Array Tutorial of C Language Advanced Tutorial
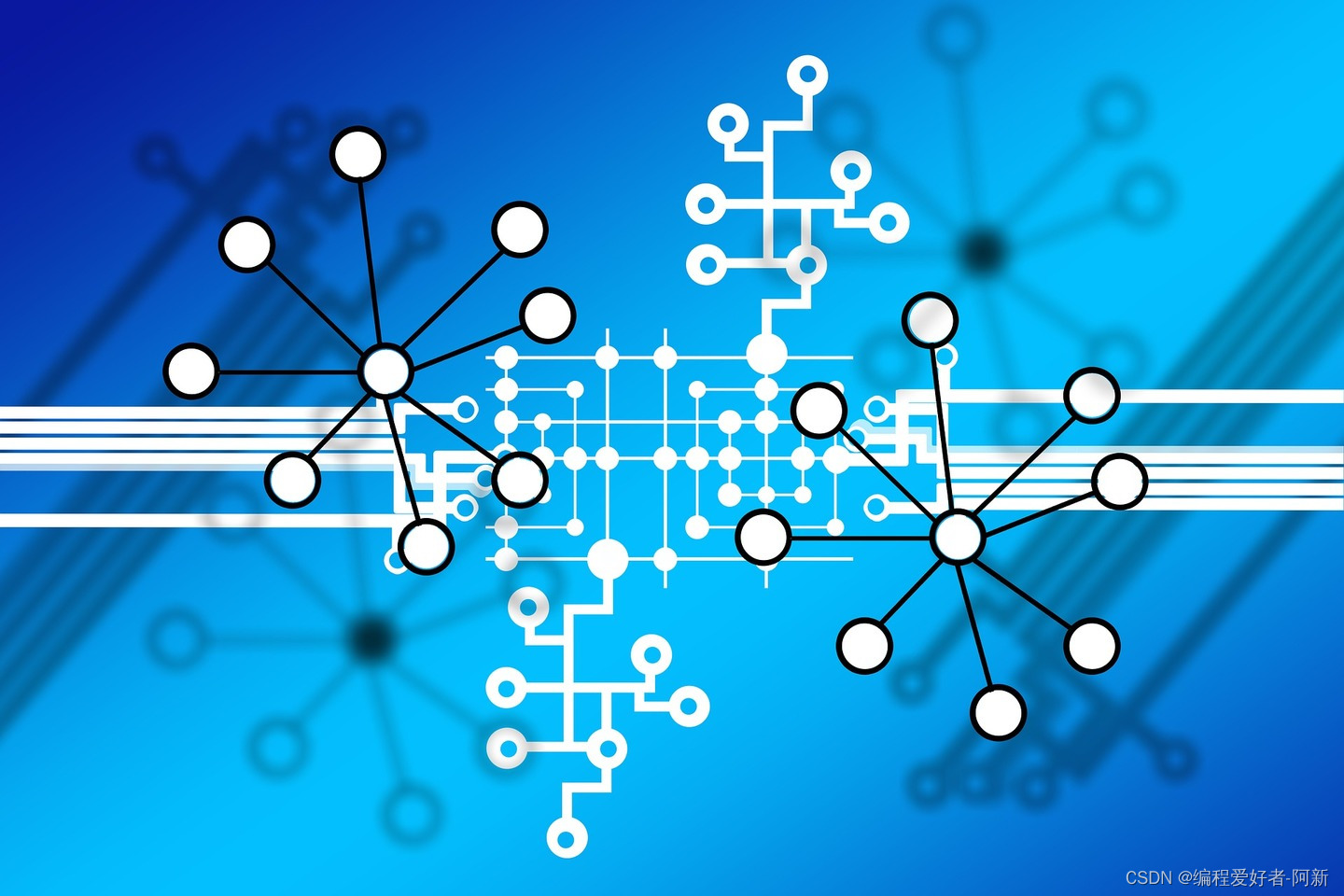
The compilation environment of this article
The compilation environment of this article uses an integrated development environment: Visual Studio 2019
The link to the official website of Visual Studio 2019 is as follows
Visual Studio 2019 official website link
The features of the Visual Studio 2019 integrated development environment are
- Visual Studio 2019 installs the Live Share code collaboration service by default.
