Article Directory
Introduction to WebSocket
WebSocket is a protocol based on full-duplex communication on a single TCP connection. It is a network technology for full-duplex communication between browsers and servers that has been provided since HTML5. It solves the problem that the HTTP protocol is not suitable for real-time communication. Compared with the HTTP protocol, the WebSocket protocol realizes persistent network communication, can realize long-term connections between the client and the server, and can perform two-way real-time communication. The protocol name is "ws".
The difference between HTTP and WebSocket
- Both are based on the TCP protocol for data transmission, with reliable transmission capabilities, and both are application layer protocols
- HTTP is a one-way communication. The client can only send requests to the server, and the server cannot actively send messages to the client.
- WebSocket is a two-way communication. The client and the server establish a connection through a handshake, which can realize full-duplex communication. Both parties can actively send messages to each other at the same time.
- When WebSocket establishes a connection, the data is transmitted through the HTTP protocol, but after the establishment, the more reliable TCP protocol is used to transmit the data.
relationship between the two
- When WebSocket establishes a connection, HTTP uses the Upgrade message header to notify the client that it needs to use the WebSocket protocol to complete the request. After sending the last blank line of the response, the server will switch to the WebSocket protocol, and the interface returns a status code of , which
101
means The server has understood the client's request and needs to upgrade the protocol
WebSocket test method
use online tools
There are many online tools, Baidu it yourself! I am used to using this tool
Use Postman
Postman supports testing the interface of the WebSocket protocol. When this article was published, it was still in the public beta stage. It needs to be logged in before it can be used. The entrance is shown in the figure below
The operation is similar to testing HTTP requests, but let’s introduce more! As shown below
Use Jmeter
Using Jmeter, you can test the interface alone or perform performance testing, but you need to install a plug-in to test the WebSocket protocol. After downloading the plug-in , put it in the directory in the Jmeter installation path lib/ext
. After restarting Jmeter, [Plugins Manager] will appear in the [Options] menu. , then search for WebSocket on the available plug-ins Tab page, and install it, as shown in the figure below
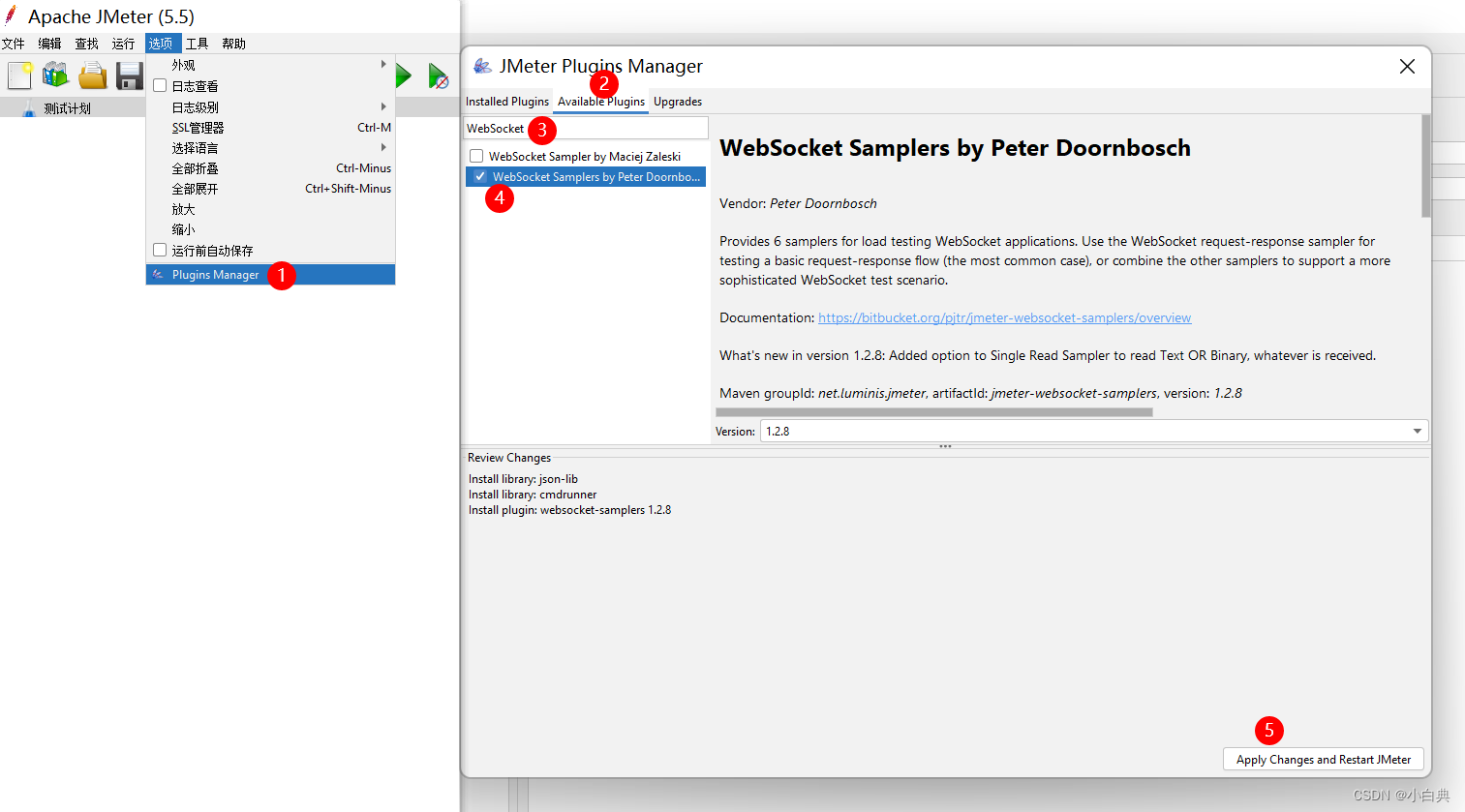
Create a new [Thread Group] after installation, and 6 WebSocket components will appear in the Add [Sampler]
- WebSocket Close: used to close the WebSocket connection
- WebSocket Open Connection: Only establish a WebSocket connection without sending data
- WebSocket Ping/Pong: used for heartbeat detection, in order to maintain a long connection and prevent the client from being disconnected if it is judged to be inactive by the server
- WebSocket Single Read Sampler: used to receive one (text or binary) data
- WebSocket Single Write Sampler: used to send one (text or binary) data
