1. Trie tree
Trie tree is a data structure for efficiently storing and finding collections of strings.
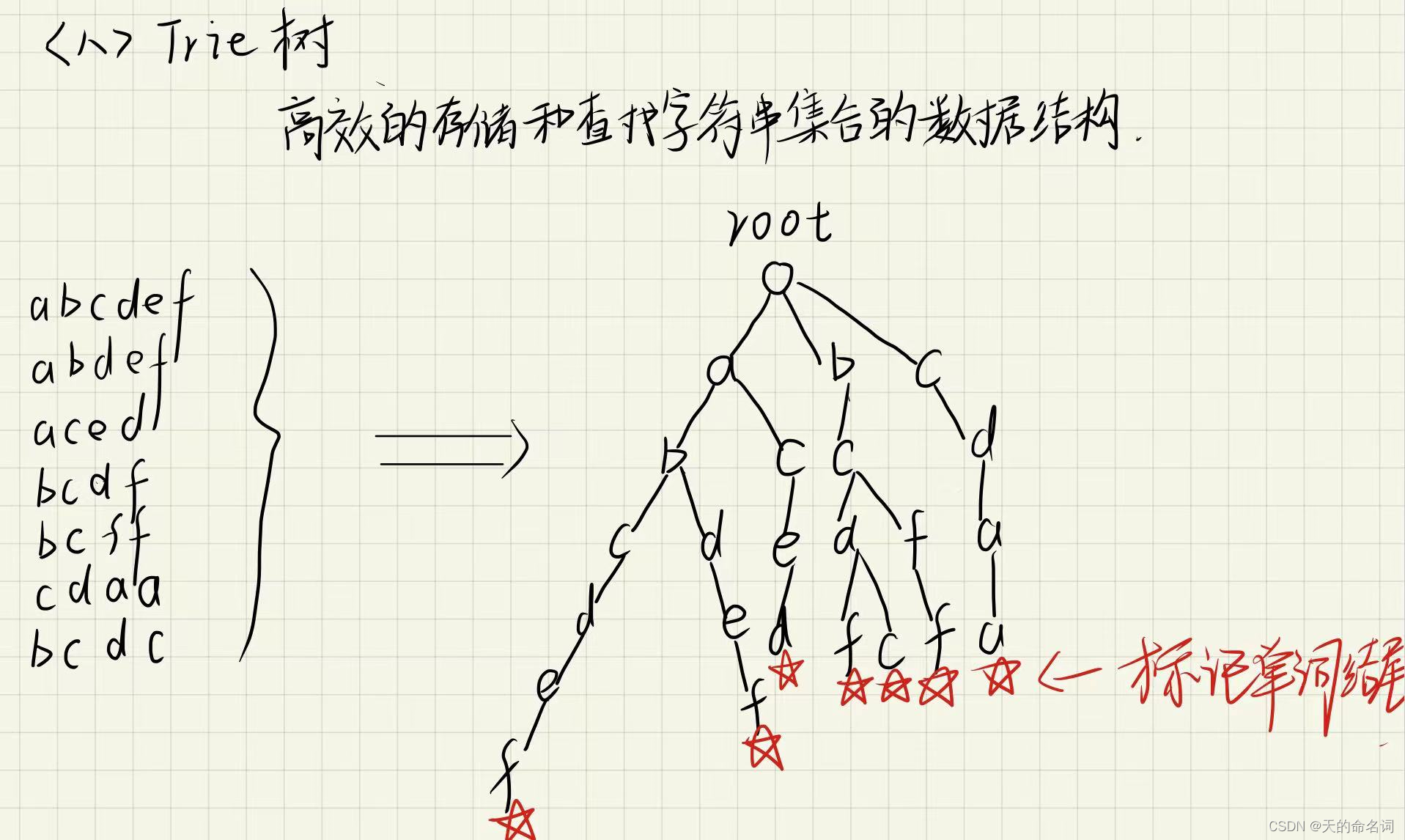
Two, Trie string statistics
Maintain a collection of strings and support two operations:
I x inserts a string x into the collection;
Q x asks how many times a string occurs in the set.
There are N � operations in total, and the total length of all input strings does not exceed 10 5 , and the strings only contain lowercase English letters.
input format
The first line contains the integer N , denoting the operand.
