1. Determine whether it is an array, a string, etc.
a instanceof b
a is the data you need to judge
b is the type of judgment
//直接判断原型
var a = [1,5,8]
var b = '123456'
console.log(a instanceof Array)//true
console.log(a instanceof String)//false
console.log(b instanceof String)//true
2. Split the string
a.split(str,num)
a is the string to be split
str is the delimiter, the default is empty
num times of division
var a ='api/Getuser'
var b = a.split("/");
console.log(b)//['api','Getuser']
3. Replace the specified string
a.replace(old,new)
a is the string to be replaced
old is the string to be replaced (old string)
The string replaced by new (new string)
var a ='{
{url}}/api/GetList'
var b = a.split('/')//先分割字符串
console.log(b) //['{
{url}}','api','GetList']
//第一种方法--变量
var c = a.replace(b[0],'http://198.4.100:8090');
//第二种--字符串
var c = a.replace(/{
{url}}/,'http://198.4.100:8090');
console.log(c) //'http://198.4.100:8090/api/GetList'
4. Array sorting
Perform multiple selections in the table, and the order of data after multiple selections is sorted according to the order you selected. My need is to reorder the multi-selected data according to the id from small to large.
get () {
this.numbers = [
{ id: 1, zu: '王小虎1', state: 1, },
{ id: 4, zu: '王小虎2', state: 1 },
{ id: 3, zu: '王小虎3', state: 1 },
{ id: 2, zu: '王小虎4', state: 1 },
]
var compare = function (prop) {
return function (obj1, obj2) {
var val1 = obj1[prop];
var val2 = obj2[prop];
if (!isNaN(Number(val1)) && !isNaN(Number(val2))) {
val1 = Number(val1);
val2 = Number(val2);
}
if (val1 < val2) {
return -1;
} else if (val1 > val2) {
return 1;
} else {
return 0;
}
}
}
this.numbers.sort(compare('id'))
console.log(this.numbers.sort(compare('id')));
}
Sorting according to name ( string ), it is sorting according to abc order
get () {
var arr = [{ name: "zlw", age: 24 }, { name: "wlz", age: 25 }];
var compare = function (obj1, obj2) {
var val1 = obj1.name;
var val2 = obj2.name;
if (val1 < val2) {
return -1;
} else if (val1 > val2) {
return 1;
} else {
return 0;
}
}
console.log(arr.sort(compare));
}
5. Chinese characters are evenly wrapped, that is, the context words are as consistent as possible
The meaning of text wrapping equally is that if there are 10 characters, 5 characters will be displayed above and below, and 4 characters will be displayed above and below if there are 8 characters. Try to make the two lines of text display the same
What I use is to get the length of the string first, and then divide it by 2 to see if it is completely divided. If it is completely divided, it will break the line. If it cannot be divided, add 1 and then change the line.
