foreword
For a code, it may be necessary to keep the generated results during runtime, such as calculated values, filtered values, record points or the scores of small games. Normally, we want to save a piece of data, and we must open our text software and roll it by hand. Text, today's article, will take you together to teach code to write down its "memory"
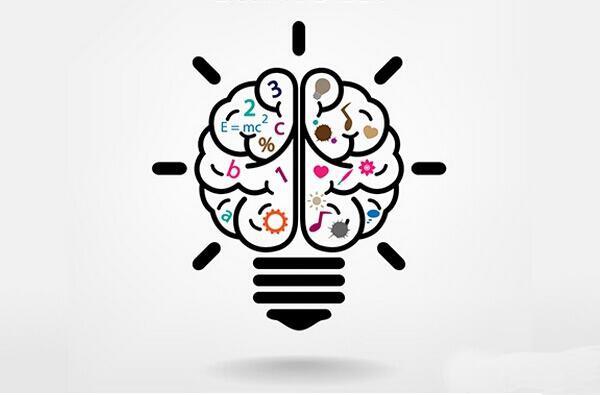
Understand the essence of the document
For text files and binary files, they actually represent a series of bytes. C language provides low-level calls to process files on storage devices, and to move bytes from a C language program, this byte stream is called data flow
A data stream (data stream) is an ordered set of data sequences with start and end bytes. Including input stream and output stream.
file read and write
When we need to create or open a file, we can use the fopen() function, and to close the file, we need to use the fclose() function
An object of type FILE will be initialized when it is created or opened, in the format:
FILE *fopen( const char *filename, const char *mode );
Among them, filename is the name of the file, and mode is the open mode, and a table is made for easy reference
mode |
Function |
r |
Open an existing text file as read-only, allowing the file to be read (an error if it does not exist) |
w |
Open the text file in the form of writing, if it does not exist, create a new file, otherwise, write from the beginning of the file and overwrite the original content |
a |
Open the text file in append mode. If it does not exist, create it. The content will be appended to the content of the existing file, and the original content will not be overwritten. |
r+ |
Open the text file for reading and writing, start reading from the beginning, and write new data to only cover the occupied space |
w+ |
Create a new file, write it, and read it from the beginning. If the file exists, the original content will disappear, and if it does not exist, create a new file |