- New welcome window to help users write code quickly, improved search functionality, general performance improvements.
- Visual Studio IntelliCode AI Help.
- Better Python virtualization and Conda support.
- And support for .NET Core 3.0 projects including WinForms and WPF, etc.
1. Previous article: Links to C language arrays (1, 2)
1.1, C language array - article link
In the tutorial of C language array (1), the following points are briefly introduced
- Introduces how to use arrays in C language programs.
- Finally, when writing a program to use an array, how to refer to a set of values by a name.
- Mastered the definition and usage of C language one-dimensional array through program examples.
The article link of C language array (1) is as follows
Article: Arrays in C Language (1)
1.2, C language array 2 article links
In the tutorial of C language array (2), the following points are briefly introduced
- Introduces the addressing method of the array of C language high-level programming.
- Through several example programs to master the application of C language array addressing.
The article link of C language array (2) is as follows
Article: Arrays in C Language (2)
Second, the initialization of the array
2.1. Several methods of array initialization
- It is possible to assign initial values to the elements of the array, probably just to be on the safe side. Predetermining the initial value of the array elements makes it easier to find errors.
- To initialize the elements of an array, simply specify a list of initial values within curly braces in the declaration statement, separating them with commas.
For example:
double values[5] = {
1.5, 2.5,3.5, 4.5, 5.5 };
- This statement declares an array value containing 5 elements.
- The initial value of values[0] is 1.5, the initial value of value[1] is 2.5, and so on.
- To initialize the entire array, each element should have a value.
- If the number of initial values is less than the number of elements, elements without initial values are set to 0.
So if you write
double values1[5] = {
1.5, 2.5, 3.5 };
- The first 3 elements are initialized with the values inside the brackets, and the last two elements are initialized to 0.
- If no initial value is provided for the elements, the compiler will provide them with an initial value of 0, so the initial value provides a simple way to initialize the entire array to 0.
Just provide 0 for one element:
double values2[5] = {
0.0 };
- The entire array is initialized to 0.0.
- If the number of initial values exceeds the number of array elements, the compiler will report an error.
When specifying an initial value for a column, it is not necessary to provide the size of the array, the compiler can infer the number of elements from the column value.
int primes[] = {
2, 3,5, 7,11, 13, 17, 19,23, 291 };
- The size of the array in the above statement is determined by the number of initial values in the list, so the primes array has 10 elements.
3. Arrays and addresses
3.1, the relationship between array and address
The following declares an array with 4 elements:
long number[4];
- The array name number specifies the address of the memory area where the data item is stored, and each element can be found by combining the address and the index value, because the index value represents the offset of each element from the beginning of the array.
- When declaring an array, you provide the compiler with all the information needed to allocate memory for the array, including the type of the value
and the dimensions of the array, and the type of the value determines the number of bytes required for each element. - The array dimension specifies the number of elements.
- The number of bytes occupied by an array is the number of elements multiplied by the number of bytes per element.
- The address of an array element is the address at which the array begins, plus the element's index value multiplied by the number of bytes required for each element index type in the array.
The following is the case where the array variable is stored in memory.
How arrays are organized in memory
- The way to get the address of an array element is similar to a normal variable. For value integer variable
- Its address can be output with the following statement:
printf("数组value的地址是%p\n", &values);
- To output the address of the third element of the number array, you can write the following code:
printf("\n数组number的第三个元素的地址是%p%p\n\n", &number[2]);
3.2. Output the address value of the array
- The following code snippet sets the values of the elements in the array and then outputs the address and content of each element.
int data[5];
for (unsigned int i=0;i<5; ++i)
data[i] = 12*(i + 1);
printf ("data[&d] Address: 易p Contents: d\n", i, &data[i], data[i]) ;
- The for loop variable i traverses all legal index values in the data array.
- In this loop, the value of the element at index position i is set to 12*(i+1).
- The output statement shows the current element and its index value, the address of the array element determined by the current value of i, and the value stored in the element.
