Article directory
- JS
-
- js object deep copy
- js random color
- Array object sorting
- Compatible with PC and mobile events
- PC effect! [Insert picture description here] (https://img-blog.csdnimg.cn/aeffbae0b30b4d838617f332db04e928.png)
- scrollIntoView scrolls to the visible area
- Understand call apply bind in 10 minutes
- addEventListener - event binding
- div input box
- Vue
- CSS
JS
js object deep copy
/**
* 深拷贝对象
* @param {Object,Array} obj 要复制的对象
* @returns {Object,Array} 返回一个复制的对象
*/
const copyObj = obj => {
// 非对象 {}
const isObj = typeof obj !== 'object' || obj === null
if (isObj) return obj
// 是数组 []
const isArr = Array.isArray(obj)
// 确认数据类型
const newObj = isArr === true ? [] : {
}
// 遍历对象
for (const key in obj) {
newObj[key] = copyObj(obj[key])
}
return newObj
}
const a1 = [
{
a: [
{
b: ['我是老6']
}
]
}
]
const a2 = copyObj(a1)
a1[0].a[0].b = '我不是老666666666666'
console.log(a1)
console.log(a2)
operation result
js random color
function ramColor() {
//随机生成RGB颜色
const arr = [];
for (let i = 0; i < 3; i++) {
// 暖色
// arr.push(Math.floor(Math.random() * 128 + 64));
// 亮色
arr.push(Math.floor(Math.random() * 128 + 128));
}
const [r, g, b] = arr;
// rgb颜色
// var color=`rgb(${r},${g},${b})`;
// 16进制颜色
const color = `#${
r.toString(16).length > 1 ? r.toString(16) : "0" + r.toString(16)
}${
g.toString(16).length > 1 ? g.toString(16) : "0" + g.toString(16)}${
b.toString(16).length > 1 ? b.toString(16) : "0" + b.toString(16)
}`;
return color;
}
End
2023/4/20 9:21
Array object sorting
const arr = [{
age: 1 }, {
age: 100 }, {
age: 23 }, {
age: 87 }, {
age: 4 }, {
age: 10 }, {
age: 27 }, {
age: 99 }, {
age: 54 }, {
age: 23 }, {
age: 55 }, {
age: 23 }, {
age: 87 }]
console.log(sortArrKey(arr, 'age', 1));
/**
* @param {Array} arr 要排序的数组
* @param {string} sortKeyName 排序的键(Key)
* @param {Number} sortType 排序类型 1是正序 非1是负序
* @return {Array} 返回一个排序的数组
*/
function sortArrKey(arr, sortKeyName, sortType = 1) {
return arr.sort((o1, o2) => sortType === 1 ? o1[sortKeyName] - o2[sortKeyName] : o2[sortKeyName] - o1[sortKeyName])
}
Demo
End
2023/4/19 12:07 Series
Compatible with PC and mobile events
1. Encapsulation is compatible with PC and mobile events
src/tuil/events.js
/**
* 判断是PC端还是移动端
* 通过不同的设备定义不同的事件名
*/
const isMobile =
navigator.userAgent.match(
/(phone|pad|pod|iPhone|iPod|ios|iPad|Android|Mobile|BlackBerry|IEMobile|MQQBrowser|JUC|Fennec|wOSBrowser|BrowserNG|WebOS|Symbian|Windows Phone)/i
) !== null
const events = isMobile
? ['touchstart', 'touchmove', 'touchend']
: ['mousedown', 'mousemove', 'mouseup']
export {
events
}
2. use
If used in vue
src/views/Home.vue
<template>
<div ref='div'>home</div>
</template>
<script setup>
import {
ref, onMounted } from 'vue'
//引入
import {
events } from '@/utils/event'
const [start, move, end] = events
console.log(start, move, end);
//获取元素
const div = ref(null)
onMounted(() => {
// 使用
div.value.addEventListener(start, _ => console.log('你好呀'))
})
</script>
pc effect
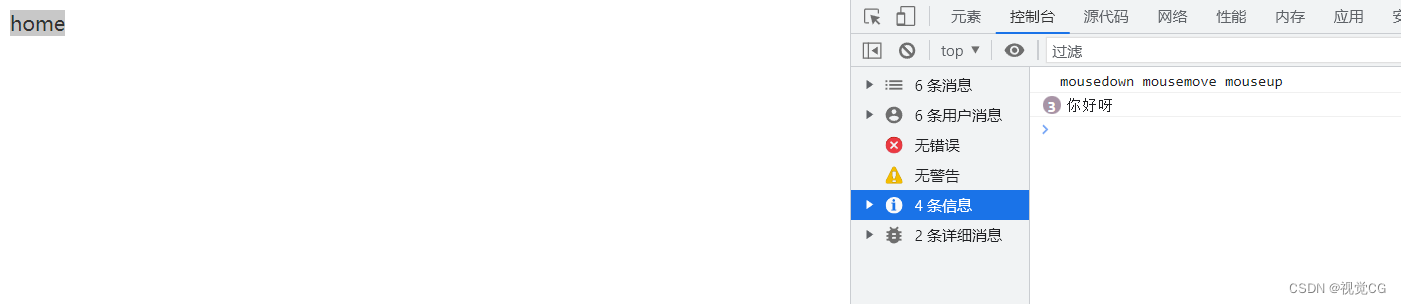
mobile effect
End
2023/4/17 19:19 Sunny
scrollIntoView scrolls to the visible area
basic usage
el.scrollIntoView()
el.scrollIntoView(true)
el.scrollIntoView(false)
el.scrollIntoView(opts)
el.scrollIntoView({
behavior: 'smooth', block: 'start', inline: 'nearest' })
el.scrollIntoView({
behavior: 'auto', block: 'start', inline: 'nearest' })
el.scrollIntoView(opts)
- el element to scroll, such as div
- opts:
- true scrolls to the top of the parent
- false scroll to bottom of parent
- { behavior: 'smooth', block: 'start', inline: 'nearest' } When the parameter is an object↓
- behavior
- auto no animation
- smooth smooth transition
- inline horizontal alignment (default start)
- start
- center
- end
- nearest
- block vertical alignment (default start)
- start
- center
- end
- nearest
- behavior
Understand call apply bind in 10 minutes
name | address |
---|---|
call apply bind - transfer [novice tutorial] - invasion and deletion | https://www.runoob.com/w3cnote/js-call-apply-bind.html |
call apply bind - turn - standby | https://www.runoob.com/w3cnote/js-call-apply-bind.html |
call apply bind summary
- call apply bind function => change this point
name | usage |
---|---|
call | 对象.call(this指向,参数1,参数2...) |
apply | 对象.apply(this指向,[参数1,'参数2'....].) |
bind | 对象.bind(this指向,参数1,参数2...) |
3 difference
the difference |
---|
call , apply , and bind the first parameter are all改变this指向 |
3. When the first parameter is passed null or undefined , this points to window , such asfn.call(null,...agrs) |
