Article directory
- foreword
- 1. Material preparation
- 2. Use the PIL library to add image watermarks to images
-
- 1. Import library
- 2. Define the image path
- 3. Open the original image
- 4. Open the watermark image
- 5. Calculate the watermark image size
- 6. Calculate the size of the original image
- 7. Adjust the watermark image size
- 8. Calculate the position of the watermark image
- 9. Add watermark
- 10. Save the new graph
- Summarize
foreword
Hello everyone, I am Kongkong star. In this article, I will share with you how to add image watermarks to images through Python's PIL library.
The previous article has already introduced what is the PIL library? Install PIL and view the PIL version, which will not be introduced here.
1. Material preparation
1. Original image
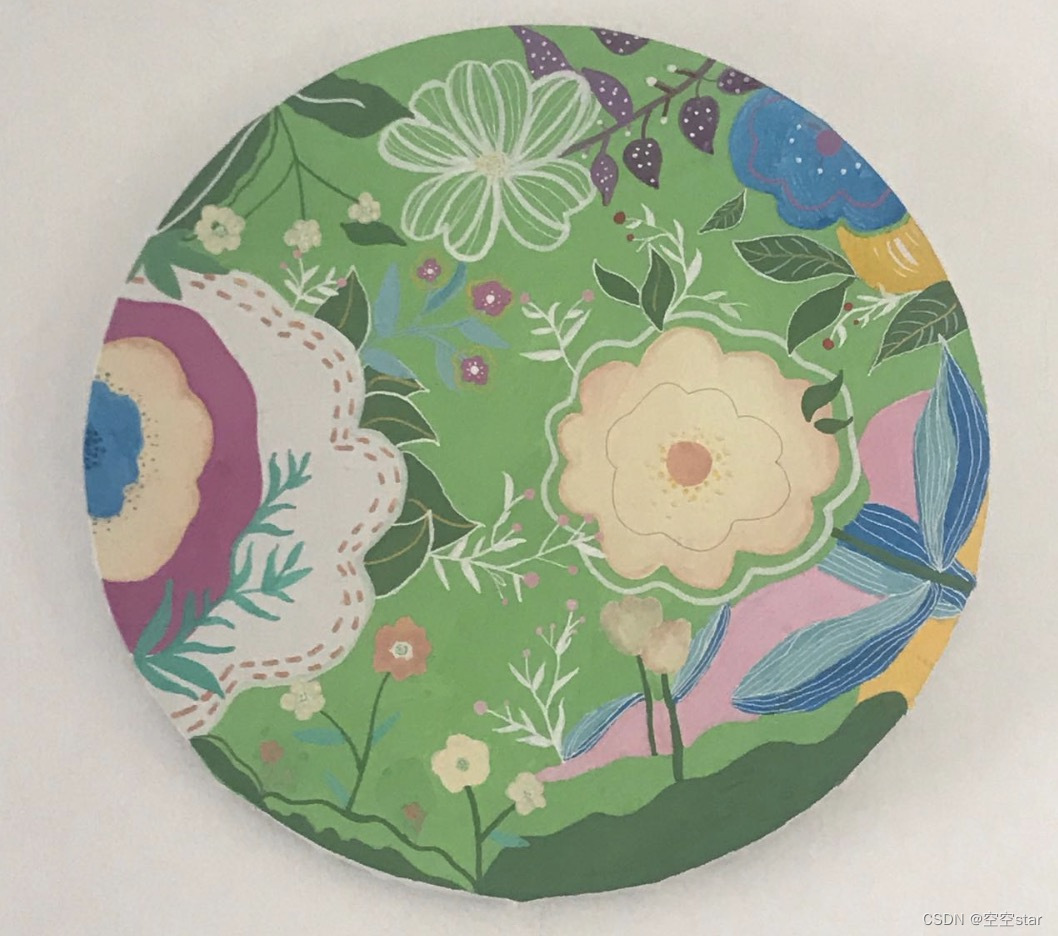
2. Watermark image
Here I went to the homepage of Station C to find one. Does it look cool?
