When DIYing a robot, we can use a remote connection to the robot to view relevant information, that is, the aforementioned remote connection VNC-Viewer and secure transmission of WinSCP software , connected through VNC-Viewer, but for those who are inconvenient to use a computer or want to be simple and intuitive See the common attributes of the robot, such as IP address, memory and CPU, etc., generally use a small display to display directly.
What we use here is a 128*32 resolution OLED display, which can display IP address, memory, CPU, hard disk size, etc. This information is enough for us.
If you want to display information on the display, you need to use a driver. Here we use the Adafruit_Python_SSD1306 driver. As the name suggests, the driver of the OLED screen is called SSD1306 , which is a very common OLED driver. Open source code: https://github.com/adafruit/Adafruit_Python_SSD1306/
We open https://github.com/adafruit/Adafruit_Python_SSD1306/blob/master/Adafruit_SSD1306/SSD1306.py
SSD1306_I2C_ADDRESS = 0x3C # 011110+SA0+RW - 0x3C or 0x3D
It can be known that the I2C peripheral address of OLED is 0x3C , which stores all the methods and register information about driving the screen.
Linux commands
Before this, in order to avoid the stats.py program run by jetbotmini_stats.service, which is enabled by Jetbotmini for real-time refresh display, and the program we are testing now, both use an I2C peripheral OLED screen at the same time, resulting in an abnormal conflict
We first stop the operation of refreshing the OLED service in the Jetbotmini command console: sudo systemctl stop jetbotmini_stats
Memory, CPU and hard disk conditions use Linux commands
Here I run the test directly in wsl to format:
The content of f.sh is as follows. Of course, it is also possible to execute a single command, just like the batch processing in Windows:
free -m | awk 'NR==2{printf "Memory Usage: %s/%sMB (%.2f%%)\n", $3,$2,$3*100/$2 }'
df -h | awk '$NF=="/"{printf "Disk Usage: %d/%dGB (%s)\n", $3,$2,$5}'
top -bn1 | grep load | awk '{printf "CPU Load: %.2f\n", $(NF-2)}'
Execute to see the effect (usage and proportion of memory, hard disk, and CPU load):
(base) tony@TonyComputer:/mnt/c/Users/Tony$ ./f.sh
Memory Usage: 65/12747MB (0.51%)
Disk Usage: 4/251GB (2%)
CPU Load: 0.00
Of course, you can also execute commands on the jetbotmini platform, that is, on the Ubuntu system
New file: vi f.sh
After copying and pasting : wq press Enter to save, and then execute directly: ./f.sh
If you get a permission error: bash: ./f.sh: Permission denied
Modify file permissions: chmod 777 f.sh
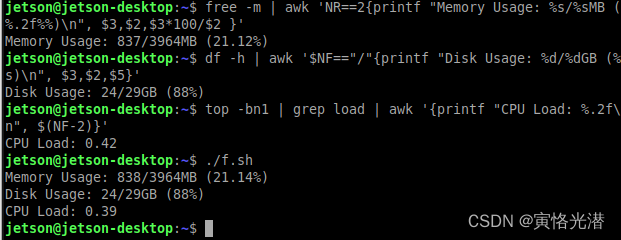
Then we will display the memory and other information on the OLED display in real time, first import the required modules
import time
import Adafruit_SSD1306
from PIL import Image
from PIL import ImageDraw
from PIL import ImageFont
from jetbotmini.utils.utils import get_ip_address
import subprocess
SSD1306 driver
Initialize the driver SSD1306, clear the screen, set fonts, etc.
# 128x32 显示与硬件I2C:
disp = Adafruit_SSD1306.SSD1306_128_32(rst=None, i2c_bus=0, gpio=1)# 将gpio设置hack为1,以避免平台检测
# 初始化库。
disp.begin()
# 清除显示
disp.clear()
disp.display()
# 为绘图创建空白图像
# 确保创建带有模式为'1'即1位颜色的图像
width = disp.width
height = disp.height
image = Image.new('1', (width, height))
# 获取要在图像上绘制的绘图对象
draw = ImageDraw.Draw(image)
# 画一个黑色填充框来清除图像
draw.rectangle((0,0,width,height), outline=0, fill=0)
# 画一些形状
# 首先定义一些常量,以方便调整形状的大小
padding = -2
top = padding
# 从左到右移动,以跟踪绘图图形的当前x位置。
x = 0
# 加载默认字体
font = ImageFont.load_default()
You can check the driver SSD1306 to get familiar with the attributes and methods:
print(dir(disp))
#['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', '_buffer', '_gpio', '_i2c', '_initialize', '_log', '_pages', '_rst', '_spi', 'begin', 'clear', 'command', 'data', 'dim', 'display', 'height', 'image', 'reset', 'set_contrast', 'width']
Then, the information is refreshed and displayed on the OLED screen every second.
