1. File operations
(1) Write the file by character
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
void main()
{
int i = 0;
FILE *fp = NULL;
char *filename2 = "c:/2.txt";
char a[27] = "dsadsadefsfdggsfadqw";
fp = fopen(filename2, "r+"); //读写的方式,打开文件 如果文件不存在,则报错
if (fp == NULL)
{
printf("func fopen() err:%d \n");
return;
}
printf("打开成功\n");
for (i = 0; i < strlen(a); i++)
{
fputc(a[i], fp);
}
printf("写入成功\n");
fclose(fp);
printf("hello...\n");
system("pause");
return;
}
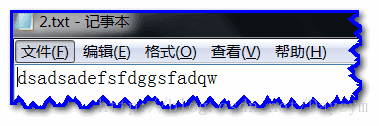
(2) Read the file by character
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
void main()
{
int i = 0;
FILE *fp = NULL;
char *filename2 = "c:/2.txt";
fp = fopen(filename2, "r+");//读写的方式,打开文件 如果文件不存在,则报错
if (fp == NULL)
{
printf("func fopen() err:%d \n");
return;
}
printf("打开成功\n");
while (!feof(fp))
{
char tempc = fgetc(fp);
printf("%c", tempc);
}
printf("\n读取成功\n");
if (fp != NULL)
{
fclose(fp);
}
printf("hello...\n");
system("pause");
return;
}
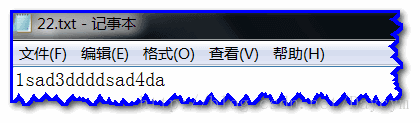
(4) Read the file line by line
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
void main()
{
int i = 0;
FILE *fp = NULL;
char *filename2 = "c:/22.txt";
char buf[1024];
fp = fopen(filename2, "r+");
if (fp == NULL)
{
printf("func fopen() err:%d \n");
return;
}
printf("打开成功\n");
while (!feof(fp))
{
char *p = fgets(buf, 1024, fp); //C 函数库会 一行一行的copy数据到buf指针所指的内存空间中并且变成C风格的字符串
if (p == NULL)
{
goto End;
}
printf("%s", buf);
}
End:
if (fp != NULL)
{
fclose(fp);
}
printf("读取成功\n");
fclose(fp);
printf("hello...\n");
system("pause");
return;
}
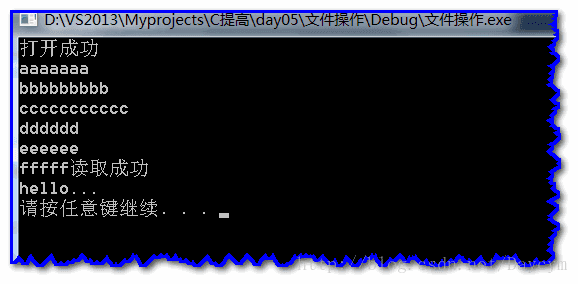
(5) Write files in blocks
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
//直接把内存数据写入到文件中
typedef struct Teacher
{
char name[64];
int age;
}Teacher;
void main()
{
int i = 0;
FILE *fp = NULL;
char *fileName = "c:/3.data";
Teacher tArray[3];
int myN = 0;
for (i = 0; i < 3; i++)
{
sprintf(tArray[i].name, "%d%d%d", i + 1, i + 1, i + 1);
tArray[i].age = i + 31;
}
fp = fopen(fileName, "wb");
if (fp == NULL)
{
printf("建立文件失败\n");
return;
}
printf("建立文件成功\n");
for (i = 0; i < 3; i++)
{
// fwrite(_In_count_x_(_Size*_Count) const void * _Str, _In_ size_t _Size, _In_ size_t _Count, _Inout_ FILE * _File);
//函数参数
//_Str : 从内存块的开始
//_Size: 写多长
//_Count: 写多少次
//_File : 写入到文件指针所指向的文件中
//函数的返回值
myN = fwrite(&tArray[i], sizeof(Teacher), 1, fp);
//myN 用于判断有没有写满磁盘
}
printf("写入成功\n");
if (fp != NULL)
{
fclose(fp);
}
printf("hello...\n");
system("pause");
return;
}
(6) Read the file in blocks
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
typedef struct Teacher
{
char name[64];
int age;
}Teacher;
void main()
{
int i = 0;
FILE *fp = NULL;
char *fileName = "c:/3.data";
Teacher tArray[3];
int myN = 0;
fp = fopen(fileName, "r+b");
if (fp == NULL)
{
printf("建立文件失败\n");
return;
}
for (i = 0; i < 3; i++)
{
