The need for statistical counting is often used in many scenarios, such as counting the number of k label values when implementing the KNN algorithm, and then finding the label value with the largest number of labels as the final prediction result of the kNN algorithm. The Counter class in Python's built-in collections collection module can implement statistical counting concisely and efficiently.
Counter is a subclass of dict dictionary. Counter has key keys and value values similar to dictionaries, except that the key in Counter is the element to be counted, and the value value is count of the number of times the corresponding element appears. For the convenience of introduction, the unified use of element and count Count to represent. Although the count in Counter represents counting, Counter allows the value of count to be 0 or negative.
1. Instantiate the Counter class
If you want to use Counter, you must instantiate it. While instantiating, you can pass parameters to the constructor to specify different types of element sources.
from collections import Counter
#实例化元素为空的Counter对象
a=Counter()
#从可迭代对象中实例化 Counter 对象
b=Counter('andhajs')
#从mapping中实例化Counter对象,字典也属于mapping类对象
c=Counter({'a':2,'b':9,'c':1})
#从关键词参数中实例化 Counter 对象
d=Counter(a=2,b=4,c=1)
Instantiate the Counter object whose element is empty, and then add elements to the Counter object by adding elements to the dictionary.
from collections import Counter
#实例化元素为空的Counter对象
a=Counter()
#为 Counter 添加元素以及对应的 count 计数
a['a']=1
a['b']=a.get('b',10)
print(a)
operation result:
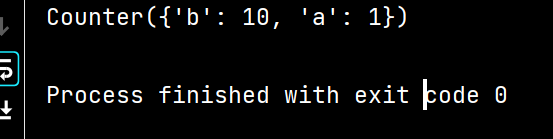
Get elements from iterable objects such as string (characters are elements of list list), list and tuple.
from collections import Counter
# 从可迭代对象中实例化 Counter
b = Counter("chenkc") # string
b2 = Counter(['c', 'h', 'e', 'n', 'k', 'c']) # list
b3 = Counter(('c', 'h', 'e', 'n', 'k', 'c')) # tuple
print('b:',b)
print('b2:',b2)
print('b3:',b3)
operation result:
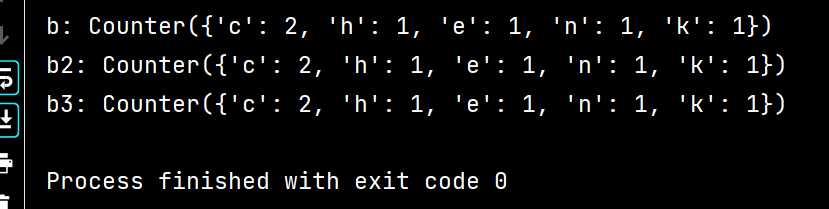
Instantiate the Counter object from the mapping. The data of the mapping type is a list whose elements are (x, y), and the dictionary also belongs to the data of the mapping type.
from collections import Counter
# 从 mapping 中实例化 Counter 对象
c = Counter([('a', 1), ('b', 2), ('a', 3), ('c', 3)])
c2 = Counter({'a': 1, 'b': 2, 'a': 3, 'c': 3}) # 字典
print('c:',c)
print('c2:',c2)
operation result:

Although the data of the incoming mapping type is the same, since the keys in the dictionary are unique, if the keys in the dictionary are repeated, the last one will be kept.
dic = {'a':1, 'b':2, 'a':3, 'c':3}
>>> print(dic)
{'a': 3, 'b': 2, 'c': 3}
Instantiate the Counter object from the keyword parameter. The keyword specified in the keyword parameter must be unique, but unlike a dictionary, if the specified keyword is repeated, the program will throw a SyntaxError exception.
from collections import Counter
# 从关键词参数中实例化 Counter 对象
d = Counter(a = 1, b = 2, c = 3)
# d2 = Counter(a = 1, b = 2, a = 3, c = 3) # SyntaxError
>>> print(d)
Counter({'c': 3, 'b': 2, 'a': 1})
We all know that if you look for a key that does not exist in the dictionary, the program will throw an exception of KyeError, but because the Counter is used for counting, the Counter is different from the dictionary. If you look for an element that does not exist in the Counter, no exception will be generated. Instead, it will return 0, which is actually easy to understand. The Counter count sets the count value of the non-existing element to 0.
from collections import Counter
c = Counter({'a':1, 'b':2, 'c':3})
>>> print(c['d']) # 查找键值为'd'对应的计数
0
>>> print(c)
Counter({'c': 3, 'b': 2, 'a': 1})
The search represented by c['d'] returns the count count whose element value is d, and if c['d'] = 0, it means adding elements to the Counter.
from collections import Counter
c = Counter({'a':1, 'b':2, 'c':3})
c['d'] = 4 # 为 Counter 添加元素
>>> print(c['d'])
4
>>> print(c)
Counter({'d': 4, 'c': 3, 'b': 2, 'a': 1})
2. Methods in Counter
After instantiating the Counter class object, you can use the methods in the Counter object. Since the Counter class inherits from the dict class, the Counter class can use the methods of the dict class. The following introduces the unique methods of Counter and some common methods of dictionaries.
