draw a kite
The specific code is as follows:
import spen
spen.set_defaults(canvas=document['canvas'])
p = spen.Turtle("mouse")
p.hideturtle()
p.width(3)
p.speed(8)
#code here!
for i in range(3):
p.fd(100)
p.lt(120)
for i in range(3):
p.fd(100)
p.rt(120)
p.lt(60)
p.fd(100)
p.rt(150)
p.fd(300)
p.bk(100)
p.lt(90)
p.fd(30)
p.bk(60)
spen.done()
Result graph:
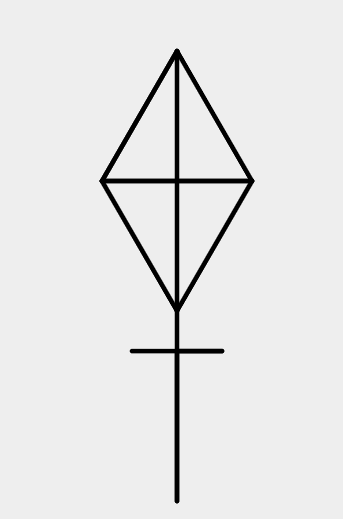
draw petals
Idea: Get petals by drawing polygon rotation.
The specific code is as follows:
import spen,random
spen.set_defaults(canvas=document['canvas'])
p = spen.Turtle("mouse")
p.hideturtle()
p.width(3)
p.speed(15)
#code here!
r=random.randint(0,255)
g=random.randint(0,255)
b=random.randint(0,255)
color='rgb('+str(r)+','+str(g)+','+str(b)+')'
p.color(color)
n=8
for i in range(12):
for i in range(n):
p.fd(30)
p.lt(360/n)
p.lt(30)
p.rt(90)
p.color('brown')
p.fd(200)
spen.done()
Result graph:
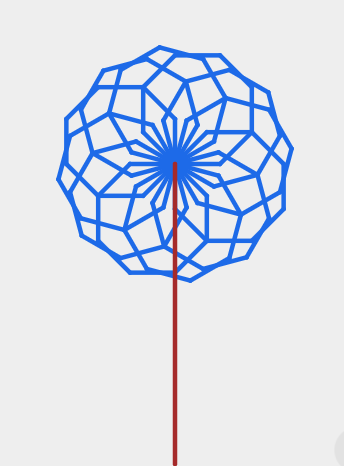
draw smiley
Idea: First draw the face, then draw the eyes and mouth.
The specific code is as follows:
import spen,random
spen.set_defaults(canvas=document['canvas'])
p = spen.Turtle("mouse")
p.hideturtle()
p.width(3)
p.speed(15)
#code here!
#绘制脸
p.color('gold')
p.fillcolor('gold')
p.begin_fill()
p.circle(60)
p.end_fill()
#绘制眼睛
p.color('black')
p.fillcolor('black')
p.up()
p.goto(-25,70)
p.down()
p.begin_fill()
p.circle(6)
p.end_fill()
p.up()
p.goto(25,70)
p.down()
p.begin_fill()
p.circle(6)
p.end_fill()
#绘制嘴巴
p.up()
p.goto(-25,45)
p.rt(60)
p.down()
p.circle(30,120)
#结束绘制
spen.done()
Result graph:
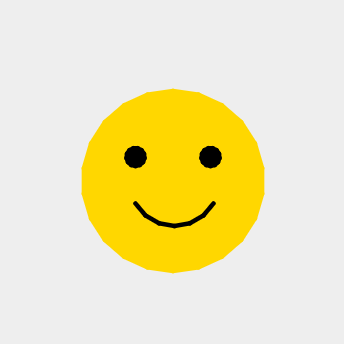
Draw a Tai Chi diagram
The specific code is as follows:
import spen,random
spen.set_defaults(canvas=document['canvas'])
p = spen.Turtle("mouse")
p.hideturtle()
p.width(3)
p.speed(5)
#code here!