Next N lines, each line contains an operation instruction, and the instruction is one of I x or Q x .
output format
For each query instruction Q x , an integer must be output as a result, indicating the number of times x appears in the set.
Each result occupies one line.
data range
1≤N≤2∗104
Input sample:
5
I abc
Q abc
Q ab
I ab
Q ab
Sample output:
1
0
1
code:
#include <iostream>
using namespace std;
const int N = 100010;
// 每个结点的子节点最多为26
//cnt存储以当前单词结尾的单词有多少个
//idx存储当前用到了哪个下标
//下标是0的点即使根节点,又是空结点
int son[N][26], cnt[N], idx;
char str[N];
void insert(char str[])
{
int p = 0;
for(int i = 0;str[i]; i++)
{
int u = str[i] - 'a'; //当前字母的编号
if(!son[p][u]) son[p][u] = ++idx;
p = son[p][u];
}
cnt[p]++;
}
int query(char str[])
{
int p = 0;
for (int i = 0; str[i]; i ++ )
{
int u = str[i] - 'a';
if (!son[p][u]) return 0;
p = son[p][u];
}
return cnt[p];
}
int main()
{
int n;
cin>>n;
while (n -- )
{
char op[2];
cin >> op >> str;
if(op[0] =='I') insert(str);
else cout << query(str) <<endl;
}
return 0;
}
Three, the largest XOR pair
Select two of the given N integers A 1 , A 2 ... A N for xor (exclusive OR) operation, what is the maximum result obtained?
input format
The first line enters an integer N.
