1.process.env is an environment in Node.js
Open the command line to view the environment:

2. process.env and Vue CLI project
Vue Cli has the following three modes of operation
development mode is used for vue-cli-service serve
The test mode is used for vue-cli-service test:unit
Production mode is used for vue-cli-service build and vue-cli-service test:e2e
We view the package.json configuration information and run the Vue CLI command:
The development environment runs npm run dev,
To run npm run build when the deployment server releases to production,
They just correspond to the development mode and production mode in Vue CLI, and the NODE_ENV in this mode will be loaded into it.
Now that we know which mode the project is running in, can we run different variable information in different modes, such as the development environment uses port 8080, and the online (production) environment uses port 80. At this time, you will think, I know Different modes configure different information, so which file is the information configured in?

1) You can place the following files in your project root directory to specify environment variables:
.env # 在所有的环境中被载入
.env.local # 在所有的环境中被载入,但会被 git 忽略
.env.[mode] # 只在指定的模式中被载入
.env.[mode].local # 只在指定的模式中被载入,但会被 git 忽略
there will be a sequence
An environment file contains only "key=value" pairs of environment variables:
#设置端口号
port=9999
# 开发环境配置
ENV = 'development'
Only NODE_ENV, BASE_URL and variables starting with VUE_APP_ will be statically embedded in the code on the client side via webpack.DefinePlugin
2) .env file configuration
.env : The global default configuration file, which will be loaded and merged regardless of the environment.
.env.development : Configuration file for development environment
.env.production : Configuration file for production environment
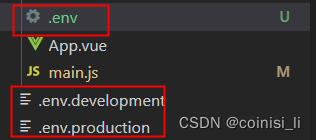
Note that the file name is fixed, do not customize it.
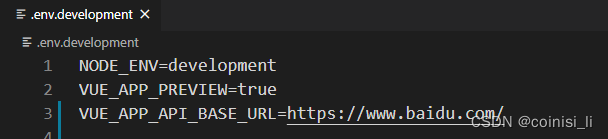
The attribute name must start with VUE_APP_, such as: VUE_APP_XXX
Vue will automatically load the corresponding environment configuration file according to the startup command. Vue is loaded according to the file name, so don't change it without authorization.
For example, executing the npm run serve command will automatically load the .env.development file
The development environment loads .env and .env.development.
The production environment loads .env and .env.production.
When running npm run serve, it mainly depends on what is followed by the --mode of the server attribute in package.json. If it is development, the .env.development file will be loaded.
vue-cli-service will read the env.development file by default;
vue-cli-service - -mode dev specifies to read the env.dev file;
Configure it in package.json, and use the development environment when executing serve. build is packaged for production or testing
package.json configuration items:
"scripts": {
"serve": "vue-cli-service serve --mode development",
"build": "vue-cli-service build",
"build:sit": "vue-cli-service build --mode production.sit",
"build:uat": "vue-cli-service build --mode production.uat",
"build:prod": "vue-cli-service build --mode production",
"lint": "vue-cli-service lint",
"et": "node_modules/.bin/et",
"et:init": "node_modules/.bin/et -i",
"et:list": "gulp themes"
}
Environment Profiles > Global Profiles
When the global configuration file and the environment configuration file have the same configuration items, the environment configuration items will override the global configuration items.
That is, the global configuration file .env file will override .env.development.
How the properties defined in the configuration file are accessed in other files:
Properties can be accessed using process.env.xxx
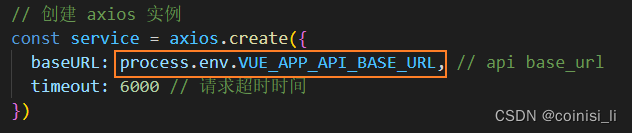
3. What is process.env used for?
Since our project needs to run in different environments (development, production, testing, etc.), this avoids us needing to switch the requested address and related configuration multiple times. Vue-cli2 can be configured directly in the config file , but vue-cli4 and vue-cli3 have been simplified, what if there is no config file?
1) Create .env series files
First, we create 3 new files in the root directory, namely .env.development, .env.production, .env.test
( Note that the file has only the suffix ).
The .env.development mode is used for serve, development environment, that is, the configuration in this file will be referenced when starting the environment.
The env.production mode is used for build and online environment.
.env.test test environment
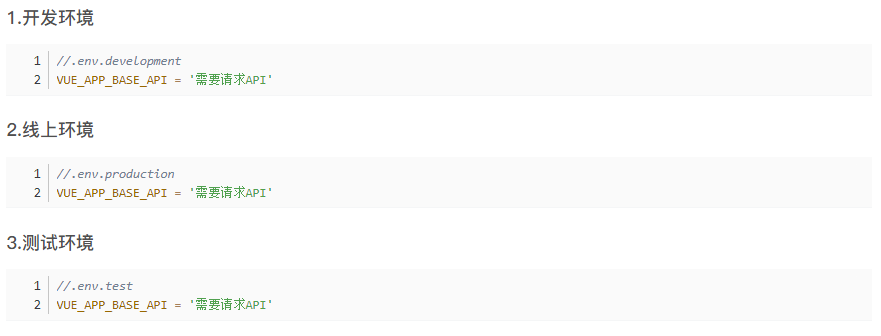
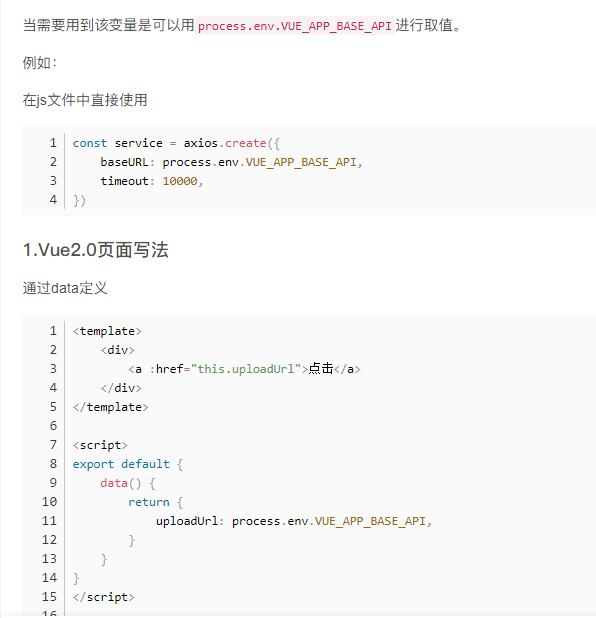
4. Development mode operation
Create .env.development in the project root directory

