Embedded design and development project - DS18B20 temperature sensor program design
Knowledge expansion:
① DS18B20 is a single-wire interface digital temperature sensor, the measurement range is -55 ~ +125°C, the accuracy within the range of -10°C ~ +85°C is +0.5°C, and the measurement resolution is 9 ~ 12 bits (the reset value is 12 bits , the maximum conversion time is 750ms)
② DS18B20 includes parasitic power supply circuit , 64-bit ROM and single-line interface circuit , temporary register , EEPROM , 8-bit CRC generator and temperature sensor , etc. The parasitic power supply circuit can realize external power supply and single-line parasitic power supply. The 48-bit serial number stored in the 64-bit ROM is used to identify multiple DS18B20 connected on the same single line to realize multi-point temperature measurement.
③The format of the 64-bit ROM code is: 8-bit CRC check code + 48-bit serial number + 8-bit serial code (0x28)
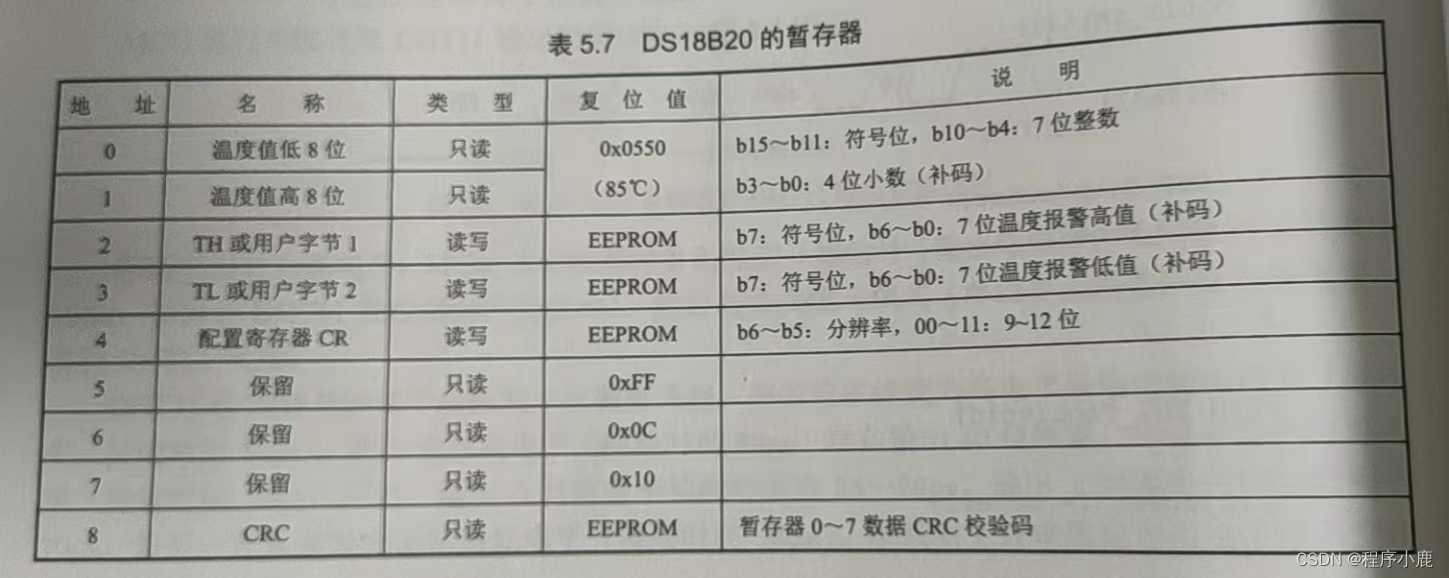
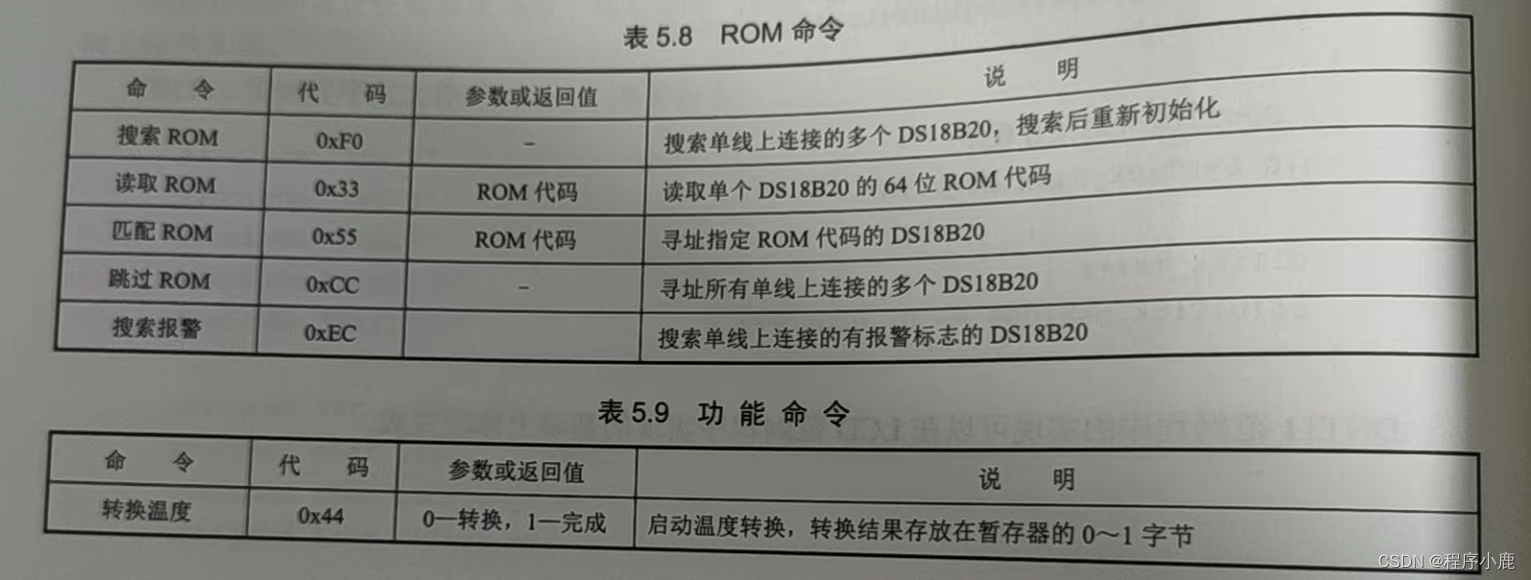
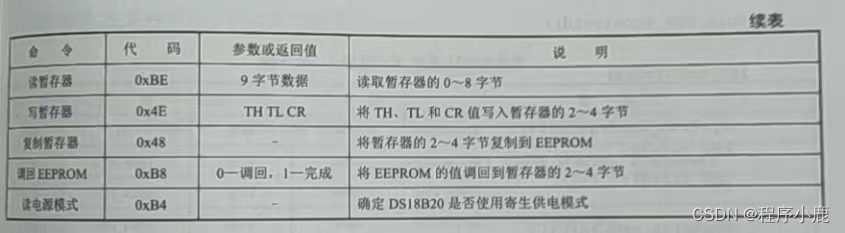
1. Implemented functions
- ①Get the temperature data of DS18B20 in real time and display it on the LCD screen;
- ② 8 LED running water lights control, light up a light every 1s, repeat the above steps;;
- ③ Write the process of dsp_read() according to the data acquisition requirements of the sensor;
