1. Data type
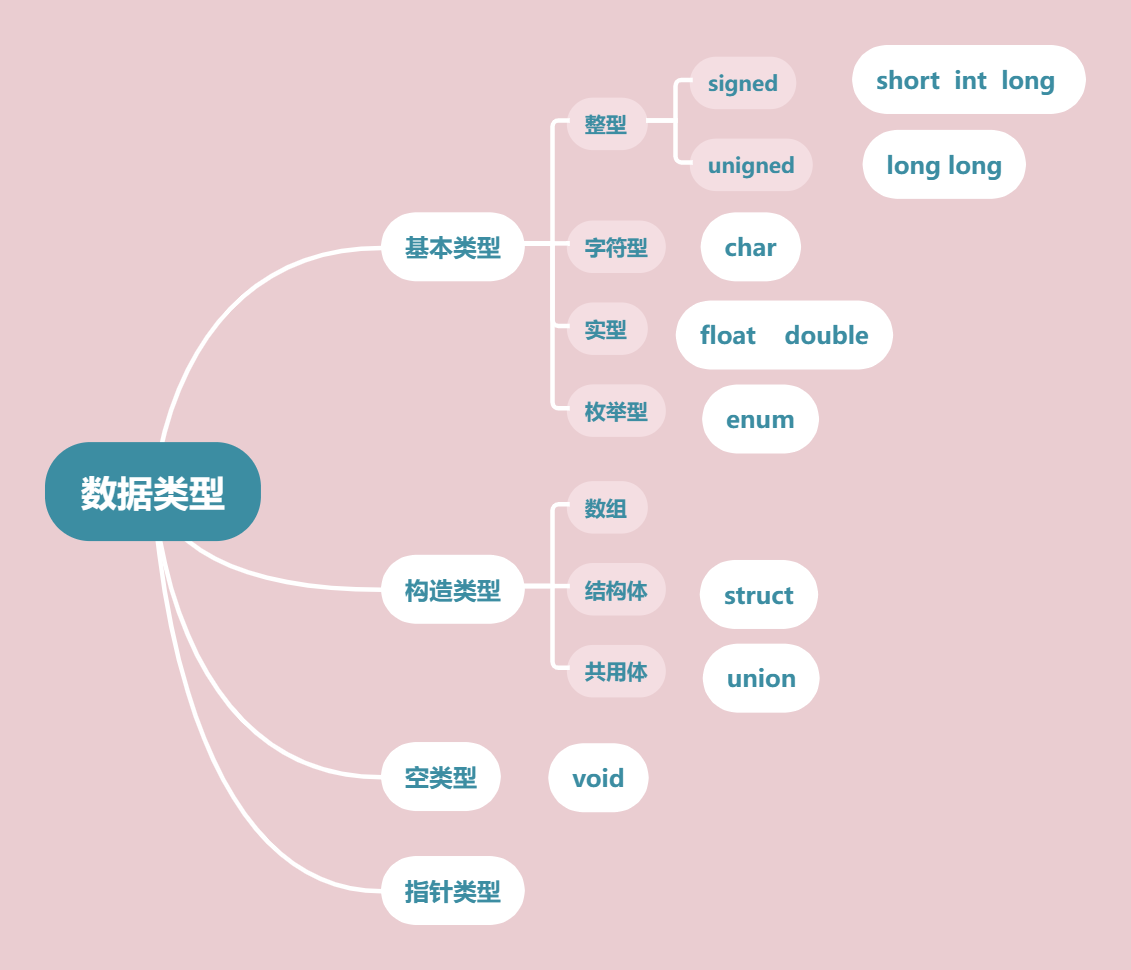
signed: can access signed numbers, including positive and negative numbers
unsigned: can only access unsigned numbers, positive numbers
1byte=8bit
The original inverse complement of positive numbers is the same, the inverse of negative numbers is equal to the sign bit of the original code, and the other bits are reversed in turn, the complement is equal to the inverse plus 1, 0 is positive, 1 is negative
Two, constant
Octal numbers start with 0 eg 06434 3 digits
Hex starts with 0X, such as 0Xd1c 4 digits
- 1.176e+10 means 1.176*10 to the 10th power-
- -3.5789e-8 means -3.5789*10 to the negative 8th power
-Usually means a particularly large or small number, it is not necessary to write
The difference between uppercase and lowercase letters in ASCII code is 32, and the difference between character 0 and number 0 is 48;
'A'+ ' ' = 'a' ' ' means 32
0='0'-48
Identity constants #define PI 3.1415926
The macro is just expanded as it is, and it is not calculated. Parentheses can be added to avoid errors
3. Variables
The variable name is composed of letters, numbers, and underscores, and cannot start with a number, and cannot have the same name as a C keyword
The first address of a variable in the memory space is called the address of the variable.
Register variable cannot get address
The default value of static variable is 0
Fourth, the operator
float/double cannot take remainder
Logical AND''&&'': cut off every 0, and the following ones will not be executed
Logical OR''||'': cut off every 1, the following will not be executed
~ |
bit logic inversion |
~a |
& |
bit logical AND |
a&b |
| |
bit OR |
a|b |
^ |
Bit Logical XOR |
a^b (different is true) |
>> |
right shift |
a>>1() |
<< |
left shift |
b<<4 (relationship of shifting to the left and filling the right with 0, multiplying by 2) |
#include <stdio.h>
voin main(int argc,char *argv[]){
unsigned char x=0x17,y;
y=~x;
printf("%#x\n",y);
return 0;
}
The above x represents hexadecimal, # represents the output result with 0x
The comma operator operates sequentially in parentheses, and the last one is taken
sizeof (type or variable name): used to calculate the space occupied by memory
#include <stdio.h>
voin main(int argc,char *argv[]){
int a=5;
long b=6;
printf("%d %d %d\n",sizeof(a),sizeof(b));
printf("%d %d %d\n",sizeof(int),sizeof(long));
return 0;
}