foreword
Array flattening (flattened array) is a knowledge point that is often examined in interviews. I have posted a basic version of array flattening before . Portal
Through the subsequent study, I also learned about some other array flattening operations. For details, please refer to the following article
flat
The flat method can be directly used to flatten an array. When no parameters are passed in, the flat layer is flattened by default.
const arr = ["1", ["2", "3"], ["4", ["5", ["6"]], "7"]];
// 不传参数时,默认“拉平”一层
arr.flat(); // ["1", "2", "3", "4", ["5", ["6"]], "7"]
Pass in an integer parameter, the integer is the number of "flattened" layers.
Passing an integer <=0 will return the original array.
When the "flattened" Infinity keyword is not used as a parameter, it will be converted into a one-dimensional array no matter how many layers of nesting
const arr = [1, [2, 3], [4, [5, [6]], 7]];
// 传入一个整数参数,整数即“拉平”的层数
arr.flat(2);
// [1, 2, 3, 4, 5, [6], 7]
// 传入 <=0 的整数将返回原数组,不“拉平”
arr.flat(0);
arr.flat(-10);
// [1, [2, 3], [4, [5, [6]], 7]];
// Infinity 关键字作为参数时,无论多少层嵌套,都会转为一维数组
arr.flat(Infinity);
// [", 2, 3, 4, 5, 6, 7]
If the original array has space, the flat() method will skip the space.
[1, 2, 3, 4,,].flat(); //[1, 2, 3, 4]
The toString method is a relatively simple method. It converts each element of the array into a string and flattens it directly,
and then traverses each element through the map to make it a Number type .
const arr = [1, [2, 3], [4, [5, [6]], 7]];
arr.toString();
arr.toString().split(',');
arr.toString().split(',').map(item) => Number(item);
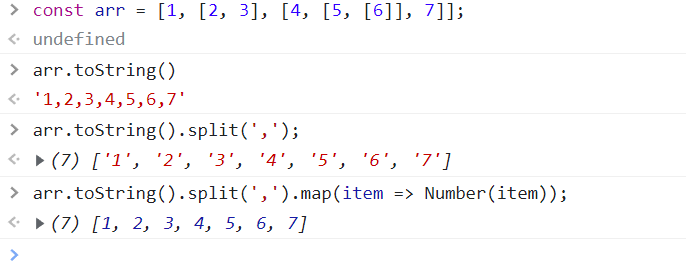
join
The operation of join is similar to that of toString . Finally, each element must be traversed through map to make it a Number type .
const arr = [1, [2, 3], [4, [5, [6]], 7]];
arr.join();
arr.join().split(',');
arr.join().split(',').map(item) => Number(item);
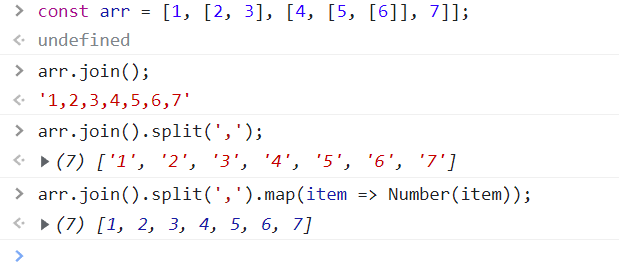
+1
This method is rather strange, it should be converted to string type after arr '+1', it will be automatically aligned
let a = [1, 2, [3, [4, 5]]] + '1'
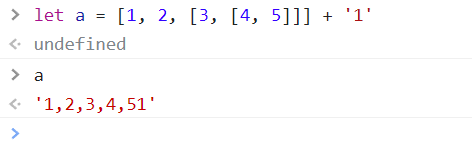
let a = [1, 2, [3, [4, 5]]] + ',1'
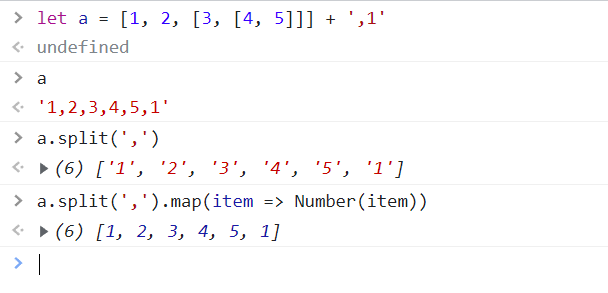
handwritten code
simple array flattening
Before ES2019, it can be realized by reduce + concat
. Because Array.prototype.concat can connect both arrays and single items, it is very clever
const flatten = (list) => list.reduce((a, b) => a.concat(b), []);
A simpler implementation is Array.prototype.concat with the operator
const flatten = (list) => [].concat(...list);
Deep Array Flattening
如果要求深层数组打平,可以加一个判断,递归 调用函数
const flatten = (list) =>
list.reduce((a, b) => a.concat(Array.isArray(b) ? flatten(b) : b), []);
优化上面的代码,加上自定义拍平的层数,默认为 1
function flatten(list, depth = 1) {
if (depth === 0) return list;
return list.reduce(
(a, b) => a.concat(Array.isArray(b) ? flatten(b, depth - 1) : b),
[]
);
}
文章如有错误,恳请大家提出问题,本人不胜感激 。 不懂的地方可以评论,我都会 一 一 回复
文章对大家有帮助的话,希望大家能动手点赞鼓励,大家未来一起努力 长路漫漫,道阻且长