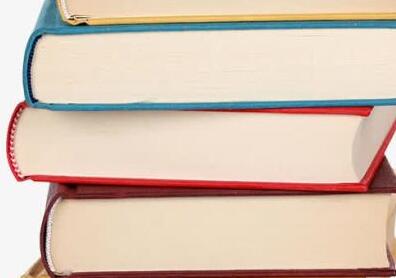
Reposted from: Micro reading https://www.weidianyuedu.com
We often write HTML, CSS, and JavaScript. After writing these, we will see the page in the browser. So what does the browser do behind the scenes? This article will reveal the answer!
Understanding the rendering principle of the browser is indispensable in our front-end development leading to a deeper level. It allows us to consider performance optimization from a deeper level and perspective~
Enter the text below~
process, thread
The browser will assign a thread to parse and render the code sequentially "from top to bottom, from left to right", so what are processes and threads, and what is the relationship between them?
process
A process is an instance of a program running. When starting a program, the operating system will create a block of memory for the program to store code, running data, and a main thread for executing tasks. Such an operating environment is called a process
thread
A thread cannot exist alone, it is started and managed by a process. Threads are attached to processes, and the use of multi-threaded parallel processing in processes can improve computing efficiency
relationship between the two
1. Any thread execution error in the process will cause the entire process to crash
2. Data can be shared between threads
3. When a process is closed, the operating system will reclaim the memory occupied by the process
4. The content between processes is isolated from each other
rendering mechanism
Start with HTML, CSS, and JavaScript
To understand the rendering principle of the browser, we must start by understanding HTML, CSS and JavaScript. Let's first look at a picture
HTML (Hypertext Markup Language), as the name suggests, consists of tags (labels) and text, each tag has its own semantics, and the browser will display the corresponding content according to the tags and text.
CSS (Cascading Style Sheets ), consisting of selectors and attributes, can change the style of HTML. For example, in the above figure, we changed the color of the span from blue to green.
JavaScript, we can accomplish many things through JS, such as modifying the style in the above picture.
Let's start to analyze the principle of rendering
rendering pipeline
Due to the complexity of the rendering mechanism, the rendering module is divided into many sub-stages. The input HTML passes through these sub-stages and is finally output as pixels. Such a processing flow is called the rendering pipeline.
In the chronological order of rendering, the pipeline can be divided into several sub-stages: building DOM tree, style calculation, layout stage, layering, drawing, tiling, rasterization and compositing
Build the DOM tree
Since browsers cannot directly understand and use HTML, it is necessary to convert HTML into a structure that browsers can understand (DOM tree)
Tree Structure Diagram
The construction process of the DOM tree
Let's analyze what kind of DOM tree will be built by the following code
Let's run the above code first, and then enter document in the browser console to see what effect it will have
When we open layer by layer, we will see the effect shown in the figure above. We can draw a DOM tree structure according to the effect of each layer, as follows:
Next, let's try to use JS to modify the content to see what changes:
We can see that the text of "browser" has changed to "chrome"
Let's see if the DOM tree has changed
We see that "browser" is replaced by "chrome", so how to make DOM nodes have styles?
style calculation
Style calculation, as the name suggests, is to calculate the specific style of each element in the DOM node. This stage will be divided into three parts:
Convert CSS to a structure that browsers can understand
Transform attribute values in stylesheets to normalize them
Calculate the style of each node in the DOM tree
CSS style source
link Import external style resources
The browser will create a new thread to go to the server to obtain the corresponding resource file (without hindering the rendering of the main thread)
style inline style
Parse from top to bottom, and continue to parse the DOM structure after parsing. In real projects, if there are not many css codes, or mobile projects, we should use inline to reduce http resource requests and improve page rendering speed
inline style
@import import
It is synchronous, it will not open up a new thread to load the resource file, but let the main thread get it, which hinders the continuous rendering of the DOM structure; only after the external style is imported and parsed, the DOM structure will continue to be rendered
Convert CSS to a structure that browsers can understand
Browsers don't understand CSS just like they can't understand HTML, so when the rendering engine receives a CSS file, it will perform a conversion operation to convert the CSS text into a styleSheets structure that the browser can understand.