2. Realize the code according to the function
1. The main file main.c
#include"key.h"
#include"led.h"
#include"lcd.h"
#include"stdio.h"
#include"ds18b20.h"
unsigned int uiDsb_Val;
unsigned char ucSec , ucSec1;
unsigned char pucStr[21];
unsigned long ulTick_ms;
void DSB_Proc(void);
int main(void)
{
SysTick_Config(72000); //定时1ms(HCLK = 72MHz)
KEY_Init();
LED_Init();
STM3210B_LCD_Init();
LCD_Clear(Blue);
LCD_SetBackColor(Blue);
LCD_SetTextColor(White);
ds18b20_init_x();
while(1)
{
LED_Disp(ucSec);
DSB_Proc();
}
}
void DSB_Proc(void)
{
if(ucSec != ucSec1)
{
ucSec1 = ucSec;
uiDsb_Val = dsp_read();
sprintf((char*)pucStr," Temp:%5.2fC",uiDsb_Val/16.0);
LCD_DisplayStringLine(Line5,pucStr);
}
}
//SysTick 中断处理程序
void SysTick_Handler(void)
{
ulTick_ms++;
if(ulTick_ms % 1000 ==0)
ucSec++;
}
Main function analysis: ❤️ ❤️ ❤️
- The 16-bit binary number uiDsb_Val returned by DS18B20 represents the detected temperature value at the moment, and its upper five bits represent positive and negative. If the upper five bits are all 1, it means that the returned temperature value is negative. If the upper five bits are all 0, it means the returned temperature value is positive. The following 11-bit data represents the absolute value of the temperature . After converting it to a decimal value, multiply it by 0.0625 to obtain the temperature value at this time .
