Json multiple choice questions: https://blog.csdn.net/falsedewuxin/article/details/129651872?spm=1001.2014.3001.5502
Json multiple choice questions: https://blog.csdn.net/falsedewuxin/article/details/129653639?spm=1001.2014.3001.5502
1. Make a simple UI answer panel and score panel
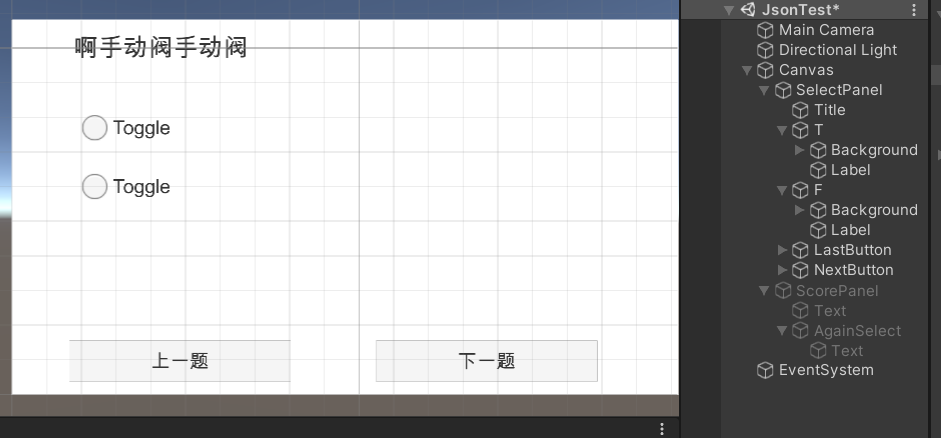
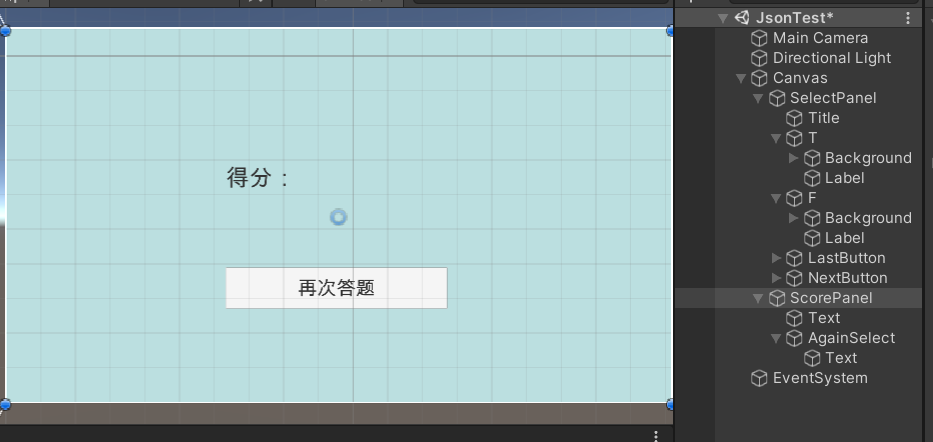
2. Create a new DataTest folder, and create a new Json file MyData in this folder
Note: The encoding format of the Json file should be UTF-8, otherwise there will be Chinese garbled characters (you can create the json file, use Notepad to open it, and then save it as, change the encoding), if it has been saved as and change the encoding to UTF-8, go back to unity and click on the file to view and find that the characters are still garbled, you can try to create a new word file, change the word file to a json file, and then use Notepad to save it as UTF-8 encoding format
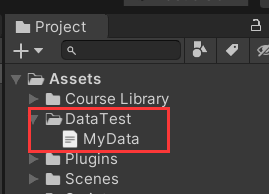
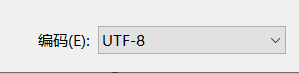
3. Put the question data into json (the question and answer are scribbled)
{
"selects": [
{
"title": "1.c#的数据类型有",
"selectAnswer": [ "T", "F"],
"answer": "F"
},
{
"title": "2.下列描述错误的是",
"selectAnswer": [ "T", "F"],
"answer": "T"
},
{
"title": "3.下列关于构造函数的描述正确的是",
"selectAnswer": [ "T", "F"],
"answer": "T"
},
{
"title": "4.装箱、拆箱操作发生在",
"selectAnswer": [ "T", "F" ],
"answer": "F"
},
{
"title": "5.下列选项中可以被渲染的纹理是",
"selectAnswer": [ "T", "F" ],
"answer": "T"
}
]
}
Check whether the json format is correct: https://www.bejson.com/
4. Add a Toggle Group component to the Canvas, check the Allow Switch Off property, select four toggles, and drag the Canvas to the Group property of the toggle component of the four toggles
5. Mount the script to the Canvas, and drag the corresponding object to the script properties
