Table of contents
(1) Content rendering instructions
(2) Attribute binding instructions
(3) Event binding instructions
(4) Two-way binding instructions
Edit (5) Conditional rendering instructions
(6) List rendering instructions
(1) Parent to child component data sharing
(2) Child to parent component data sharing
(3) Brother component data sharing
(1) Private custom instructions
(2) Global custom instructions
(4) Declarative Navigation & Programmatic Navigation
(5) vue-router programmatic navigation API
1. Introduction to vue
1. Concept
Front-end framework for building user interfaces
2. Characteristics
Two major features of vue: data-driven view, two-way data binding
(1) Data Driven View
- Data changes will drive the view to update automatically. When the data changes, vue will monitor the data changes and automatically re-render the page structure (no need to manually manipulate the DOM)
- One-way data binding (data changes cause page changes)
(2) Two-way data binding
- When filling in the form, two-way data binding can assist developers to automatically synchronize the content filled by the user to the data source without manipulating the DOM
- When the js data changes, it will be automatically rendered to the page; when the page form data changes, it will be automatically acquired by vue and updated to js
3.MVVM
- MVVM is the core principle of vue to realize data-driven view and two-way data binding (bottom layer)
- MVVM refers to Model (data source), View (DOM structure) and ViewModel (vue instance)
- It splits each HTML page into these three parts (Model represents the data source on which the current page is rendered, View represents the DOM structure rendered by the current page, and ViewModel represents the instance of Vue, which is the core of MVVM)
- When the data source changes, it will be monitored by the ViewModel and automatically re-render the page structure
- When the value of the form element changes, it will also be monitored by the VM and automatically synchronize the latest value after the change to the Model data source
4. Basic steps
- Import the script script file of vue.js
- Declare a DOM area in the page that will be controlled by vue
- Create a vm instance object (vue instance object)
5. Debugging tools
- More tools in the browser -> Extensions (just drag the downloaded file in) -> Vue.js devtools details (check on all networks, allow access to the file URL)
- Chrome browser installs vue-devtools online: https://chrome.google.com/webstore/detail/vuejs-devtools/nhdogjmejiglipccpnnnanhbledajbpd
- FireFox browser online installation: https://addons.mozilla.org/zh-CN/firefox/addon/vue-js-devtools/
6.{ { }}
- The interpolation expression Mustache is just a content placeholder and does not cover the content of the reason
- Can only be used in element content nodes, not attribute nodes <p>Gender: { { sex }}</p>
- Can't recognize the label
Two. Vue basics
1. Instructions
(1) Content rendering instructions
- v-text: will override the default content of the element <p v-text="username"></p>
- v-html : Render the tagged content and implement the tag style, which will override the default content of the element <div v-html="attribute"></div>
(2) Attribute binding instructions
- v-bind: (abbreviated English:): Dynamically bind the attribute value for the attribute of the element, one-way binding (data source affects DOM change)
- Support for binding simple data values <imgsrc="" v-bind:placeholder="tips">
- Support the operation of binding js expressions <div :title=" 'box-' + index">div box</div> The string needs to add ' '
(3) Event binding instructions
- v-on: (abbreviated @): Bind event listeners for DOM elements
- The processing function is written under the methods attribute of the instance object <button @click="add()">click</button> () to pass parameters
- Native DOM object onclick, oninput, onkeyup and other native events, replaced by @click, @input, @keyup
$event
The special variable provided by vue, the native event parameter object event, can solve the problem that the event parameter object event is overwritten (click the button to change color)
event modifier
Convenient trigger control of events <a href=" " @click.prevent=" "></a>
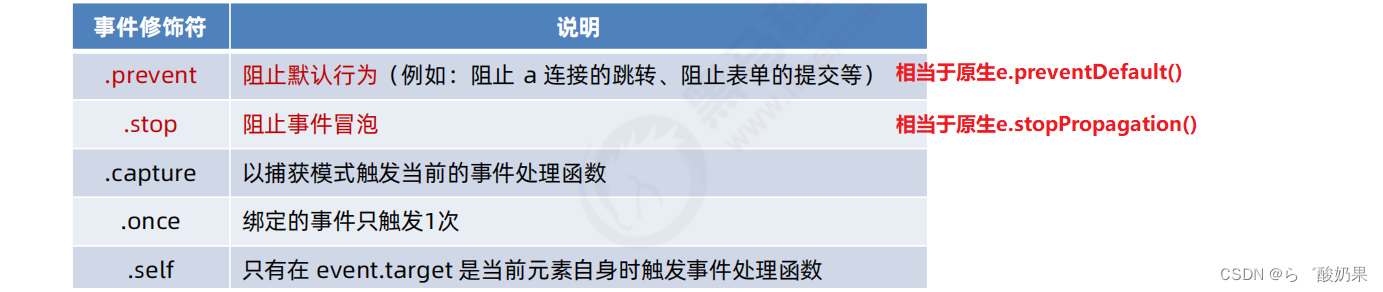
key modifier
When listening to keyboard events, judge the detailed keys, and add key modifiers to keyboard-related events
eg: <input @keyup.enter="submit"> Only when pressing Enter, call submit()
(4) Two-way binding instructions
v-model: Two-way data binding instructions, quickly get the data of the form without manipulating the DOM
(5) Conditional rendering instructions
