Rust divides strings into different types according to different application scenarios. Today we only introduce the two most common types of str and String
2.1 str
str is a native type in Rust and is stored in a static storage area. Because it is a dynamic type, the size cannot be determined at compile time, so we often use its reference form &str. str is always valid UTF-8 encoded
It can be directly created by literals, converted from other types, or converted to other types
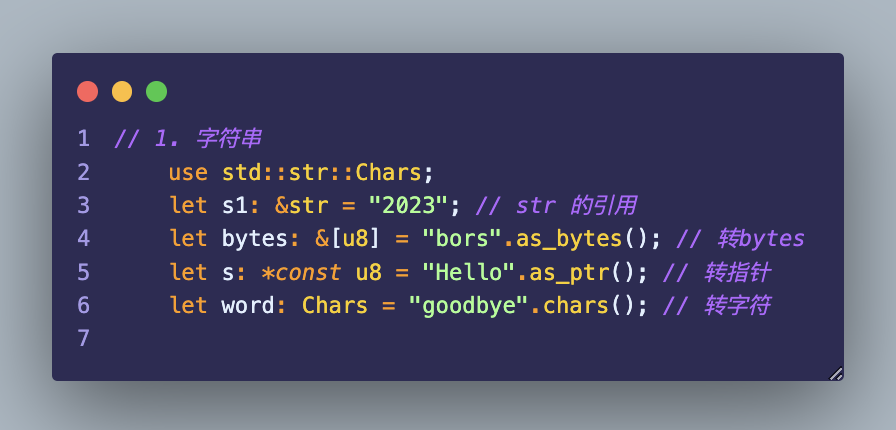
In fact, as a native type of rust, str implements many methods. Supports operations such as converting, splitting, parsing, and searching strings. Specific readers can refer to the standard library
https://doc.rust-lang.org/std/primitive.str.html#
In addition, str implements common traits, such as AsMut, Debug, From, etc., and also implements Send and Sync, which can be used worry-free in concurrent programming.
Special Note: Reference types are first-class citizens in Rust and are Copy
2.2 String
String is a fat pointer (and a smart pointer: it can be automatically dereferenced and its destructor called). It contains three words: ptr, cap and len respectively. Data is stored on the heap with a dynamic size. is essentially a structure
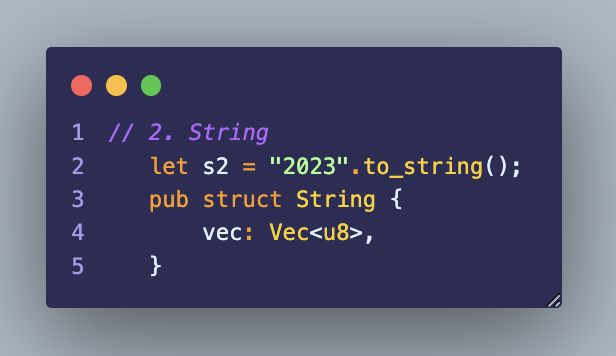
Note: String does not implement the Copy trait
A common scenario for String is to process some file content. Let's look at a more comprehensive example. This example reads the numbers in a local file and performs a summation operation.
Local file name: sum_text
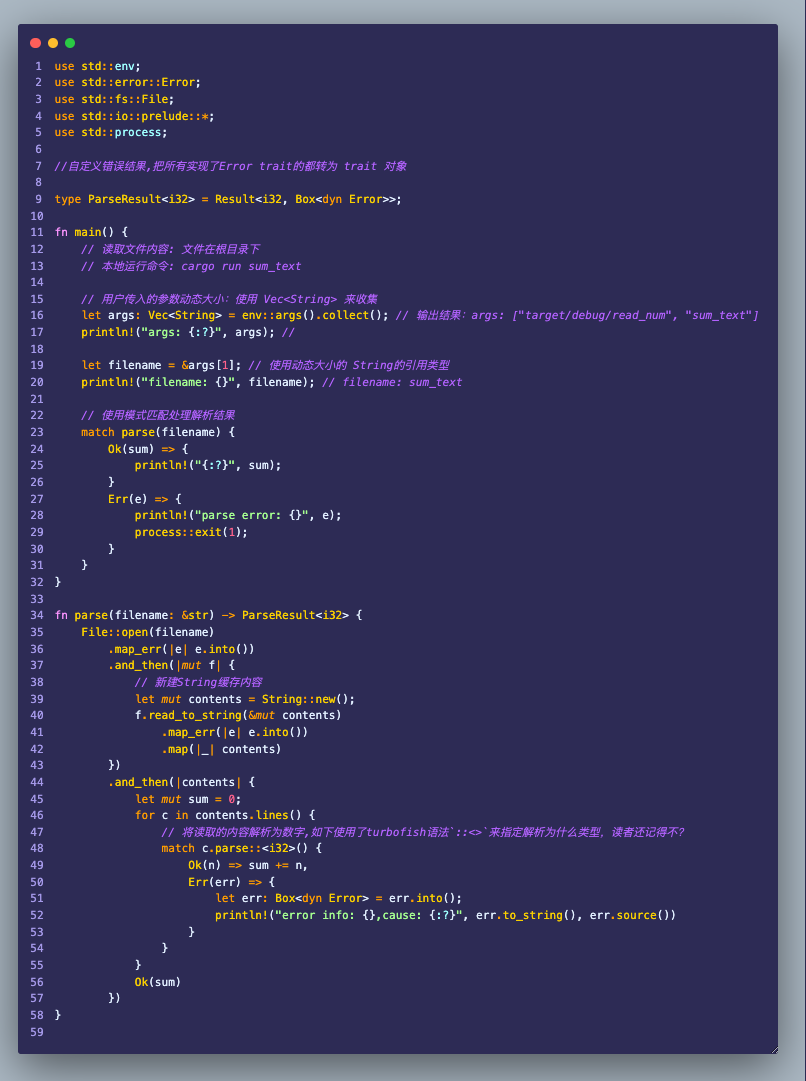
Through the above example, we can see that in some scenarios where data is dynamic, we often use String. In addition, there are many knowledge points involved in String, such as ownership mechanism, smart pointer, various traits, memory, function call stack, etc. We will explain these knowledge points one by one later
Sample code Github address:
https://github.com/shiyivei/from-principle-to-practice/blob/main/src/parse-file/src/refactor_one.rs
更多内容,欢迎关注公众号拾一维