- WebSocket request-response Sampler: Used to perform basic request and response exchanges, both sending and receiving data
If you have used Jmeter, you will definitely understand how to use these components. Taking WebSocket request-response Sampler as an example, the page information is introduced as shown in the figure below
use python
First you need to install websocket-client
pip install websocket-client
For testing whether the sending and receiving of messages by the WebSocket interface is normal and does not require a long connection, you can use the following short connection method, which will actively disconnect after the request ends
from websocket import create_connection
user1 = create_connection("ws://localhost:6688/user/1") # 用户1打开连接
user2 = create_connection("ws://localhost:6688/user/2") # 用户2打开连接
print("获取响应状态码:", user1.getstatus()) # 正常应返回101
print("获取响应头:", user2.getheaders())
user1.send("你好呀!") # 用户1向服务端发送消息
res1 = user1.recv() # 查看服务端推送的消息
res2 = user2.recv()
if res1 == res2:
print("服务端消息推送成功!\n")
else:
print("消息获取异常!\n")
print("查看用户1收到的消息:",res1,"\n查看用户2收到的消息:",res2)
If you want to do continuous monitoring work on the WebSocket interface, you need a long connection, but you don't need to pass parameters, just monitor messages, it is more appropriate to use the WebSocketApp class! Methods as below
import websocket
def on_open(ws): # 定义用来处理打开连接的方法
print("打开连接")
def on_message(ws, message): # 定义用来监听服务器返回消息的方法
print("监听到服务器返回的消息,:\n", message)
def on_error(ws, error): # 定义用来处理错误的方法
print("连接出现异常:\n", error)
def on_close(ws): # 定义用来处理断开连接的方法
print("关闭连接")
if __name__ == "__main__":
websocket.enableTrace(True) # 可选择开启跟踪,在控制台可以看到详细的信息
ws = websocket.WebSocketApp("ws://localhost:6688",
on_open=on_open,
on_message=on_message,
on_error=on_error,
on_close=on_close)
ws.run_forever() # 调用run_forever方法,保持长连接
You can also use Pytest to automate the testing of the WebSocket interface, as shown in the following examples
import pytest
from websocket import create_connection
class TestDyd():
url = "ws://localhost:6688"
@classmethod
def setup_class(cls):
cls.ws = create_connection(cls.url) # 建立连接
cls.ws.settimeout(5) # 设置超时时间
def test_connect(self):
# 通过状态码判断连接是否正常
assert self.ws.getstatus() == 101
def test_send(self):
params = "你好呀!" # 定义传参
self.ws.send(params) # 发送请求
result = self.ws.recv() # 获取响应结果
print("收到来自服务端的消息:", result) # 打印响应结果
# 因为该测试项目传参会显示在响应中,所以通过判断传参是否在响应结果中进行断言
assert params in result
if __name__ == '__main__':
pytest.main(["-vs"])
You can use @pytest.mark.parametrize()
decorators to pass parameters, examples are as follows
import pytest
from websocket import create_connection
class TestDyd():
data = [("/user/2", "今天开直播卖鱼!!!!"),("/user/3", "鱼可以买来放生吗?"),
("/user/4", "那条翻白肚的鱼有死亡证明吗?"),("/user/5", "你的鱼会唱歌吗?"),
("/user/6", "你的鱼上大学了吗?"),("/user/7", "你的鱼买回来需要隔离吗?")]
@pytest.mark.parametrize("user, word", data)
def test_send(self, user, word):
ws = create_connection("ws://localhost:6688"+user)
params = word
ws.send(params) # 发送请求
result = ws.recv() # 获取响应结果
print("来自服务端的消息:", result)
assert params in result # 断言
Let’s briefly introduce these first. Regarding Python scripts, you can refer to the official documentation , which contains examples and solutions to common problems.