The second line inputs N integers A 1 to A N .
output format
Output an integer representing the answer.
data range
1≤N≤105
0≤Ai<231
Input sample:
3
1 2 3
Sample output:
3
code:
1. Violent solution
#include <iostream>
#include <algorithm>
using namespace std;
const int N =1e5;
int n;
int a[N];
int main()
{
cin >> n;
for(int i = 0; i < n; i++) cin >> a[i];
int res = 0;
for(int i = 0; i < n;i++)
for (int j = 0; j < n; j ++ )
res = max(res,a[i]^a[j]);
cout << res << endl;
return 0;
}
2.Trie tree solution
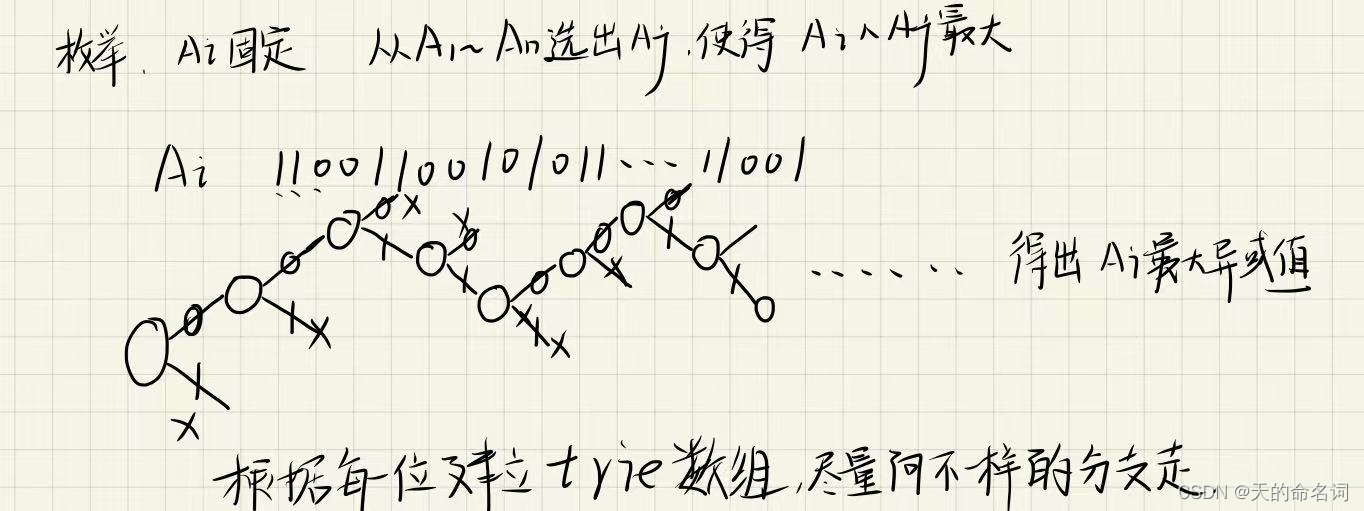
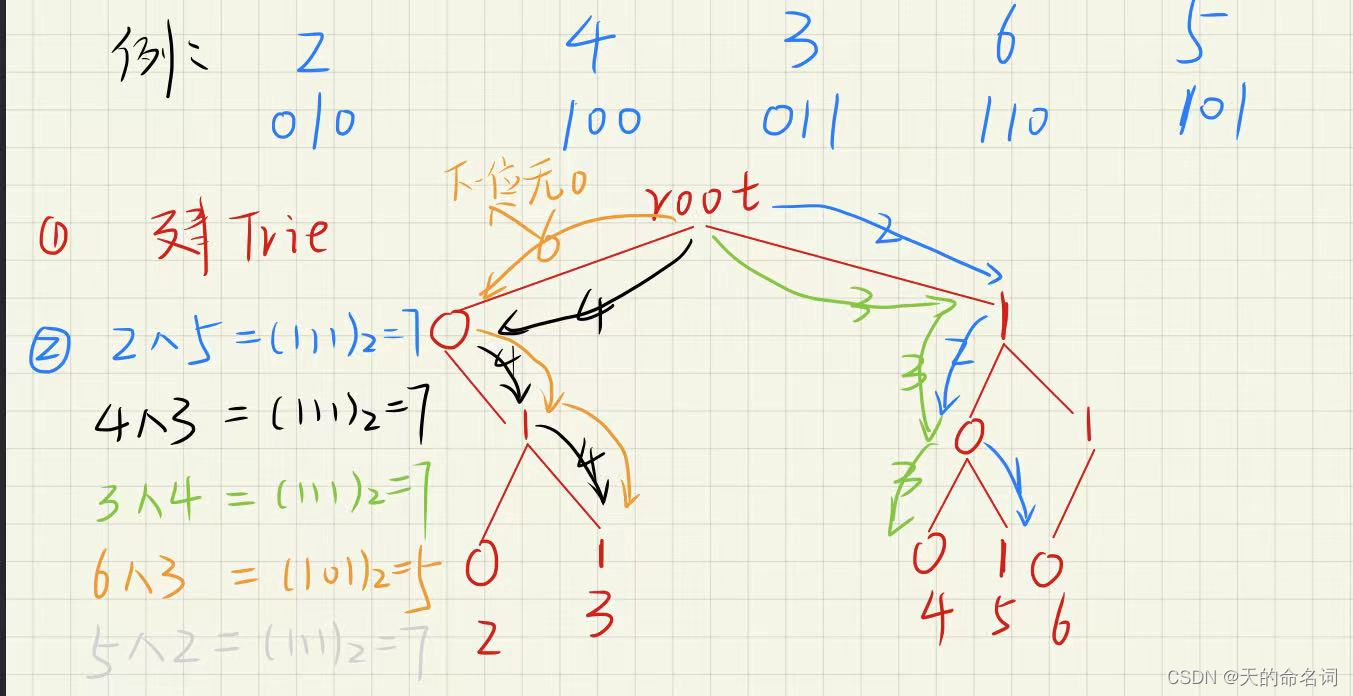
#include <iostream>
#include <algorithm>
using namespace std;
const int N =100010, M = 3100010;
int n;
int son[M][2], idx;
int a[N];
void insert(int x)
{
int p = 0;
for( int i = 30; i>=0; i--)
{
int &s = son[p][x >> i & 1];
if(!s) s = ++ idx;//创建新结点
p = s;
}
}
int query(int x)
{
int res= 0,p = 0;
for (int i = 30; i >=0; i-- )
{
int s= x>>i &1;
if(son[p][!s])
{
res +=1<<i;
p = son[p][!s];
}
else p = son[p][s];
}
return res;
}
int main()
{
cin >> n;
for(int i = 0; i < n; i++)
{
cin >> a[i];
insert(a[i]);
}
int res = 0;
for(int i = 0; i < n;i++) res = max(res,query(a[i]));
cout << res<<endl;
return 0;
}