// fread(_Out_bytecap_x_(_ElementSize*_Count) void * _DstBuf, _In_ size_t _ElementSize, _In_ size_t _Count, _Inout_ FILE * _File);
myN = fread(&tArray[i], sizeof(Teacher), 1, fp);
}
for (i = 0; i < 3; i++)
{
printf("name:%s, age:%d \n", tArray[i].name, tArray[i].age);
}
if (fp != NULL)
{
fclose(fp);
}
printf("hello...\n");
system("pause");
return;
}
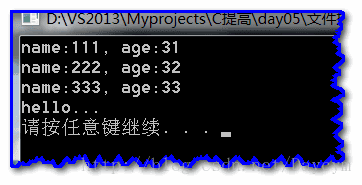
2. Configuration file read and write case
1. Requirements
- 1). Configuration file read (according to the key, read the value, and print it out)
- 2). Configuration file writing (input key, value)
- 3). Configuration file modification (input key, value)
- 4). Optimization ==> interface, module
2. Implementation steps
- 1). Write a header file my.h (write interface)
- 2). Write a source file cfg.c (to implement read and write operations)
- 3). Write a source file test.c (for testing the code)
- 4). Write a configuration file mycfg.ini
3. Header file my.h code
#ifndef __MY__
#define __MY__
#ifdef __cplusplus
extern "C" {
#endif
//获取配置项
int GetCfgItem(char *pFileName /*in*/, char *pKey /*in*/, char * pValue/*in out*/, int * pValueLen /*out*/);
//写配置项
int WriteCfgItem(char *pFileName /*in*/, char *pItemName /*in*/, char *pItemValue/*in*/, int itemValueLen /*in*/);
#ifdef __cplusplus
}
#endif
#endif
4. Source file cfg.c code
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
#define MaxLine 2048 //最大行数
//获取配置项
int GetCfgItem(char *pFileName /*in*/, char *pKey /*in*/, char * pValue/*in out*/, int * pValueLen /*out*/)
{
int ret = 0;
FILE *fp = NULL;
char *pTmp = NULL, *pEnd = NULL, *pBegin = NULL;
char lineBuf[MaxLine];
fp = fopen(pFileName, "r");
if (fp == NULL)
{
ret = -1;
return ret;
}
while (!feof(fp))
{
memset(lineBuf, 0, sizeof(lineBuf));
//fgets(_Out_z_cap_(_MaxCount) char * _Buf, _In_ int _MaxCount, _Inout_ FILE * _File);
fgets(lineBuf, MaxLine, fp);
//printf("lineBuf:%s ",lineBuf );
pTmp = strchr(lineBuf, '=');
if (pTmp == NULL) //如果此行没有=号,跳下一行
{
continue;
}
pTmp = strstr(lineBuf, pKey);
if (pTmp == NULL) //判断key是不是在所在行
{
continue;
}
pTmp = pTmp + strlen(pKey); //mykey1 = myvalude1 ==> "= myvalude1"
//可能key与=号之间有空格,此时可以直接去找=号
pTmp = strchr(pTmp, '=');
if (pTmp == NULL) //在判断有没有=号
{
continue;
}
pTmp = pTmp + 1;
//获取value起点
while (1)
{
if (*pTmp == ' ')
{
pTmp ++ ;
}
else
{
pBegin = pTmp;
if (*pBegin == '\n')
{
//没有配置value
//printf("配置项:%s 没有配置value \n", pKey);
goto End;
}
break;
}
}
//获取valude结束点
while (1)
{
if ((*pTmp == ' ' || *pTmp == '\n'))
{
break;
}
else
{
pTmp ++;
}
}
pEnd = pTmp;
//赋值,此时就去除了前后的空格
*pValueLen = pEnd-pBegin;
memcpy(pValue, pBegin, pEnd-pBegin);
}
End:
if (fp == NULL)
{
fclose(fp);
}
return 0;
}
//写配置项
//实现流程
//循环读每一行,检查key配置项是否存在 若存在修改对应value值
//若不存在,在文件末尾 添加 "key = value"
int WriteCfgItem(char *pFileName /*in*/, char *pKey /*in*/, char * pValue/*in*/, int ValueLen /*in*/)