while True:
# 画一个黑色填充框来清除图像。
draw.rectangle((0,0,width,height), outline=0, fill=0)
# 从这个链接可以获取系统监视的Shell脚本 :
#https://unix.stackexchange.com/questions/119126/command-to-display-memory-usage-disk-usage-and-cpu-load
cmd = "top -bn1 | grep load | awk '{printf \"CPU Load: %.2f\", $(NF-2)}'"
CPU = subprocess.check_output(cmd, shell = True )
cmd = "free -m | awk 'NR==2{printf \"Mem:%s/%sM %.2f%%\", $3,$2,$3*100/$2 }'"
MemUsage = subprocess.check_output(cmd, shell = True )
cmd = "df -h | awk '$NF==\"/\"{printf \"Disk:%d/%dGB %s\", $3,$2,$5}'"
Disk = subprocess.check_output(cmd, shell = True )
draw.text((x, top), "eth0:" + str(get_ip_address('eth0')), font=font, fill=255)
draw.text((x, top+8), "wlan0:" + str(get_ip_address('wlan0')), font=font, fill=255)
draw.text((x, top+16), str(MemUsage.decode('utf-8')), font=font, fill=255)
draw.text((x, top+25), str(Disk.decode('utf-8')), font=font, fill=255)
# 显示图像
disp.image(image)
disp.display()
time.sleep(1)
The figure below is the interface of Jetbotmini usage information displayed by OLED
Clear the screen --> real-time display
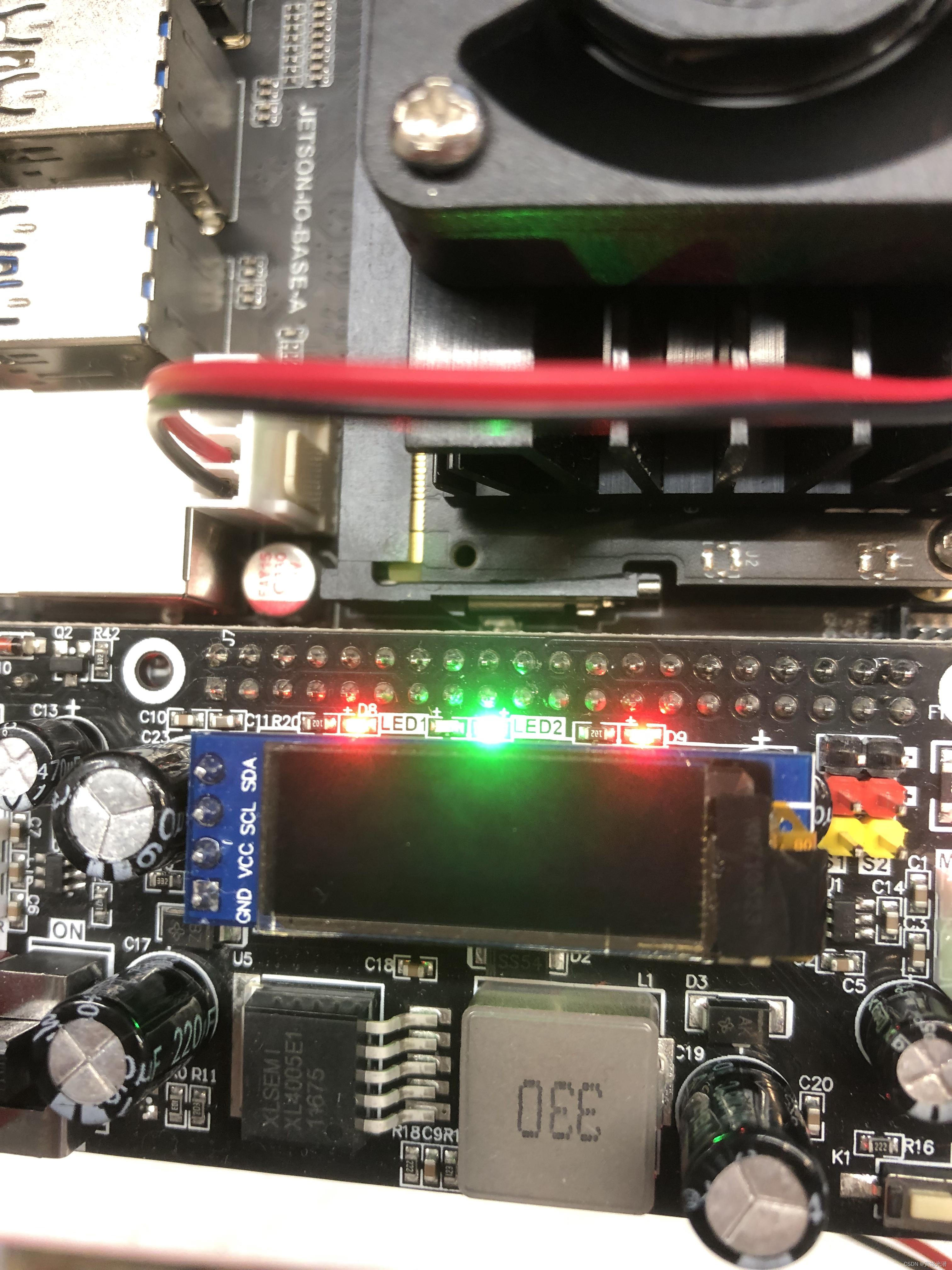
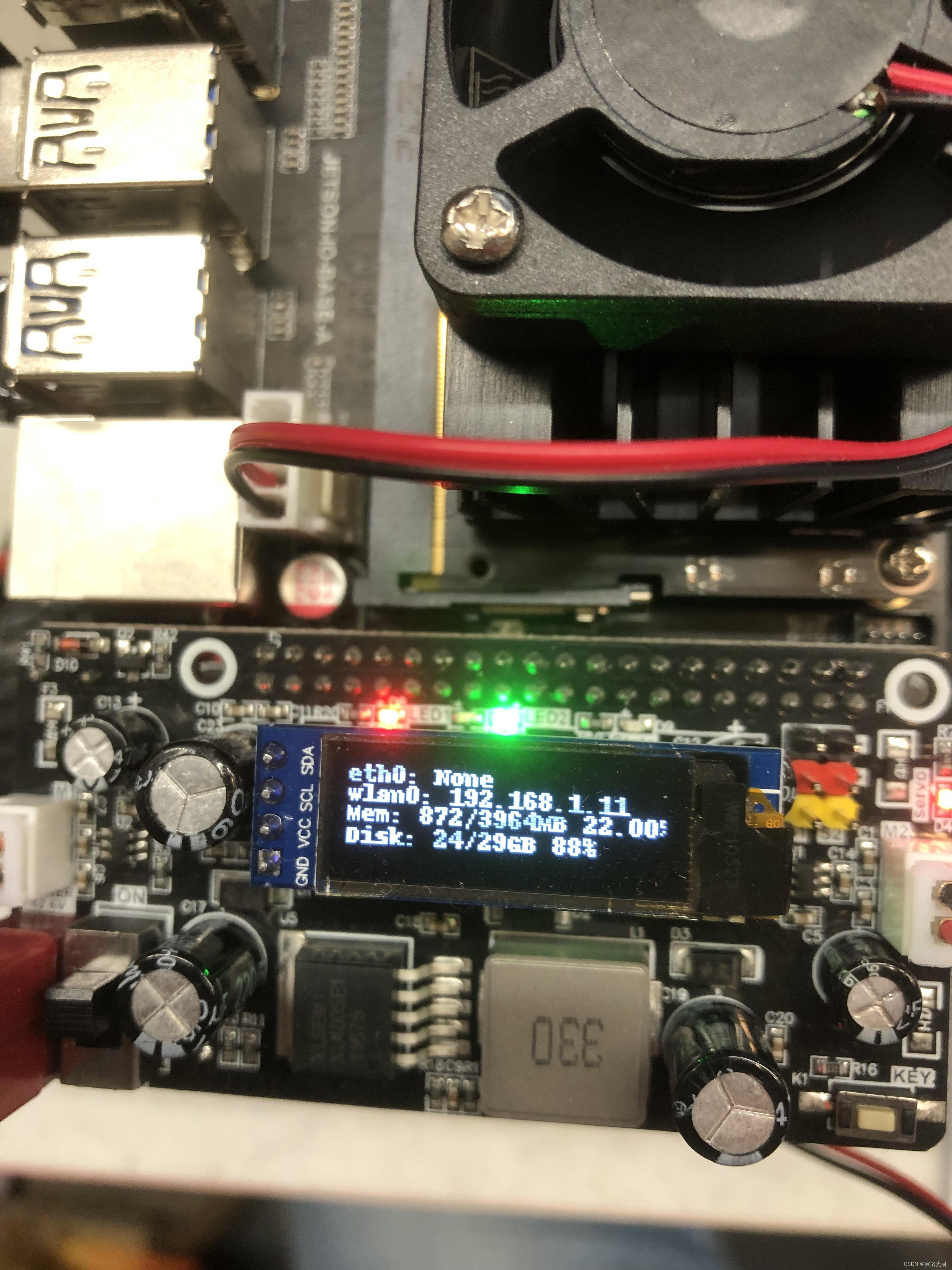
1. 第一行内容:有线网络的IP地址,这里我使用无线连接的,所以是None
2. 第二行内容:WiFi无线网络的IP地址
3. 第三行内容:运行内存使用情况与已用运行内存百分比
4. 第四行内容:磁盘内存使用情况与已用磁盘内存百分比