mock a login example
install mockjs
npm install mockjs --save-dev
First create two files index.js and userList.js under the mock package
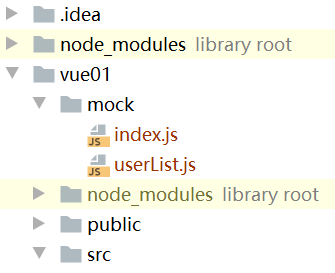
Then add it to the global main.js
require('../mock')
In index.js write
// 首先引入Mock
const Mock = require('mockjs');
import userList from './userList'
// 设置拦截ajax请求的相应时间
Mock.setup({
timeout: '200-600'
});
let configArray = [];
// 使用webpack的require.context()遍历所有mock文件
const files = require.context('.', true, /\.js$/);
files.keys().forEach((key) => {
if (key === './index.js') return;
configArray = configArray.concat(files(key).default);
});
// 注册所有的mock服务
configArray.forEach((item) => {
for (let [path, target] of Object.entries(item)) {
let protocol = path.split('|');
Mock.mock(new RegExp('^' + protocol[1]), protocol[0], target);
}
});
In userList.js write
import Mock from 'mockjs' //导入mockjs
const userList = { //定义用户数据
data: {
total: 6,
//前两个用户数据分别固定设为管理员和普通用户,为后续权限设置做准备,其他用户随机生成
userinfo: [{
username: 'dza6196172',
password: '123456',
roles: 'admin',
name: '张三',
age: 23,
job: '前端工程师',
token: '000111222333444555666',
id: '100',
}, {
username: '123',
password: '123123',
roles: 'editor',
name: '测试1',
'age|20-30': 23,
job: '前端工程师',
token: '145145145123123123111',
id: '101',
}, {
username: '@word(3, 5)',
password: '123456',
roles: 'editor',
name: '@cname',
'age|20-30': 23,
'job|1': ['前端工程师', '后端工程师', 'UI工程师', '需求工程师'],
token: '@guid()',
id: '102',
}, ],
meta: {
status: 200,
message: 'success',
}
},
};
//定义请求方法与路径
export default {
'get|/user': userList,
}
Then find the login button on the login page, bind the following click event, and perform a request test
async getuser(){
const {data:res} = await this.$http.get('/user')
console.log(res);
}
The test is successful when the page receives the returned data.
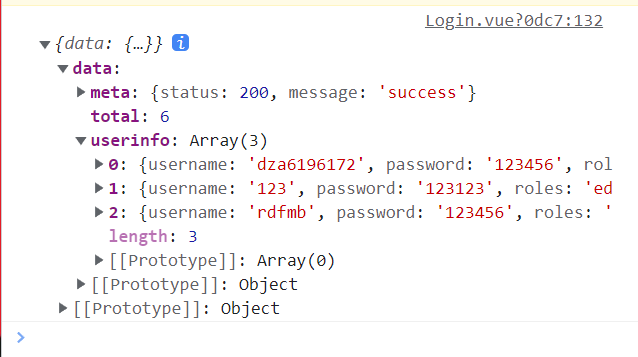
After the test can send the request, write the simulated login method request in methods, remember to change the click event of the button
loginButton(){
this.$refs.loginForm.validate(async valid => { //规则校验
let data={
username:this.loginForm.username,
password:this.loginForm.password
}
if(!valid)return
const {data:res} = await this.$http.post('/user',data) //发送请求
console.log(res.data)
console.log(res.data.userinfo[1].name)
if(res.data.meta.status!==200){
return this.$message.error('用户名或密码错误')
}
this.$message.success('登录成功')
this.$router.push('home')
})
}
Then add code to mock/index.js
Mock.mock('/user', 'post', req => { //路径与请求方式
const { username, password } = JSON.parse(req.body); //将传递进来的数据保存
for (let i = 0; i < userList.data.userinfo.length; i++) {
//判断userList中是否存在该用户并且用户密码是否正确
if (username === userList.data.userinfo[i].username && password === userList.data.userinfo[i].password) {
return {
meta: {
msg: 'success',
status: 200
},
user: {
username: userList.data.userinfo[i].username,
roles: userList.data.userinfo[i].roles
}
}
}
}
return {
meta: {
msg: 'error',
status: 201
}
}
})
At the same time, don't forget to change the get method in mock/userList.js to the post method
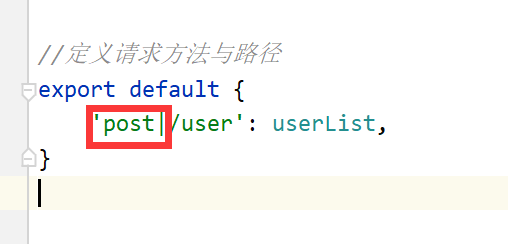
Enter the corresponding account password, and the mock login is successful.