{
int rv = 0, iTag = 0, length = 0;
FILE *fp = NULL;
char lineBuf[MaxLine];
char *pTmp = NULL, *pBegin = NULL, *pEnd = NULL;
char filebuf[1024*8] = {0};
if (pFileName==NULL || pKey==NULL || pValue==NULL)
{
rv = -1;
printf("SetCfgItem() err. param err \n");
goto End;
}
//打开文件
fp = fopen(pFileName, "r+");
if (fp == NULL)
{
rv = -2;
printf("fopen() err. \n");
}
if (fp == NULL)
{
//如果文件不存在,则建一个文件(为了友好)
fp = fopen(pFileName, "w+t");
if (fp == NULL)
{
rv = -3;
printf("fopen() err. \n");
goto End;
}
}
fseek(fp, 0L, SEEK_END); //把文件指针从0位置开始,移动到文件末尾
//获取文件长度;
length = ftell(fp);
fseek(fp, 0L, SEEK_SET);
if (length > 1024*8)
{
rv = -3;
printf("文件超过1024*8, nunsupport");
goto End;
}
while (!feof(fp))
{
//读每一行
memset(lineBuf, 0, sizeof(lineBuf));
pTmp = fgets(lineBuf, MaxLine, fp);
if (pTmp == NULL)
{
break;
}
//key关键字是否在本行
pTmp = strstr(lineBuf, pKey);
if (pTmp == NULL) //key关键字不在本行, copy到filebuf中
{
strcat(filebuf, lineBuf);
continue;
}
else //key关键字在本行中,替换旧的行,再copy到filebuf中
{
sprintf(lineBuf, "%s = %s\n", pKey, pValue);
strcat(filebuf, lineBuf);
//若存在key
iTag = 1;
}
}
//若key关键字,不存在追加
if (iTag == 0)
{
fprintf(fp, "%s = %s\n", pKey, pValue);
}
else //若key关键字,存在,则重新创建文件
{
if (fp != NULL)
{
fclose(fp);
fp = NULL; //避免野指针
}
fp = fopen(pFileName, "w+t");
if (fp == NULL)
{
rv = -4;
printf("fopen() err. \n");
goto End;
}
fputs(filebuf, fp);
}
End:
if (fp != NULL)
{
fclose(fp);
}
return rv;
}
5. Source file test.c code
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
#include "my.h"
#define CFGNAME "c:/mycfg.ini"
void mymenu()
{
printf("=============================\n");
printf("1 写配置文件\n");
printf("2 读配置文件\n");
printf("0 退出\n");
printf("=============================\n");
}
//读配置项
int TGetCfg()
{
int ret = 0;
char name[1024] = {0};
char value1[1024] = { 0 };
int vlen = 0;
printf("\n请键入key:");
scanf("%s", name);
ret = GetCfgItem(CFGNAME /*in*/, name /*in*/, value1/*in*/, &vlen);
if (ret != 0)
{
printf("func WriteCfgItem err:%d \n", ret);
return ret;
}
printf("value:%s \n", value1);
}
//写配置项
int TWriteCfg()
{
int ret = 0;
char name[1024] = {0};
char value1[1024] = {0};
printf("\n请键入key:");
scanf("%s", name);
printf("\n请键入value:");
scanf("%s", value1);
ret = WriteCfgItem(CFGNAME /*in*/, name /*in*/, value1/*in*/, strlen(value1) /*in*/);
if (ret != 0)
{
printf("func WriteCfgItem err:%d \n", ret);
return ret;
}
printf("你的输入是:%s = %s \n", name, value1);
return ret;
}
void main()
{
int choice;
for (;;)
{
//显示一个菜单
mymenu();
scanf("%d", &choice);
switch (choice)
{
case 1:
TWriteCfg();//写配置项
break;
case 2:
TGetCfg(); //读配置项
break;
case 0:
exit(0);
default:;
exit(0);
}
}
printf("hello...\n");
system("pause");
return ;
}
6. Configuration file mycfg.ini
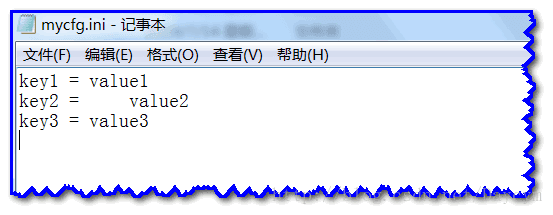
7. Test Results
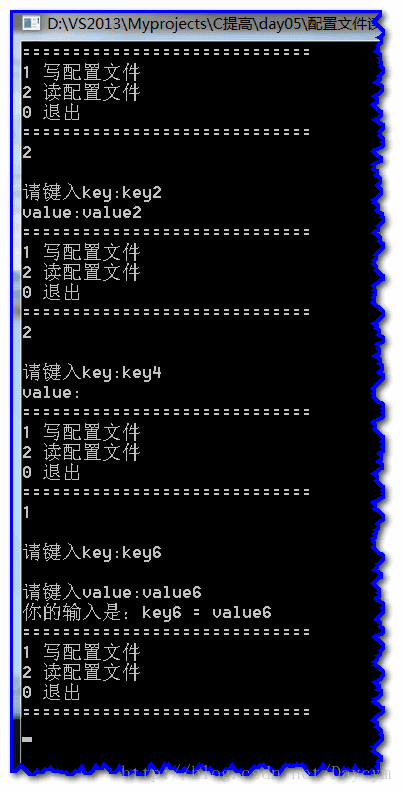
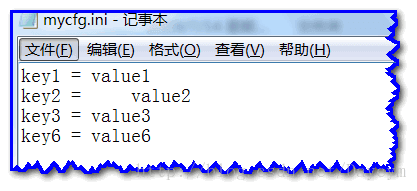