According to project requirements, it is necessary to perform remote search for each corresponding search field
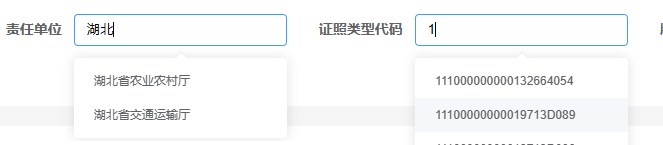
There are many places in the project that need to be used, so it is very convenient to perform secondary packaging.
Create an ElSelectV2 folder ==> index.vue
<template>
<div>
<el-select-v2
v-model="valueName"
filterable
remote
:remote-method="remoteMethod"
:options="options"
:loading="loading"
placeholder="请输入"
/>
</div>
</template>
<script setup name="ElSelectV2">
import {reactive, toRefs, watch} from "vue";
const emit = defineEmits();
const valueName = ref(''); // 选中的值
const states = ref([]); // 接收数据
const options = ref([]); // 遍历出来的选项
const loading = ref(false); // 是否加载中
const props = defineProps({
modelValue: [String, Object, Array],
isShowTip: {
type: String,
default: true,
},
});
// 监听 valueName不能写成valueName.value
watch(valueName, (val) => {
remoteMethod(val);
});
function remoteMethod(query) {
// 判断props.modelValue和props.isShowTip是否存在
if (!props.modelValue || !props.isShowTip) {
return
}
const list = props.modelValue.map((item) => {
return { value: `${item[props.isShowTip]}`, label: `${item[props.isShowTip]}` }
})
if (query !== '') {
loading.value = true
setTimeout(() => {
loading.value = false
options.value = list.filter((item) => {
return item.label.toLowerCase().includes(query.toLowerCase())
})
// 去重
options.value = Array.from(new Set(options.value.map(item => item.label))).map(label => {
return options.value.find(item => item.label === label)
})
}, 200)
emit("update:nameValue",valueName.value);
} else {
options.value = []
}
}
</script>
In parent component use:
// 这里的 dataList 就是我们请求过来的表格数据
<el-form :model="queryParams" ref="queryRef" :inline="true" v-show="showSearch">
<el-form-item label="责任单位" prop="sibleUnit">
<ElSelectV2 :isShowTip=" 'sibleUnit' " v-model="dataList" v-model:nameValue="queryParams.sibleUnit"></ElSelectV2>
</el-form-item>
<el-form-item label="证照类型代码" prop="typeCode">
<ElSelectV2 :isShowTip=" 'typeCode' " v-model="dataList" v-model:nameValue="queryParams.typeCode"></ElSelectV2>
</el-form-item>
</el-form>
<script setup>
import ElSelectV2 from "../../components/ElSelectV2/index.vue";
const data = reactive({
queryParams: {
pageNo: 1,
pageSize: 10,
sibleUnit: undefined,
typeCode: undefined
}
});
const {queryParams} = toRefs(data);
</script>