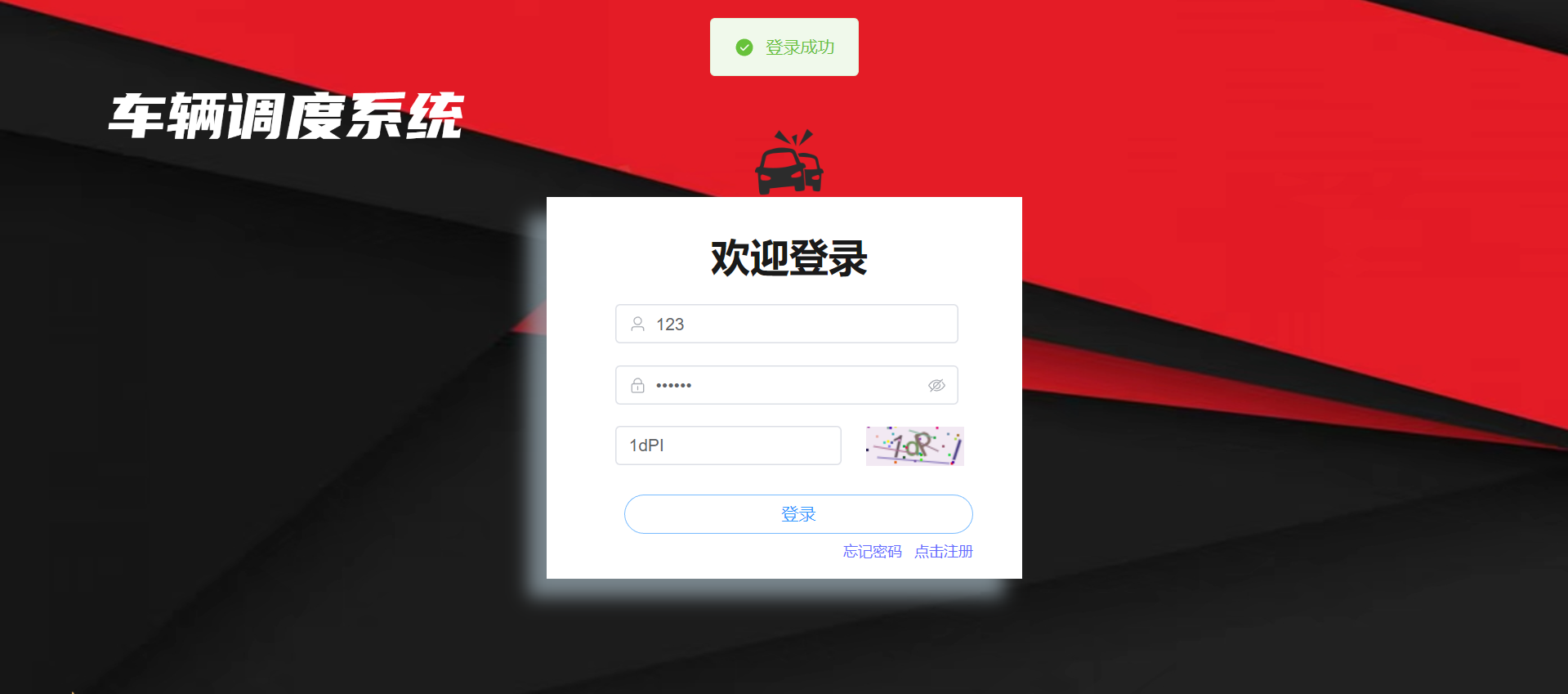
Use of Vuex
I want to display the user name after login, so the user name must be saved in vuex during login, so that data transfer between pages can be realized.
