Take a screenshot first:
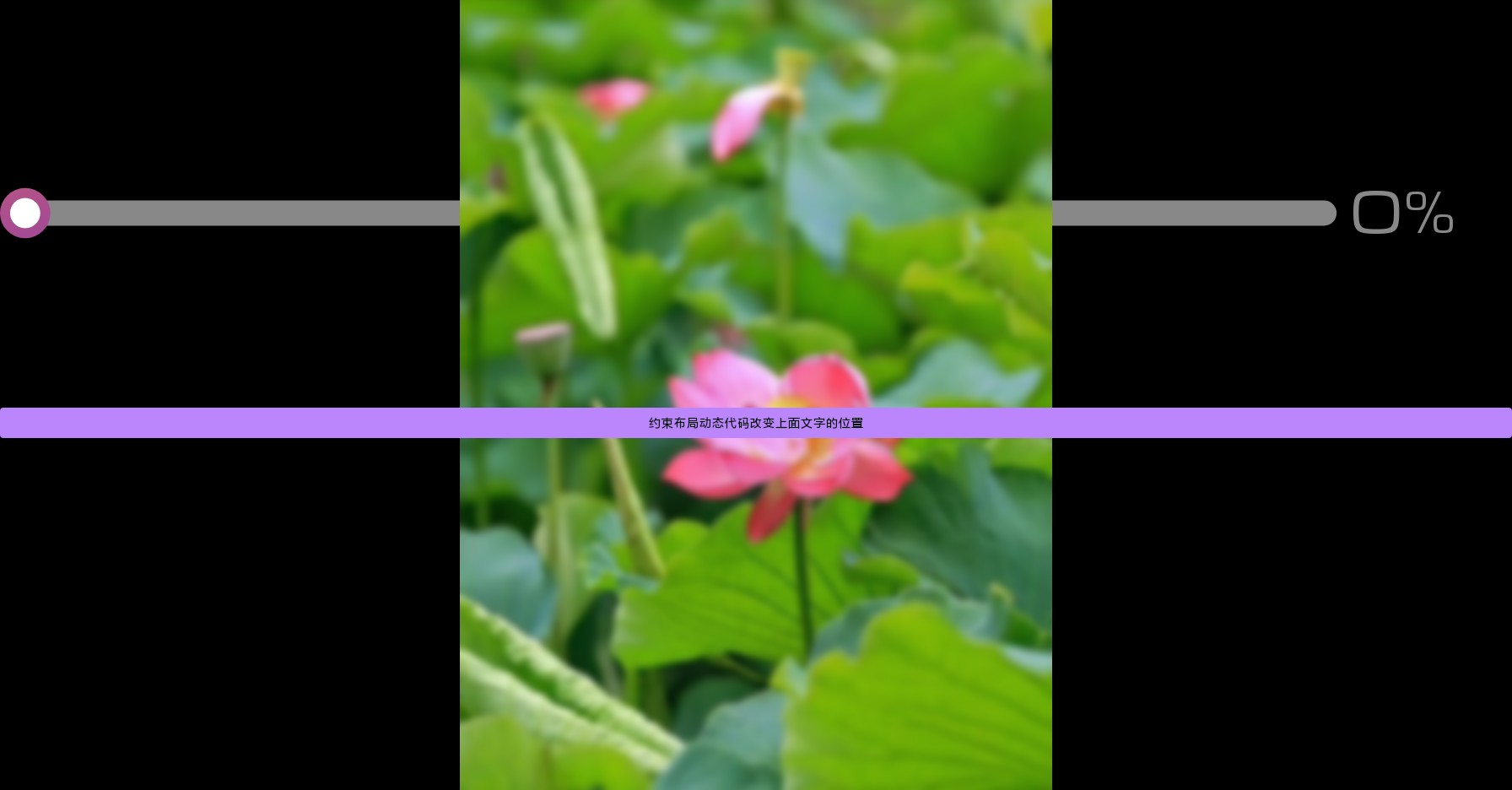
There are two methods, one is the Java version and the other is the Kotlin version
Java version screenshotView
/**
* 截图当前View的Java版本方法
* @param view view
* @return 返回截图
*/
public Bitmap getBitmapFromView(View view) {
Bitmap bitmap = Bitmap.createBitmap(view.getWidth(), view.getHeight(), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(bitmap);
view.layout(0, 0, view.getWidth(), view.getHeight());
Log.d("ss", "combineImages: width: " + view.getWidth());
Log.d("ss", "combineImages: height: " + view.getHeight());
view.draw(canvas);
view.requestLayout();
return bitmap;
}
Kotlin version screenshot View
Very simple one line of code
view.drawToBitmap()
Note that this method cannot be called immediately when the view is opened, and the following exception will be thrown
Caused by: java.lang.IllegalStateException: View needs to be laid out before calling drawToBitmap()
Caused by: java.lang.IllegalStateException: The view needs to be laid out before calling drawToBitmap()
Let’s see how to save it to the album ( you can check method 3 of this link : https://blog.csdn.net/xiayiye5/article/details/115251706)
package cn.yhsh.appwidget;
import android.content.ContentValues;
import android.content.Context;
import android.graphics.Bitmap;
import android.net.Uri;
import android.provider.MediaStore;
import android.util.Log;
import java.io.FileNotFoundException;
import java.io.OutputStream;
/**
* @author xiayiye5
* @date 2023/3/23 9:54
*/
public class SavePicUtils {
private static final SavePicUtils SAVE_PIC_UTILS = new SavePicUtils();
private SavePicUtils() {
}
public static SavePicUtils getInstance() {
return SAVE_PIC_UTILS;
}
/**
* API29 中的最新保存图片到相册的方法
*/
public void saveImage29(Context context, Bitmap toBitmap) {
//开始一个新的进程执行保存图片的操作
Uri insertUri = context.getContentResolver().insert(MediaStore.Images.Media.EXTERNAL_CONTENT_URI, new ContentValues());
//使用use可以自动关闭流
try {
OutputStream outputStream = context.getContentResolver().openOutputStream(insertUri, "rw");
if (toBitmap.compress(Bitmap.CompressFormat.JPEG, 90, outputStream)) {
Log.e("保存成功", "success");
} else {
Log.e("保存失败", "fail");
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
How to call?
SavePicUtils.getInstance().saveImage29(context, view.drawToBitmap())
Finally, remember to apply for dynamic permissions yourself. Writing pictures requires appropriate permissions
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />