Article Directory
1. The relationship between physical memory and disk
How to understand physical memory?
The width of the physical memory is 1 byte.
If you use the C language, you can define the char type (1 byte), and you can map the 1-byte unit into the memory in the virtual address space.
If you think that memory is divided in units of bytes, it is actually incorrect
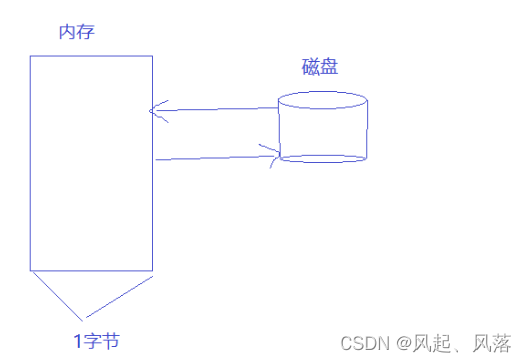
- Swapping data from the disk into the physical memory and vice versa is a high-frequency work. The physical disk is a mechanical device. As a peripheral, it will be very slow as a whole, making the efficiency of the whole machine low
. More IO will lead to too many addressing operations, that is, inefficiency - When there is 100MB of space to write to the disk, is it more efficient to write to the disk once, or to write 100 times and write 1MB of data each time?
- 1 write to disk is definitely higher since only one seek operation is required
- Explain that when the OS and the disk perform IO interaction, it is definitely not in units of bytes, but in units of blocks (4KB)
- If you only want to modify one bit, you must also IO4KB, because 4KB as a block is a whole
To load a file into memory, in units of 4KB, two aspects need to be considered
1. If the file system + compiler
wants to read 4KB from the peripheral disk, the premise is that the file system recognizes 4KB, and the file itself is stored as 4KB. Therefore,
when the file is on the disk, the 4KB file in block units also
includes executable programs. And dynamic libraries also need to be stored on the disk, and they must be stored on the disk in units of 4KB
2. Operating system + memory
When the disk stores data in the form of 4KB, the physical memory also provides space for storing 4KB of data
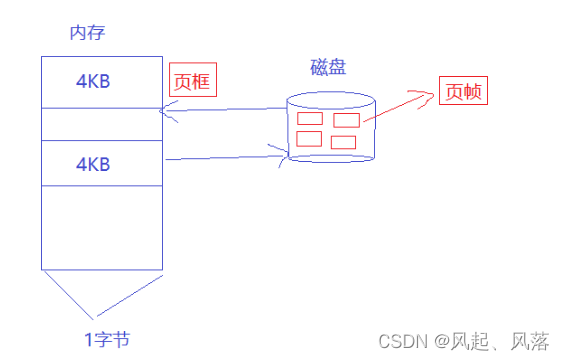
The space for storing data in the physical memory is called a page frame
. The data block in the disk is called a page
frame memory. When actually performing memory management, the unit is also 4KB.
The essence of memory management: put a specific 4KB block (data content) in the disk into the 4KB space (data storage space) of physical memory
Why should the physical memory be divided into 4KB each?
The operating system needs to manage these configurations
, and the essence of management is to describe first. In the
kernel
, there is a corresponding configuration data structure struct page to manage the corresponding configuration.
The struct page is a structure that contains the state of this configuration
and then organized
through an array way to manage
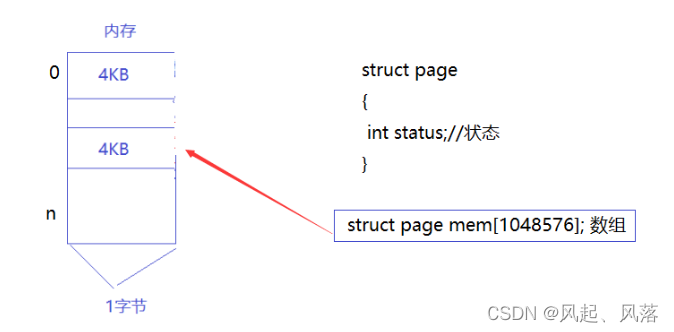
Arrays have subscripts, and blocks are numbered 0-n in physical memory.
If you want to query which locations in physical memory are occupied, you can see the corresponding state in the corresponding structure by finding the corresponding array subscript.
If not assigned, set the status to 1
If it is stored in blocks, is the extra space wasted?
Assuming that accessing a piece of code is only 10 bytes, and loading it into the disk in 4KB blocks, there will be an extra 2KB of space
The characteristic of the principle of locality, which allows early loading of adjacent or nearby data that is accessing the data
Reduce the number of future IOs by preloading the data near the data to be accessed
. The essence of loading more data is data preloading
If you don't understand yet, click to view: Detailed explanation of the principle of locality
2. Virtual address to physical address translation
A virtual address corresponds to 32 bits
The virtual address is not used as a whole, it is divided into 10 +10 +12
The current page table needs 2^10 rows
According to the binary sequence of the first 10 bits, find the corresponding position of page table 1
The left side of page table 1 represents the first 10 bits, and the right side represents the start address of another page table
According to the second 10 bits, find the corresponding position of page table 2.