2.1 Unique methods in Counter
Counter supports three additional methods not found in dictionaries: elements(), most_common([m]), and subtract([iterable-or-mapping]).
elements method
The elements() method returns an iterator, and the elements in the iterator can be output through list or other methods, and the output result is the element corresponding to the number of occurrences.
from collections import Counter
c = Counter({'a':1, 'b':2, 'c':3})
c2 = Counter({'a':0, 'b':-1, 'c':3}) # 将出现次数设置为 0 和负值
>>> print(c.elements())
<itertools.chain object at 0x0000022A57509B70>
>>> print(list(c.elements()))
['a', 'b', 'b', 'c', 'c', 'c']
>>> print(c2.elements())
<itertools.chain object at 0x0000022A57509B70>
>>> print(list(c2.elements()))#只输出正的value对应的key
['c', 'c', 'c']
In Counter, the count is allowed to be 0 or a negative value, but it can be seen from the above code that the elements function does not print out the element values corresponding to 0 and negative values .
most_common method
most_common([n]) is the most common method of Counter, returning a list of the first n elements with the number of occurrences in descending order.
from collections import Counter
c = Counter({'a':1, 'b':2, 'c':3})
>>> print(c.most_common()) # 默认参数
[('c', 3), ('b', 2), ('a', 1)]
>>> print(c.most_common(2)) # n = 2
[('c', 3), ('b', 2)]
>>> print(c.most_common(3)) # n = 3
[('c', 3), ('b', 2), ('a', 1)]
>>> print(c.most_common(-1)) # n = -1
[]
n is an optional parameter, which can be concluded from the above code:
If n is not entered, all will be returned by default;
If the input n is less than the longest length, the first n numbers will be returned;
Input n is equal to the longest length, then return all;
Input n = -1, return empty;
subtract method
The subtract([iterable_or_mapping]) method actually subtracts the counts corresponding to the elements in the two Counter objects.
from collections import Counter
c = Counter({'a':1, 'b':2, 'c':3})
d = Counter({'a':1, 'b':3, 'c':2, 'd':2})
c.subtract(d)
>>> print(c)
Counter({'c': 1, 'a': 0, 'b': -1, 'd': -2})
In fact, it is the subtraction of the counts of the corresponding elements in the two Counters. When the corresponding element in one of the Counters does not exist, its count is set to 0 by default, which is why the count of 'd' is -2.
2.2 Dictionary methods supported by Counter
Generally, the conventional dictionary methods are valid for the Counter object, apply these dictionary methods to the Counter object c below, and draw it in the table below.
from collections import Counter
c = Counter({'a':1, 'b':2, 'c':3, 'd':0, 'e':-1})
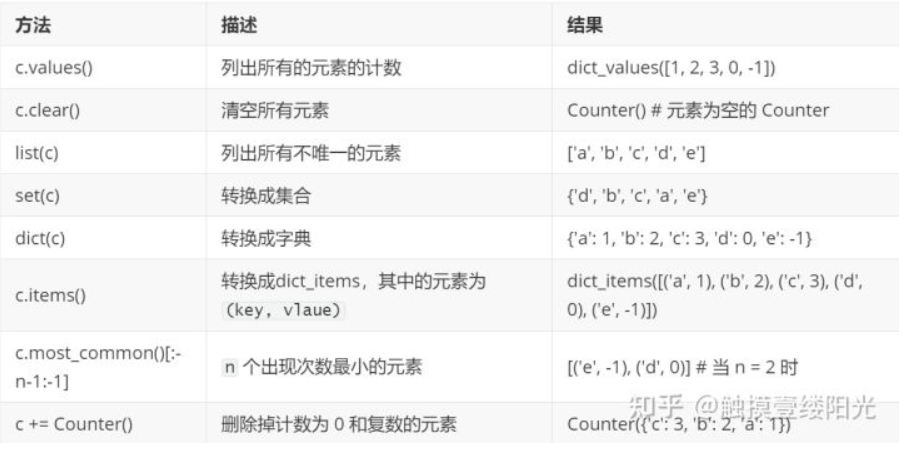
But there are two methods in Counter that are slightly different from those used in dictionaries:

from collections import Counter
c = Counter({'a':1, 'b':2, 'c':3, 'd':0, 'e':-1})
c.update({'a':2, 'd':2, 'e':1})
>>> print(c)
Counter({'a': 3, 'c': 3, 'b': 2, 'd': 2, 'e': 0})
For the update function in Counter, simply speaking, it is to increase the count of the corresponding element.
2.3 Set operators
The code example of the set operator is posted directly here.
>>> c = Counter(a=3, b=1)
>>> d = Counter(a=1, b=2)
>>> c + d # add two counters together: c[x] + d[x]
Counter({'a': 4, 'b': 3})
>>> c - d # subtract (keeping only positive counts)
Counter({'a': 2})
>>> c & d # intersection: min(c[x], d[x])
Counter({'a': 1, 'b': 1})
>>> c | d # union: max(c[x], d[x])
Counter({'a': 3, 'b': 2})