1. Application management API for TCP network connection
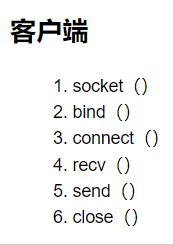
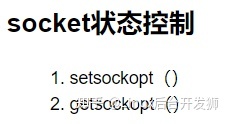
For clients, calling bind() explicitly is unnecessary in most cases.
1. API introduction
1.1 socket()
Calling socket() creates a socket object. A socket consists of two parts, a file descriptor (fd) and a TCP Control Block (tcb).
The socket will save a five-tuple (remote IP, remote PORT, local IP, local PORT, protocol) as its own identity.
1.2 bind()
Call bind to bind the socket to the local IP and port (port).
1.3 listend()
After the server calls listen(), it starts to monitor the connection requests sent to the socket on the network.
listen(fd, size), fd is the file descriptor of the socket, and size refers to the length of the full connection queue in Linux, that is, at most size connection requests can be saved at one time.
1.4 connect()
The client calls the connect() function to initiate a connection request to the specified server.
1.5 accept()
The accept() function only does two things, take the connection request out of the full connection queue, assign an fd to the connection and return.
1.6 recv()
Receive data function, this function copies data from the receive buffer in kernel mode to user space.
1.7 send()
Send function, this function copies data from the user mode to the send buffer of the kernel mode, and the specific sending is controlled by the protocol.
1.8 close()
Close the connection function.
2. TCP three-way handshake
The three-way handshake is initiated by the client calling the connect() function. The server must call the listen() function before the client initiates connect to receive the connection request.
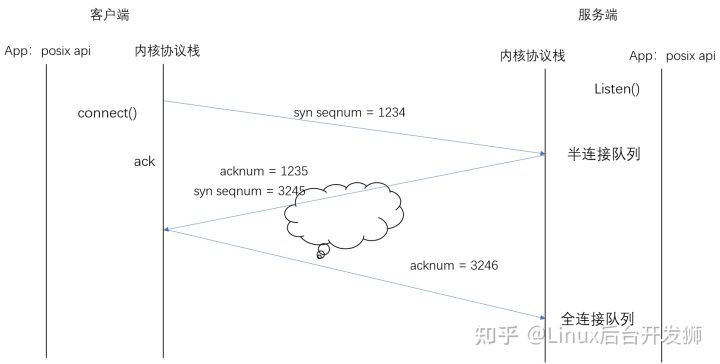
2.1 Message
syn is the request message, and seqnum is the number of the sent message. The acknum is the number of the message that the receiver wants the sender to send, and it also notifies the sender that all the previous numbered messages have been received.
During the TCP connection establishment process, the server sends the confirmation message and the connection request message together, so this two-way connection process only has three handshakes.
2.2 The relationship between three-way handshake and API
In the TCP protocol stack, the server receives the syn message for the first time, and stores the request in the semi-connection queue. After receiving the ack message, the server will check whether there is a corresponding semi-connection through the five-tuple in the semi-connection queue. If it exists, it will transfer the connection request to the full connection queue, and then wait for the server to call accept( ).
When a connection request is placed in the semi-connection queue, the server will apply for a TCB for the connection. After calling the accept() function, the connection gets fd.
The connect() function initiates the first handshake and blocks until the second handshake is complete.
2.3 TCP characteristics
In fact, there are only 65535 ports for an operating system, so how do they create millions of connections? This involves port multiplexing (this article does not explain this content).
There is a common problem in TCP transmission of messages, sticky packets. The sticky packet problem is caused by the TCP protocol stack combining or splitting the data we send and then sending it. There are generally two solutions to this: (the order of the data received by default)
- Add packet length information to the protocol header
- Set the delimiter at the end of the message packet
TCP has a timeout retransmission mechanism for sending messages, and a delayed ack mechanism for receiving data confirmation, all of which are controlled by timers. TCP mainly has four timers, namely:
- Retransmission timer: Retransmission Timer
When TCP sends a segment, it creates a retransmission timer for this particular segment, and two situations may occur: if an acknowledgment for the segment is received before the timer expires, the timer is revoked; If the timer expires before the acknowledgment of a specific message segment, the message is retransmitted and the timer is reset. The timer time is generally set to 2RTT (RTT is the round-trip time from the client message to the server, which generally needs to be calculated dynamically).
- Persistent Timer: Persistent Timer
The persistence timer is specially set up to deal with zero-window notifications (the receiving port has not been opened by the other party). When the sender receives the zero-window confirmation, it starts the persistence timer. When the persistence timer expires, the sender TCP sends a special segment called a detection segment. There is only one segment in this segment. bytes of data. Probe segment sequence numbers, but sequence numbers are never acknowledged, and are even ignored when calculating acknowledgments for other parts of the data. The detection segment reminds the receiving end TCP that the confirmation has been lost and must be retransmitted. The deadline of the persistence timer is set to the value of the retransmission time, but if no response is received from the receiving end, another probe segment is sent, and the value of the persistence timer is doubled and reset, and the sending end continues Probe segments are sent, doubling and resetting the persistence timer until the value increases to a threshold (usually 60 seconds). After that, the sender sends a segment every 60s until the window is reopened.
