1. Introduction to json
There are two data structures in json: dictionary {} and list []
{
"name": "熊猫",
"like": [
"听歌",
"看书",
"运动"
],
"address": {
"country": "中国",
"city": "上海"
}
}
Strings in json must use double quotes
Adding comments // or /**/ is not allowed in json files, so how can JSON add comments? You can use key: value to add a data element that acts as a comment in json
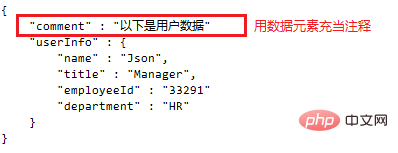
Second, python reads json files
json.load():
import json # 导入包
with open('Info.json', encoding='utf-8') as a:
result = json.load(a) # 导入json文件,a是文件对象,result是一个字典
print(result.get('name')) # 熊猫
print(result.get('address').get('city')) # 上海
Three, python writes json file
Dictionaries and sequences (lists or tuples) in python can be written to json files
json.dump():
my_list = [('熊猫', '听歌', '上海'), ('老虎', '运动', '北京')]
with open('Info2.json', 'w', encoding='utf-8') as b:
json.dump(my_list, b, ensure_ascii=False, indent=2) # 不以ASCII的方式显示(显示中文),indent 缩进
Info2.json:
[
[
"熊猫",
"听歌",
"上海"
],
[
"老虎",
"运动",
"北京"
]
]
4. Convert python dictionary or sequence to json string
json.dumps()
import json
data = {"hi" : "你好", "who" : "全世界"}
json_str = json.dumps(data, indent=4, ensure_ascii=False) # 缩进4,显示中文
print(json_str)
'''
输出:
{
"hi": "你好",
"who": "全世界"
}
'''
reference:
How to add comments to json files? -js Tutorial-PHP Chinese Network
python——reading of json files - Extraordinary - Blog Garden (cnblogs.com)