call Same as bind passing parameters, such as fn.call(null,...agrs) ,fn.bind(null,...agrs) |
apply The parameter is passed as an array, such asfn.aapply(null,['a','b']) |
call , apply Only change the this point once, and execute the function once immediately |
bind Permanently change the point of this, and the function will not be executed immediately , such as: fn1.bind(obj1) the fn1 function will not be executed immediately, for example fn2.bind(obj2)() , adding parentheses will immediately execute the fn2 function |
apply and call is a one-time input parameter, which bind can be divided into multiple input |
2023/3/11 10:17
addEventListener - event binding
el.addEventListener(event,callback)
For binding events, such as click (click)- The element to which el is bound
- event event
- callback callback function
example:
HTML元素.addEventListener("事件名", function(){
// js代码
});
PC event
event name | effect |
---|---|
click | Click event - note: the mobile terminal can also be triggered |
context menu | right click |
dblclick | Double-click - note: the mobile terminal can also be triggered |
mousedown | mouse down |
mouseup | mouse release |
mouseenter | Fired when the mouse pointer moves over the element - note: only fires once |
mouseover | Move the mouse over the element - note: only trigger once |
mousemove | Triggered when the mouse is moved - Note: Triggered multiple times |
mouseleave | Triggered when the mouse pointer moves out of the element |
mouseout | Mouse out of element |
keyboard events | …slightly |
example
HTML元素.addEventListener('mouseenter', e =>{
console.log('0')})
HTML元素.addEventListener('mouseleave', e =>{
console.log(e)
})
Mobile event (touch)
event name | effect |
---|---|
touchstart | finger pressed |
touchmove | finger movement |
touchend | finger away |
touches | List of all fingers |
targetTouches | first finger |
changedTouches | A list of fingers involved in the current event |
example
HTML元素.addEventListener('touchstart', e=>{
console.log(e)})
HTML元素.addEventListener('touchmove', e=>{
console.log(e)
})
2023/3/11 14:21
div input box
Add contenteditable="true"
attributes to div, which can be used as an input box
- Function :
- Textarea and input input boxes cannot insert pictures and other elements
- Div can not only input text, but also insert elements, such as converting
img
pictures into emoticon input,
Vue
scrollIntoView is invalid in Vue | scrollIntoView in Vue is invalid
Solve the failure of Vue to use scrollIntoView
- Just use scrollIntoView in nextTick
example
<script setup>
import {
nextTick } from 'vue';
nextTick(()=> {
HTML元素.scrollIntoView({
behavior: 'smooth', block: 'start', inline: 'nearest' })
})
</script>
2023/3/10 18:46
CSS
Text is forced not to wrap
- must be set
width
.user{
width: 100%;
white-space:nowrap;
word-wrap: break-all;/* 英文不换行 */
}
multiline text hidden
.txt{
overflow: hidden;
text-overflow: ellipsis;
display: -webkit-box;
-webkit-line-clamp: 3; /* 保留几行 */
-webkit-box-orient: vertical;
}
single line text hidden
.txt{
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
width: 100pvw; /* 必须设置宽 */
white-space:nowrap;
word-wrap: break-all;/* 英文不换行 */
}
css text omission table
required attributes | effect |
---|---|
overflow: hidden | beyond hidden text |
text-overflow: ellipsis; | Show ellipsis beyond |
-webkit-line-clamp: 3; | keep a few lines |
2023/3/12 9:19
Rare attribute
attribute name | value | effect | type |
---|---|---|---|
caret-color | Color value, for example red | Set the input box cursor color | CSS |
contenteditable | false 、true | Set html element as input box | HTML |
The image is aligned horizontally with the text (vertical-align)
available for comment box
.emjio {
vertical-align: middle;
}
renderings
2023/3/17 22:08
range slider beautification
input[type=range] {
margin: 0;
-webkit-appearance: none;
appearance: none;
background-color: transparent;
}
input[type=range]::-webkit-slider-runnable-track {
height: 4px;
background: #eee;
}
input[type=range]::-webkit-slider-container {
height: 20px;
overflow: hidden;
}
input[type=range]::-webkit-slider-thumb {
-webkit-appearance: none;
appearance: none;
width: 20px;
height: 20px;
border-radius: 50%;
cursor: pointer;
background-color: #f44336;
border: 1px solid transparent;
margin-top: -8px;
border-image: linear-gradient(#f44336, #f44336) 0 fill / 8 20 8 0 / 0px 0px 0 2000px;
}
Effect
End
2023/4/19 23:46