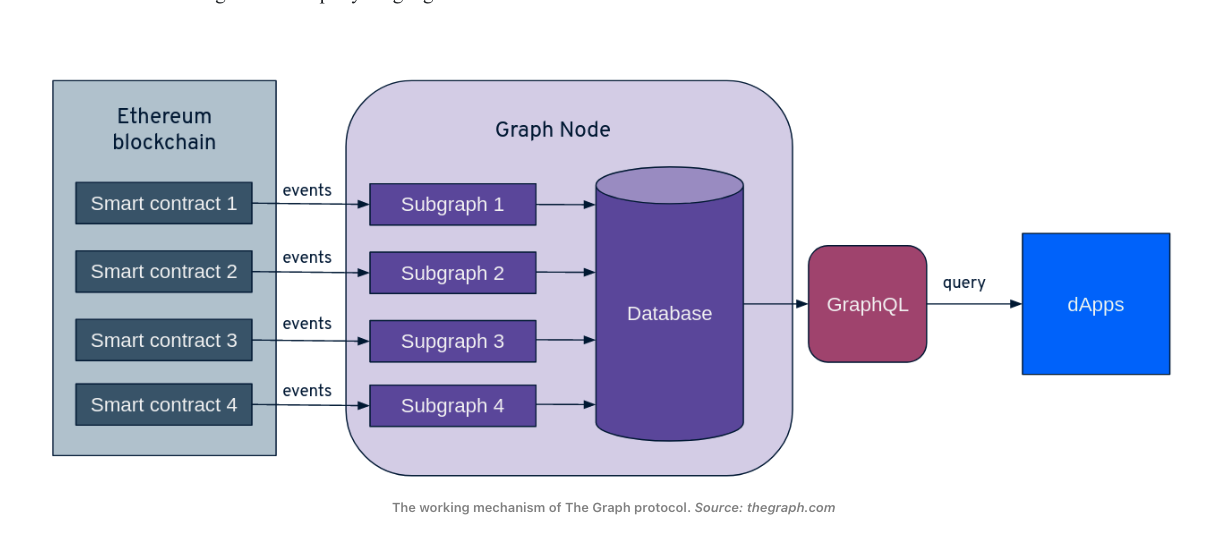
Installation Environment
Truffle && Ganache
Truffle is the most comprehensive smart contract development suite
Built-in smart contract compilation, linking, deployment and binary management.
Advanced debugging with breakpoints, variable analysis, and step functionality.
Truffle is written based on JavaScript and can be debugged using console.log in smart contracts
Deploy and trade with MetaMask and Truffle Dashboard, protecting your mnemonic.
An external script runner that executes scripts within the Truffle environment.
Automated contract testing for rapid development.
Use NPM for package management, using the ERC190 standard
Scriptable, scalable deployment and migration architecture
Interactive console available for contract communication
# 安装node
nvm install 18
# 安装Truffle
npm install -g truffle
# 查看Truffle版本
truffle version
# 安卓ganache
https://trufflesuite.com/docs/ganache/quickstart/
Install IDE plugins
https://marketplace.visualstudio.com/items?itemName=trufflesuite-csi.truffle-vscode
https://trufflesuite.com/blog/build-on-web3-with-truffle-vs-code-extension/
vscodeInstall Truffle for VS Code
Instructions for use
https://blog.csdn.net/VictorXSS/article/details/127852997
In this document, pay attention to adding 2_deploy_contracts.js in migrations
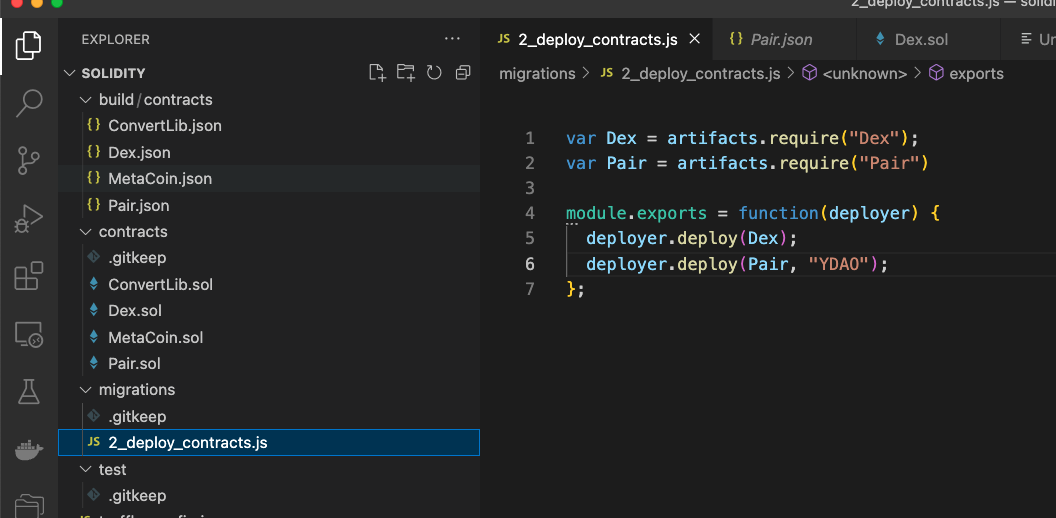
In this way, the deploy can be successful
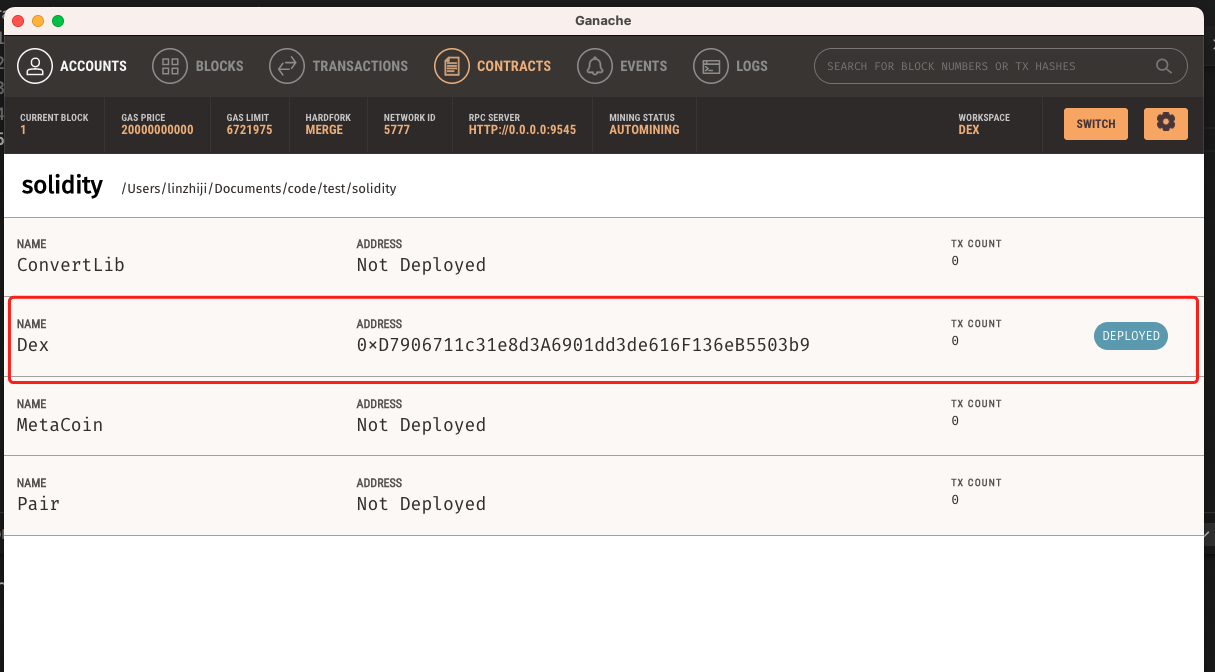
solidity code
// SPDX-License-Identifier: MIT
pragma solidity >=0.4.22 <0.9.0;
contract Pair{
string private _name;
uint private _liquidity;
constructor(string memory name){
_name = name;
}
function addLiquidity(uint amount) public {
_liquidity+=amount;
}
function getLiquidity() public view returns(uint){
return _liquidity;
}
function getName() public view returns(string memory){
return _name;
}
}
contract Dex {
address private _owner;
uint private _index = 0;
mapping(address => Pair) private _pairs;
mapping(uint => address) private _indexs;
modifier onlyOwner(){
require(msg.sender == _owner,"must be owner");
_;
}
event PairCreated(string name, address pairAddress);
event AddLiquidity(address pairAddress, uint liquidity);
