. The execution environment of the Javascript language is "single thread" (single thread)
. The so-called "single thread" means that only one task can be completed at a time. If there are multiple tasks, it must be queued up, the previous task is completed, the next task is executed, and so on.
Advantages : It is relatively simple to implement, and the execution environment is relatively simple.
Disadvantages: As long as one task takes a long time, the following tasks are Having to wait in line will delay the execution of the entire program. Common browsers do not respond (feign death), often because a certain piece of Javascript code runs for a long time (such as an infinite loop), causing the entire page to get stuck in this place, and other tasks cannot be executed.
To solve this problem, the Javascript language changes the execution mode of the task. Divided into two types: Synchronous and Asynchronous
"Synchronous mode" means that the next task waits for the previous task to finish before executing, and the execution order of the program is consistent and synchronous with the arrangement order of the tasks; "Asynchronous mode" It is completely different. Each task has one or more callback functions. After the previous task ends, it does not execute the latter task, but executes the callback function. The latter task is executed without waiting for the end of the previous task. Therefore, the execution order of the program is inconsistent with the arrangement order of the tasks, and the asynchronous
"asynchronous mode" is very important. On the browser side, operations that take a long time should be performed asynchronously to avoid the browser becoming unresponsive. The best example is Ajax operations. On the server side, "async mode" is even the only mode, because the execution environment is single-threaded, and if you allow all http requests to be executed synchronously, the server performance will drop dramatically and will quickly become unresponsive
"
When writing asynchronous functions, don't use "return" to return values. You must return values through
callback
This is the most basic method of asynchronous programming. Suppose there are two functions f1 and f2, the latter waiting for the execution result of the former
f1(); f2();
click and drag to move
If f1 is a time-consuming task, consider rewriting f1 and writing f2 as f1 Callback function
var func1=function(callback){ console.log(1); (callback && typeof(callback)==='function') && callback(); } func1(func2); var func2=function(){ console .log(2); } function f1(callback){ setTimeout(function () { // f1's task code callback(); }, 1000); } f1(f2);
click and drag to move
in this way , we turn the synchronous operation into an asynchronous operation, f1 will not block the running of the program, which is equivalent to executing the main logic of the program first, and delaying the execution of time-consuming operations. The most common form of
asynchronous callback is Ajax.
In this way, we Turning a synchronous operation into an asynchronous operation, f1 will not block the running of the program, which is equivalent to executing the main logic of the program first and delaying the execution of time-consuming operations.
The advantage of the callback function is that it is simple, easy to understand and deploy, but the disadvantage is that it is not conducive to the reading and maintenance of the code. The task can only specify one callback function
, using event-driven mode (event monitoring)
Another idea is to use an event-driven model. The execution of tasks does not depend on the order of the code, but on whether an event occurs or not. The decoupling of the code is achieved
through the event mechanism. js is the event mechanism used to handle DOM interaction. We are just implementing some custom events here. Custom events are already well supported in JS
First , bind an event to f1 (using jQuery here)
f1.on('done', f2);
click and drag to move the meaning of the
above line of code Yes, when a done event occurs in f1, f2 is executed. Then, rewrite f1:
function f1(){ setTimeout(function () { // f1's task code f1.trigger('done'); //trigger() method triggers the specified event type of the selected element}, 1000 ); }
Click and drag to move
f1.trigger('done') to indicate that after the execution is completed, the done event is triggered immediately to start executing f2
. The advantage of this method is that it is easier to understand and can bind multiple events. An event can specify multiple callback functions, and can be "decoupling" (Decoupling), which is conducive to the realization of modularization. The disadvantage is that the entire program has to become event-driven, and the running process will become very unclear.
Observer mode (publish/subscribe mode)
We assume that there is a "signal center", and a task will be "published to the signal center" when it is completed. "(publish) a signal, other tasks can "subscribe" to the signal center to know when they can start executing. This is called the "publish-subscribe pattern", also known as "
First, f2 subscribes "done" signal to "signal center" jQuery
jQuery.subscribe("done", f2);
click and drag to move
Then , f1 is rewritten as follows:
function f1(){ setTimeout(function () { / / The task code of f1 jQuery.publish("done"); }, 1000); }
Click and drag to move
jQuery.publish("done") means that after f1 is executed, publish to "signal center" jQuery "done" signal, which triggers the execution of f2. In addition, after f2 is executed, you can also unsubscribe (unsubscribe)
Original
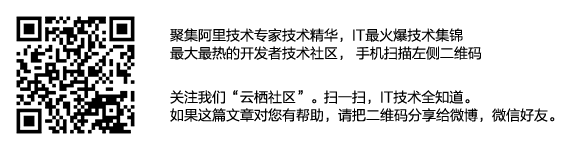
link http://click.aliyun.com/m/24027/