- v-if: Dynamically create or move out to display and hide elements, which has higher switching overhead, and conditions rarely change at runtime, so it is better to use (eg: when you first enter the page, some elements do not need to be displayed by default, later no need to show)
- v-show: Dynamically add or remove style="display: none;" styles for elements to display and hide, which has higher initial rendering overhead and needs to be switched very frequently. It is better to use
-
v-else must be used together with the v-if command, otherwise it will not be recognized
-
v-else-if must be used together with the v-if command, otherwise it will not be recognized
(6) List rendering instructions
- v-for: Loop to render a list structure based on an array
- Use item in list form special syntax (item to be looped array, item to be looped for each item);
- When using index (item, index) in list, item item and index index are both formal parameters, which can be renamed
Use key to maintain list state
- The official suggestion is that the use of v-for must bind the attribute key, and the value is usually id
- When the data of the list changes, by default, vue will reuse the existing DOM elements as much as possible to improve the performance of rendering. However, this default performance optimization strategy will cause the stateful list to not be updated correctly
- In order to give vue a hint so that it can track the identity of each node, so as to improve the rendering performance under the premise of ensuring that the stateful list is updated correctly, it is necessary to provide a unique key attribute for each item
- e.g:<tr v-for="(item,index) in list" :key="item.id" :title="index + item.uname ">
Precautions for keys
- The value of key can only be a string or a number
- The value of the key must be unique (ie: the value of the key cannot be repeated)
- It is recommended to use the value of the id attribute of the data item as the value of the key (the value of the id attribute is unique)
- It does not make any sense to use the value of index as the value of key (the value of index is not unique and has no mandatory relationship with each item)
- It is recommended to specify the value of the key when using the v-for command (both to improve performance and prevent list state disorder)
2. Filter
(1) concept
- Filters are often used for formatting text, and filters can be used in interpolation expressions and v-bind attribute bindings
- The filter should be added at the end of the JS expression and invoked by the pipe character ( | ). Multiple calls can be made consecutively, and the processing results of the former are passed to the latter filter
- The essence is a js function, which can accept parameters
compatibility
It is only supported in vue 2.x and 1.x, and the filter-related functions are removed in the version of vue 3.x (the official recommendation is to use computed properties or methods instead of removed filter functions)
(2) classification
- The filters defined under the filters node can only be used in the el area controlled by the current vm instance
global filter
- Share filters between multiple vue instances
- Vue.filter('filter name', (reference 1, reference 2, reference 3)=>{ }) must be defined before the vue instance
- Parameter 1 is always the pipe symbol | the value to be processed before, and the second parameter starts, which is the parameter passed by the call filter
3. Listener
- The watch listener monitors data changes, so as to perform specific operations on data changes, written under the watch node
- The listener is essentially a function, which data is monitored, it is used as the function name, the new value in the parameter is first, and the old value is after
- immediate option: to achieve the first page rendering, the listener is called immediately immediate: true, default false
- deep option: deep listening, can monitor the changes of properties in the object deep: true
- Listen to a single property in the object: object. property: { handler (parameter) { } } handler is the listener processing function
4. Computed properties
- Calculated attributes refer to a series of operations, and finally get an attribute value, which is written under the computed node
- Defined as a method format, use it as an attribute when using it, and realize code reuse
- The result of the calculation will be cached. Only when the data source changes, the calculation property will be re-evaluated
5.vue-cli
(1) Single Page Application
(2) view-cli
- A standard tool for Vue.js development, which simplifies the process for programmers to create engineered Vue projects based on webpack
- https://cli.vuejs.org/zh/
- Install: npm i -g @vue/cli
(3) use
- Quickly generate engineered Vue projects based on vue-cli: the name of the vue create project (excluding capital letters, Chinese characters, spaces, etc.)
