flat structure data
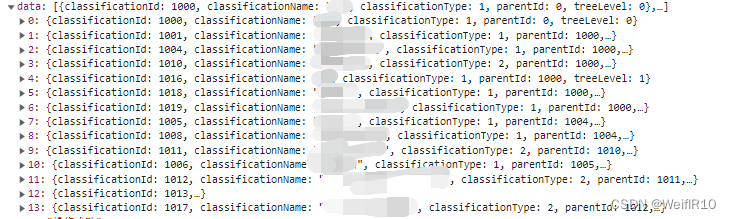
tree structure data
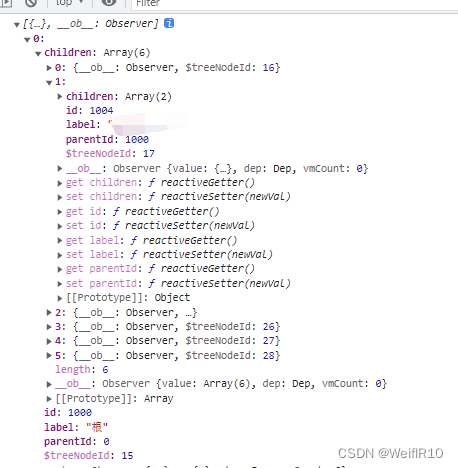
Implementation idea: use recursive implementation
// reOptions是从后端获取到的扁平结构
getOption() {
const tree = []
// 我是利用el-tree做的,所以先把后端给的数据构建成el-tree里需要的字段名称
const list = this.reOptions.map(item => ({
label: item.classificationName,
id: item.classificationId,
parentId: item.parentId
}))
// 遍历构建好字段名称数据,调用递归函数
for (let i = 0, len = list.length; i < len; i++) {
if (!list[i].parentId) {
const item = this.queryChildren(list[i], list)
tree.push(item)
}
}
// 赋值
this.options = tree
},
// 将父级分类的数据结构转换为树形结构
queryChildren(parent, list) {
const children = []
for (let i = 0, len = list.length; i < len; i++) {
if (list[i].parentId === parent.id) {
const item = this.queryChildren(list[i], list)
children.push(item)
}
}
if (children.length) {
parent.children = children
}
return parent
},
Refer to the department management of Ruoyi system
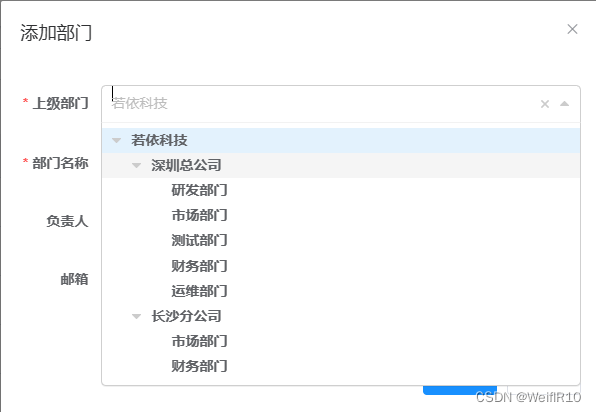
When modifying, the parent department cannot select itself and its own subset
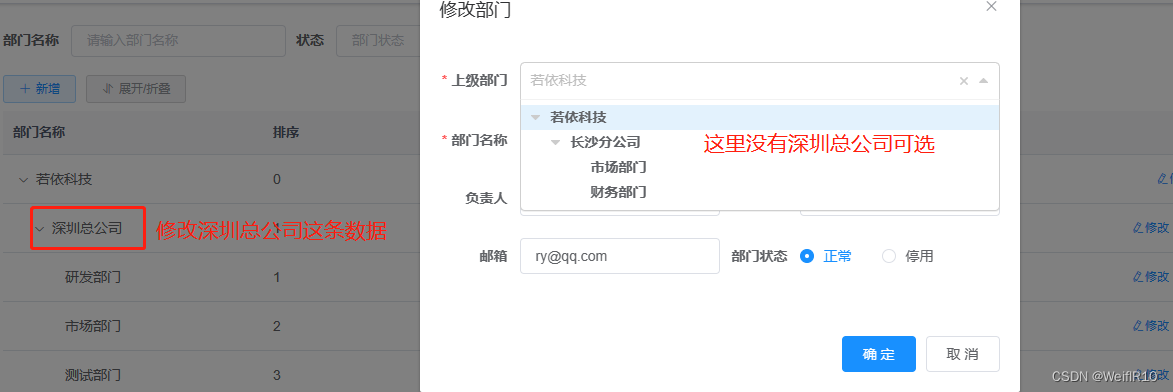
So you can disable or delete according to your own needs (I personally think that deleting is relatively simple, and disabling is more complicated. Secondly, the usage method of el-tree as shown in the figure does not seem to have the disable function)
// 在树形结构中找到当前选中的值,并删除他以及他的子节点
this.searchTree(this.options, this.classificationId) //调用
// 参数nodes是构建好的树形结构数据;searchKey是你要修改的当前数据id
searchTree(nodes, searchKey) {
for (let _i = 0; _i < nodes.length; _i++) {
// 遍历树形结构数据,在其中找到当前数据id,进行删除
if (nodes[_i].id === searchKey) {
nodes.splice(_i, 1) //可用delete nodes[_i],但不知道为啥,我用delete删除后成undefined了,导致渲染数据时报错
return nodes
} else {
if (nodes[_i].children && nodes[_i].children.length > 0) {
let res = this.searchTree(nodes[_i].children, searchKey);
if (res) {
return res
}
}
}
}
return null
},
According to the implementation of Ruoyi, only the last level name is displayed after selection

If you want the following effect, you need to process the data again
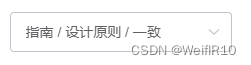
// 父级分类数据回显筛选出当前级的父级
echoOptions() {
this.sortArr = []
// 筛选出当前级的父级
this.parentArr = this.reOptions.filter(item => item.classificationId == this.parentId)[0]
this.sortArr.push(this.parentArr.classificationName)
this.recursionOptions()
},
recursionOptions() {
//筛选出当前级 父级 的 父级 即:当前级的爷级
if (this.parentArr.parentId != 0) {
this.parentArr = this.reOptions.filter(item => item.classificationId == this.parentArr.parentId)[0]
this.sortArr.push(this.parentArr.classificationName)
this.recursionOptions()
} else {
var reSortArr = this.sortArr.reverse() // 追加进去的数据是倒序的,而页面展示是正序的,故需要翻转过来
this.form.parentName = reSortArr.join('/') // 使用 / 拼接
}
return
},