At work, we often need to parse specified parameters from the command line, and Python also provides corresponding standard libraries to do this, such as sys, optparse, getopt, argparse. The most powerful function here is argparse, let's take a look at its usage.
import argparse# 使用 argparse 分为以下几步# 1. 创建命令行解析器对象parse = argparse.ArgumentParser( description="这是命令行解析器")# 2.给解析器添加命令行参数,可以添加任意个parse.add_argument("-n", dest="name")# 3. 从命令中将参数解析出来args = parse.parse_args()# 然后通过 args 便可以拿到相应的参数值print(args.name)
Let's execute it and see that the current file is called tools.py.
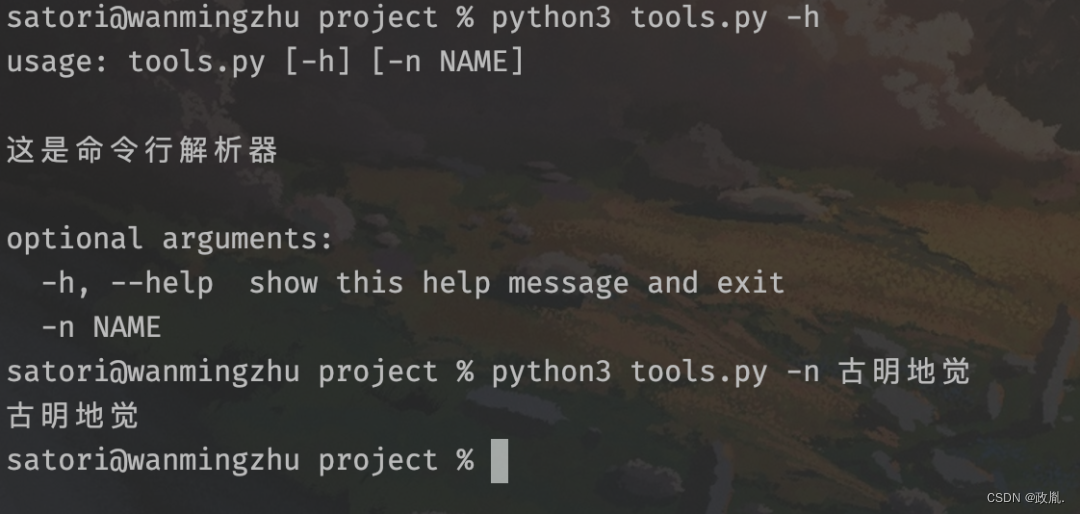
How, is not very simple? So our focus is on the add_argument method to see what parameters it supports.
import argparseparse = argparse.ArgumentParser()# 这里出现了 "-n" 和 "--name"# 在命令行中可以通过 '-n 古明地觉' 或者 '--name 古明地觉' 进行指定# 两者的含义是一样的,但 - 后面一般跟短参数,-- 后面跟长参数# 然后是 dest,它表示获取相关参数值时,使用的名称parse.add_argument("-n", "--name", dest="name")# 这里只有一个短参数,那么在命令行中需要通过 -a 来指定parse.add_argument("-a", dest="age")# 这里只有一个长参数,那么在命令行中需要通过 --gender 来指定parse.add_argument("--gender", dest="gender")args = parse.parse_args()print(f"name: {args.name}, age: {args.age}, gender: {args.gender}")
Let's try it out:

It's still very simple, one - followed by short parameters, two - followed by long parameters. Use - or -- to specify on the command line, and then obtain it through the name specified by the dest parameter after parsing.
In addition, although - is followed by short parameters and -- is followed by long parameters, it is also possible for us to write like this.
parse.add_argument("-name", "--n", dest="name")
It's just that this way of writing is weird, it should be written as --name and -n, one horizontal bar is followed by short parameters, and two are long parameters.
Then these parameters can not be specified, and the default value None will be used.

What if I want it to be a required parameter?
import argparseparse = argparse.ArgumentParser()parse.add_argument("--host", dest="host", required=True)parse.add_argument("-p", dest="port", default=6379)args = parse.parse_args()print(f"host: {args.host}, port: {args.port}")
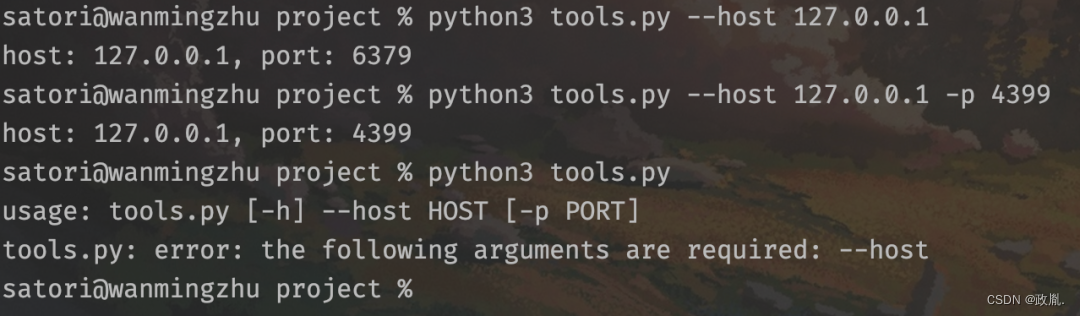
We see that when the host is not specified, an error will be reported. And we also specified the default value through the default parameter.