- Select a configuration item
- Automatically create projects, initialize git, and install required dependencies
- After the installation configuration is complete, it will display npm run serve to run the project in a development mode (serve is equivalent to dev, in the configuration file scripts node, release build)
- Project Directory Description
6. Components
(1) Component development
- According to the idea of encapsulation, the reusable UI structure on the page is encapsulated into components, so as to facilitate the development and maintenance of the project
- Vue is a front-end framework that supports component-based development. The component suffix in Vue is .vue
(2) Vue component components
template
- The template structure of the component (required)
- It only plays the role of wrapping, and will not be rendered as a real DOM element
- Can only contain unique root nodes
script
- JavaScript behavior of the component (optional)
- Just write the script, which must contain export default {can contain data, methods... } default export
- The data source data in the component must be a function, and the return object can define data; it cannot directly point to a data object (it will cause the problem that multiple component instances share the same data) eg:data(){ return { username: 'ff ' } }
style
- The style of the component (optional)
- Enable less preprocessor <style lang="less">
(3) Use of components
relation
- After the components are encapsulated, they are independent of each other, and there is no parent-child relationship
- When using components, according to the nesting relationship with each other, a parent-child relationship and a brother relationship are formed
Steps for usage
- Use the components node to register components, components:{ Left , // 'Left': Shorthand for Left }
- Use the import syntax to import the required components, import Left from '@/components/left.vue'
- Use the component just registered in the form of a label, <Left></Left> in the template
private subcomponent
- Private subcomponents are registered through components
- Under the components node of component A, register component F, then component F can only be used in component A, and cannot be used in component C
global component
- In the main.js entry file, global components can be registered through the Vue.component() method
- import Count from '@/components/Count.vue'; Vue.component('MyCount',Count)
(4)props
- Custom properties, encapsulation of common components, can improve the reusability of components
- prop:['custom A', 'custom B'...], props:{ property A:{ //default value... }}
- Custom properties are read-only, and you cannot directly modify the value of props, otherwise an error will be reported directly. If you modify the value of props, you can transfer the value of props to data (the data in data is both readable and writable)
- default When declaring a custom attribute, define the default value of the attribute (if not passed, use the default value)
- type When declaring a custom attribute, define the value type of the attribute (the passed value type does not match, and an error is reported)
- required Set the attribute as a required item, forcing the user to pass the value of the attribute (check whether the attribute exists, regardless of the default value)
(5) Component style conflicts
- By default, styles written in .vue components will take effect globally, so it is easy to cause style conflicts between multiple components
The root cause is:
- In a single-page application, the DOM structure of all components is presented based on the unique index.html page
- The styles in each component will affect the DOM in the entire index.html page
solve:
- Assign unique custom attributes to each component, and control the scope of styles through attribute selectors
- The scoped attribute <style scoped></style> can prevent style conflicts between components, but the style of the current component is not effective for its subcomponents
- /deep/ deep selector allows certain styles to take effect on child components
7. Component life cycle
(1) concept
- Life Cycle (Life Cycle) refers to the entire stage of a component from creation -> operation -> destruction, emphasizing a time period
- Life cycle function: built-in function, which will be automatically executed in order along with the life cycle of the component, emphasizing the time point
(2) classification
- Component creation phase new Vue()->beforeCreate->created->beforeMount->mounted
- Component runtime beforeUpdate->updated
- Component destruction stage beforeDestroy->destroyed
8. Data sharing
(1) Parent to child component data sharing
Use custom attributes
(2) Child to parent component data sharing
Use custom events
(3) Brother component data sharing
In vue2.x, the data sharing scheme between sibling components is EventBus (eventBus.js)
9. ref reference