Create index.js under the store package
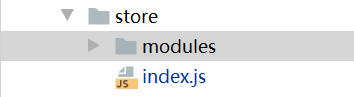
write the following code
import { createStore } from 'vuex'
export default createStore({
//公共state对象,存储所有组件的状态
state: {
user:{
name:'',
}
},
//唯一取值的方法,计算属性
getters: {
getUser(state){
return state.user;
}
},
//唯一可以修改state值的方法,同步阻塞
mutations: {
updateUser(state,user){
state.user = user;
}
},
//异步调用 mutations方法
actions: {
asyncUpdateUser(context,user){
context.commit('updateUser',user);
}
},
modules: {
}
})
At the same time, add it to the loginButton method in login.vue
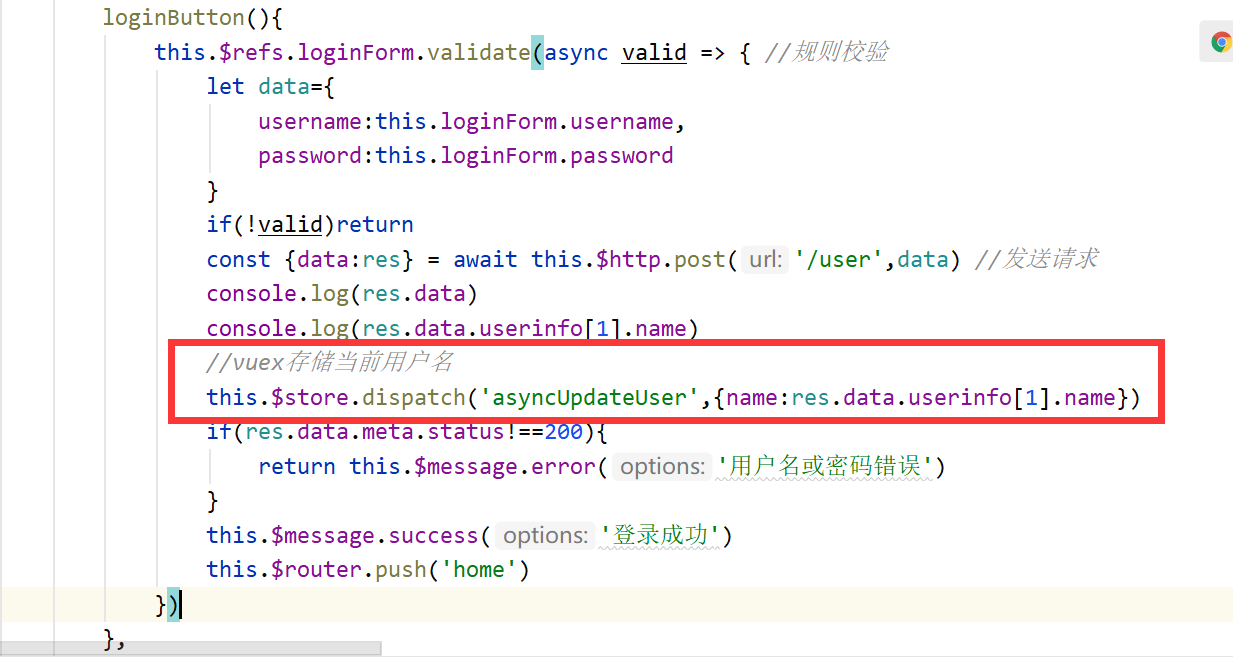
//vuex存储当前用户名
this.$store.dispatch('asyncUpdateUser',{name:res.data.userinfo[1].name})
Then add the following paragraph on the home page after the login jump, and the user name can be displayed.
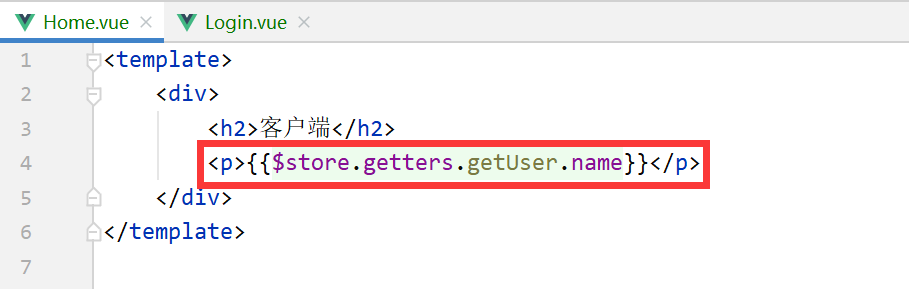
<p>{
{$store.getters.getUser.name}}</p>
But there will be a problem, if you refresh the page, the username will disappear.