function createPair(string memory name) public {
Pair pair = new Pair(name);
_pairs[address(pair)] = pair;
_indexs[_index] = address(pair);
_index++;
emit PairCreated(name, address(pair));
}
function addLiquidity(address pairedAddress,uint liquidity) public {
_pairs[pairedAddress].addLiquidity(liquidity);
emit AddLiquidity(pairedAddress,liquidity);
}
function getCount() public view returns(uint){
return _index;
}
function pairAddress(uint index) public view returns(address){
return _indexs[index];
}
function getPairName(address pairedAddress) public view returns(string memory){
return _pairs[pairedAddress].getName();
}
function getLiquidity(address pairedAddress) public view returns(uint liquidity){
return _pairs[pairedAddress].getLiquidity();
}
}
core code
# solidity 里的代码
emit PairCreated(name, address(pair));
emit AddLiquidity(pairAddress,liquidity);
# subgraph.yaml
eventHandlers:
- event: PairCreated(string,address)
handler: handleNewPairCreated
- event: AddLiquidity(address,uint256)
handler: handleAddLiquidity
import {AddLiquidity, PairCreated} from '../generated/study/Dex'
import {Pair} from '../generated/schema'
export function handleNewPairCreated(event: PairCreated): void {
let pair = new Pair(event.params.pairAddress.toHexString())
pair.displayName = event.params.name
pair.liquidity = 0
pair.save()
}
export function handleAddLiquidity(event: AddLiquidity): void {
let id = event.params.pairAddress.toHexString()
let pair = Pair.load(id)
if (pair == null) {
return
}
pair.liquidity += event.params.liquidity.toI32()
pair.save()
}
PairCreated is the emit method in solidity
handleNewPairCreated is the code in mapping.ts
When PairCreated in solidity is called, handleNewPairCreated will be triggered to save the data. Save data can be database or file
The trigger is provided by the provider
provider 是Web3 provider is a website running geth or parity node which talks to Ethereum network.
参考
https://blog.csdn.net/u013705066/article/details/123573546
https://blog.quicknode.com/the-importance-of-web3-provider-redundancy
https://ethereum.stackexchange.com/questions/31218/what-are-providers-in-the-ethereum
https://techfi.tech/introduction-to-the-graph-the-google-of-web3/