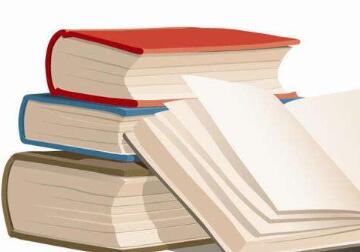
Reposted from: Micro reading https://www.weidianyuedu.com
1. Swap two numbers in place
Python provides an intuitive method of assigning and exchanging (variable values) in one line of code, see the example below:
x, y = 10, 20
print(x, y)
x, y = y, x
print(x, y)
#1 (10, 20)
#2 (20, 10)
The right-hand side of the assignment forms a new tuple, and the left-hand side immediately unpacks (unpacks) that (unreferenced) tuple into the variables <a> and <b>.
Once the assignment is complete, the new tuple becomes unreferenced and marked for garbage collection , eventually completing the variable swap.
2. Chained comparison operators
Aggregation of comparison operators is another sometimes handy trick:
n = 10
result = 1 < n < 20
print(result)
# True
result = 1 > n <= 9
print(result)
# False
3. Use the ternary operator for conditional assignment
The ternary operator is a shortcut for the if-else statement, or conditional operator:
[return value if expression is true] if [expression] else [return value if expression is false]
Here are a few examples that you can use to make your code compact and concise. The following statement says "if y is 9, assign x the value 10, otherwise assign the value 20". We can also extend this chain of operations if necessary.
x = 10 if (y == 9) else 20
Similarly, we can do this with classes:
x = (classA if y == 1 else classB)(param1, param2)
In the above example, classA and classB are two classes, and the constructor of one of the classes will be called.
Here's another example of multiple conditional expressions chained together to compute a minimum:
def small(a, b, c):
return a if a <= b and a <= c else (b if b <= a and b <= c else c)
print(small(1, 0, 1))
print(small(1, 2, 2))
print(small(2, 2, 3))
print(small(5, 4, 3))
#Output
#0 #1 #2 #3
We can even use the ternary operator in list comprehensions :
[m**2 if m > 10 else m**4 for m in range(50)]
#=> [0, 1, 16, 81, 256, 625, 1296, 2401, 4096, 6561, 10000, 121, 144, 169, 196, 225, 256, 289, 324, 361, 400, 441, 484, 529, 576, 625, 676, 729, 784, 841, 900, 961, 1024, 1089, 1156, 1225, 1296, 1369, 1444, 1521, 1600, 1681, 1764, 1849, 1936, 2025, 2116, 2209, 2304, 2401]
4. Multi-line strings
The basic way is to use backslashes from the C language:
multiStr = "select * from multi_row
where row_id < 5"
print(multiStr)
# select * from multi_row where row_id < 5
Another trick is to use triple quotes:
multiStr = """select * from multi_row
where row_id < 5"""
print(multiStr)
#select * from multi_row
#where row_id < 5
The common problem with the above methods is the lack of proper indentation, if we try to indent it will insert spaces in the string. So the final solution is to split the string into multiple lines and enclose the whole string in parentheses:
multiStr= ("select * from multi_row "
"where row_id < 5 "
"order by age")
print(multiStr)
#select * from multi_row where row_id < 5 order by age
5. Store list elements into new variables
We can use a list to initialize multiple variables. When parsing a list, the number of variables should not exceed the number of elements in the list:
testList = [1,2,3]
x, y, z = testList
print(x, y, z)
#-> 1 2 3
6. Print the file path of the imported module
If you want to know the absolute path referenced to a module in your code, you can use the following trick:
import threading
import socket
print(threading)
print(socket)
#1- <module "threading" from "/usr/lib/python2.7/threading.py">
#2- <module "socket" from "/usr/lib/python2.7/socket.py">
7. "_" operator in interactive environment
This is a useful feature that most of us are unaware of. In the Python console, whenever we test an expression or call a method, the result is assigned to a temporary variable: _ (an underscore).
>>> 2 1
3
>>> _
3
>>> print _
3
"_" is the output of the last executed expression.
8. Dictionary/Set comprehension
Similar to the list comprehensions we use, we can also use dictionary/set comprehensions, they are simple and effective to use, here is an example:
testDict = {i: i * i for i in xrange(10)}
testSet = {i * 2 for i in xrange(10)}
print(testSet)
print(testDict)
#set([0, 2, 4, 6, 8, 10, 12, 14, 16, 18])
#{0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
Note: There is only one <:> difference between the two statements. In addition, when running the above code in Python3, change <xrange> to <range>.
9. Debug script
We can set breakpoints in Python scripts with the help of <pdb> module, here is an example −
import pdb
pdb.set_trace()
We can specify <pdb.set_trace()> anywhere in the script and set a breakpoint there, quite easily.
10. Enable file sharing
Python allows running an HTTP server to share files from the root path, the command to start the server is as follows:
# Python 2
python -m SimpleHTTPServer
# Python 3
python3 -m http.server
The above command will start a server on the default port 8000, you can pass a custom port number to the above command as the last parameter.
11. Inspecting objects in Python
We can inspect objects in Python by calling the dir() method, here is a simple example:
test = [1, 3, 5, 7]
print( dir(test) )
["__add__", "__class__", "__contains__", "__delattr__", "__delitem__", "__delslice__", "__doc__", "__eq__", "__format__", "__ge__", "__getattribute__", "__getitem__", "__getslice__", "__gt__", "__hash__", "__iadd__", "__imul__", "__init__", "__iter__", "__le__", "__len__", "__lt__", "__mul__", "__ne__", "__new__", "__reduce__", "__reduce_ex__", "__repr__", "__reversed__", "__rmul__", "__setattr__", "__setitem__", "__setslice__", "__sizeof__", "__str__", "__subclasshook__", "append", "count", "extend", "index", "insert", "pop", "remove", "reverse", "sort"]