Article directory
1. Preparation
<!DOCTYPE web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"
"http://java.sun.com/dtd/web-app_2_3.dtd" >
<web-app>
<display-name>Archetype Created Web Application</display-name>
</web-app>
3) Introduce dependencies (Servlet, Jackson)
2. Agreed front-end and back-end interaction interface
The so-called " front-end and back-end interaction interface " is a key link in web development.
In order to complete the front-end and back-end interaction, two interactive interfaces should be agreed:
1. Get all messages from the server:
Request : GET /message
Response : JSON format
{
{from:"xxx" ,to:"xxx", message:"xxxxxx"}
............
.............
}
2. Submit data to the server
Request : body is also in JSON format
POST/message
{from:"xxx" ,to:"xxx", message:"xxxxxx"}
Response : JSON format
{ok:1}
3. Implement server-side code
//这个类表示一条消息的数据格式
class Message{
public String from;
public String to;
public String message;
}
@WebServlet("/message")
public class MessageServlet extends HttpServlet {
// 用于转换 JSON 字符串
private ObjectMapper objectMapper = new ObjectMapper();
// 用于保存所有的留言
private List<Message> messageList = new ArrayList<>();
//doGet方法用来从服务器上获取消息
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
resp.setContentType("application/json;charset=utf-8");
//writeValue就是把messageList对象转换成json格式的字符串,并通过写入响应(resp.getWriter())返回
objectMapper.writeValue(resp.getWriter(),messageList);
}
//doPost方法用来把客户端的数据提交到服务器
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//readValue第一个参数可以支持一个字符串,也可以放inputStream对象;第二个参数是用来接收读取到的结果
//返回值放到Message对象中
//通过这个代码就完成了读取body,并且解析成json的过程
Message message = objectMapper.readValue(req.getInputStream(),Message.class);
messageList.add(message);
resp.setContentType("application/json;charset=utf-8");
resp.getWriter().write("{\"ok\":1}");
}
}
Fourth, adjust the front-end page code
<script src="https://lib.baomitu.com/jquery/1.12.4/jquery.min.js"></script>
<script>
//1.在页面加载的时候访问服务器,从服务器这边获取到消息列表,并展示出来
function load(){
$.ajax({
type:'GET',
url:'message',
success: function(data,status){
let container = document.querySelector('.container');
for(let message of data){
let row = document.createElement('div');
row.className = 'row';
row.innerHTML = message.from + '对' + message.to + '说: ' + message.message;
container.appendChild(row);
}
}
});
}
load();
//2.点击提交按钮的时候,把当前的数据构造成一个http请求,发送给服务器
let submitButon = document.querySelector('#submit');
submitButon.onclick = function(){
//1.先获取到编辑框中的内容
let edits = document.querySelectorAll('.edit');
console.log(edits);
let from = edits[0].value;
let to = edits[1].value;
let message = edits[2].value;
console.log(from+'对'+to+'说,'+message);
if(from == '' || to == '' || message == ''){
return;
}
//2.根据内容构造html元素(.row里面包含用户输入的话
let row = document.createElement('div');
row.className = 'row';
row.innerHTML = from+'对'+to+'说,'+message;
//3.把这个元素添加到DOM树上
let container = document.querySelector('.container');
container.appendChild(row);
//4.清空原来的输入框
for(let i=0; i<edits.length; i++){
edits[i].value = '';
}
//5.构造成一个http请求,发送给服务器
$.ajax({
type:'POST',
url:'message',
//data里面就是body数据
data: JSON.stringify({from:from, to:to, message:message}),
contentType: "application/json;charset=utf-8",
success: function(data,status){
if(data.ok == 1){
console.log('提交成功');
}else{
console.log('提交失败');
}
}
})
}
</script>
The data is now stored in the server's memory ( private List<Message> messages = new ArrayList<Message>(); ), and once the server restarts, the data will still be lost.
Five, data storage file
In the above code, we save the data in the variable messageList. If we want to put the data in a file for persistent storage, this variable is not needed.
FileWriter fileWriter = new FileWriter(filePath,true)
There are three main ways to open files in java:
1. Open in read mode (when using an input stream object)
2. Open the write mode (when using the output stream object) This method will clear the original content
3. The additional writing method is opened (when using the output stream object), this method will not clear the original content, but splicing directly behind the file content. Add true after it to append the write state.