a+ |
You can append content like a, while reading from the beginning |
wb |
Open binary file in write-only mode |
rb |
Open binary files in read-only mode |
ab |
Open binary file in append mode |
rb+ |
Read-write opens a binary file, allowing only reading and writing of data. |
rt+ |
read-write opens a text file, allowing reading and writing. |
wb+ |
read-write opens or creates a binary file, allowing both reading and writing. |
wt+ |
Read and write Open or create a text file; allow reading and writing. |
at+ |
Read-write opens a text file, allowing reading or appending data to the end of the text. |
ab+ |
Read-write opens a binary file, allowing data to be read or appended to the end of the file. |
Binary files can also be processed with r+b, w+b, a+b, etc. |
以上面的格式为例,打开或者创建一个文本文件,然后再关闭
int main()
{
FILE* zh;
f = fopen("word.txt", "w"); //格式例子
if (zh != NULL)
{
fputs("fopen example", zh);
fclose(zh);
zh=NULL;
}
return 0;
}
如果成功关闭文件,fclose( ) 函数返回零,如果关闭文件时发生错误,函数返回 EOF。这个函数实际上,会清空缓冲区中的数据,关闭文件,并释放用于该文件的所有内存。
EOF 是一个定义在头文件 stdio.h 中的常量。
C 标准库提供了各种函数来按字符或者以固定长度字符串的形式读写文件。
学会了文件的打开模式后,我们就开始书写代码的“记忆”
文件的读写函数
fgetc():读取一个字符
fputc():写入一个字符
fgets():读取一个字符串
fputs():写入一个字符串
fprintf():写入格式化数据
fscanf():格式化读取数据
fread():读取数据
fwrite():写入数据
我们可以先小试牛刀
试着写入字符进一个txt文件里
第一步:先在一个地方创建文件夹(方便存储产生的文件,这里以C盘为例,我创建了一个名为tmp的文件夹)
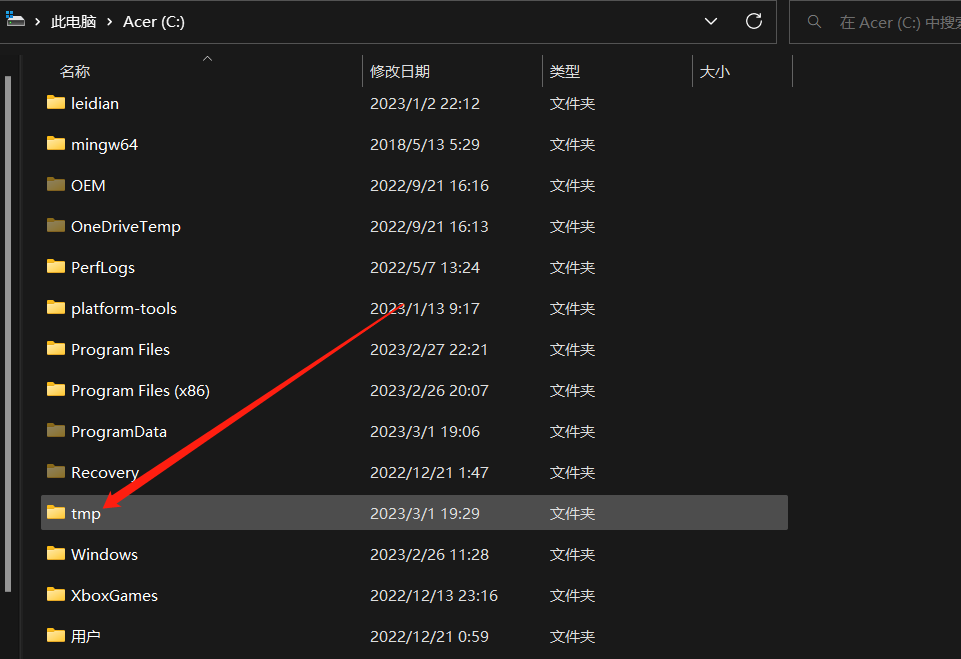
第二步:敲入我们的代码
#include <stdio.h>
int main(){
FILE *fp = NULL; //FILE *fp 是声明,声明fp是指针,用来指向FILE类型的对象。
fp = fopen("C:/tmp/1.txt","w+"); /*fopen,以w+允许读写的模式打开路径上的1.txt文件
此时没有1.txt文件,则自动新建*/
char c = 'c';
fputc(c,fp);
fprintf(fp,"\n");
fprintf(fp,"This is testing for fprintf...\n");
fputs("This is testing for fputs...\n",fp);
fclose(fp);
}
看我们的第五行代码,因为tmp文件夹是新建的,所以里面没有文件,此时我们运行代码后,文件夹里就自动生成了1.txt文件
然后使用了fputc()输入单个字符,fprintf()和fputs()写入了两行字符串
当字符多于一个时,fputc()就会报错
我们先看刚刚运行代码后的结果

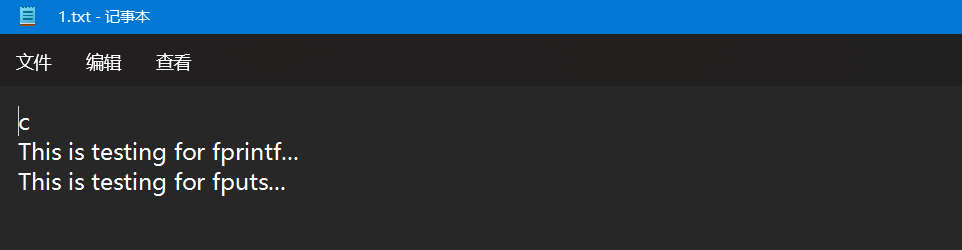
此时txt文件中就出现了我们想要的字符
如何实现回应键盘效果,将键盘上输入的字符都写入文件中,实现打字效果呢?
