Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 101412 | Accepted: 22561 |
Description
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
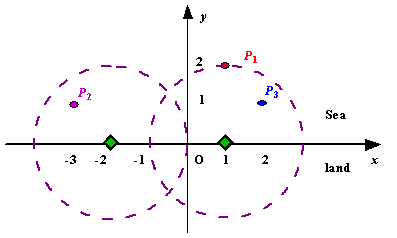
Figure A Sample Input of Radar Installations
Input
The input is terminated by a line containing pair of zeros
Output
Sample Input
3 2 1 2 -3 1 2 1 1 2 0 2 0 0
Sample Output
Case 1: 2 Case 2: 1
Idea: Be greedy, build radar from left to right, and cover as many islands as possible. Draw a circle with the island as the center and d as the radius. If the drawn circle has no intersection with the x-axis, it cannot be achieved. If there is an intersection, the coordinates of the intersection of the i-th island and the x-axis are calculated and stored in the structure arrays rad[i].sta and rad[i].end. Sort the structure array in ascending order according to rad[i].end, and then look for the radar from left to right at a time. For rad[i].end is the left coordinate of the current rightmost, for the next island, if rad[j].sta<ran[i].end, then there is no need to build a new radar, and the next island needs to be updated. Otherwise a new radar needs to be built.
#include<iostream> #include<cstdio> #include<algorithm> #include<cstring> #include<cmath> using namespace std; struct island { int x; int y; }isl[1005];//Island coordinates struct range { float sta; float end; }ran[1005];//With the island as the center and d as the radius, make the intersection area of the circle and the x-axis bool cmp(range a,range b) { return a.end<b.end; } intmain() { int n,d,t=1; while(cin>>n>>d&&n!=0) { int i,j,maxy=0; for(i=0;i<n;i++) { cin>>isl[i].x>>isl[i].y; if(isl[i].y>maxy) maxy=isl[i].y; } cout<<"Case "<<t++<<": "; if(maxy>d||d<0) { cout<<"-1"<<endl; continue; } float len; for(int i=0;i<n;i++)//求小岛对应雷达的可能覆盖范围 { len=sqrt(1.0*d*d-isl[i].y*isl[i].y); ran[i].sta=isl[i].x-len; ran[i].end=isl[i].x+len; } sort(ran,ran+n,cmp);//根据ran的end值进行排序 int ans=0; bool vis[1005];memset(vis,false,sizeof(vis)); for(int i=0;i<n;i++) {//类似的活动选择 if(!vis[i]) { vis[i]=true; for(j=0;j<n;j++) { if(!vis[j]&&ran[j].sta<=ran[i].end) vis[j]=true; } ans++; } } cout<<ans<<endl; } return 0; }