The output of the above program debugging results is as follows
数组value的地址是00CFF814
数组number的第三个元素的地址是00CFF77400491023
数组data[0] 的地址是: 00CFF750, 数组data[0]的数据是: 12
数组data[1] 的地址是: 00CFF754, 数组data[1]的数据是: 24
数组data[2] 的地址是: 00CFF758, 数组data[2]的数据是: 36
数组data[3] 的地址是: 00CFF75C, 数组data[3]的数据是: 48
数组data[4] 的地址是: 00CFF760, 数组data[4]的数据是: 60
请按任意键继续. . .
- The value of i is shown in parentheses after the array name.
- The address of each element is 4 greater than the previous element, so each element occupies 4 bytes.
4. Determine the size of the array
4.1. The sizeof operator determines the size of the array
- The sizeof operator can calculate the number of bytes occupied by a variable of a specified type.
- Applies the sizeof operator to a type name.
as follows:
// 输出字节数
printf("long类型变量的大小为%zu 字节。\n", sizeof(long));
-
The parentheses around the type name after the sizeof operator are required.
-
If you leave it out, the code won't compile.
-
You can also apply the sizeof operator to a variable, which will calculate the number of bytes the variable occupies.
-
The sizeof operator yields a value of type size_t, which is implementation-dependent and typically an unsigned integer type.
-
If the output is given using
%u
- Specifier, the compiler defines size_t as unsigned long or unsigned long long, the compiler may issue a warning: the use of the %u specifier does not match the value output by the print() function.
- Using %u will suppress the warning message.
4.2, the sizeof operator outputs the number of bytes occupied by the array
- The sizeof operator can also be used with arrays. The following statement declares an array:
double values[5] = ( 1.5, 2.5,3.5,4.5, 5.5 };
The following statement can be used to output the number of bytes occupied by this array:
// 输出数组字节数
printf("\n数组values的大小为%zu 字节。\n", sizeof(values));
The debugging result output is as follows
数组value的地址是00B3F7B0
数组number的第三个元素的地址是00B3F71000631023
数组data[0] 的地址是: 00B3F6EC, 数组data[0]的数据是: 12
数组data[1] 的地址是: 00B3F6F0, 数组data[1]的数据是: 24
数组data[2] 的地址是: 00B3F6F4, 数组data[2]的数据是: 36
数组data[3] 的地址是: 00B3F6F8, 数组data[3]的数据是: 48
数组data[4] 的地址是: 00B3F6FC, 数组data[4]的数据是: 60
long类型变量的大小为4 字节。
数组values的大小为40 字节。
请按任意键继续. . .
- You can also use the expression
sizeof values[0]
- Calculates the number of bytes occupied by an element in the array.
- The value of this expression is 8. Of course, using the element's legal index values yields the same result.
- The memory occupied by an array is the number of bytes of a single element multiplied by the number of elements.
- So you can use the sizeof operator to count the number of elements in an array:
size_t element_count = sizeof values / sizeof values[0];
- After executing this statement, the variable element_count contains the number of elements in the array values.
- element.count is declared as type size t because that is the type generated by the sizeof operator.
- The sizeof operator can be applied to data types, so the previous statement can be rewritten to count the number of array elements.
As follows
size_t elementCount = sizeof values / sizeof(double);
- This will give the same result as before, because the type of the array is double, sizeof(double) will get the number of bytes occupied by the element.
- Sometimes the wrong type is used occasionally, so it's better to use the previous statement.
- Parentheses are not required when the sizeof operator is applied to variables, but they are generally used anyway.
4.3. The sizeof operator is applied to the for loop
The previous example could be written as follows
double values[5] = {
1.5, 2.5,3.5, 4.5, 5.5 };
size_t element_count = sizeof values / sizeof values[0];
printf("\n数组values的大小为%zu 字节。\n", sizeof(values));
printf("数组values有 %u 元素, 数组每个元素的字节数是%zu\n", element_count, sizeof(values[0]));
The output of these statements is as follows
数组value的地址是00EFFC1C
数组number的第三个元素的地址是00EFFB7C00491023
数组data[0] 的地址是: 00EFFB58, 数组data[0]的数据是: 12
数组data[1] 的地址是: 00EFFB5C, 数组data[1]的数据是: 24
数组data[2] 的地址是: 00EFFB60, 数组data[2]的数据是: 36
数组data[3] 的地址是: 00EFFB64, 数组data[3]的数据是: 48
数组data[4] 的地址是: 00EFFB68, 数组data[4]的数据是: 60
long类型变量的大小为4 字节。
数组values的大小为40 字节。
数组values的大小为40 字节。
数组values有 5 元素, 数组每个元素的字节数是8
请按任意键继续. . .