Vuex data loss problem when browser refreshes
After we pass the data to the next page, after refreshing again, the data in the store will be reset, resulting in data loss. The data in Vuex should be stored persistently, so that the data stored in the store can be guaranteed not to be lost when the page is refreshed.
resolution process
Monitor whether the page is updated. If the page is updated, store the state object in sessionStorage. After the page is opened, judge whether there is a state object in the sessionStorage. If it exists, it means that the page has been refreshed. Take out the data stored in the sessionStorage and assign it to the state in vuex. If it does not exist, it means that it is opened for the first time, and the initial value of the state defined in vuex is taken.
Note: We want to save the passed data to sessionStorage. You must know that only strings can be stored in sessionStorage, so convert it first and then store it.
在app.vue中写
mounted() {
window.addEventListener('unload', this.saveState)
},
methods: {
saveState() {
sessionStorage.setItem('state', JSON.stringify(this.$store.state))
},
},
在store/index.js中,给state添加判断条件:
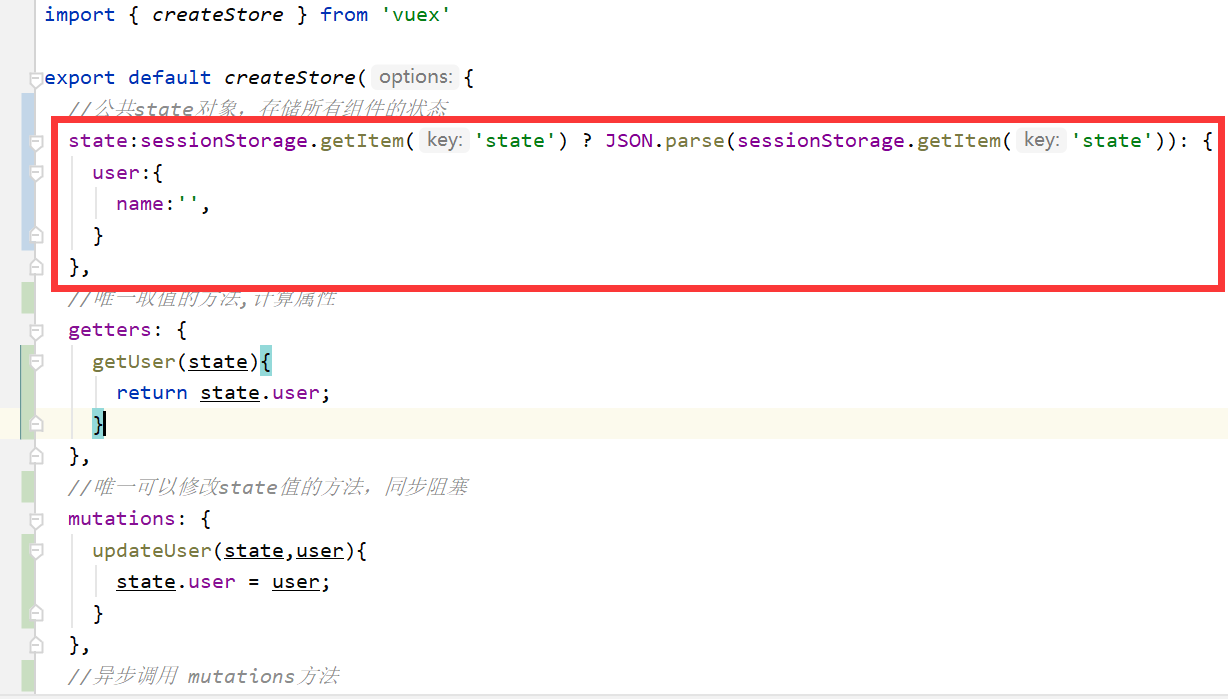
//公共state对象,存储所有组件的状态
state:sessionStorage.getItem('state') ? JSON.parse(sessionStorage.getItem('state')): {
user:{
name:'',
}
},
再次测试,刷新以后用户名不会消失。
参考资料:
https://blog.csdn.net/iufesjgc/article/details/108665553
https://www.bilibili.com/video/BV1cU4y1j7aP?p=1&vd_source=6264a75f147feaf5cd9f6d06ff0ba040