- ref is used to assist developers to obtain references to DOM elements or components without relying on jQuery
- $refs object: Each vue component instance contains one, which stores a reference to the corresponding DOM element or component. By default, $refs points to an empty object
- Use ref to create instances for subcomponents, and directly manipulate methods and properties in subcomponents <Myref ref="comRef"></Myref>
this.$nextTick(callback) method
- Will defer the cb callback until after the next DOM update cycle
- After the DOM update of the component is completed, execute the cb callback function, so as to ensure that the cb callback function can operate the latest DOM element
10. Dynamic components
(1) concept
- Dynamic components refer to the display and hiding of dynamic switching components
- Through the built-in component <component>, the rendering of dynamic components is specially realized <component is="Left"></component>
- The is attribute can be bound to achieve dynamic switching <component :is="comName"></component>
(2)keep-alive
- <keep-alive> The component keeps the state. By default, the state of the component cannot be kept when switching dynamic components (the component will be destroyed
The life cycle function corresponding to keep-alive
- When the component is cached, the component's deactivated lifecycle function is automatically triggered
- When the component is activated, the activated lifecycle function of the component is automatically triggered
Attributes
- include: Specify the components that can be cached, and the rest of the components will be destroyed after switching
- exclude: Specifies components that are not cached
- cannot be used at the same time
(3)name
- The name attribute is used to specify the name of the component when declaring the component, combined with the keep-alive tag to implement the component cache function, and displayed in the debugging tool
- The name when registering the component, which is used to render and display the registered component on the page in the form of a label components:{ Left, }
11. Slot
(1) concept
- Slot (Slot) is the ability provided by vue for the packager of the component, which allows the developer to define the uncertain part that is expected to be specified by the user as a slot (a placeholder for the content reserved for the user) when packaging the component )
- The content that needs to be filled is not used to wrap <template>, and it is filled into the default slot by default
- If no <slot> slots are reserved when wrapping the component, any custom content provided by the user will be discarded
- Backup content: the content written in the slot, the user-defined content will be filled and displayed first, if there is no content filled in the slot, the backup content will be displayed
(2) Named slots
- <slot name="xxx"></slot> A slot with a specific name is called a "named slot"
- The slot with no specified name name, the default slot name is default
v-slot: (#) instruction:
- The v-slot (#) directive specifies which slot to fill
- It cannot be used directly on the element, it must be used on the <template> virtual tag
(3) Scope slot
- <slot> Slot binding props data (custom attributes and values, or dynamic data)
- <template #content="scope"> The scope parameter can be used to receive objects
- The received object is originally an object, and it can also be directly deconstructed to get the attributes { msg, user }
12. Custom commands
(1) Private custom instructions
- Statements under the directives node
- When using it, just add the v- prefix, and in the template structure, = can be used to dynamically bind parameter values for the current command
// 私有自定义指令节点
directives:{
/* color:{ // 定义名为color的自定义指令, 指向一个配置对象
// 当指令首次被绑定到元素上时,会立即触发bind函数, 若DOM更新时,不会触发
// 形参el为当前被绑定的DOM对象 binding接收data传的值
bind(el, binding){
el.style.color = binding.value;
},
update(el, binding){ // DOM更新时触发
el.style.color = binding.value;
},
} */
// 简写
color(el, binding){
el.style.color = binding.value;
},
},
(2) Global custom instructions
// 全局自定义指令 定义在main.js中 通常使用全局自定义指令, 私有的意义不大
Vue.directive('col',(el, binding)=>{
el.style.color = binding.value;
})
3. Routing
1 Introduction
router, is the corresponding relationship
(1) Front-end routing
- Front-end routing : the correspondence between Hash addresses and components
- Hash address: Anchor link, the content after # and # in the url address is the hash address
- SPA and front-end routing: In SPA (Single Page Program) projects, switching between different functions depends on front-end routing
Way of working:
- The user clicks the routing link on the page, causing the Hash value in the URL address bar to change
- The front-end routing listens to the change of the Hash address, and renders the component corresponding to the current Hash address to the browser
Implement simple front-end routing:
- You can bind dynamic properties to render components through the <component> tag