The complete code of data storage file is as follows:
class Message{
public String from;
public String to;
public String message;
}
@WebServlet("/message")
public class MessageServlet extends HttpServlet {
// 用于转换 JSON 字符串
private ObjectMapper objectMapper = new ObjectMapper();
// 用于保存所有的留言
// private List<Message> messageList = new ArrayList<>();
//保存文件的路径
private String filePath = "d:code/java/messageWall924/messages924.txt";
//doGet方法用来从服务器上获取消息
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
resp.setContentType("application/json;charset=utf-8");
//writeValue就是把messageList对象转换成json格式的字符串,并通过写入响应(resp.getWriter())返回
List<Message> messageList = load();
objectMapper.writeValue(resp.getWriter(),messageList);
}
private List<Message> load(){
//把读到的数据放到List<Message>中
List<Message> messageList = new ArrayList<>();
System.out.println("开始从文件加载数据!");
//此处需要按行读取,FileReader不支持,需要套上一层BufferedReader
try(BufferedReader bufferedReader = new BufferedReader(new FileReader(filePath))){
while (true){
String line = bufferedReader.readLine();
if (line == null){
break;
}
//读取到的内容,就解析成Message对象
String[] tokens = line.split("\t");
Message message = new Message();
message.from = tokens[0];
message.to = tokens[1];
message.message = tokens[2];
messageList.add(message);
}
}catch (IOException e){
e.printStackTrace();
}
return messageList;
}
//doPost方法用来把客户端的数据提交到服务器
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//readValue第一个参数可以支持一个字符串,也可以放inputStream对象;第二个参数是用来接收读取到的结果
//返回值放到Message对象中
//通过这个代码就完成了读取body,并且解析成json的过程
Message message = objectMapper.readValue(req.getInputStream(),Message.class);
//这里进行一个写文件操作
save(message);
resp.setContentType("application/json;charset=utf-8");
resp.getWriter().write("{\"ok\":1}");
}
private void save(Message message){
System.out.println("数据开始写入文件");
try(FileWriter fileWriter = new FileWriter(filePath,true)){
fileWriter.write(message.from + '\t' + message.to +
'\t' + message.message + '\n');
}catch (IOException e){
e.printStackTrace();
}
}
}
6. Data is stored in the database
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
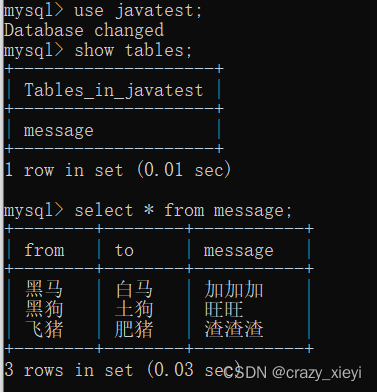
3) Create DBUtil class
public class DBUtil {
private static final String URL = "jdbc:mysql://127.0.0.1:3306/messageWall924?characterEncoding=utf8&useSSL=false";
private static final String USERNAME = "root";
private static final String PASSWORD = "1234";
private static volatile DataSource dataSource = null;
public static DataSource getDataSource(){
if (dataSource == null){
synchronized (DBUtil.class){
if (dataSource == null){
dataSource = new MysqlDataSource();
((MysqlDataSource)dataSource).setURL(URL);
((MysqlDataSource)dataSource).setUser(USERNAME);
((MysqlDataSource)dataSource).setPassword(PASSWORD);
}
}
}
return dataSource;
}
public static Connection getConnection() throws SQLException {
return getDataSource().getConnection();
}
public static void close(Connection connection, PreparedStatement statement, ResultSet resultSet){
if (resultSet != null){
try {
resultSet.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (statement != null){
try {
statement.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (connection != null){
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//readValue第一个参数可以支持一个字符串,也可以放inputStream对象;第二个参数是用来接收读取到的结果
//返回值放到Message对象中
//通过这个代码就完成了读取body,并且解析成json的过程
Message message = objectMapper.readValue(req.getInputStream(),Message.class);
//这里进行一个写数据操作
save(message);
resp.setContentType("application/json;charset=utf-8");
resp.getWriter().write("{\"ok\":1}");
}
private void save(Message message){
System.out.println("向数据库写入数据!");
//1.先和数据库建立连接
Connection connection = null;
PreparedStatement statement = null;
try {
//1.先和数据库建立连接
connection = DBUtil.getConnection();
//2.拼装sql
String sql = "insert into message values(?,?,?)";
statement = connection.prepareStatement(sql);
statement.setString(1,message.from);
statement.setString(2,message.to);
statement.setString(3,message.message);
//执行sql
int ret = statement.executeUpdate();
if (ret == 1){
System.out.println("插入数据库成功");
}else {
System.out.println("插入数据库失败");
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
DBUtil.close(connection,statement,null);
}
}
//doGet方法用来从服务器上获取消息
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
resp.setContentType("application/json;charset=utf-8");
//writeValue就是把messageList对象转换成json格式的字符串,并通过写入响应(resp.getWriter())返回
List<Message> messageList = load();
objectMapper.writeValue(resp.getWriter(),messageList);
}
private List<Message> load(){
//把读到的数据放到List<Message>中
List<Message> messageList = new ArrayList<>();
System.out.println("从数据库开始读取数据!");
Connection connection = null;
PreparedStatement statement = null;
ResultSet resultSet = null;
try {
connection = DBUtil.getConnection();
String sql = "select * from message";
statement = connection.prepareStatement(sql);
resultSet = statement.executeQuery();
while (resultSet.next()){
Message message = new Message();
message.from = resultSet.getString("from");
message.to = resultSet.getString("to");
message.message = resultSet.getString("message");
messageList.add(message);
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
DBUtil.close(connection,statement,resultSet);
}
return messageList;
}