这里我们就可以写一个while循环体,运用getchar()每次都从键盘读取一个字符存进遍历中,然后写入文件
运用键盘往文件里写入字符(实现打字效果)
#include<stdio.h>
int main(){
FILE *fp;
char ch;
fp=fopen("C:/tmp/2.txt","wt+");
printf("输入一段字符串,回车代表终止\n");
while((ch=getchar())!='\n'){
fputc(ch,fp); //可以输入中文喔
}
fclose(fp);
return 0;
}
此时我们尝试一下运行,输入:
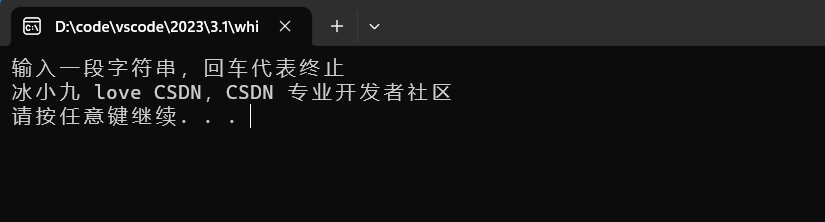
结果:
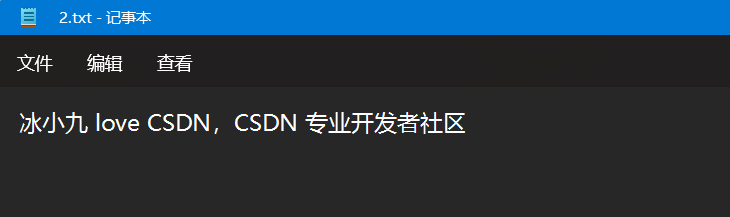
就是这么的神奇
接下来我们还可以进行一些有趣的功能
读取”记忆“
实现统计文章某个字出现频率
对于一个不需要写入的文本,我们就以r只读的方式来打开它
如果打不开,给出错误提示
运用fgetc()读取字符,通过while循环重复操作,直到无法读取到字符为止(即读完文章最后一个字符)
#include <stdio.h>
int main ()
{
FILE * fp;
int ch;
int n = 0;
fp = fopen ("C:/tmp/myfile.txt", "r");
if (fp == NULL) perror ("无法打开此文件"); // 打开失败
else
{
while (ch != EOF)
{
ch = fgetc (fp); // 获取一个字符
if (ch == 'a') n++; // 统计美元符号 'a' 在文件中出现的次数
}
fclose (fp); // 一定记得要关闭文件
printf ("a的个数为%d个。\n",n);
}
return 0;
}
我们预存一个文件,内容有这些
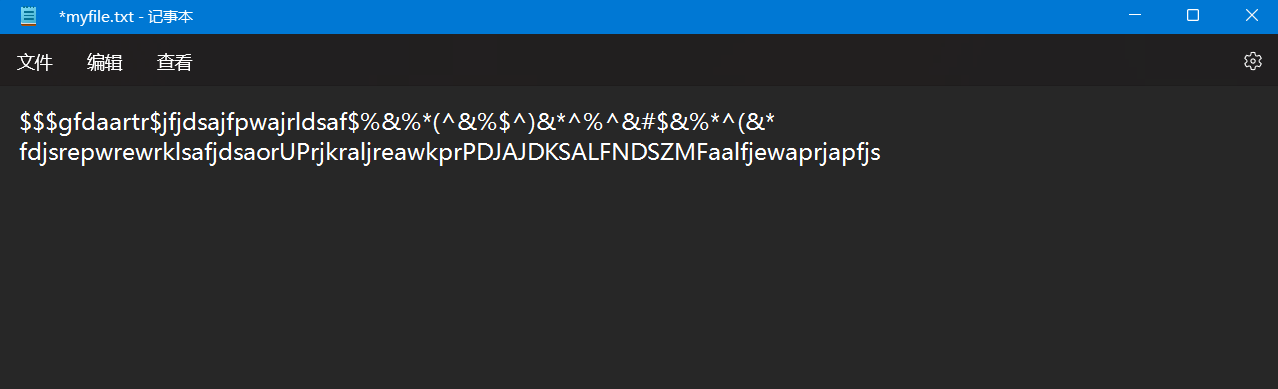
接下来我们执行上面的代码,十分简单地就把这段乱码中的”a“成员给揪出来了
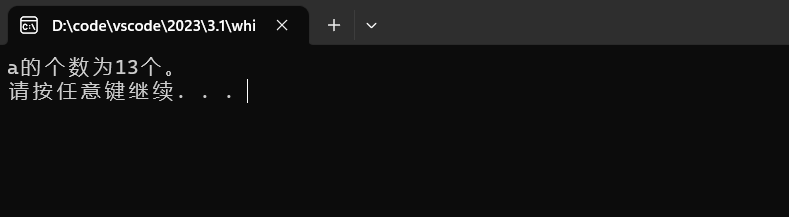