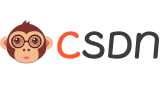
2. Use the PIL library to add image watermarks to images
1. Import library
from PIL import Image
2. Define the image path
local = '/Users/kkstar/Downloads/video/pic/'
3. Open the original image
img = Image.open(local+'demo.jpg')
4. Open the watermark image
watermark = Image.open(local+'csdn.png')
5. Calculate the watermark image size
wm_width, wm_height = watermark.size
6. Calculate the size of the original image
img_width, img_height = img.size
7. Adjust the watermark image size
7.1 Before adjustment
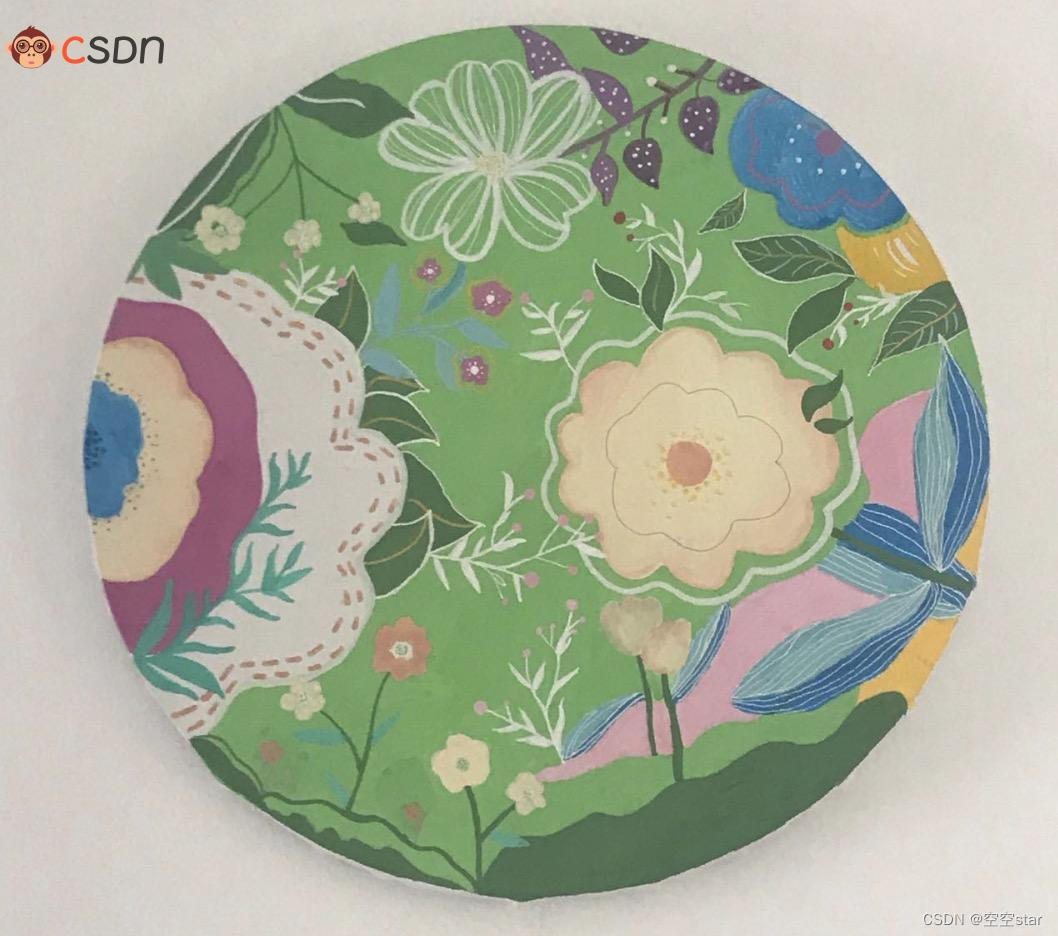
7.2 After adjustment
wm_width = int(wm_width*1.5)
wm_height = int(wm_height*1.5)
watermark = watermark.resize((wm_width, wm_height))
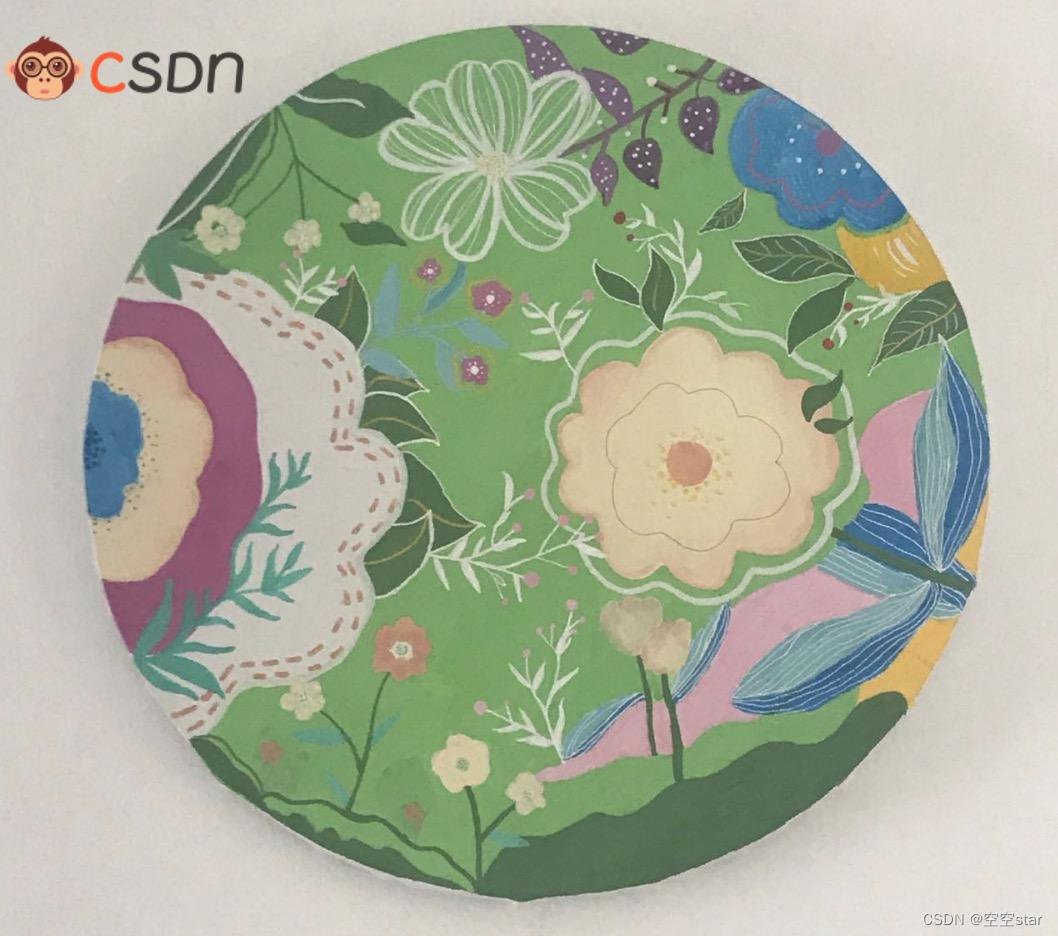
8. Calculate the position of the watermark image
8.1 upper left
x = 5
y = 5
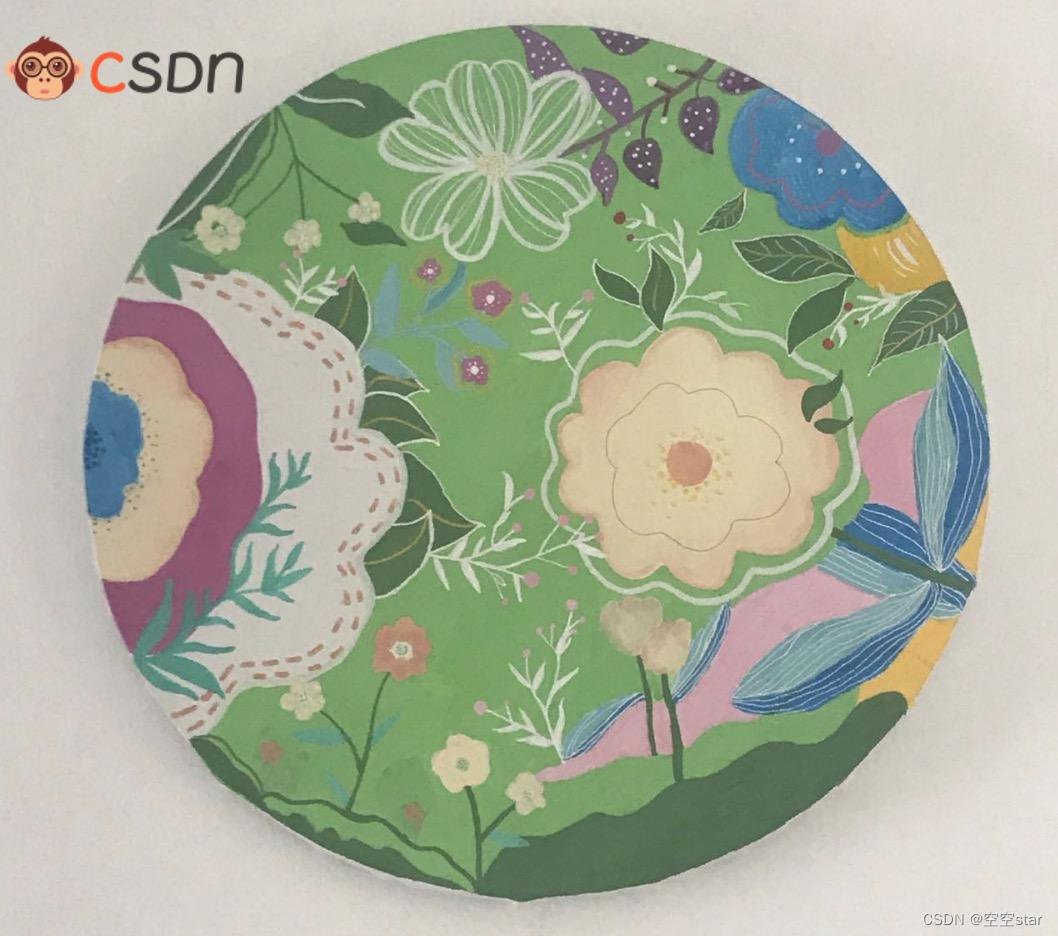
8.2 lower left
x = 5
y = img_height - wm_height - 5
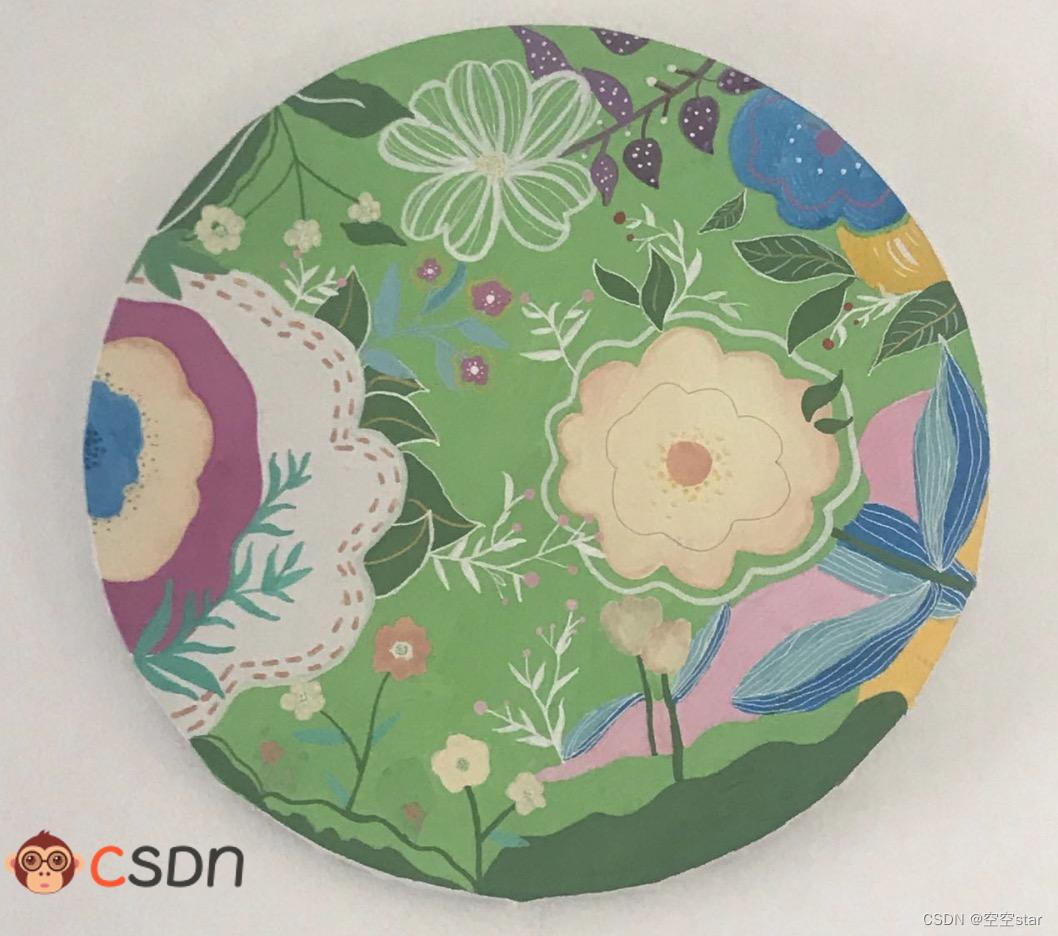
8.3 upper right
x = img_width - wm_width - 5
y = 5
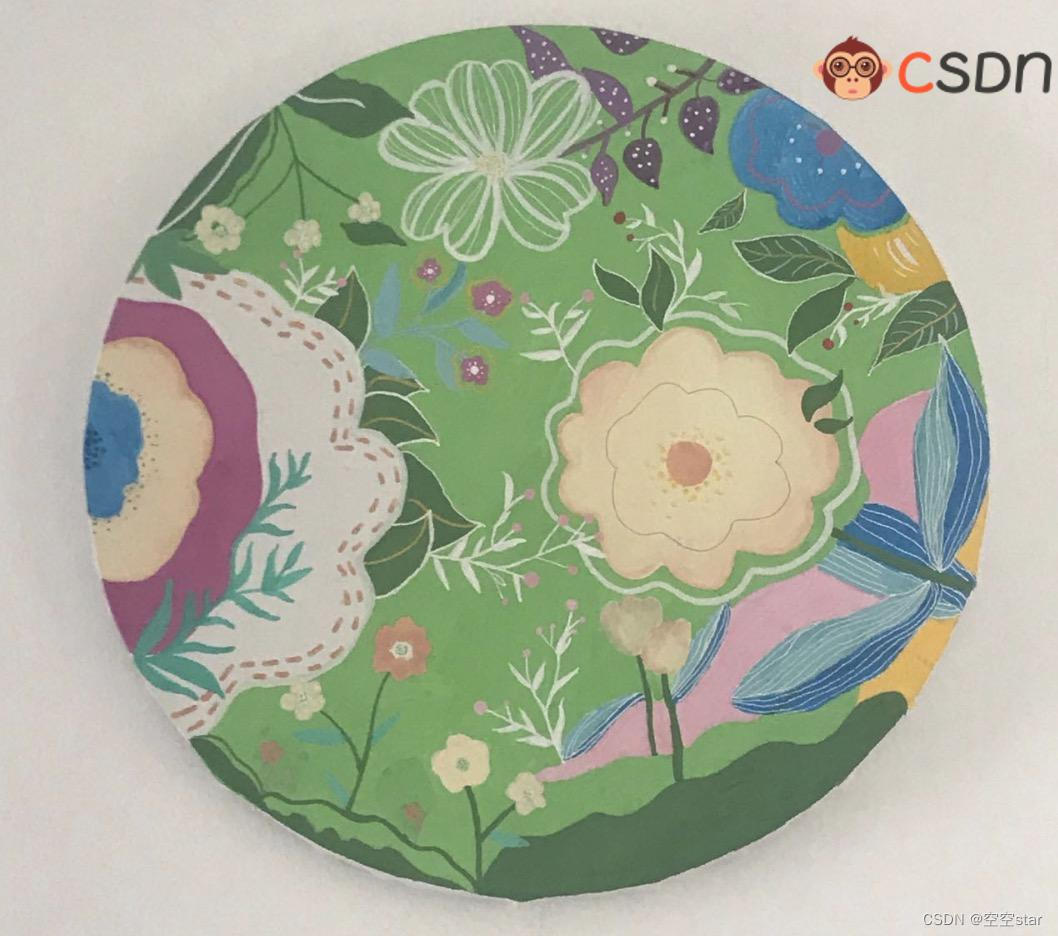
8.4 lower right
x = img_width - wm_width - 5