Then there is the type of the parameter value. No matter what the parameter is, as long as we pass it in the command line, the parsed default value is a string type.
import argparseparse = argparse.ArgumentParser()parse.add_argument("-p", dest="port", default=6379)args = parse.parse_args()print(f"port 类型: {args.port.__class__}")

So can you specify the type of the parameter? The answer is yes.
import argparseparse = argparse.ArgumentParser()parse.add_argument("-p", dest="port", type=int)args = parse.parse_args()print(f"port 类型: {args.port.__class__}")
By specifying the type as int, after parsing the parameter value, int will be called for conversion, and an error will be reported if the conversion fails.
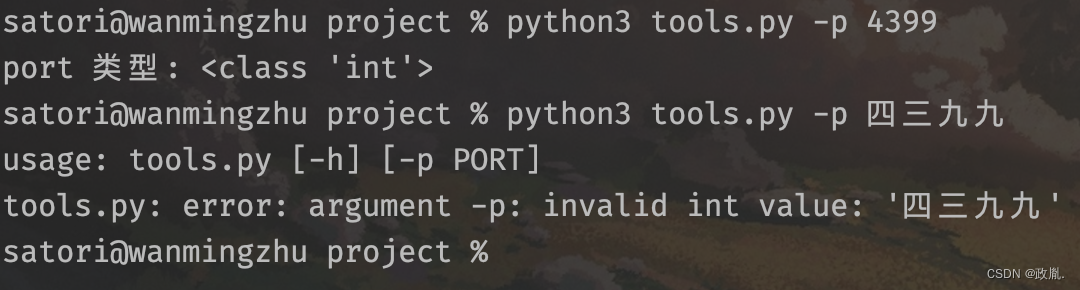