The left side of page table 2 represents the second 10 bits, the right side represents the physical address (the start address of the page frame)
corresponding to the start address of the page frame + the address data corresponding to the lower 12 bits of the virtual address is essentially an intra-page offset
Navigate to any address within the page frame
3. Page fault interrupt
After applying for physical memory, it may not be used immediately. If you apply for physical memory immediately, the memory will always be occupied and idle, which is not an efficient performance.
When actually applying for malloc memory, the operating system only needs to apply for you in the virtual address space. When actually accessing, the operating system will automatically apply or fill the page table and apply for specific physical memory
The MMU cannot find the page table, but it is true that the space has been applied, and a page fault interrupt will be triggered.
The OS will execute the appropriate processing method, that is, apply for physical memory to fill the page table, and then return to continue executing the code.
4. Why is the character constant area not allowed to be modified?
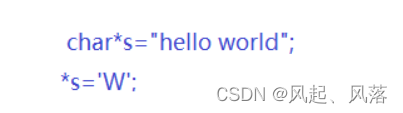
hello world is in the constant area and can only be read but not modified, so *s='w' is wrong
What is stored in s is the virtual start address of the character pointed to
*s addressing, it will be accompanied by the conversion from virtual address to physical address
By looking up the page table, the operation authority is queried, and it is found that there is only read authority, but no modify authority, so the MMU (memory management unit) is abnormal, the OS recognizes the exception, and sends a signal to the target process, which is converted
into In user mode, perform signal processing - terminate the process
5. Disadvantages of threads
1. Performance loss
Too many threads are created, only a few are running, and most threads are scheduled back and forth, resulting in performance loss, that is, multi-thread creation is unreasonable
2. Reduced robustness
If a thread has a problem, it may affect the entire process
3. Lack of access control
Each execution flow sees the same resources through the same address space
Validation for reduced robustness
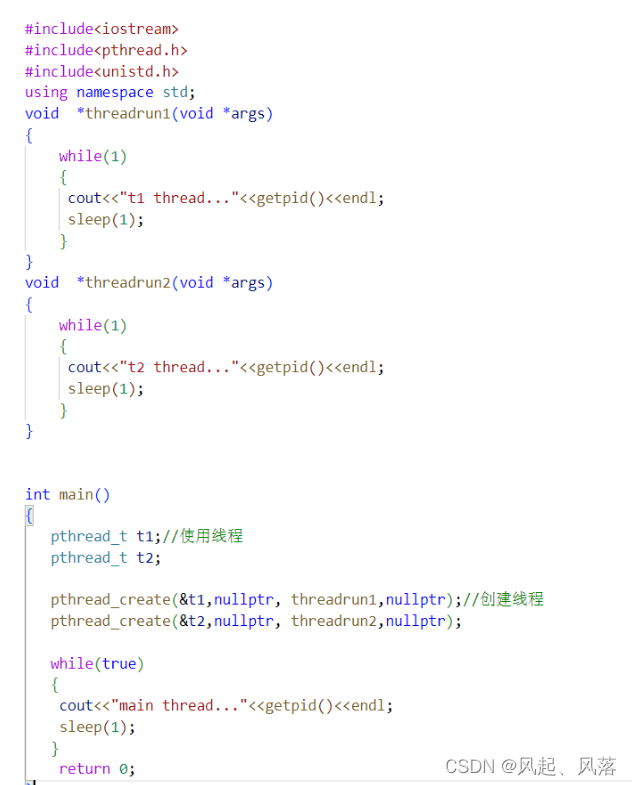
There are three dead loops in total. If there is only one execution flow, the three dead loops cannot be executed.
If all of them are executed, there must be three execution flows.

shows that there are three execution flows
LWP is called a lightweight process, that is, a thread.
The PID of each thread is the same, indicating that it belongs to the same process.
The PID and LWP are the same, indicating that it is the main thread.
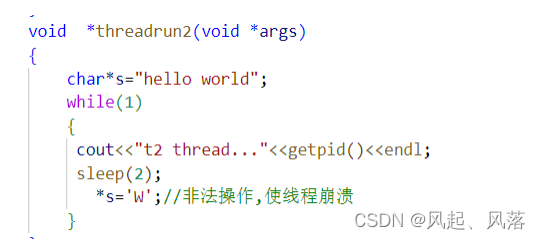
Illegal operation set in thread 2, crashing the thread
A segfault will occur during runtime. The thread can run at the beginning, but after the segfault occurs, the process crashes
In a multi-threaded program, any thread crashes, eventually causing the process to crash
Lack of authentication for access control
Define a global variable, thread 1 and the main thread print out the address of the global variable and the data of the global variable,
while thread 2 prints the address and data of the global variable, and prints the data ++
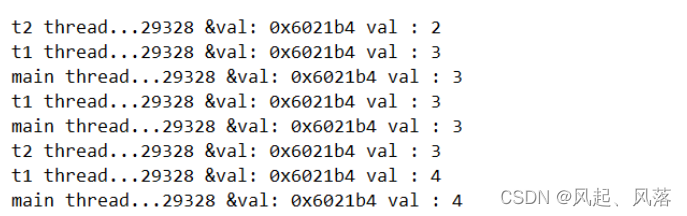
When the data in one thread is modified, the data in all threads are modified accordingly
Taking global variables as an example, in a multi-threaded scenario, multiple threads see the same global variable