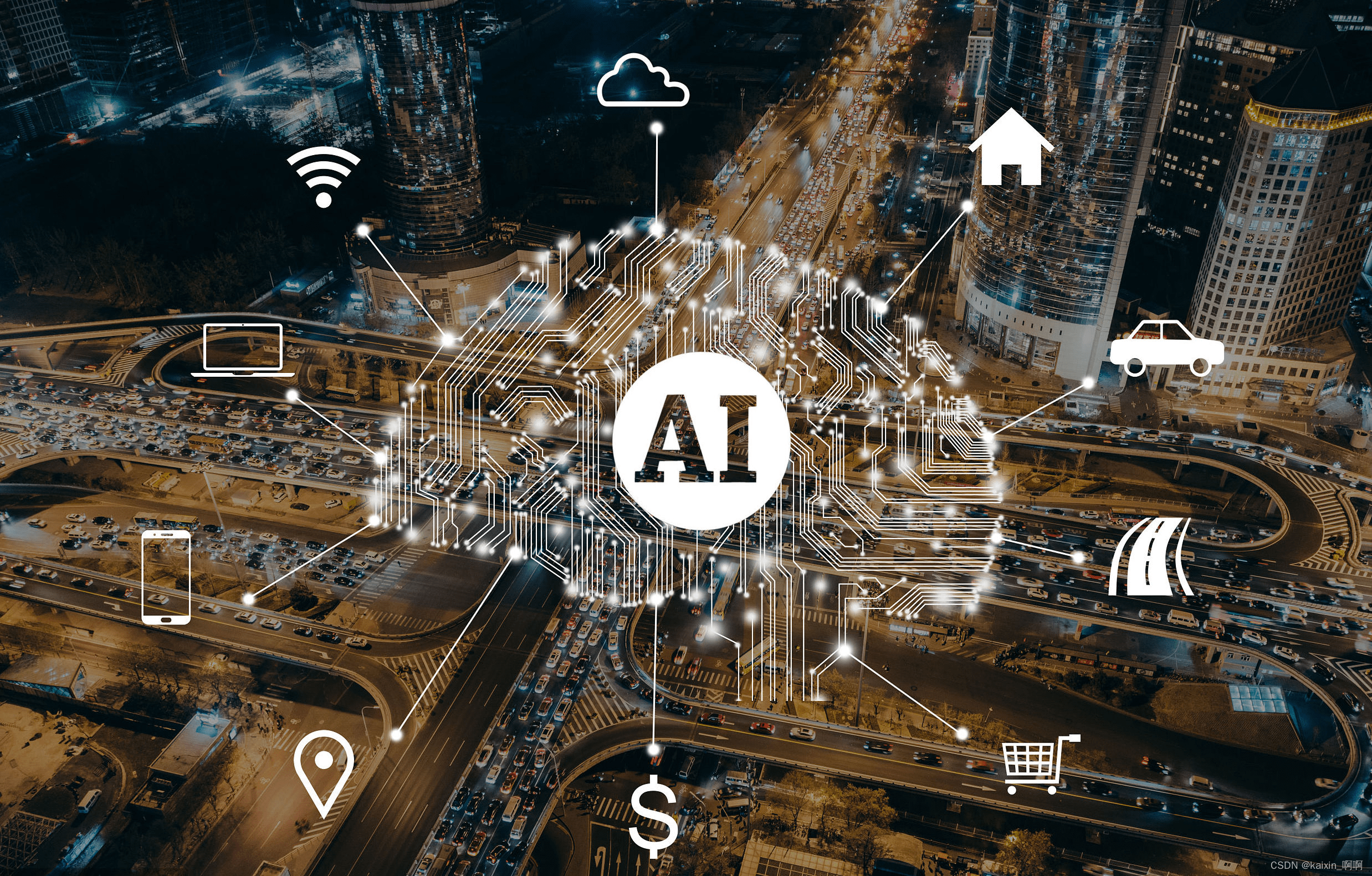