In the same way, we can also switch to other types, for example.
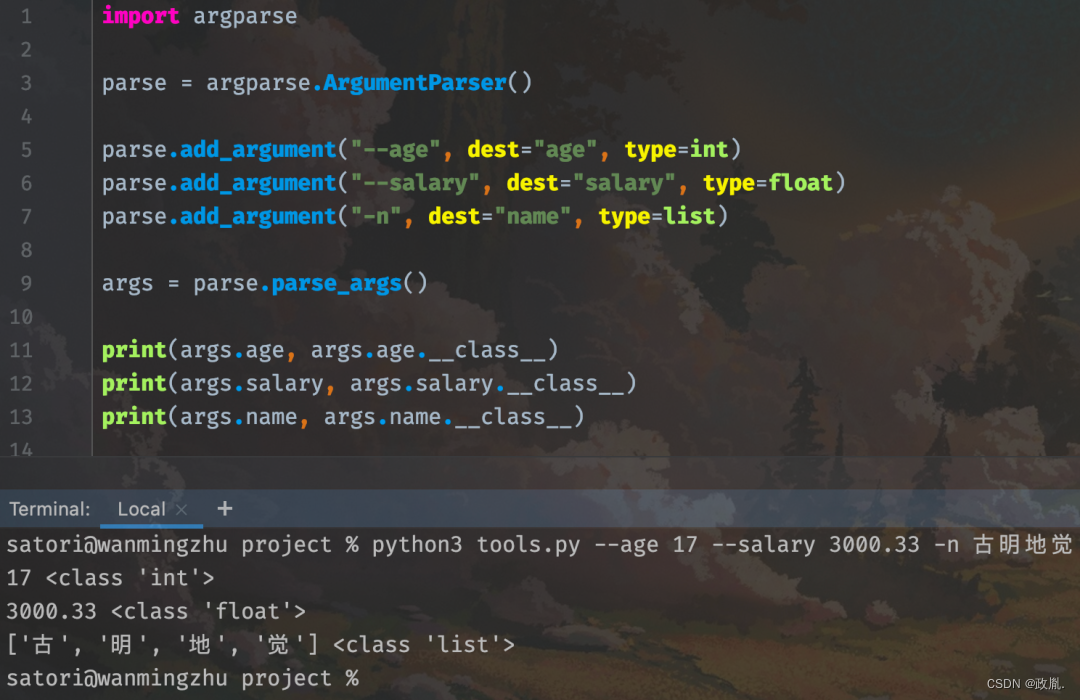
It's still very simple, even it can be replaced with our custom class, or a function,
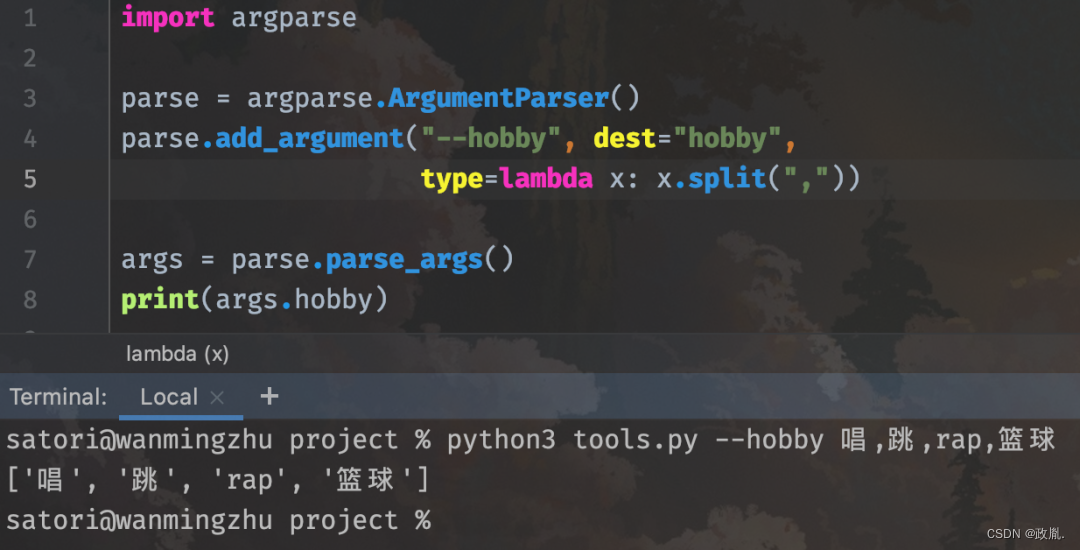
At this point, we have realized passing multiple values to one parameter, but this approach is a curve to save the country, and we should realize it through another parameter.
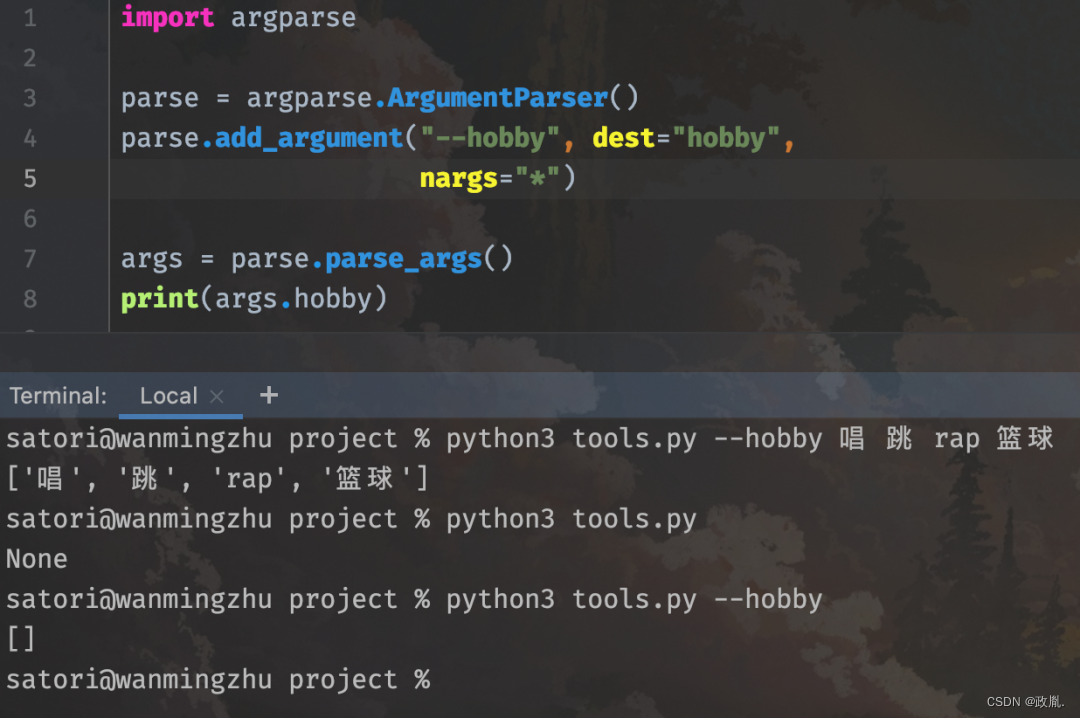
If nargs is specified as *, it means --hobby can receive multiple values, and the values are separated by spaces, and finally a list will be obtained. If you specify --hobby, but don't pass a value, you will get an empty list.
The above is the basic usage of argparse. For more information, please refer to the official documentation.