Table of contents
3. The basic process of promise
2.1. The way to specify the callback function is more flexible
2.2. Support chain calls, which can solve the problem of callback hell
1. What is Promise?
understand
1. Abstract expression :
1) Promise is a new technology
(ES6
specification
)
2) Promise
is
a new solution for asynchronous programming in
JS
Remarks: The old solution is to simply use the callback function
2.
Concrete expression
:
1)
Syntactically
: Promise
is a constructor
2)
From a functional point of view
: the promise
object is used to encapsulate an asynchronous operation and can obtain its success
/failure result value
2. The state of the promise changes
1. pending
becomes
resolved
2. pending
becomes
rejected
Description
:
There are only these
two
types
,
and a
promise
object can only be changed once
Whether it becomes success or failure
,
there will be a result data
The successful result data is generally called
value, and
the failed result data is generally called
reason
3. The basic process of promise
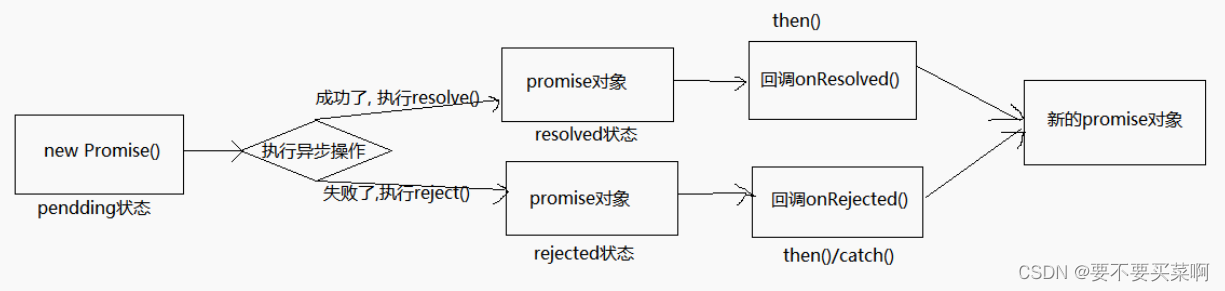
4. Basic usage of promise
Use 1: Basic encoding process
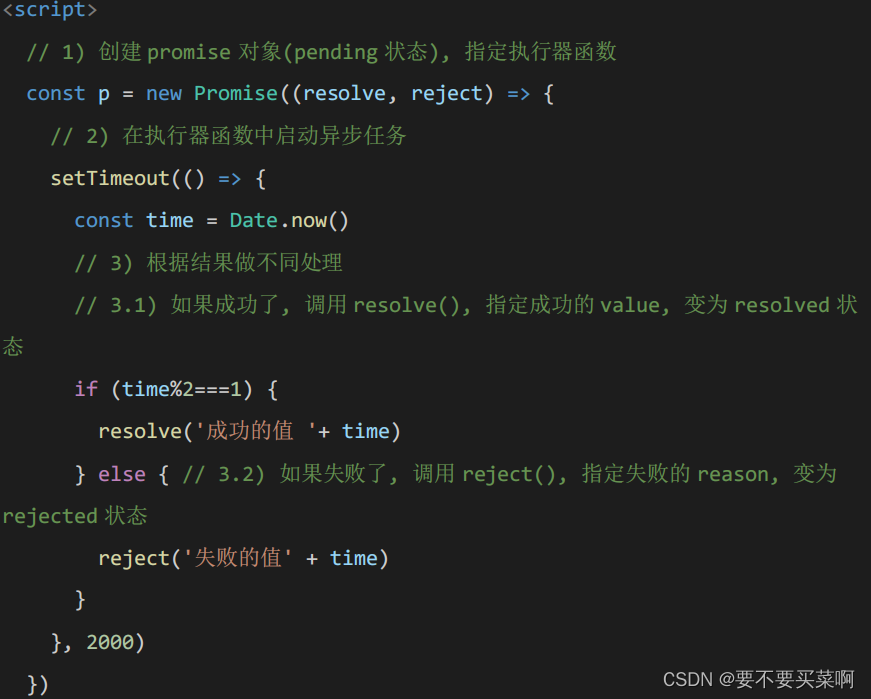
Use 2: Use promise to encapsulate timer-based asynchrony
Use 3: use promise to encapsulate ajax asynchronous request
2. Why use Promise?
2.1. The way to specify the callback function is more flexible
1.
Old
:
must be specified through the callback function before starting the asynchronous task
Promise之前的旧方案都是使用回调函数完成异步编程的操作
1、fs 文件操作 如 NodeJS
require('fs').requireFile('./index.html',(error,data)=>{})
2、数据库操作 如 MongoDB MySQL
3、 AJAX 网络请求
$.get('/server',(data)=>{})
4、定时器 setTimeout(()=>{},2000)
2. promise: start asynchronous task => return promise object => bind callback function to promise object
Number
( can be specified
/ multiple
even after the end of the asynchronous task
)
2.2. Support chain calls , which can solve the problem of callback hell
1.
