How to judge whether the object can be recycled?
There are two ways to judge whether an object can be recycled: the reference counting method and the reachability analysis algorithm.
The reference counting method means that as long as an object is referenced by other objects, the count of this object will be increased by one. If it is referenced twice, the count will be increased by two. If a variable is no longer referencing it, its count will be decreased by one. When the count becomes 0, it means that it is no longer referenced and can be collected as garbage. However, there is an important disadvantage of the reference counting method, that is, the problem of circular reference. There are two objects A and B. The object A is referencing B, and the count of B is 1, and then B is referencing the A object, and the count of A is It is also 1, but now there are no other variables that refer to objects A and B, because the count of the AB object is 1, so the AB object cannot be collected as garbage, which causes a memory leak. The early Python virtual machine garbage collection mechanism used the reference counting method, but it was not used in Java. Instead, it used the second algorithm—the reachability analysis algorithm.
For the reachability analysis algorithm, it is first necessary to determine a series of root objects. The so-called root objects can be understood as those objects that cannot be regarded as garbage. Before garbage collection, all objects in the heap are scanned to see if each object is directly or indirectly referenced by the root object. If it is, it cannot be recycled, otherwise it can be recycled as garbage. A very simple example is to eat grapes in summer. After we wash the grapes, we lift up the roots of the grapes. The grape wine connected to the roots of the grapes is equivalent to the root objects. These cannot be recycled, because The root is referencing them, and the grapes that are disconnected from the root and dropped on the plate are those objects that can be recycled.
The specific approach of the reachability analysis algorithm is to scan the objects in the heap to see if the scanned object can be found along the application chain starting from the GC Root object. If it can be found, it means that it can be retained. If it cannot be found, it means that it can be recycled. .
There are four kinds of references in the Java virtual machine. In fact, strictly speaking, there are five kinds of references, namely strong, soft, weak, virtual, and terminator (as shown in the figure). The implementation arrows in the figure indicate strong references, and the dotted lines indicate soft, weak, virtual, and terminator references.
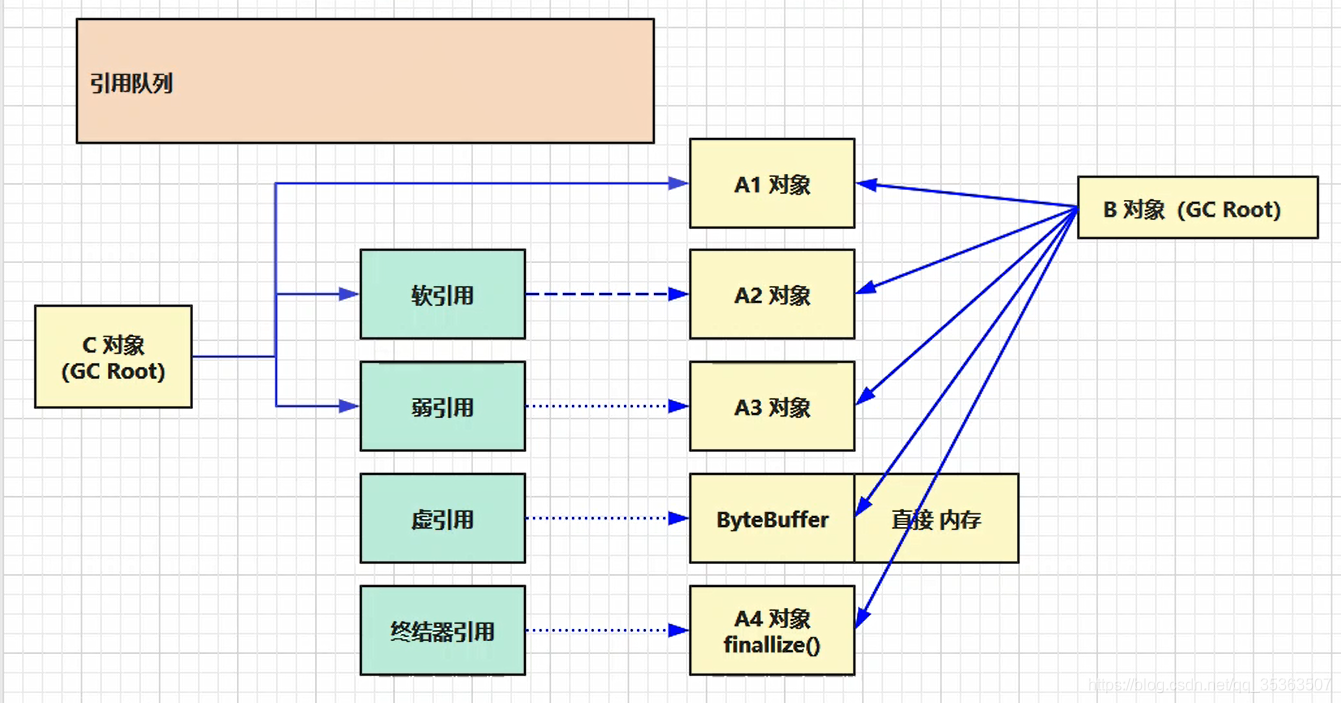
For strong references, in fact, what we usually refer to are strong references. For example, we create an object through new, and then give this object to a variable through the operator of the equal sign. At this time, this variable strongly refers to the object just now. , The characteristics of strong references: as long as the reference chain of GC root can be found, it cannot be garbage collected. The A1 object in the figure is strongly referenced, so A1 cannot be recycled. Only when there are no direct and brief references to A1 from the GC root will it be recycled.
Soft references are similar to weak references. The difference between weak references and strong references is that as long as A2 and A3 are not directly referenced by strong references, they may be recycled during garbage collection. In the figure, A2 is referenced by the introduction of C, although it is through strong references A soft reference object, and then to the A2 object, there is an indirect call, and A2 is strongly referenced by B. At this time, garbage collection cannot be recovered. But when the object B no longer strongly references A2, and only one soft reference object references A2, the object referenced by the soft reference will be released when the garbage is collected and the memory is insufficient. Weak references are also when there are no strong references, regardless of whether the memory is sufficient, the weakly referenced objects will be recycled during garbage collection. Weak references can also work with a reference queue, that is, when the object referenced by the soft reference object is recycled, the soft reference object itself is also an object. If a reference queue is assigned to it when it is created, when garbage is collected, the soft reference object The reference object will enter the reference queue, and the same is true for weak references. Weak references themselves also occupy memory. If you want to release the memory occupied by them, you need to use the reference pair to find them and then deal with them accordingly.
Phantom references must be used with reference pairs, mainly used with ByteBuffer. When the referenced object is recycled, the phantom reference will be queued, and the Reference Handler thread will call the phantom reference related methods to release the direct memory.
Finalizer reference, no manual coding is required, but it is used internally with reference pairs. During garbage collection, the finalizer is introduced into the queue (the referenced object has not been recycled temporarily), and then the Finalizer thread finds the referenced object through the finalizer reference and calls Its finalize method, the referenced object can only be recovered during the second GC.