The sizeof operator can be used when using a loop to process all elements in an array.
For example:
doub1e values[5] = {
1.5, 2.5, 3.5, 4.5, 5.5 };
double sum = 0.0;
for (int i = 0; i < sizeof(values) / sizeof(values[0]); i++)
{
sum += values[i];
}
printf("\n数组values中的这些值的和为 %.2f\n\n", sum);
The debug output for these statements is as follows
数组value的地址是00D3FE0C
数组number的第三个元素的地址是00D3FD6C00211023
数组data[0] 的地址是: 00D3FD48, 数组data[0]的数据是: 12
数组data[1] 的地址是: 00D3FD4C, 数组data[1]的数据是: 24
数组data[2] 的地址是: 00D3FD50, 数组data[2]的数据是: 36
数组data[3] 的地址是: 00D3FD54, 数组data[3]的数据是: 48
数组data[4] 的地址是: 00D3FD58, 数组data[4]的数据是: 60
long类型变量的大小为4 字节。
数组values的大小为40 字节。
数组values的大小为40 字节。
数组values有 5 元素, 数组每个元素的字节数是8
数组values中的这些值的和为 17.50
请按任意键继续. . .
- This loop adds up the values of all elements in the array.
- Using the sizeof operator to count the number of elements in an array ensures that the upper bound on the loop variable i is always correct regardless of the size of the array.
Five, the complete program
The complete program for this article is as follows
5.1 Main.h file program
#pragma once
#include <stdio.h>
#include <stdlib.h>
5.2 Main.c file program
#define _CRT_SECURE_NO_WARNINGS
#include "Main.h"
int main()
{
system("color 3E");
// 定义数组
double values[5] = {
1.5, 2.5,3.5, 4.5, 5.5 };
double values1[5] = {
1.5, 2.5, 3.5 };
double values2[5] = {
0.0 };
int primes[] = {
2, 3,5, 7,11, 13, 17, 19,23, 291 };
long number[4];
int data[5];
double sum = 0;
// 数组数组的地址
printf("数组value的地址是%p\n", &values);
printf("\n数组number的第三个元素的地址是%p%p\n\n", &number[2]);
for (unsigned int i = 0; i < 5; ++i)
{
data[i] = 12 * (i + 1);
printf("数组data[%d] 的地址是: %p, 数组data[%d]的数据是: %d\n", i, &data[i], i, data[i]);
}
printf("\n");
// 输出字节数
printf("long类型变量的大小为%zu 字节。\n", sizeof(long));
// 输出数组字节数
printf("\n数组values的大小为%zu 字节。\n", sizeof(values));
size_t element_count = sizeof values / sizeof values[0];
size_t elementCount = sizeof values / sizeof(double);
/*double values[5] = { 1.5, 2.5,3.5, 4.5, 5.5 };
size_t element_count = sizeof values / sizeof values[0];*/
printf("\n数组values的大小为%zu 字节。\n", sizeof(values));
printf("数组values有 %u 元素, 数组每个元素的字节数是%zu\n", element_count, sizeof(values[0]));
for (int i = 0; i < sizeof(values) / sizeof(values[0]); i++)
{
sum += values[i];
}
printf("\n数组values中的这些值的和为 %.2f\n\n", sum);
system("pause");
return 0;
}
6. Summary
This article mainly introduces several methods of array initialization in C language advanced programming.
Describes the relationship between arrays and addresses.
Describes how to determine the size of an array.
Describes how sizeof determines the size of an array.
Master the application of C language arrays and addresses through example programs.
This article ends here.
I hope that the initialization of C language arrays, arrays and addresses, and the determination of the size of arrays in this article are tutorials.
can help you.