What is callback hell
?
The callback function is called nestedly
, and
the result of the asynchronous execution of the external callback function is the condition of the nested callback execution
For example, if I want to say a sentence, the word order must be as follows: In martial arts, peace is the most important thing, and martial ethics should be emphasized, and no fighting in the nest should be avoided. If they are all asynchronous tasks, the following operations must be performed to ensure the correct order:
setTimeout(function () { //第一层
console.log('武林要以和为贵');
setTimeout(function () { //第二程
console.log('要讲武德');
setTimeout(function () { //第三层
console.log('不要搞窝里斗');
}, 1000)
}, 2000)
}, 3000)
Use promise to solve this problem
function fn(str){
var p=new Promise(function(resolve,reject){
//处理异步任务
var flag=true;
setTimeout(function(){
if(flag){
resolve(str)
}
else{
reject('操作失败')
}
})
})
return p;
}
fn('武林要以和为贵')
.then((data)=>{
console.log(data);
return fn('要讲武德');
})
.then((data)=>{
console.log(data);
return fn('不要搞窝里斗')
})
.then((data)=>{
console.log(data);
})
.catch((data)=>{
console.log(data);
})
2. Disadvantages of callback hell ?
not easy to read
Not easy to handle exceptions
3.
The solution
?
Promise
chain calls
4.
The ultimate solution
?
async/await
3. How to use Promise?
3.1. API
Promise
constructor
: Promise (excutor) {}
(1) executor
function
:
executor
(resolve, reject) => {}
(2) resolve
function
:
the function we call when the internal definition is successful
value => {}
(3) reject
function
:
the function we call when the internal definition fails
reason => {}
Description
: The executor
will
be called synchronously inside
the Promise
, and the asynchronous operation will be executed in the executor
Promise.prototype.then
method
: (onResolved, onRejected) => {}
(1) onResolved
function
:
successful callback function
(value) => {}
(2) onRejected
function
:
failed callback function
(reason) => {}
Description
:
Specify the success callback for getting the success
value
and
the failure callback for getting the failure
reason
returns a new
promise
object
Promise.prototype.catch
method
: (onRejected) => {}
(1) onRejected
function
:
failed callback function
(reason) => {}
Description :
syntactic sugar of
then()
, equivalent to : then(undefined, onRejected)
Promise.resolve
method
: (value) => {}
(1) value:
successful data or
promise
object
Description
:
Returns a success
/
failure
promise
object
Promise.reject
method
: (reason) => {}
(1) reason:
the reason for the failure
Description
:
Returns a failed
promise
object
// 1.创建一个新的promise对象
const p = new Promise((resolve, reject) => {
// 执行器函数 同步回调
// 2.执行异步操作任务
setTimeout(() => {
const time = Date.now();
// 如果当前时间是偶数代表成功,否则代表失败
// 3.1 如果成功了,调用resolve(value)
if (time % 2 == 0) {
resolve("成功的数据, time=" + time);
} else {
// 3.2 如果失败了,调用reject(reason)
reject("失败的数据, time=" + time);
}
});
}, 1000);
//如果传入的参数为 非Promise类型的对象, 则返回的结果为成功promise对象
//如果传入的参数为 Promise 对象, 则参数的结果决定了 resolve 的结果
p.then(
(value) => {
// 接收得到成功的value数据
console.log("onResolved", value);
},
(reason) => {
// 接收得到失败的reason数据
console.log("onRejected", reason);
}
);
Promise.all
method
: (promises) => {}
(1) promises:
an array
containing
n
promises
Explanation
:
Return a new
promise,
only if all
promises
succeed
,
and only if one fails
fail directly
let p1 = new Promise((resolve, reject) => {
resolve('OK');
})
// let p2 = Promise.resolve('Success');
let p2 = Promise.reject('Error');
let p3 = Promise.resolve('Oh Yeah');
//
const result = Promise.all([p1, p2, p3]);
console.log(result); // Uncaught (in promise) Error
Promise.race method: (promises) => {}
(1) promises:
an array
containing
n
promises
Race means race, which means that whichever result in
Promise.race
([p1, p2, p3]) is obtained quickly, that result (only one) will be returned, regardless of whether the
result itself is in a successful state or a failed state
.
Description
:
Return a new
promise, the result state of
the first completed
promise
is the final result state
let p1 = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('success')
},1000)
})
let p2 = new Promise((resolve, reject) => {
setTimeout(() => {
reject('failed')
}, 500)
})
Promise.race([p1, p2]).then((result) => {
console.log(result)
}).catch((error) => {
console.log(error) // 打开的是 'failed'
})