using System.Collections;
using System.Collections.Generic;
using System.IO;
using UnityEngine;
using UnityEngine.UI;
public class JsonTest : MonoBehaviour
{
Selections data;
//题目
public Text titleText;
//选项
public Toggle[] toggles = new Toggle[2];
//记录自己选择的所有答案
List<string> answers = new List<string>();
//记录答题时的临时答案
string ans = null;
//拿题库里面的答案
List<string> allAnswers = new List<string>();
int currentIndex = 0;
public Button last;
public Button next;
//得分
int score = 0;
//得分面板
public GameObject scorePanel;
// Start is called before the first frame update
void Start()
{
//Application.dataPath这个只对PC有效
string path = Application.dataPath + "/DataTest/MyData.json";
string str = File.ReadAllText(path);
data = JsonUtility.FromJson<Selections>(str);
//记录题库中的所有答案
for (int i = 0; i < data.selects.Count; i++)
{
allAnswers.Add(data.selects[i].answer);
}
for (int i = 0; i < allAnswers.Count; i++)
{
answers.Add(null);
}
ShowSelect(currentIndex);
}
//加载题目
public void ShowSelect(int index)
{
//显示题目
titleText.text = data.selects[index].title;
//显示题目
for (int i = 0; i < toggles.Length; i++)
{
toggles[i].transform.GetChild(1).GetComponent<Text>().text = data.selects[index].selectAnswer[i];
}
}
//检测选项是否点击
public void Tt(bool isSelect)
{
if (isSelect)
ans = "T";
else
ans=null;
}
public void Ft(bool isSelect)
{
if (isSelect)
ans = "F";
else
ans = null;
}
//下一题
public void NextSelect()
{
answers.RemoveAt(currentIndex);
answers.Insert(currentIndex, ans);
currentIndex++;
ans = null;
if (currentIndex < allAnswers.Count)
{
ShowSelect(currentIndex);
ShowAnswer(currentIndex);
}
else
{
for (int i = 0; i < allAnswers.Count; i++)
{
if (allAnswers[i].Equals(answers[i]))
{
score += 20;
}
}
scorePanel.SetActive(true);
