When making mutual calls between Swift and C, it is necessary to first understand the type conversion relationship between the two languages:
Type C | Swift type |
bool | CBool |
char, signed char | CChar |
unsigned char | CUnsignedChar |
short | CShort |
unsigned short | CUnsignedShort |
int | CInt |
unsigned int | CUnsignedInt |
long | CLong |
unsigned long | CUnsignedLong |
long long | CLongLong |
unsigned long long | CUnsignedLongLong |
wchar_t | CWideChar |
char16_t | CChar16 |
char32_t | CChar32 |
float | CFloat |
double | CDouble |
Let's first demonstrate how to call C methods in Swift and create a Swift project:
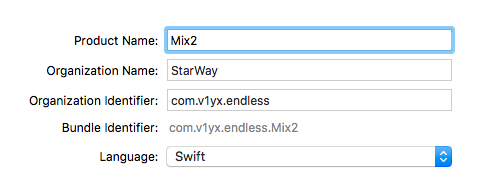
Create a C code file in the project:

At this time, you will be prompted whether you want to generate a Bridging Header, select Create.
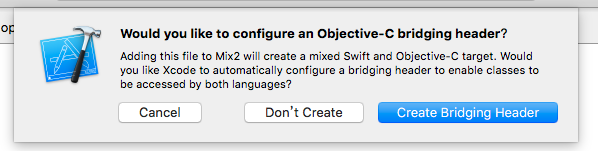
The code structure after the file is created is as follows, you can see that there is a file named: <project name>-Bridging-Header.h, which is the file for bridging between Swift and C.
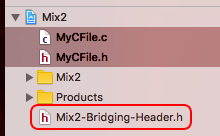
In the configuration of the project, you can see that the bridge file is specified here, so if you forget to create this file, don't worry, you can create a new one and specify it here.

Code to implement C:
MyCFile.h
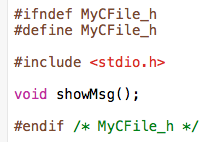
MyCFile.c
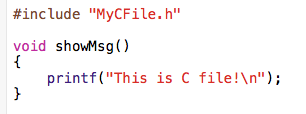
Introducing the header file of the C code in the bridging file is very simple, just import it directly.
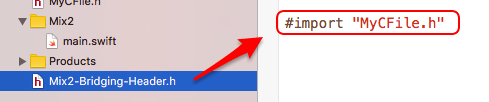
Then you can directly call the C method in the swift file:
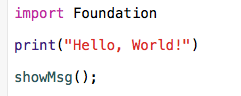
Now let's add some code to achieve two things:
1. Calling Swift code in C code
2. Mutual transfer of data (take strings as an example)
MyCFile.h
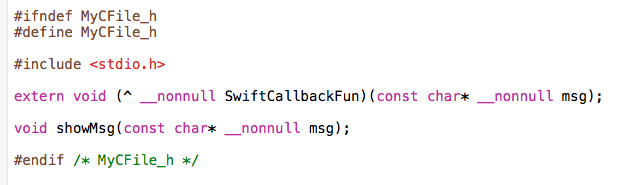
C calls to Swift methods are actually equivalent to registering a global function pointer, see the definition of SwiftCallbackFun.
There are some OC syntax in this:
The ^ operator indicates that this is a closure, that is, a Block. Functions in Swift are passed in in the form of closures.
__nonnull means that the object should not be empty, because the Swift compiler does not know whether an Objective-C object is optional or non-optional when mixing, so Xcode 6.3 introduces a new Objective-C feature: nullability annotations , here can be defined as __nullable or __nonnull.
MyCFile.c
Here is a callback to a Swift method.
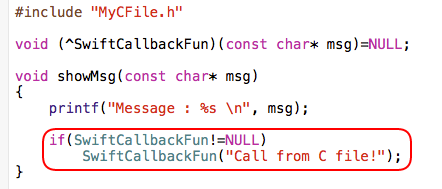
main.swift
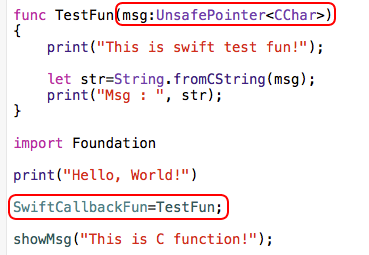
There are two points to note in the code in Swift:
1. How to handle incoming strings in C in Swift
Second, assign a value to the global Callback first, so that the method in Swift can be called from C.