p.fillcolor('black')
p.begin_fill()
p.circle(50,180)
p.circle(-50,180)
p.circle(-100,180)
p.end_fill()
p.fillcolor('white')
p.begin_fill()
p.circle(-100,180)
p.circle(-50,-180)
p.circle(50,-180)
p.end_fill()
p.up()
p.goto(-10,135)
p.fillcolor('white')
p.begin_fill()
p.down()
p.circle(15)
p.end_fill()
p.up()
p.goto(-10,35)
p.fillcolor('black')
p.begin_fill()
p.down()
p.circle(15)
p.end_fill()
spen.done()
Result graph:
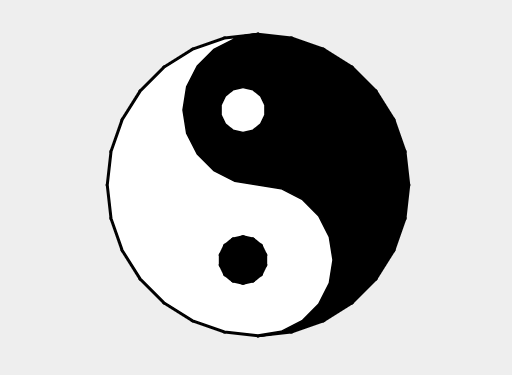
draw windmill meadow
Idea: Draw the grass first, and then draw a group of windmills.
The specific code is as follows:
import spen,random
spen.set_defaults(canvas=document['canvas'])
p = spen.Turtle("mouse")
p.hideturtle()
p.width(3)
p.speed(10)
#code here!
#绘制草地
p.up()
p.goto(-300,-110)
p.down()
p.color('green')
p.begin_fill()
for i in range(2):
p.fd(600)
p.rt(90)
p.fd(200)
p.rt(90)
p.end_fill()
#绘制风车
def fengche(color):
p.color(color)
p.fillcolor(color)
p.begin_fill()
p.circle(20,180)
p.lt(90)
p.fd(40)
p.end_fill()
color_list=['red','yellow','blue','green']
p.up()
p.goto(-160,-20)
p.down()
for i in range(4):
for j in range(4):
c=color_list[j]
fengche(c)
p.rt(90)
p.fd(140)
p.up()
p.lt(90)
p.fd(100)
p.lt(90)
p.fd(140)
p.rt(90)
p.down()
spen.done()
Result graph:
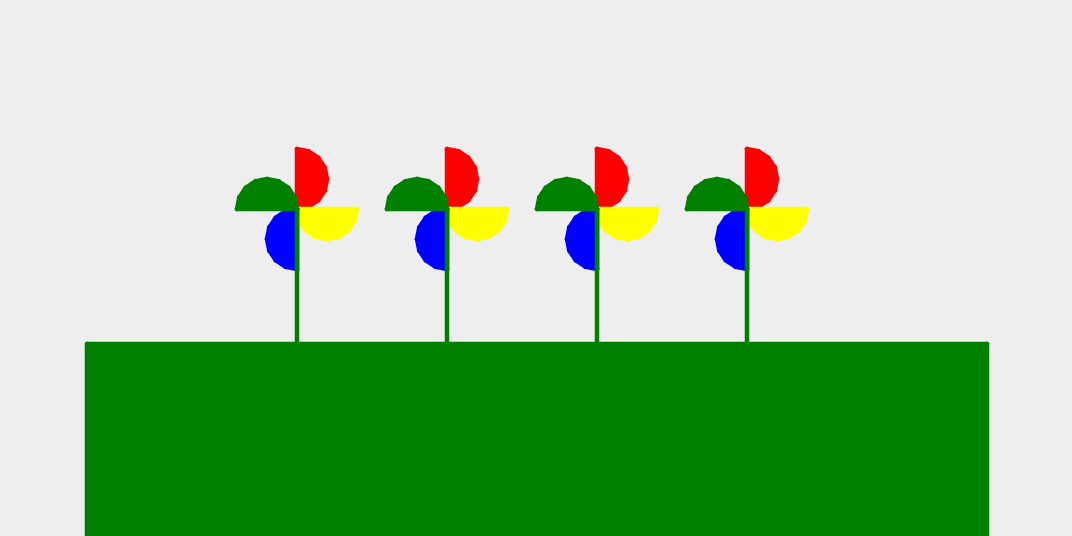
Note: The examples in this article are all based on the Aliyun Tianchi sea turtle editor. For more information, please refer to the Alibaba Cloud platform.