huanhang(_str) {
var str = _str,
result = "";
// 判断字符串长度多少后除以2,除后有小数点就加1换行,没有则直接换行
var rep = /[\.]/;
if (str.length > 5) {//低于5个字就不换行
let a = (str.length / 2)
if (rep.test(a)) {
var n = parseInt(str.length / 2) + 1; //指定第n位换行
} else {
var n = parseInt(str.length / 2); //指定第n位换行
}
for (var i = 0, len = str.length; i < len; i++) {
result += str[i];
if ((i + 1) % n == 0)
result += "\r\n";
}
return result;
} else {
return str
}
},
use:
<text>{
{huanhang(SceneData.Title1)}}</text>
6. Line break according to the symbols in the string
String: 'Detection item 1; Detection item 2'
Note: must use v-html
<div v-html="jcbz.replace(/\;/g, '<br />')">
7. Remove repeated strings from array objects
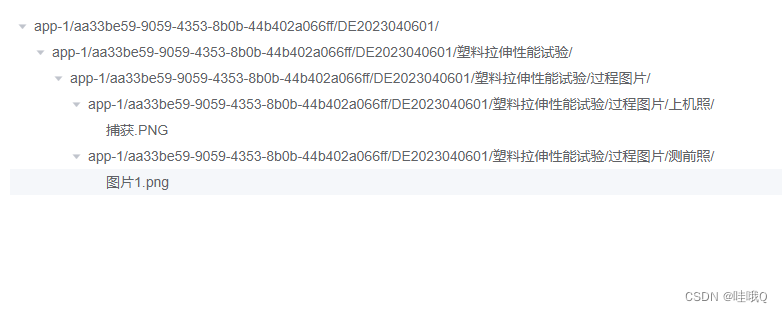
modify it like this
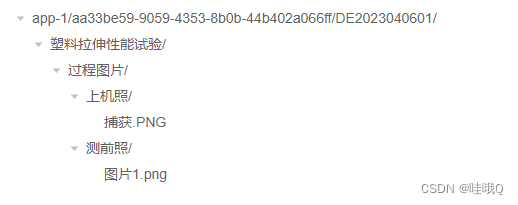
The return type of the interface is as follows, the last level does not return children, only fileName and id are returned, and the rest return children and fileName
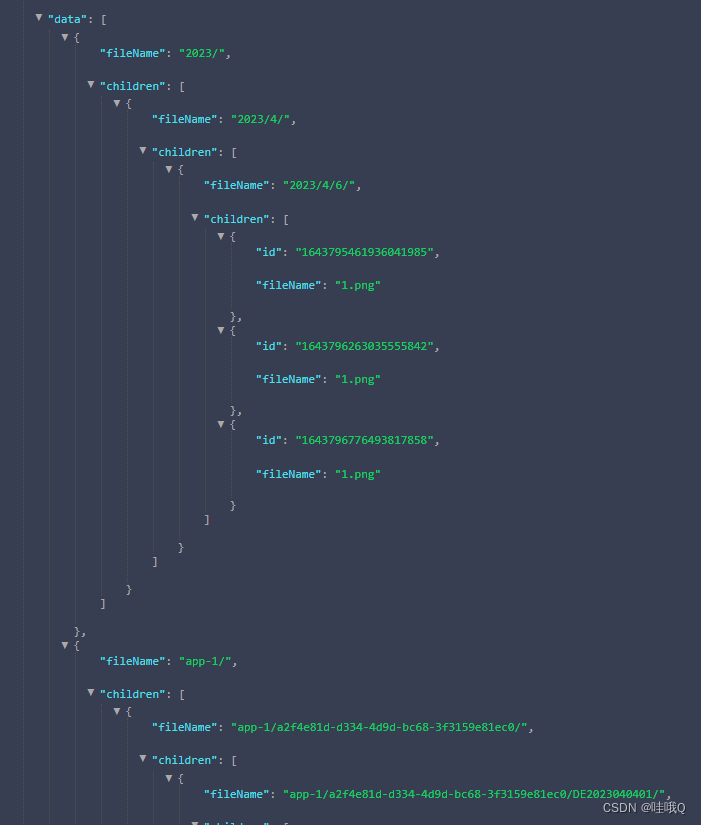
getne(item) {
item.map((i, index) => {
if (i.children && i.children.length > 0) {
i.children.forEach((j, ind) => {
if (j.fileName.indexOf(i.fileName) !== -1) {
const a = j.fileName.split(i.fileName);
if (a[0] === "") {
// 第一次
j.fileName = j.fileName.replace(i.fileName, "");
} else {
// 剩余次数
j.fileName = a[a.length - 1];
}
this.getne(i.children);
}
});
}
});
},
//接口调用
async getFileAllList(item) {
// const res = await getFileAllList({ FilePath: "" });//查看全部文件
const res = await getFileAllList({
FilePath: item["文件路径"],
});
this.getne(res.data);
this.treeData = res.data;
},