C# Object-Oriented Programming Course Experiment 4: Experiment Name: Basics of C# Object-Oriented Programming
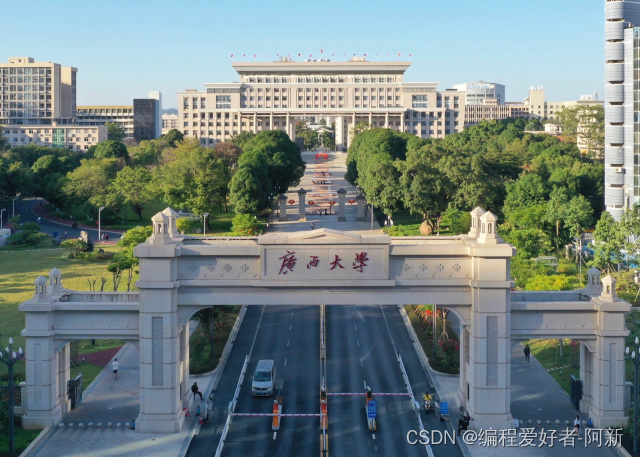
Experiment content: C# object-oriented programming foundation
1. Purpose of the experiment
- (1) Master the concept and basic composition of C# classes, learn to design classes, and master how to use classes;
- (2) Familiar with the use of fields and methods;
- (3) Master four access modifiers;
- (4) Familiar with the definition and use of attributes;
- (5) Add comments to the program to make it clear and readable;
2. Experimental environment
Microsoft Visual Studio 2008
3. Experimental content and steps
3.1.1. Experiment content
Write a console application that simulates a simple calculator. Define a class named Cal that contains two private fields n1 and n2. Write a constructor to initialize the two fields. Then define four public member methods of Addition, Subtraction, Multiplication, and Division for this class, and perform addition, subtraction, multiplication, and division operations on two of the member variables. Create an object of the Cal class in the Main( ) method, call each method, and display the calculation result.
3.1.2. Experimental steps
1. The program design of the experiment is as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace 实验4_1_
{
class Cal
{
private double digit1; //定义两个数字字段
private double digit2;
public Cal(double Digit1, double Digit2) //定义构造方法
{
digit1 = Digit1;
digit2 = Digit2;
}
public double Add() //定义加减乘除的方法
{
return digit1 + digit2;
}
public double Sub()
{
return digit1 - digit2;
}
public double Mul()
{
return digit1 * digit2;
}
public double Div()
{
return digit1 / digit2;
}
}
class Program
{
static void Main(string[] args)
{
double digit1 = 10;
double digit2 = 2;
Cal digit = new Cal(digit1, digit2); //成绩Cal对象digit
Console.WriteLine("两数分别为:{0},{1}", digit1, digit2);
Console.WriteLine();
Console.WriteLine("两数相加得:");
Console.WriteLine("{0}", digit.Add()); //(digit.Add())通过类的对象来访问类的方法
Console.WriteLine("两数相减得:");
Console.WriteLine("{0}", digit.Sub());
Console.WriteLine("两数相乘得:");
Console.WriteLine("{0}", digit.Mul());
Console.WriteLine("两数相除得:");
Console.WriteLine("{0}", digit.Div());
Console.ReadLine();
}
}
}
2. The running effect of the experiment is as follows:
3.2.1. Experimental content
Please define a Vehicle class, which contains properties such as Speed (read-only), volume (Size () readable and writable), etc.; methods include Move(), set speed SetSpeed(int speed), and accelerate SpeedUp (), deceleration SpeedDown () and so on. Finally, instantiate a vehicle object in the Main( ) method, initialize the value of Size, initialize Speed for it through the method, and print it out through the output method. In addition, call the methods of acceleration and deceleration to change the speed.
3.2.2. Experimental steps
The experimental program is designed as follows
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace 实验4_2_
{
class Vehicle
{
private int CarSpeed; //定义字段
private int Volume;
public Vehicle(int a) //构造方法
{
Volume = a;
}
public int Speed //速度属性
{
get
{
return CarSpeed;
}
}
public int Size //体积属性
{
get
{
return Volume;
}
set
{
Volume = value;
}
}
public void Move() //定义Move方法
{
}
public void SetSpeed(int a) //定义SetSpeed方法
{
CarSpeed = a;
}
public void SpeedUp(int a) //定义SpeedUp方法
{
CarSpeed += a;
}
public void SpeedDown(int a) //定义SpeedDown方法
{
CarSpeed -= a;
}
}
class Program
{
static void Main(string[] args)
{
int Volume = 5; //设置体积
int CarSpeed = 10; //设置初始速度
int Change = 1; //速度的改变量
Vehicle Car = new Vehicle(Volume); //创建Vehicle类的对象
Car.SetSpeed(CarSpeed);
Console.WriteLine("size={0},speed={1}", Car.Size ,Car.Speed);
Car.SpeedUp(Change); //对速度进行加速
Console.WriteLine("size={0},speed={1}", Car.Size, Car.Speed);
Car.SpeedDown(Change); //对速度进行减速
Console.WriteLine("size={0},speed={1}", Car.Size, Car.Speed);
Console.ReadLine();
}
}
}
The running effect of the experiment is as follows:
4. Experimental summary
- 1. Through experiments, master the concept and basic composition of C# classes, learn to design classes, and master how to use classes.
- 2. This experiment has deepened my grasp of attributes
- 3. Master the construction method of the class and the application of the object calling method