.env.development file content
# 开发环境配置
ENV = 'development'
#设置端口号
port=8081
# 前端请求路径
VUE_APP_BASE_API = '/api'
#后端服务器地址不要忘记添加http或https
BASE_URL_REAR='http://127.0.0.1:8080/'
The configuration of vue.config.js is as follows
process.env The configuration environment currently running, used to read the parameters of the corresponding configuration file
//提示:process.env 是Node.js 中的一个环境,Vue CLI 项目中一个重要的概念
//:有以下三种运行模式
// development 模式用于 vue-cli-service serve
// test 模式用于 vue-cli-service test:unit
// production 模式用于 vue-cli-service build 和 vue-cli-service test:e2e
//当配置中有,配置port端口则使用配置的端口反之使用80端口
const port = process.env.port || 80 // 端口
// vue.config.js 配置说明
//官方vue.config.js 参考文档 https://cli.vuejs.org/zh/config/#css-loaderoptions
// 这里只列一部分,具体配置参考文档
module.exports = {
//build后的输出目录
outputDir: 'dist',
publicPath: process.env.NODE_ENV === 'production' ? '/' : '/',
lintOnSave: false,
configureWebpack: {
// devtool 添加这个debugger可以看源码
devtool: 'cheap-module-source-map'
},
// webpack-dev-server 相关配置
devServer: {
//本机地址
host: 'localhost',
//获取port端口
port: port,
open: true,
//如果你的前端应用和后端 API 服务器没有运行在同一个主机上,你需要在开发环境下将 API 请求代理到 API 服务器
proxy: {