In HTML, type document in your browser to view the structure of the html. In css, you can enter document.styleSheets to see the structure of css
The current structure is empty, let's add some styles to see the effect
Transform attribute values in stylesheets to normalize them
Normalization of attribute values is to convert all values into standardized calculation values that are easy to understand for the rendering engine. Let's take a look at the effect:
before standardization
body { font-size: 2em; color: black; font-weight: bold; ...}
After normalization
body { font-size: 16px; color: rgb(0, 0, 0); font-weight: 700; ...} Copy the code to calculate the specific style of each node in the DOM tree
Style calculation has two CSS rules: inheritance rules and cascading rules
CSS Inheritance Rules
CSS inheritance means that each DOM node contains the styles of the parent node. Let's take a look at how the following code is applied to DOM nodes
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <title>Document</title> <style> h1 { color: red; } div { color: blue; } span { font-size: 16px; } </style></head><body> <h1>Nuggets</h1> <div> <span>Browser</span> <span>Rendering principle</span > Build DOM tree</div></body></html>Copy code
The child node will have the style of the parent node, so we can draw such a picture
We can also open the console and see what will be seen when the span tag is selected
Through the above figure, we can see the style, inheritance process, etc. of an element. The userAgent style is the default built-in style of the browser. If we do not provide any style, this style will be used.
Style cascading rules
Cascading is at the heart of CSS, a fundamental feature of CSS that defines algorithms for how to combine property values from multiple sources.
The final output of the style calculation stage is the style of each DOM node, which is stored in ComputedStyle. We can see the final calculation style of a DOM element through the console
layout stage
Now we don't know the geometric position information of DOM elements, so now we need to calculate the geometric position of visible elements in the DOM tree, this calculation process is called layout. The layout phase has two processes:
Create a layout tree
layout calculation
Create a layout tree
Creating a layout tree means creating a tree that contains only visible elements. Let's look at the process of creating a layout tree in the following piece of code
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <title>Document</title> <style> h1 { color: red; } div { color: blue; } div span { font-size: 16px; } div span:last-child { display: none; } </style></head><body> <h1>Nuggets</h1> <div> <span>Browse Device</span> <span>Rendering principle</span> Build a DOM tree</div></body></html>Copy code
During the process of building the layout tree, all invisible nodes in the DOM tree will not be included in this tree. The browser will traverse all visible nodes in the DOM tree, and then add these nodes to the layout; invisible nodes will be ignored, and the content under the head tag and the last span node under the div will not be in the layout tree , let's take a look at this process diagram to feel~
layout calculation
Layout calculation is to calculate the coordinate position of the layout tree node. This calculation process is extremely complicated.
layered
The rendering engine will generate a dedicated layer for a specific node and generate a corresponding layer tree. This is because the page may contain many complex effects, we can open the console to see the layering of the page
We can see that the rendering engine divides the page into many layers, and these layers will be superimposed in a certain order to form the final page
So what are the sources of layers?
1. Elements with stacking context attributes will be promoted to a separate layer
The cascading context can make HTML elements have a three-dimensional concept, and these HTML elements are distributed on the z-axis perpendicular to this two-dimensional plane according to the priority of their own attributes. Which elements have the stacking context attribute?
2. The place that needs to be clipped will be created as a layer
When we create a div with width and height, the text content inside may exceed this area. At this time, the rendering engine will use part of the cropped text content to display in the div area, for example
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> < title>Document</title> <style> div { width: 100px; height: 100px; background: yellow; overflow: auto; } </style></head><body> <div> We often write `HTML`, `CSS` and `JavaScript`, after writing these, we will see the page in the browser, so what exactly does the browser do behind this? This article will reveal the answer! Understanding the rendering principle of the browser is indispensable in our front-end development leading to a deeper level. It allows us to consider performance optimization from a deeper level and perspective~ </div></body></html> copy code
operation result
Let's open the layers of the console again to see the effect
You can see that the rendering engine has created a separate layer for the text part.
If a node in the layout tree has a corresponding layer, this node is a layer, if not, this node belongs to the layer of the parent node, as shown in the following figure:
layer drawing
After the layer tree is created, the rendering engine will draw each layer in the layer tree. The rendering engine will decompose layer drawing into many small drawing instructions, and then compose these instructions in order to form a list to be drawn. We can open the layers of the console, select the document layer, and see the effect
rasterization operation
Rasterization is to convert a tile into a bitmap, and a tile is the smallest unit for rasterization. The rendering process maintains a rasterization thread pool, and the rasterization of all tiles is performed in the thread pool.
After the layer drawing list is ready, the main thread will submit the drawing list to the composition thread, and the drawing operation is completed by the composition thread in the rendering engine.
The compositing thread divides the layer into tiles, and then the compositing thread prioritizes generating bitmaps in tiles near the viewport (visible area).
Composite and Display
After all the tiles are rasterized, the compositing thread generates a command to draw the tiles ( DrawQuad ), which is then submitted to the browser process. The viz component in the browser process is used to receive the DrawQuad command, draw its page content into the memory, and finally display the memory to the screen. At this time, we see the page