y = img_height - wm_height - 5
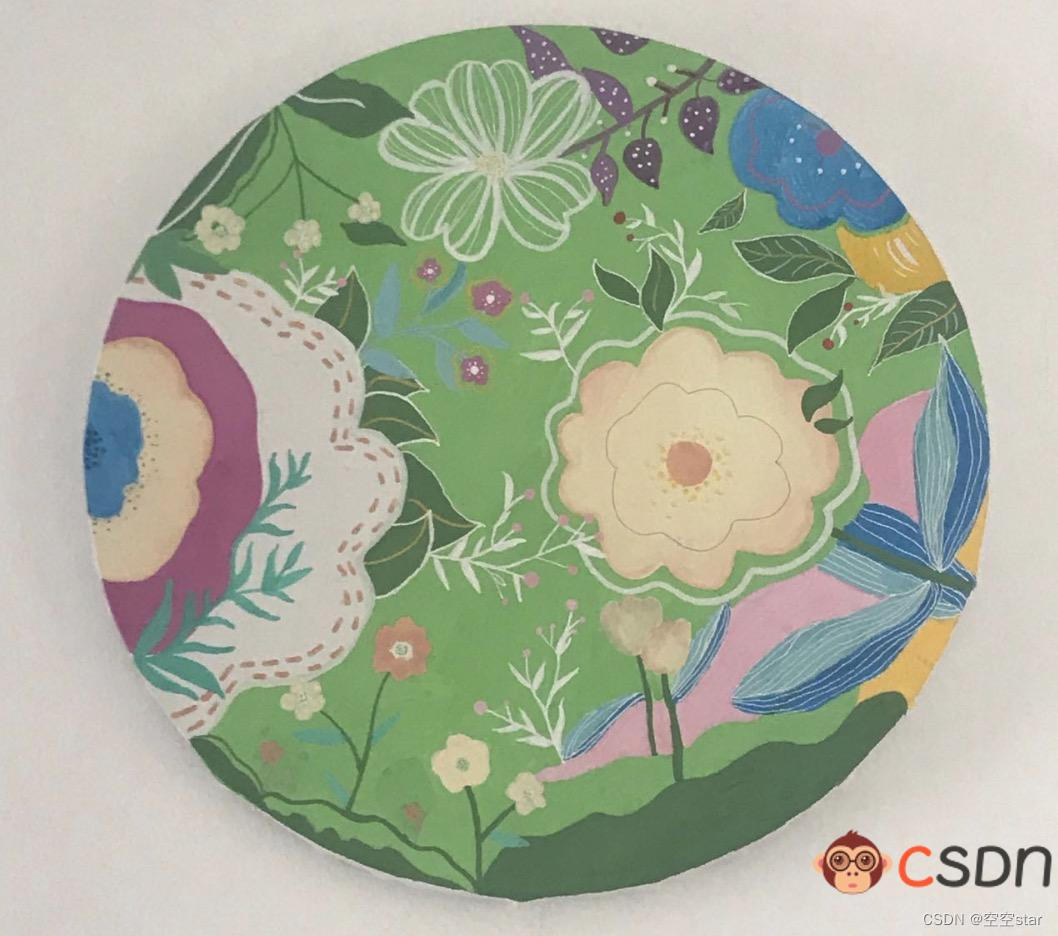
8.5 Intermediate
x = int((img_width - wm_width)/2)
y = int((img_height - wm_height)/2)
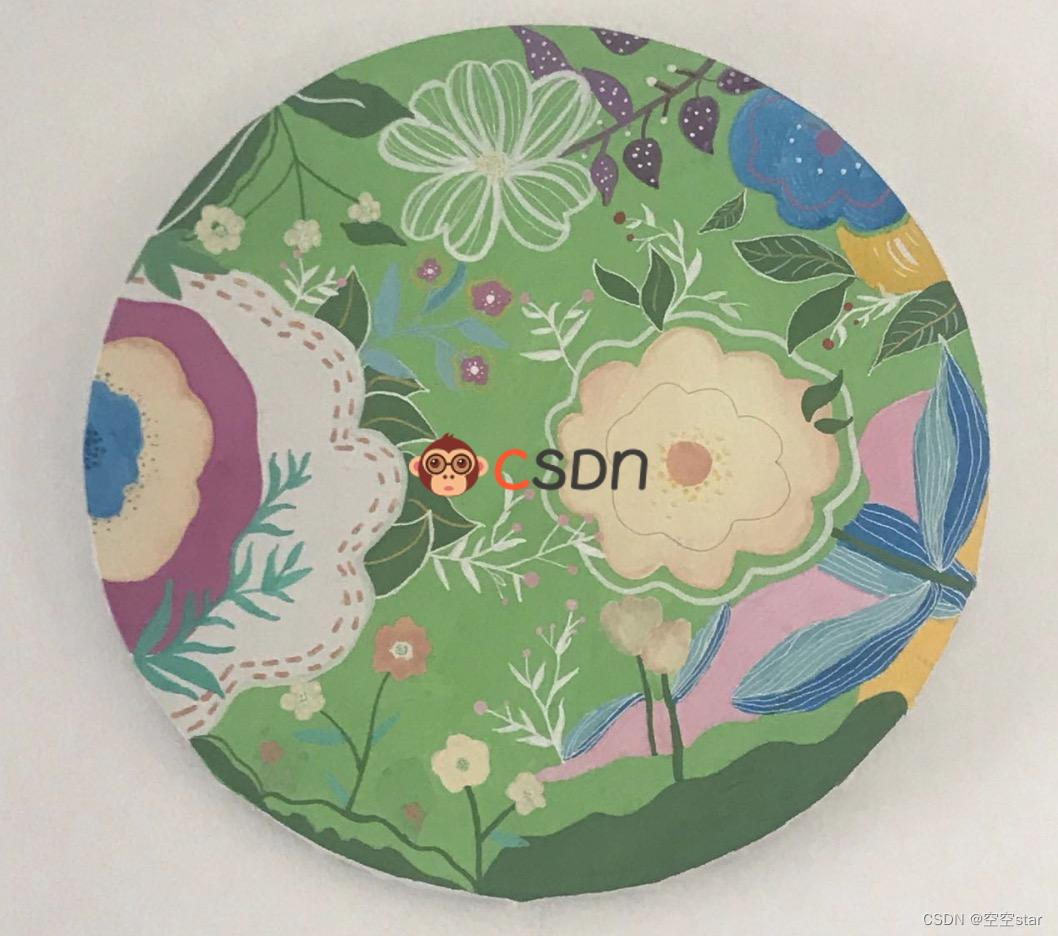
Other positions can be calculated by adjusting the values of x and y.
9. Add watermark
img.paste(watermark, (x, y), watermark)
10. Save the new graph
img.save(local+'result.jpg')
Summarize
- Use the PIL library to open the image that needs to be watermarked, and you can use the open() function.
- Use the PIL library to open the watermarked image and get the watermarked image. You can open the watermarked image in the same way as above.
- To add a watermark image to the original image, you can use the paste() function.
- To save the watermarked image, you can use the save() function.
image.size
: Returns a tuple containing image dimensions (width and height). For example, for a 400x300 pixel image, this method returns (400, 300). By calling the image.size method, you can obtain the size information of the image and perform subsequent processing.
image.resize
: It can be used to adjust the size of the image, and it can also use different interpolation methods to process pixels. By specifying the size of the image to be processed and the interpolation method, various forms of transformation such as scaling, cropping, and rotation of the image can be realized. The syntax of the resize method is: image.resize(size, resample=None), where size is a two-tuple indicating the new image size, resample is the interpolation method, and the default is None, indicating that the nearest neighbor interpolation is used.
image.paste
: It is used to paste one image onto another image, and the watermark image can be embedded into the target image. In step 9, the first input is the image to be pasted, the second is the coordinates of the upper left corner of the pasted image, and the third parameter is the mask of the image to be pasted.
image.save
: Used to save the image as a file, you can specify the image format, quality, compression and other parameters. This method supports a variety of image formats, including JPG, PNG, BMP, etc. At the same time, operations such as format conversion and size adjustment can be performed through parameters.