// detail: https://cli.vuejs.org/config/#devserver-proxy
//process.env.VUE_APP_BASE_API的参数会读取对应环境变量数据,如读取的数据是‘/api’,那么意思就是前端请求的/api路径都会被带来到,参数target的地址
[process.env.VUE_APP_BASE_API]: {
//代理的路径
target: process.env.BASE_URL_REAR,
changeOrigin: true,
pathRewrite: {
['^' + process.env.VUE_APP_BASE_API]: ''
}
}
},
disableHostCheck: true
},
};
以上配置主要是为了,前端应用和后端 API 服务器没有运行在同一个主机上需要做代理为例。
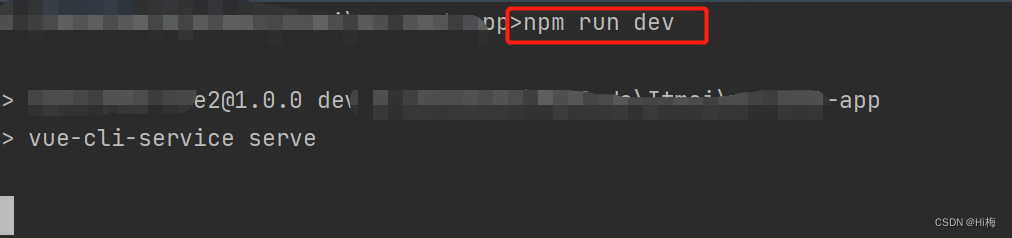
运行 npm run dev 使用到的配置文件为.env.development
配置文件的端口,请求路径,后端服务器地址配置等
网页可以看出端口
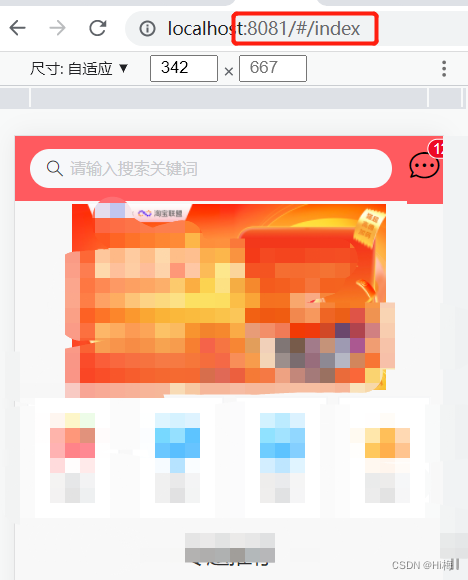
可能请求接口也拼接了配置路径中的VUE_APP_BASE_API变量数据
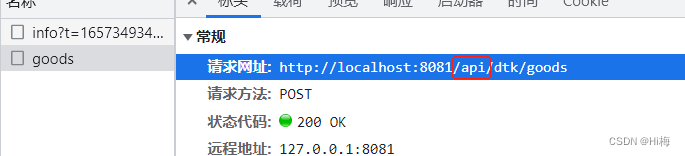
5.定义自己配置环境
因为我们知道了配置环境的定义,那么只要我们遵循规则定义也可以配置自己的环境数据
.env # 在所有的环境中被载入
.env.local # 在所有的环境中被载入,但会被 git 忽略
.env.[mode] # 只在指定的模式中被载入
.env.[mode].local # 只在指定的模式中被载入,但会被 git 忽略
我们在根路径添加配置.env.itmei配置如下
# 自己定义的开发环境配置
ENV = 'itmei'
#设置端口号
port=8088
# 前端请求路径
VUE_APP_BASE_API = '/itmei-api'
#后端服务器地址
BASE_URL_REAR='http://127.0.0.1:8080/'
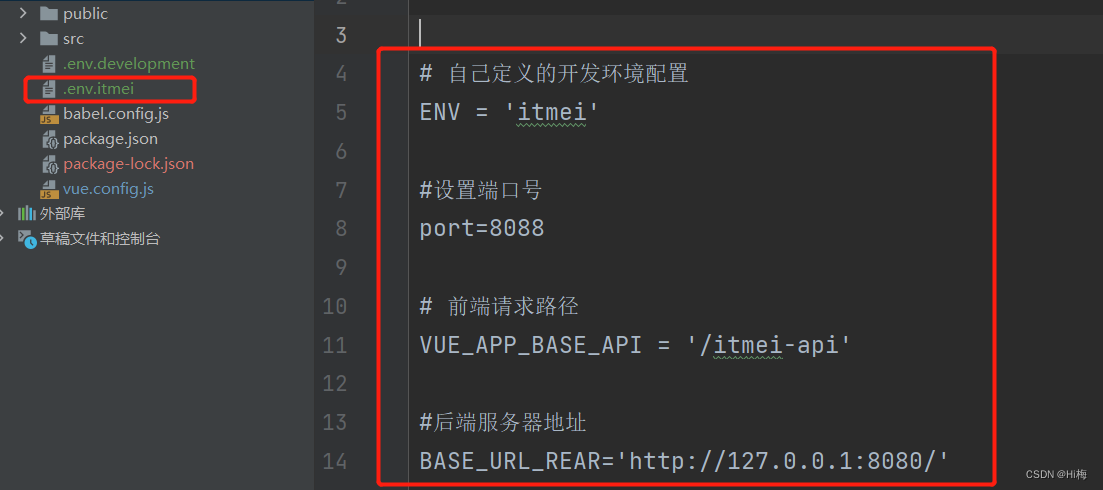
添加完成后只需要在运行命令添加--mode 配置文件.env.后面自己定义的名称
如我自己配置的.env.itmei配置名称的itmei,为了方便我们在package.json中配置运行脚本
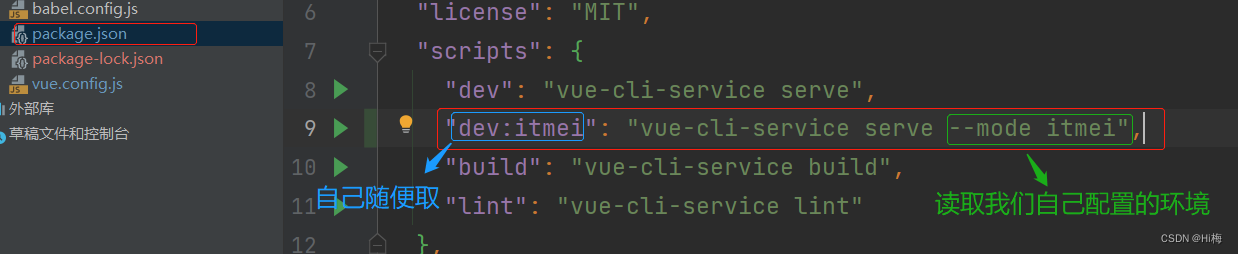
配置了上面的就可以使用npm命令运行

