The essence of IOC container:
Ioc is essentially an object container, called a bean container here, you can set the
All objects that may be used in the program are put into the Ioc container, and when an object is used, it is taken from the container.
This kind of object preloading operation can greatly improve the running speed of the program, because you don't need to
Take the time to create the object, but go directly to the Ioc container to get it. The essence is the time cost of creating the object
Moved to the moment when the program was started.
As described above, it can be seen that the basic use of Ioc lies in
How to inject objects into Ioc
How to get the object out of Ioc
The basic steps of using Ioc are as follows:
Import Maven dependencies
Create a spring applicationContext.xml configuration file in Resources under the maven project
Read the spring configuration file through the ClassPathXmlApplicationContext object in the main program
Use DI (xml-based/annotation-based) to inject beans into the spring container
Maven dependencies
Spring commonly used maven dependencies are as follows
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-expression</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.3.21</version>
</dependency>
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.42</version>
<scope>runtime</scope>
</dependency>
</dependencies>
Bean configuration file for Spring container
Suggested file name : applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<!--①使用spring约束-->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
<!-- 2.使用基于注解的包扫描器-->
<context:component-scan base-package=""/>
</beans>
The application reads the Spring configuration file
ApplicationContext ioc=new
ClassPathXmlApplicationContext("applicationContext.xml");
The returned ioc is the ioc container, through which beans can be obtained from the container
DI (xml-based)
Inject beans into the ioc container through the xml configuration file
<?xml version="1.0" encoding="UTF-8"?>
<!--使用spring约束-->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
<!-- 2.使用基于注解的包扫描器-->
<!-- <context:component-scan base-package="com.itheima"/>-->
<bean id="user" class="com.itheima.entity.User" scope="singleton">
<!-- 基于类的属性进行注入(需要类中有对应属性的GetSet方法)-->
<property name="id" value="22"/>
<property name="name" value="小米1"/>
<property name="password" value="6666"/>
</bean>
<bean id="manager" class="com.itheima.entity.Manager" scope="singleton">
<!-- 基于类的构造器进行注入(需要类中有对应属性的构造器)-->
<constructor-arg name="id" value="22"/>
<constructor-arg name="name" value="小米2"/>
</bean>
</beans>
DL (annotation based)
Inject beans into the ioc container through annotations
<?xml version="1.0" encoding="UTF-8"?>
<!--使用spring约束-->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
<!-- 设置扫描注解的包扫描器-->
<context:component-scan base-package="com.itheima"/>
</beans>
other tips
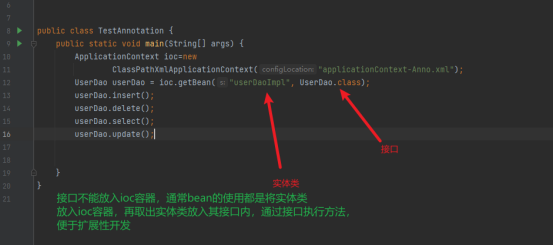