- Shifting n bits to the left is equal to multiplying by 2 n , shifting n bits to the right is equal to dividing by 2 n For example: dividing by 16 is equivalent to shifting to the right by 4 bits;
2. The header file "ds18b20.h" of DS18B20
#ifndef __DS18B20_H
#define __DS18B20_H
#include "stm32f10x.h"
#define OW_DIR_OUT() mode_output1()
#define OW_DIR_IN() mode_input1()
#define OW_OUT_LOW() (GPIO_ResetBits(GPIOA,GPIO_Pin_6))
#define OW_GET_IN() (GPIO_ReadInputDataBit(GPIOA,GPIO_Pin_6))
#define OW_SKIP_ROM 0xCC
#define DS18B20_CONVERT 0x44
#define DS18B20_READ 0xBE
void ds18b20_init_x(void);
unsigned int dsp_read(void);
#endif
Brief analysis: ❤️ ❤️
- Encapsulate the function through the macro definition #define , which can be used directly;
- Contains functions for initializing pins and getting greenhouse data;
3. The source file "ds18b20.c" of DS18B20
#include "stm32f10x.h"
#include "ds18b20.h"
#define delay_us(X) delay((X)*72/5)
void delay(unsigned int n)
{
while(n--);
}
void ds18b20_init_x(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* Enable clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA , ENABLE);
/* Configure Ports */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_2MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
//
void mode_input1(void )
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_2MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void mode_output1(void )
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_2MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
//
uint8_t ow_reset(void)
{
uint8_t err;
OW_DIR_OUT(); // pull OW-Pin low for 480us
OW_OUT_LOW(); // disable internal pull-up (maybe on from parasite)
delay_us(400); //about 480us
// set Pin as input - wait for clients to pull low
OW_DIR_IN(); // input
delay_us(66);
err = OW_GET_IN(); // no presence detect
// nobody pulled to low, still high
// after a delay the clients should release the line
// and input-pin gets back to high due to pull-up-resistor
delay_us(480-66);
if( OW_GET_IN() == 0 ) // short circuit
err = 1;
return err;
}
uint8_t ow_bit_io( uint8_t b )
{
OW_DIR_OUT(); // drive bus low
OW_OUT_LOW();
delay_us(1); // Recovery-Time wuffwuff was 1
if ( b ) OW_DIR_IN(); // if bit is 1 set bus high (by ext. pull-up)
#define OW_CONF_DELAYOFFSET 5
delay_us(15-1-OW_CONF_DELAYOFFSET);
if( OW_GET_IN() == 0 ) b = 0; // sample at end of read-timeslot
delay_us(60-15);
OW_DIR_IN();
return b;
}
uint8_t ow_byte_wr( uint8_t b )
{
uint8_t i = 8, j;
do
{
j = ow_bit_io( b & 1 );
b >>= 1;
if( j ) b |= 0x80;
}
while( --i );
return b;
}
//
uint8_t ow_byte_rd( void )
{
return ow_byte_wr( 0xFF );
}
//获取DS18B20温度传感器
unsigned int dsp_read(void)
{
unsigned char th,tl;
ow_reset(); //复位
ow_byte_wr(0xCC); //跳过Rom
ow_byte_wr(0x44); //转换温度
ow_reset();
ow_byte_wr(0xCC);
ow_byte_wr(0xBE); //读暂存器
tl = ow_byte_wr(0xFF); //读温度值低8位
th = ow_byte_wr(0xFF); //读温度值高8位
return (th<<8) + tl;
}
Brief analysis: ❤️ ❤️
- The * #define delay_us(X) delay((X) 72/5) parameter of the delay function us needs to add parentheses ();
- For the initialization port, please refer to Blue Bridge Cup - Embedded Design and Development Project - LED Indicator Program Design
- DS18B20 internal circuit and timing detailed introduction can refer to: DS18B20 temperature sensor principle detailed explanation and routine code
- The operation steps include: reset → ROM command → function command
3. Attention and learning points in the process of realizing functions
1. Precautions
1. The obtained temperature value needs to be multiplied by 0.625 to be converted into the correct temperature value;
2. Knowledge points learned
- ① Obtain the real-time data of the temperature sensor of DS18B20;
- ② Acquire the temperature data of the register according to the ROM command table and the function command table;
- ③Familiar with the application of DS18B20 temperature sensor in actual combat;
❤️ ❤️ ❤️ ❤️ ❤️ ❤️