scorePanel.transform.GetChild(0).GetComponent<Text>().text = "得分:" + score;
}
}
//上一题
public void LastSelect()
{
currentIndex--;
ans = null;
ShowSelect(currentIndex);
ShowAnswer(currentIndex);
}
//显示当前题目是否已经作答
public void ShowAnswer(int index)
{
for (int i = 0; i < toggles.Length; i++)
{
toggles[i].isOn = false;
}
switch (answers[index])
{
case "T":
toggles[0].isOn = true;
break;
case "F":
toggles[1].isOn = true;
break;
default:
break;
}
}
//再次答题按钮
public void AgainSelect()
{
answers.Clear();
for (int i = 0; i < allAnswers.Count; i++)
{
answers.Add(null);
}
score = 0;
currentIndex = 0;
ShowSelect(currentIndex);
ShowAnswer(currentIndex);
scorePanel.SetActive(false);
}
// Update is called once per frame
void Update()
{
//隐藏和显示上一题的按钮
if (currentIndex > 0)
{
last.gameObject.SetActive(true);
}
else
{
last.gameObject.SetActive(false);
}
if (currentIndex < allAnswers.Count-1)
{
next.transform.GetChild(0).GetComponent<Text>().text = "下一题";
}
else
{
next.transform.GetChild(0).GetComponent<Text>().text = "结束答题";
}
}
}
[System.Serializable]
public class SelectSingle
{
//名称要和SelectData.json中selects中的属性名中的一一对应
public string title;
public List<string> selectAnswer = new List<string>();
public string answer;
}
[System.Serializable]
public class Selections
{
//这里的selects必须和SelectData.json中的selects中一样才行
public List<SelectSingle> selects = new List<SelectSingle>();
}
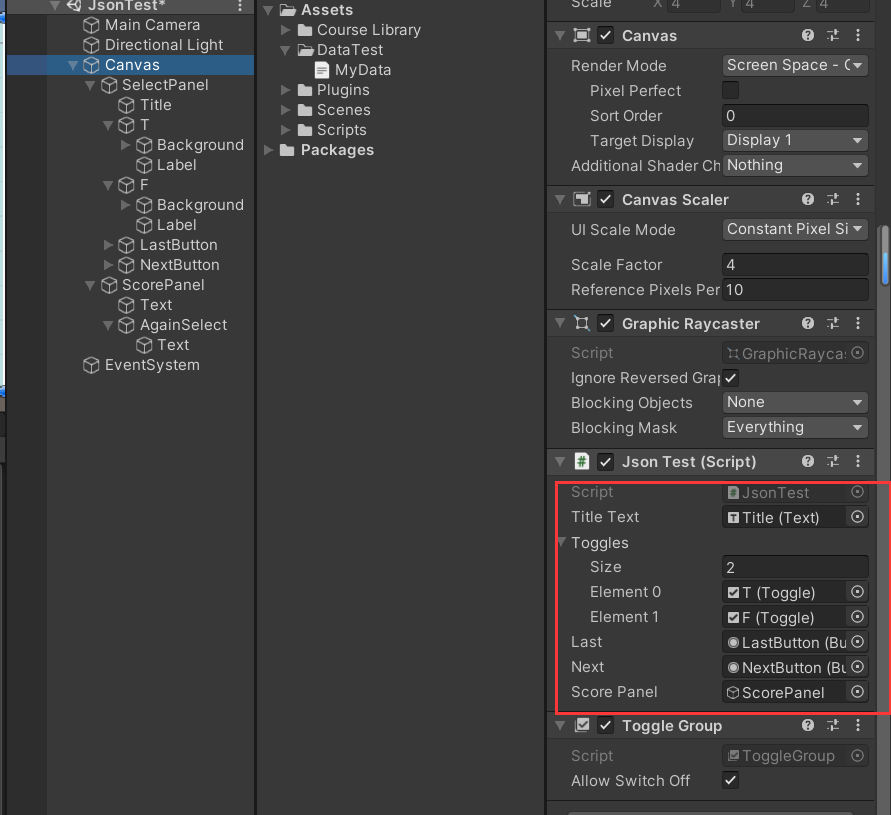
6. Add the corresponding methods in the script for different toggles and buttons
toggle
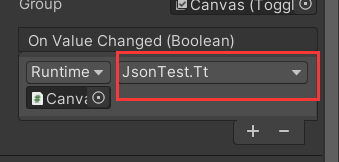
button
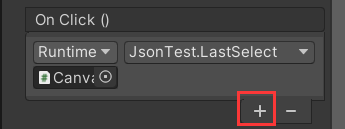
7. Just run unity
Note: If there is a bug, please correct me
Conclusion: The tree that embraces is born at the end of the hair; the nine-story platform starts from the pile of soil; the journey of a thousand miles begins with a single step.