64. Minimum Path Sum** (minimum path sum)
https://leetcode.com/problems/minimum-path-sum/
topic description
Given a m x n
grid filled with non-negative numbers, find a path from top left to bottom right which minimizes the sum of all numbers along its path.
Note: You can only move either down or right at any point in time.
Example:
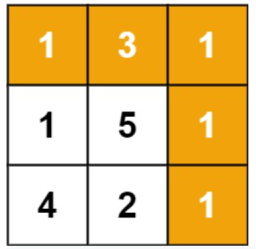
Input:
[
[1,3,1],
[1,5,1],
[4,2,1]
]
Output: 7
Explanation: Because the path 1→3→1→1→1 minimizes the sum.
Code
It has already been explained in 64. Minimum Path Sum** , but it is supplemented here with richer details, and it is specifically classified into the "Dynamic Programming" category.
This question requires the minimum path sum when starting from the upper left corner and reaching the lower right corner. To write the state transition equation, the state must first be defined. Obviously, the transition state is the position of movement. Since grid
is dp
array , where dp[i][j]
represents grid[i][j]
the minimum path sum when reaching . To arrive grid[i][j]
, either arrive grid[i][j]
from above (ie grid[i - 1][j]
) or from the left grid[i][j]
of (ie grid[i][j - 1]
), so we can quickly write the state transition equation:
dp[i][j] = min(dp[i - 1][j], dp[i][j - 1]) + grid[i][j]
After writing the state transition equation, the next step is to consider the initial situation and the boundary problem.
In order to facilitate the handling of boundary conditions, dp
let the size be (m + 1) x (n + 1)
, and initialize to be INT32_MAX
, but at the same time let dp[0][1] = 0
, as shown below:
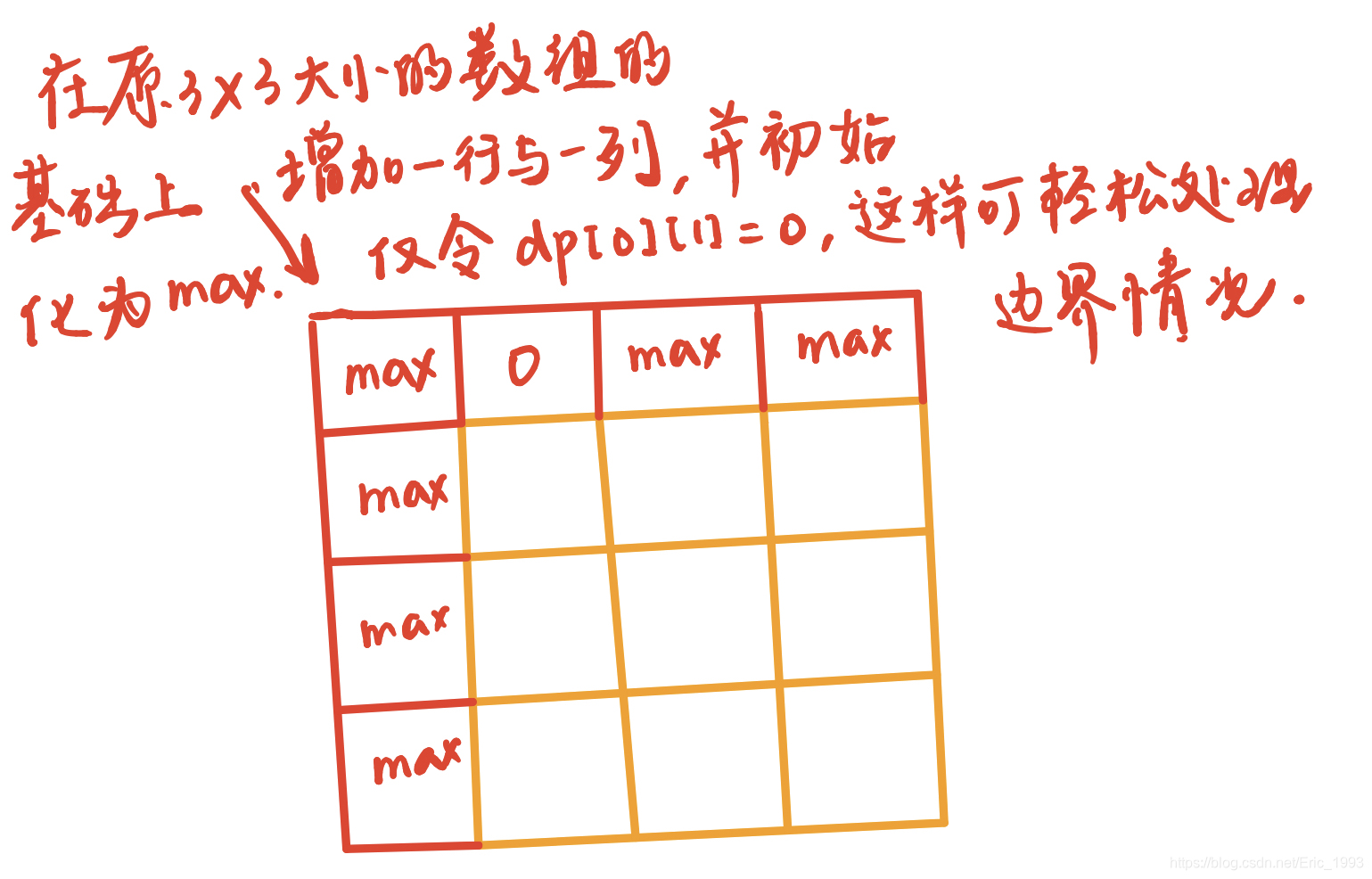
It is equivalent to adding a row and a column on the outside. In this dp[1][...]
way dp[...][1]
, the boundary conditions of and can be handled correctly. In addition, since at this time dp[i + 1][j + 1]
represents grid[i][j]
state of , then a simple modification is made to the above state transition equation:
dp[i + 1][j + 1] = min(dp[i][j + 1], dp[i + 1][j]) + grid[i][j]
The code is implemented as follows:
class Solution {
public:
int minPathSum(vector<vector<int>>& grid) {
int m = grid.size(), n = grid[0].size();
vector<vector<int>> dp(m + 1, vector<int>(n + 1, INT32_MAX));
dp[0][1] = 0;
for (int i = 0; i < m; ++ i) {
for (int j = 0; j < n; ++ j) {
dp[i + 1][j + 1] = min(dp[i][j + 1], dp[i + 1][j]) + grid[i][j];
}
}
return dp[m][n];
}
};
In addition, I wrote an article before, and the code writing method and ideas are as follows:
Dynamic programming. Use dp[i][j]
to represent grid[i][j]
the minimum cost required to reach . (Take all elements grid
in as the cost required for walking). For convenience, dp
the size of is set here (m + 1) x (n + 1)
. The boundary case is handled in for
the loop , and i == 1 && j == 1
the corresponding dp
value is specially set.
class Solution {
public:
int minPathSum(vector<vector<int>>& grid) {
if (grid.empty() || grid[0].empty()) return 0;
int m = grid.size(), n = grid[0].size();
vector<vector<int>> dp(m + 1, vector<int>(n + 1, INT32_MAX));
for (int i = 1; i <= m; ++ i) {
for (int j = 1; j <= n; ++ j) {
if (i == 1 && j == 1) dp[i][j] = grid[i - 1][j - 1];
else dp[i][j] = grid[i - 1][j - 1] + std::min(dp[i - 1][j], dp[i][j - 1]);
}
}
return dp[m][n];
}
};