- Add the hash value of the object through <a>
- Use window.onhashchange = () => { } in created() to monitor the change of hash address, and dynamically switch the components to be displayed
(2) Backend routing
- Correspondence between request method, request address and function processing function
- In node.js, express routing is the backend routing
2. Basic usage of vue-router
- The official routing solution can only be used in conjunction with the Vue project, which can easily manage the switching of components in the SPA project
- https://router.vuejs.org
basic usage
- Install the vue-router package: npm i [email protected] -S (vue2 will report an error if the router version is too high)
- Create a routing module and initialize src->router->index.js
- Import and mount the routing module
- The component declares the routing link <router-link> (replacing the normal <a> link) and the placeholder <router-view>
3. Common usage of vue-router
(1) Route redirection
- When accessing address A, force jump to address C
- The redirect attribute specifies a new routing address eg: the home page can be displayed under the / path
(2) Nested routing
- Nested display of components through routing
- Declare child route placeholders and child route links (/parent-path/child-path)
- The children attribute declares child routing rules
(3) Dynamic route matching
- Define the variable part of the Hash address as a parameter item (: parameter), thereby improving the reusability of routing rules
- $route.params parameter object can access the parameter value of dynamic matching
- props pass parameter, simplified form of receiving route parameter acquisition
(4) Declarative Navigation & Programmatic Navigation
- Declarative navigation: In the browser, click on the link to realize the navigation eg: ordinary <a> link, vue<router-link>
- Programmatic navigation: In the browser, the method of calling the API method to realize the navigation eg: ordinary call location.href
(5) vue-router programmatic navigation API
- this.$router.push('hash address') Jump to the specified hash address and add a history record
- this.$router.replace('hash address') Jump to the specified hash address and replace the current history
- this.$router.go(Number n) Realize navigation history forward (positive value) and backward (negative value)
-
$router.back() In the history, go back to the previous page
-
$router.forward() forwards to the next page in the history
(6) Navigation Guard
Control access to routes
// src->router-> index.js
// 1.装[email protected]包
// 2.导入Vue, 路由模块, 以及所需要的组件
import Vue from 'vue'
import VueRouter from 'vue-router'
import Home from '@/components/Router/Home.vue'
// 3.将VueRouter装为项目插件
Vue.use(VueRouter)
// 4.创建实例对象
const router = new VueRouter({
// 7. 定义哈希地址与组件的对应关系 { path: '哈希地址#之后的内容', component: 组件名 }
routes:[
// 重定向的路由规则 在/路径下显示主页
{ path: '/', redirect: '/home' },
// 路由规则
{ path: '/home', component: Home },
// 父级路由规则, Mine页面
{
path: '/mine', //父级地址
component: Mine, //父级组件名
// redirect: '/mine/mineabout1', //可使用路由重定向, 当访问mine时,直接展示MineAbout1上, 也可使用默认子路由
children: [ //通过children属性, 嵌套声明子级路由规则 子路由不使用/
// 默认子路由: children数组中, 某个路由规则的path为空字符串, 打开时,直接展示该子组件上
{ path: 'mineabout1', component: MineAbout1 },
{ path: '', component: MineAbout2 }
]
},
// 动态参数 在World组件中,根据参数值, 展示对应组件 变化部分写 :参数
// 方式1 this.$route.params.mid 拿到mid
// { path: '/world/:mid', component: World },
// 方式2 设置 props: true ,在组件中用props接收
{ path: '/world/:mid', component: World , props: true },
{ path: '/main', component: Main },
{ path: '/login', component: Login },
]
})
// 8.为router实例对象, 声明全局前置守卫 发生路由跳转,必然触发beforeEach指定的回调函数 to将要访问的路由信息对象, from将要离开的路由信息对象, next()函数 , 表放行
router.beforeEach( (to, from , next) => {
if(to.path === '/main'){ //判断用户将要访问的哈希地址是否为/main
//等于,则需要登录才能访问 获取localStorage中的token
const token = localStorage.getItem('token') //如果在浏览器Application->localStorage中有token,就可访问到main
if(token){ //有token,则放行
next()
}else{ // 无token, 则强制跳转到/login
next('/login')
}
}else{ //访问的不是后台主页,直接放行
next()
}
})
// 5.向外共享
export default router
// 6. main.js中挂载
// 导入路由模块 拿到实例对象
import router from '@/router/index.js'
new Vue({
render: h => h(App2),
// Vue中使用路由, 必须将路由实例对象router进行挂载
router, //router : router 属性名属性值一样,可简写为router
}).$mount('#app')
//App.vue组件中 项目中安装和配置了vue-router,就可以使用 <router-view>组件来占位 和 <router-link>替代普通a链接 自动加# -->
<router-link to="/home">主页</router-link>
<router-link to="/mine">我的</router-link> <hr>
//<!-- 动态参数 哈希地址中. /后的参数项,叫路径参数; 在路由参数对象中,用this.$route.params访问路径参数; ?后的参数项,叫查询参数,用this.$route.query访问; this.$route中, path只是路径部分 e.g:/world/2, fullPath是完整路径 e.g: /world/2?name=mm&age=18 -->
<router-link to="/world/1">W1.vue</router-link>
<router-link to="/world/2?name=mm&age=18">W2.vue</router-link> <hr>
<router-view ></router-view>
//<!-- 行内使用编程式导航跳转时,省略this,否则报错 -->
<button @click="$router.back()">back</button>
<button @click="$router.forward()">forward前进</button>