当然也可以直接使用Vue Cli命令运行vue-cli-service serve --mode itmei

首先会去找配置文件.env.itmei、.env.itmei.local 和 .env文件然后构建出生产环境应用
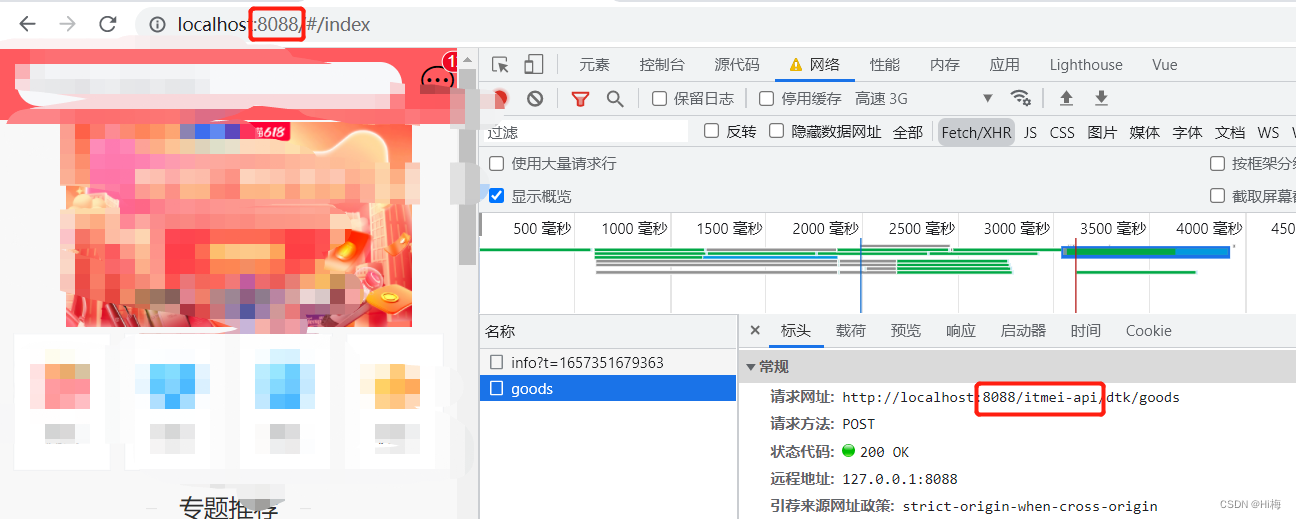
如果此时运行VueCli命令mode 的环境名称不存在,首先会去找.env.itmei00或.env.itmei00.local 或.env如找到就能正常运行找不到就报错,由于我定义了.env所以后面就使用.env的配置信息
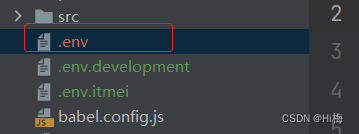

当我把.env删除,找不到会报
building 2/2 modules 0 active ERROR TypeError: Cannot read property 'upgrade' of undefined
TypeError: Cannot read property 'upgrade' of undefined

参考自:https://blog.csdn.net/qq_45502336/article/details/125693697