- Keep alive timer: Keeplive Timer
Whenever the server receives information from the client, it resets the keeplive timer. The timeout is usually set to 2 hours. If the server has not received any information from the client for more than 2 hours, it sends a probe segment. If 10 probes are sent segment (sent every 75 seconds) has not received a response, the connection is terminated.
- Time waiting timer: Time_Wait Timer
It is used during the connection termination period. When TCP closes the connection, it does not think that the connection is really closed. During the time waiting period, the connection is still in an intermediate state. In this way, repeated fin segments can be discarded after reaching the end point. The value of this timer is usually set to twice the life expectancy value of a segment (2MSL).
3. The 11 states of TCP
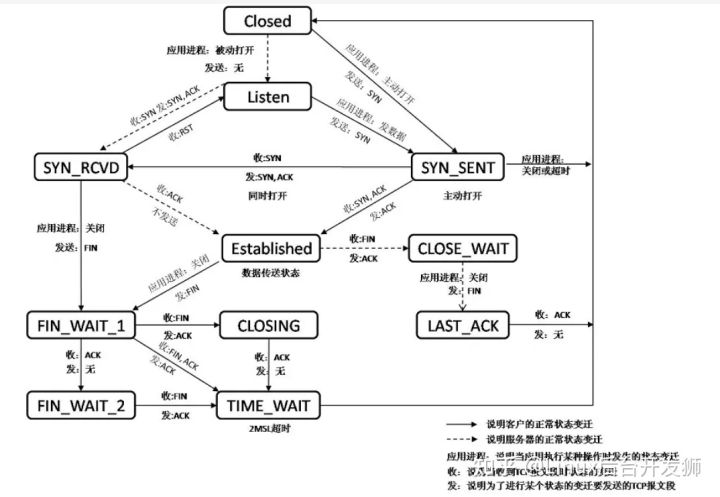
4. Four waves of TCP
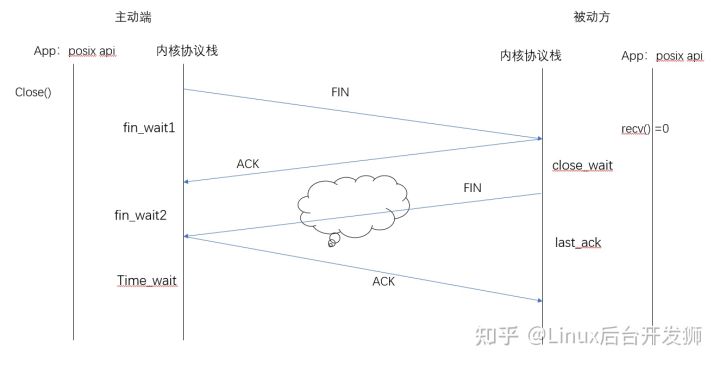
When both parties communicate, one party calls close() actively, and TCP starts to wave four times. The party that passively receives the communication close notification calls close() after processing the communication business.
The communication parties will enter the state as shown above.
When the passive party's business processing takes a long time or fails to call close() in time for other reasons, the active party will be blocked in the FIN_WAIT2 state for a long time. , how to solve the long-term blocking in the FIN_WAIT2 state:
- Passive side: put business processing into the business queue if allowed
- Active party: The active party has a keeplive timing, beyond which the active party will directly terminate the communication connection
The active party enters the TIME_WAIT state after receiving the ACK and sending the FIN. This state generally lasts for 2MSL (the maximum survival time of a message in the network).
If both parties actively initiate the shutdown notification before receiving the FIN message, the state diagram is as follows:
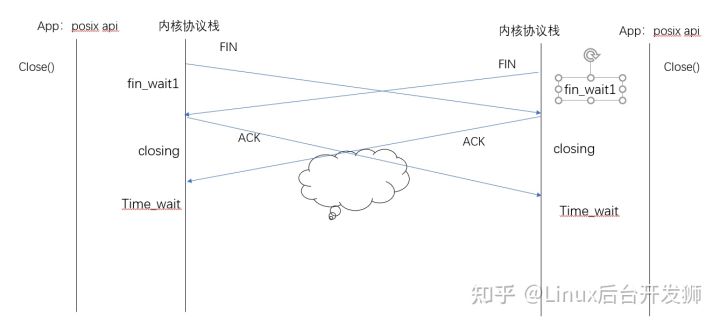
5. UDP
UDP provides higher real-time performance than TCP, and does not require message confirmation messages, which is more suitable for weak network environments.
As mentioned above, TCP has an ACK mechanism. When a message is lost, it needs to retransmit all subsequent messages starting from the lost number (even if it has been received, the sender will not know it). This will consume bandwidth. However, UDP does not need it, and UDP has no message confirmation mechanism.
Another important feature of TCP is congestion control, but UDP does not, so UDP can be used to seize bandwidth (well, Thunder accelerated download is such a thing). UDP preempting bandwidth will reduce the available network bandwidth of other users in the same network environment.
Reference Blog: Posix API and Network Stack