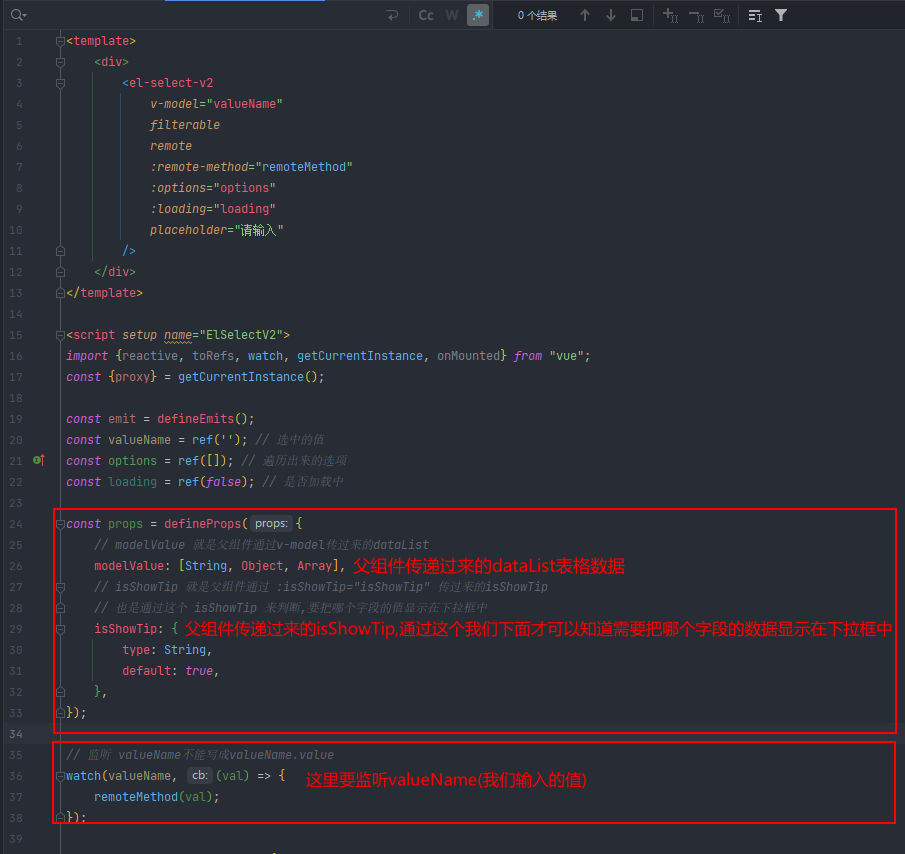
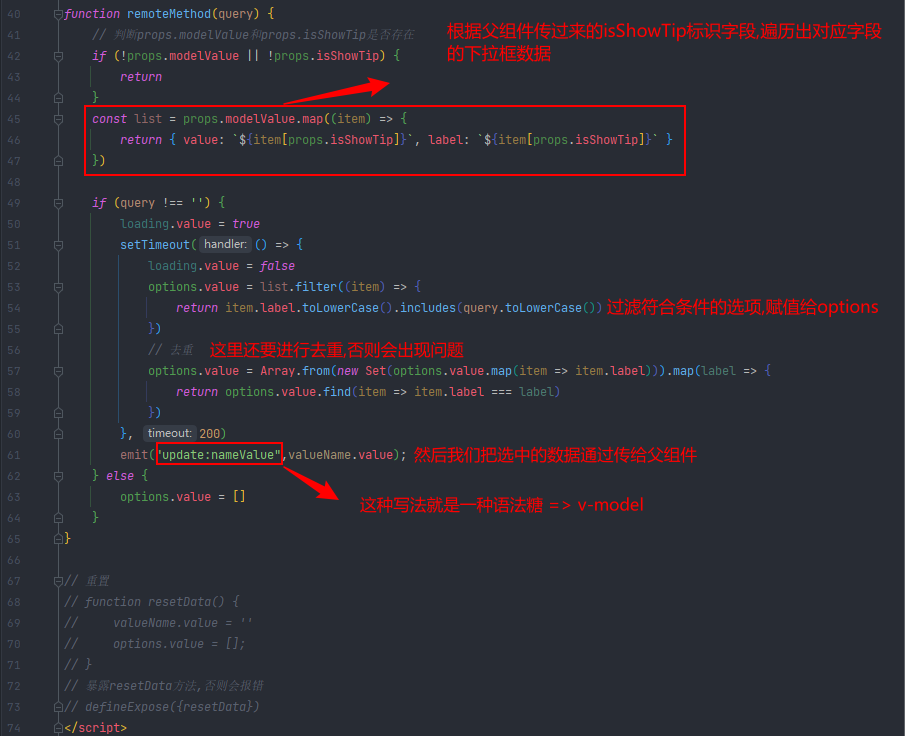
Click the reset button to refresh the subcomponent to achieve the effect of clearing the content

Here we can clear the subcomponent by refreshing the method
// 通key给子组件绑定一个timer变量,类型为number
<ElSelectV2 :key="timer" :isShowTip=" 'sibleUnit' " v-model:nameValue="queryParams.sibleUnit"></ElSelectV2>
<script setup name="certificationType">
const timer = ref(0);
/** 重置按钮操作 */
function resetQuery() {
// 把当前的时间戳赋值给timer,这样绑定子组件的key就会变化,从而达到刷新的目的
timer.value = new Date().getTime();
proxy.resetForm("queryRef");
handleQuery();
}
</script>
Error:

Unable to preventDefault inside passive event listener invocation.
This error is an error reported by Google browser when listening to touch events: Cannot passively listen to events preventDefault, which is an error reported by the new version of Chrome browser.
solve:
1. 处理函数时,用如下方式,明确声明为不是被动的
window.addEventListener('touchmove',func,{passive:false})
2. 应用css属性 touch-action: none; 这样任何触摸事件都不会产生默认行为,但是touch事件照样触发.
touch-action 还有很多选项
如果以上两个方法不生效,就用下面的
/*
查看你的项目中是否安装了 default-passive-events 依赖,安装了就卸载,然后在main.js中注释掉,
它默认情况下启用某些事件的被动侦听器,基本上每次声明新的事件监听器时,都会自动设置{passive:true}
*/
import 'default-passive-events'
The default-passive-events plugin is used to solve Google Chrome's method of eliminating Passive Event Listeners (passive event warnings).
But installing default-passive-events is to solve the warnings of Chrome 51 and later versions, which is a dilemma.
I didn't uninstall the default-passive-events dependency here, but just annotated it in main.js.
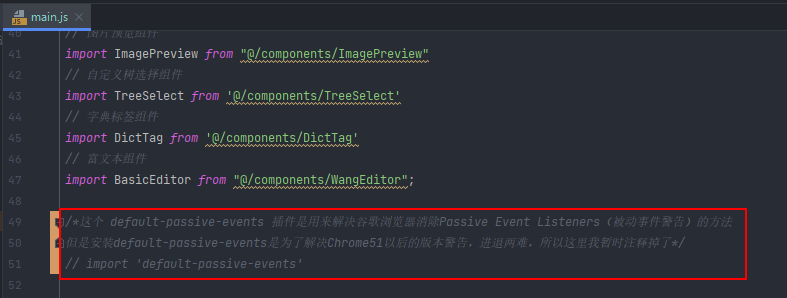